Implement singly linked list using PHP
This article mainly introduces the use of PHP to implement a singly linked list, which has certain reference value. Now I share it with you. Friends in need can refer to it
Singly linked list, as the name suggests, is a linked data structure. It has a header, and except for the last node, all nodes have their successor nodes. As shown below.
First, we write the class of the linked list node. Each node in the singly linked list saves its data field and back-drive pointer
##[php] view plain copy
//链表节点 class node { public $id; //节点id public $name; //节点名称 public $next; //下一节点 public function __construct($id, $name) { $this->id = $id; $this->name = $name; $this->next = null; } }
There are two particularly important methods in the linked list, insertion and deletion. Insertion requires finding the insertion position, pointing the next pointer of the previous element to the inserted node, and pointing the next pointer of the inserted node to the next node, as shown on the left side of the figure below. Deletion points the next pointer of the previous node to the next node and returns the data content of the deleted element, as shown on the right side of the figure below.
[php] view plain copy
//单链表 class singelLinkList { private $header; //链表头节点 //构造方法 public function __construct($id = null, $name = null) { $this->header = new node ( $id, $name, null ); } //获取链表长度 public function getLinkLength() { $i = 0; $current = $this->header; while ( $current->next != null ) { $i ++; $current = $current->next; } return $i; } //添加节点数据 public function addLink($node) { $current = $this->header; while ( $current->next != null ) { if ($current->next->id > $node->id) { break; } $current = $current->next; } $node->next = $current->next; $current->next = $node; } //删除链表节点 public function delLink($id) { $current = $this->header; $flag = false; while ( $current->next != null ) { if ($current->next->id == $id) { $flag = true; break; } $current = $current->next; } if ($flag) { $current->next = $current->next->next; } else { echo "未找到id=" . $id . "的节点!<br>"; } } //判断连表是否为空 public function isEmpty(){ return $this->header == null; } //清空链表 public function clear(){ $this->header = null; } //获取链表 public function getLinkList() { $current = $this->header; if ($current->next == null) { echo ("链表为空!"); return; } while ( $current->next != null ) { echo 'id:' . $current->next->id . ' name:' . $current->next->name . "<br>"; if ($current->next->next == null) { break; } $current = $current->next; } } //获取节点名字 public function getLinkNameById($id) { $current = $this->header; if ($current->next == null) { echo "链表为空!"; return; } while ( $current->next != null ) { if ($current->id == $id) { break; } $current = $current->next; } return $current->name; } //更新节点名称 public function updateLink($id, $name) { $current = $this->header; if ($current->next == null) { echo "链表为空!"; return; } while ( $current->next != null ) { if ($current->id == $id) { break; } $current = $current->next; } return $current->name = $name; } } $lists = new singelLinkList (); $lists->addLink ( new node ( 5, 'eeeeee' ) ); $lists->addLink ( new node ( 1, 'aaaaaa' ) ); $lists->addLink ( new node ( 6, 'ffffff' ) ); $lists->addLink ( new node ( 4, 'dddddd' ) ); $lists->addLink ( new node ( 3, 'cccccc' ) ); $lists->addLink ( new node ( 2, 'bbbbbb' ) ); $lists->getLinkList (); echo "<br>-----------删除节点--------------<br>"; $lists->delLink ( 5 ); $lists->getLinkList (); echo "<br>-----------更新节点名称--------------<br>"; $lists->updateLink ( 3, "222222" ); $lists->getLinkList (); echo "<br>-----------获取节点名称--------------<br>"; echo $lists->getLinkNameById ( 5 ); echo "<br>-----------获取链表长度--------------<br>"; echo $lists->getLinkLength ();
Copy after login
相关推荐:
The above is the detailed content of Implement singly linked list using PHP. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










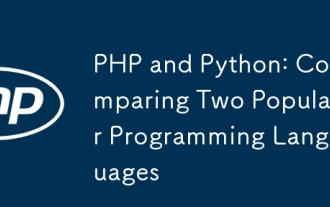
PHP and Python each have their own advantages, and choose according to project requirements. 1.PHP is suitable for web development, especially for rapid development and maintenance of websites. 2. Python is suitable for data science, machine learning and artificial intelligence, with concise syntax and suitable for beginners.
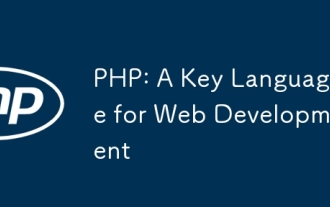
PHP is a scripting language widely used on the server side, especially suitable for web development. 1.PHP can embed HTML, process HTTP requests and responses, and supports a variety of databases. 2.PHP is used to generate dynamic web content, process form data, access databases, etc., with strong community support and open source resources. 3. PHP is an interpreted language, and the execution process includes lexical analysis, grammatical analysis, compilation and execution. 4.PHP can be combined with MySQL for advanced applications such as user registration systems. 5. When debugging PHP, you can use functions such as error_reporting() and var_dump(). 6. Optimize PHP code to use caching mechanisms, optimize database queries and use built-in functions. 7
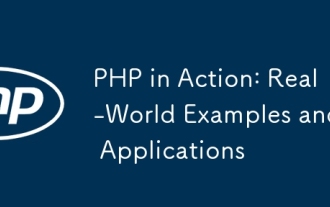
PHP is widely used in e-commerce, content management systems and API development. 1) E-commerce: used for shopping cart function and payment processing. 2) Content management system: used for dynamic content generation and user management. 3) API development: used for RESTful API development and API security. Through performance optimization and best practices, the efficiency and maintainability of PHP applications are improved.
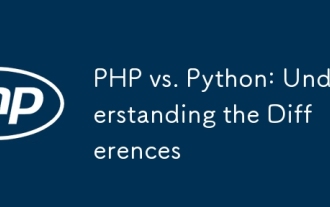
PHP and Python each have their own advantages, and the choice should be based on project requirements. 1.PHP is suitable for web development, with simple syntax and high execution efficiency. 2. Python is suitable for data science and machine learning, with concise syntax and rich libraries.
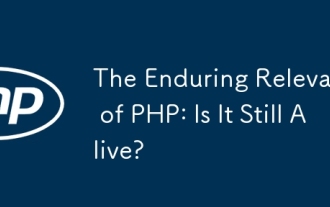
PHP is still dynamic and still occupies an important position in the field of modern programming. 1) PHP's simplicity and powerful community support make it widely used in web development; 2) Its flexibility and stability make it outstanding in handling web forms, database operations and file processing; 3) PHP is constantly evolving and optimizing, suitable for beginners and experienced developers.
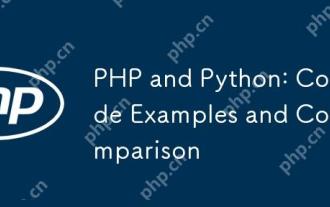
PHP and Python have their own advantages and disadvantages, and the choice depends on project needs and personal preferences. 1.PHP is suitable for rapid development and maintenance of large-scale web applications. 2. Python dominates the field of data science and machine learning.
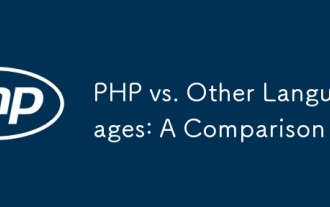
PHP is suitable for web development, especially in rapid development and processing dynamic content, but is not good at data science and enterprise-level applications. Compared with Python, PHP has more advantages in web development, but is not as good as Python in the field of data science; compared with Java, PHP performs worse in enterprise-level applications, but is more flexible in web development; compared with JavaScript, PHP is more concise in back-end development, but is not as good as JavaScript in front-end development.
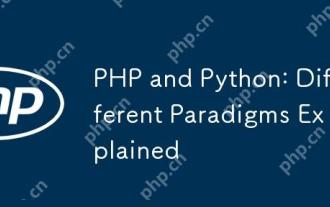
PHP is mainly procedural programming, but also supports object-oriented programming (OOP); Python supports a variety of paradigms, including OOP, functional and procedural programming. PHP is suitable for web development, and Python is suitable for a variety of applications such as data analysis and machine learning.
