Detailed explanation of Node.js module system examples
In order to allow Node files to call each other, Node.js provides a simple module system. Modules are the basic components of Node.js applications. There is a one-to-one correspondence between files and modules. So, a Node.js file is a module. This module can be json, js or a compiled C/C++ extension.
The following is a brief introduction to the module system.
Create module
The following code simply creates a module and names it main.js. Among them, ./hello means that the hello.js file in the current directory is introduced. The default suffix of Node.js is js, so there is no need to add .js.
var hello = require('./hello');hello.world();
Node.js provides two objects for use by modules, namely require and export. Export is the public interface of the module. require is used to obtain the interface of a module from the outside, that is, to obtain the export object of the module. . Next create the hello.js file.
exports.world = function() { console.log('Hello World');}
You can see that hello.js uses the export object as the interface for external access. In main.js, the module is loaded through require to directly access the member functions of the export object. To be more advanced, if we just want to encapsulate an object into a module, we can use the following method, taking hello.js as an example.
function Hello() { var name; this.setName = function(thyName) { name = thyName; }; this.sayHello = function() { console.log('Hello ' + name); }; }; module.exports = Hello
main.js: var Hello=require('./hello');hello=new Hello();hello.setName('BYVoid'); hello.sayHello();
Execute output in the console: HelloBYVoid
require file search strategy:
Related recommendations:
Detailed explanation of Node.js module loading
Learn Nodejs with me---Node.js module
Node.js module encapsulation and usage_node.js
The above is the detailed content of Detailed explanation of Node.js module system examples. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










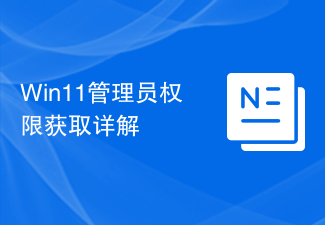
Windows operating system is one of the most popular operating systems in the world, and its new version Win11 has attracted much attention. In the Win11 system, obtaining administrator rights is an important operation. Administrator rights allow users to perform more operations and settings on the system. This article will introduce in detail how to obtain administrator permissions in Win11 system and how to effectively manage permissions. In the Win11 system, administrator rights are divided into two types: local administrator and domain administrator. A local administrator has full administrative rights to the local computer
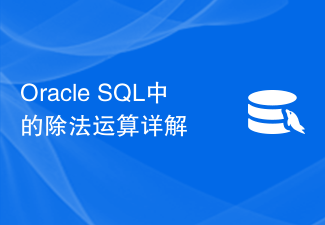
Detailed explanation of division operation in OracleSQL In OracleSQL, division operation is a common and important mathematical operation, used to calculate the result of dividing two numbers. Division is often used in database queries, so understanding the division operation and its usage in OracleSQL is one of the essential skills for database developers. This article will discuss the relevant knowledge of division operations in OracleSQL in detail and provide specific code examples for readers' reference. 1. Division operation in OracleSQL
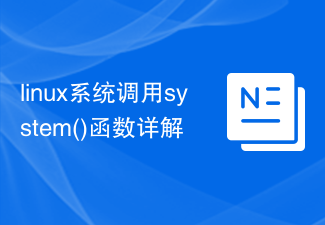
Detailed explanation of Linux system call system() function System call is a very important part of the Linux operating system. It provides a way to interact with the system kernel. Among them, the system() function is one of the commonly used system call functions. This article will introduce the use of the system() function in detail and provide corresponding code examples. Basic Concepts of System Calls System calls are a way for user programs to interact with the operating system kernel. User programs request the operating system by calling system call functions
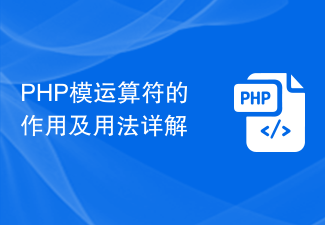
The modulo operator (%) in PHP is used to obtain the remainder of the division of two numbers. In this article, we will discuss the role and usage of the modulo operator in detail, and provide specific code examples to help readers better understand. 1. The role of the modulo operator In mathematics, when we divide an integer by another integer, we get a quotient and a remainder. For example, when we divide 10 by 3, the quotient is 3 and the remainder is 1. The modulo operator is used to obtain this remainder. 2. Usage of the modulo operator In PHP, use the % symbol to represent the modulus
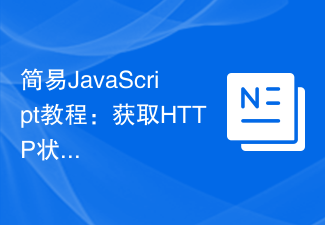
JavaScript tutorial: How to get HTTP status code, specific code examples are required. Preface: In web development, data interaction with the server is often involved. When communicating with the server, we often need to obtain the returned HTTP status code to determine whether the operation is successful, and perform corresponding processing based on different status codes. This article will teach you how to use JavaScript to obtain HTTP status codes and provide some practical code examples. Using XMLHttpRequest
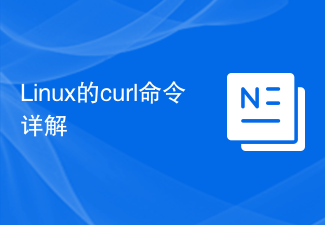
Detailed explanation of Linux's curl command Summary: curl is a powerful command line tool used for data communication with the server. This article will introduce the basic usage of the curl command and provide actual code examples to help readers better understand and apply the command. 1. What is curl? curl is a command line tool used to send and receive various network requests. It supports multiple protocols, such as HTTP, FTP, TELNET, etc., and provides rich functions, such as file upload, file download, data transmission, proxy
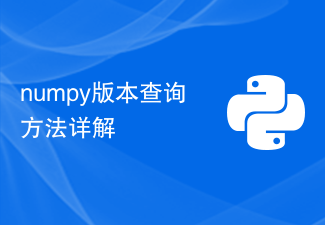
Numpy is a Python scientific computing library that provides a wealth of array operation functions and tools. When upgrading the Numpy version, you need to query the current version to ensure compatibility. This article will introduce the method of Numpy version query in detail and provide specific code examples. Method 1: Use Python code to query the Numpy version. You can easily query the Numpy version using Python code. The following is the implementation method and sample code: importnumpyasnpprint(np
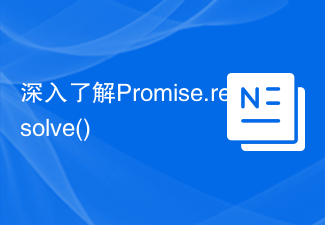
Detailed explanation of Promise.resolve() requires specific code examples. Promise is a mechanism in JavaScript for handling asynchronous operations. In actual development, it is often necessary to handle some asynchronous tasks that need to be executed in sequence, and the Promise.resolve() method is used to return a Promise object that has been fulfilled. Promise.resolve() is a static method of the Promise class, which accepts a
