PHP obtains all attributes in a class based on reflection
This article mainly introduces PHP to obtain all the methods in a class based on reflection. Combined with the example form, it analyzes in detail the steps and related precautions for PHP to obtain all properties and methods in the class using the reflection mechanism. Friends who need it You can refer to the following
The details are as follows:
When we use a class with neither source code nor documentation (especially classes provided by PHP extensions, such as mysqli, Redis classes), what should we do? Knowing what methods are provided in this class and how to use each method, it is time for the powerful reflection in PHP to appear. The following is a code demonstration using the Redis extension as an example:
<?php $ref = new ReflectionClass('Redis'); $consts = $ref->getConstants(); //返回所有常量名和值 echo "----------------consts:---------------" . PHP_EOL; foreach ($consts as $key => $val) { echo "$key : $val" . PHP_EOL; } $props = $ref->getDefaultProperties(); //返回类中所有属性 echo "--------------------props:--------------" . PHP_EOL . PHP_EOL; foreach ($props as $key => $val) { echo "$key : $val" . PHP_EOL; // 属性名和属性值 } $methods = $ref->getMethods(); //返回类中所有方法 echo "-----------------methods:---------------" . PHP_EOL . PHP_EOL; foreach ($methods as $method) { echo $method->getName() . PHP_EOL; }
Return result:
----------------consts:--------------- REDIS_NOT_FOUND : 0 REDIS_STRING : 1 REDIS_SET : 2 REDIS_LIST : 3 REDIS_ZSET : 4 REDIS_HASH : 5 ATOMIC : 0 MULTI : 1 PIPELINE : 2 OPT_SERIALIZER : 1 OPT_PREFIX : 2 OPT_READ_TIMEOUT : 3 SERIALIZER_NONE : 0 SERIALIZER_PHP : 1 OPT_SCAN : 4 SCAN_RETRY : 1 SCAN_NORETRY : 0 AFTER : after BEFORE : before --------------------props:-------------- -----------------methods:--------------- __construct __destruct connect pconnect close ping echo get set setex psetex setnx getSet randomKey renameKey renameNx getMultiple exists delete incr incrBy incrByFloat decr decrBy type append getRange setRange getBit setBit strlen getKeys sort sortAsc sortAscAlpha sortDesc sortDescAlpha lPush rPush lPushx rPushx lPop rPop blPop brPop lSize lRemove listTrim lGet lGetRange lSet lInsert sAdd sSize sRemove sMove sPop sRandMember sContains sMembers sInter sInterStore sUnion sUnionStore sDiff sDiffStore setTimeout save bgSave lastSave flushDB flushAll dbSize auth ttl pttl persist info resetStat select move bgrewriteaof slaveof object bitop bitcount bitpos mset msetnx rpoplpush brpoplpush zAdd zDelete zRange zReverseRange zRangeByScore zRevRangeByScore zRangeByLex zCount zDeleteRangeByScore zDeleteRangeByRank zCard zScore zRank zRevRank zInter zUnion zIncrBy expireAt pexpire pexpireAt hGet hSet hSetNx hDel hLen hKeys hVals hGetAll hExists hIncrBy hIncrByFloat hMset hMget multi discard exec pipeline watch unwatch publish subscribe psubscribe unsubscribe punsubscribe time eval evalsha script debug dump restore migrate getLastError clearLastError _prefix _serialize _unserialize client scan hscan zscan sscan pfadd pfcount pfmerge getOption setOption config slowlog rawCommand getHost getPort getDBNum getTimeout getReadTimeout getPersistentID getAuth isConnected getMode wait pubsub open popen lLen sGetMembers mget expire zunionstore zinterstore zRemove zRem zRemoveRangeByScore zRemRangeByScore zRemRangeByRank zSize substr rename del keys lrem ltrim lindex lrange scard srem sismember zrevrange sendEcho evaluate evaluateSha
Further, when we want to know how to use a specific method and what parameters it has, we can To further reflect this method, take the bitpos method in the above example as an example (the use of this method is not introduced in the document)
echo '---------------------params-----------------------' . PHP_EOL . PHP_EOL; $reflectMethod = $ref->getMethod('bitpos'); //传入方法名即可 echo $reflectMethod; // 会调用$reflectMethod->__toString() 返回可打印的形式;
Print results:
---------------------params----------------------- Method [ <internal:redis> public method bitpos ] { }
I didn’t see any required parameters. It may be related to the specific implementation of the method. The specific reason can only be seen in the code implementation of the redis extension. Under normal circumstances, it should be It returns the following form, taking the select_db method of mysqli as an example:
$ref = new ReflectionClass('mysqli'); echo '---------------------params-----------------------' . PHP_EOL . PHP_EOL; $reflectMethod = $ref->getMethod('select_db'); //传入方法名即可 echo $reflectMethod; // 会调用$reflectMethod->__toString() 返回可打印的形式;
---------------------params----------------------- Method [ <internal:mysqli> public method select_db ] { - Parameters [1] { Parameter #0 [ <required> $database ] } }
There is no The solution is that we can only rely on our understanding of redis and refer to similar methods to use it, such as bitop
public function bitOp( $operation, $retKey, ...$keys) {}
Post the final method call
$redis = new Redis(); $redis->connect('127.0.0.1'); $redis->setBit('bit', 15, 1); echo 'bitpos: ' . $redis->bitpos('bit', 1) . PHP_EOL; //bitpos: 15 $redis->close();
Related recommendations:
The above is the detailed content of PHP obtains all attributes in a class based on reflection. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










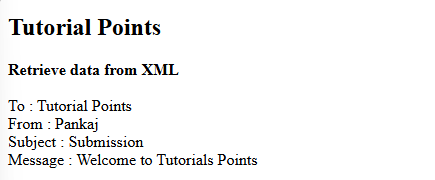
This tutorial demonstrates how to efficiently process XML documents using PHP. XML (eXtensible Markup Language) is a versatile text-based markup language designed for both human readability and machine parsing. It's commonly used for data storage an
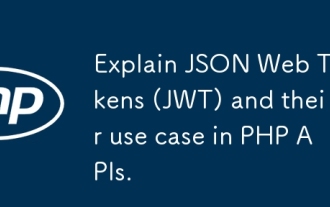
JWT is an open standard based on JSON, used to securely transmit information between parties, mainly for identity authentication and information exchange. 1. JWT consists of three parts: Header, Payload and Signature. 2. The working principle of JWT includes three steps: generating JWT, verifying JWT and parsing Payload. 3. When using JWT for authentication in PHP, JWT can be generated and verified, and user role and permission information can be included in advanced usage. 4. Common errors include signature verification failure, token expiration, and payload oversized. Debugging skills include using debugging tools and logging. 5. Performance optimization and best practices include using appropriate signature algorithms, setting validity periods reasonably,
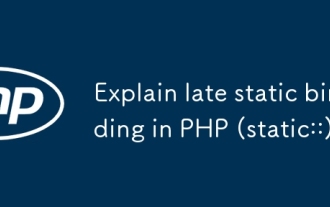
Static binding (static::) implements late static binding (LSB) in PHP, allowing calling classes to be referenced in static contexts rather than defining classes. 1) The parsing process is performed at runtime, 2) Look up the call class in the inheritance relationship, 3) It may bring performance overhead.
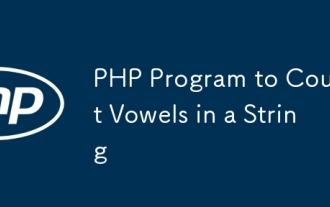
A string is a sequence of characters, including letters, numbers, and symbols. This tutorial will learn how to calculate the number of vowels in a given string in PHP using different methods. The vowels in English are a, e, i, o, u, and they can be uppercase or lowercase. What is a vowel? Vowels are alphabetic characters that represent a specific pronunciation. There are five vowels in English, including uppercase and lowercase: a, e, i, o, u Example 1 Input: String = "Tutorialspoint" Output: 6 explain The vowels in the string "Tutorialspoint" are u, o, i, a, o, i. There are 6 yuan in total

What are the magic methods of PHP? PHP's magic methods include: 1.\_\_construct, used to initialize objects; 2.\_\_destruct, used to clean up resources; 3.\_\_call, handle non-existent method calls; 4.\_\_get, implement dynamic attribute access; 5.\_\_set, implement dynamic attribute settings. These methods are automatically called in certain situations, improving code flexibility and efficiency.
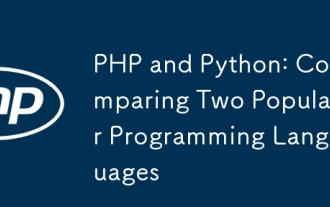
PHP and Python each have their own advantages, and choose according to project requirements. 1.PHP is suitable for web development, especially for rapid development and maintenance of websites. 2. Python is suitable for data science, machine learning and artificial intelligence, with concise syntax and suitable for beginners.
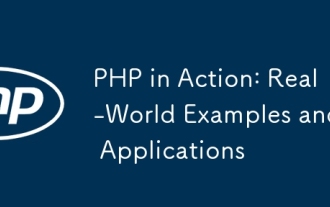
PHP is widely used in e-commerce, content management systems and API development. 1) E-commerce: used for shopping cart function and payment processing. 2) Content management system: used for dynamic content generation and user management. 3) API development: used for RESTful API development and API security. Through performance optimization and best practices, the efficiency and maintainability of PHP applications are improved.
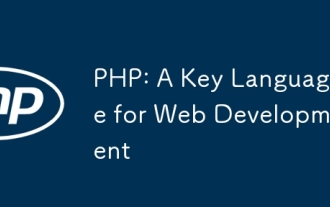
PHP is a scripting language widely used on the server side, especially suitable for web development. 1.PHP can embed HTML, process HTTP requests and responses, and supports a variety of databases. 2.PHP is used to generate dynamic web content, process form data, access databases, etc., with strong community support and open source resources. 3. PHP is an interpreted language, and the execution process includes lexical analysis, grammatical analysis, compilation and execution. 4.PHP can be combined with MySQL for advanced applications such as user registration systems. 5. When debugging PHP, you can use functions such as error_reporting() and var_dump(). 6. Optimize PHP code to use caching mechanisms, optimize database queries and use built-in functions. 7
