Code implemented by combining thinkphp with redis and queue
This article mainly introduces the implementation code of thinkphp redis queue. The editor thinks it is quite good. Now I will share it with you and give it as a reference. Let’s follow the editor to take a look.
1. Install Redis and install the corresponding redis extension according to your PHP version (this step is briefly described)
1.1. Install php_igbinary.dll. php_redis.dll extension Here you need to pay attention to your php version as shown in the figure:
1.2, add extension=php_igbinary.dll; extension=php_redis.dll to the php.ini file Extension
ok The first step of redis environment construction has been completed. Take a look at phpinfo
The actual use of redis
2.1, the first step is to configure the redis parameters as follows. The default port for redis installation is 6379:
<?php /* 数据库配置 */ return array( 'DATA_CACHE_PREFIX' => 'Redis_',//缓存前缀 'DATA_CACHE_TYPE'=>'Redis',//默认动态缓存为Redis 'DATA_CACHE_TIMEOUT' => false, 'REDIS_RW_SEPARATE' => true, //Redis读写分离 true 开启 'REDIS_HOST'=>'127.0.0.1', //redis服务器ip,多台用逗号隔开;读写分离开启时,第一台负责写,其它[随机]负责读; 'REDIS_PORT'=>'6379',//端口号 'REDIS_TIMEOUT'=>'300',//超时时间 'REDIS_PERSISTENT'=>false,//是否长连接 false=短连接 'REDIS_AUTH'=>'',//AUTH认证密码 ); ?>
2.2, redis is used in the actual function:
/** * redis连接 * @access private * @return resource * @author bieanju */ private function connectRedis(){ $redis=new \Redis(); $redis->connect(C("REDIS_HOST"),C("REDIS_PORT")); return $redis; }
2.3, the core of the flash sale The problem is that in the case of large concurrency, the purchase will not exceed the inventory. This is the key to processing, so the idea is to do some basic data generation in the flash sale category in the first step:
//现在初始化里面定义后边要使用的redis参数 public function _initialize(){ parent::_initialize(); $goods_id = I("goods_id",'0','intval'); if($goods_id){ $this->goods_id = $goods_id; $this->user_queue_key = "goods_".$goods_id."_user";//当前商品队列的用户情况 $this->goods_number_key = "goods".$goods_id;//当前商品的库存队列 } $this->user_id = $this->user_id ? $this->user_id : $_SESSION['uid']; }
2.4, the second step This is the key point. Before entering the product details page, the user first queues the inventory of the current product and stores it in redis as follows:
/** * 访问产品前先将当前产品库存队列 * @access public * @author bieanju */ public function _before_detail(){ $where['goods_id'] = $this->goods_id; $where['start_time'] = array("lt",time()); $where['end_time'] = array("gt",time()); $goods = M("goods")->where($where)->field('goods_num,start_time,end_time')->find(); !$goods && $this->error("当前秒杀已结束!"); if($goods['goods_num'] > $goods['order_num']){ $redis = $this->connectRedis(); $getUserRedis = $redis->hGetAll("{$this->user_queue_key}"); $gnRedis = $redis->llen("{$this->goods_number_key}"); /* 如果没有会员进来队列库存 */ if(!count($getUserRedis) && !$gnRedis){ for ($i = 0; $i < $goods['goods_num']; $i ++) { $redis->lpush("{$this->goods_number_key}", 1); } } $resetRedis = $redis->llen("{$this->goods_number_key}"); if(!$resetRedis){ $this->error("系统繁忙,请稍后抢购!"); } }else{ $this->error("当前产品已经秒杀完!"); } }
The next thing to do is to use ajax to asynchronously process the user's click on the purchase button to meet the conditions. The data enters the purchase queue (if the current user is not in the queue of the current product user, it enters the queue and pops an inventory queue, if it is, it throws,):
/** * 抢购商品前处理当前会员是否进入队列 * @access public * @author bieanju */ public function goods_number_queue(){ !$this->user_id && $this->ajaxReturn(array("status" => "-1","msg" => "请先登录")); $model = M("flash_sale"); $where['goods_id'] = $this->goods_id; $goods_info = $model->where($where)->find(); !$goods_info && $this->error("对不起当前商品不存在或已下架!"); /* redis 队列 */ $redis = $this->connectRedis(); /* 进入队列 */ $goods_number_key = $redis->llen("{$this->goods_number_key}"); if (!$redis->hGet("{$this->user_queue_key}", $this->user_id)) { $goods_number_key = $redis->lpop("{$this->goods_number_key}"); } if($goods_number_key){ // 判断用户是否已在队列 if (!$redis->hGet("{$this->user_queue_key}", $this->user_id)) { // 插入抢购用户信息 $userinfo = array( "user_id" => $this->user_id, "create_time" => time() ); $redis->hSet("{$this->user_queue_key}", $this->user_id, serialize($userinfo)); $this->ajaxReturn(array("status" => "1")); }else{ $modelCart = M("cart"); $condition['user_id'] = $this->user_id; $condition['goods_id'] = $this->goods_id; $condition['prom_type'] = 1; $cartlist = $modelCart->where($condition)->count(); if($cartlist > 0){ $this->ajaxReturn(array("status" => "2")); }else{ $this->ajaxReturn(array("status" => "1")); } } }else{ $this->ajaxReturn(array("status" => "-1","msg" => "系统繁忙,请重试!")); } }
Attach a debugging function to delete the specified Queue value:
public function clearRedis(){ set_time_limit(0); $redis = $this->connectRedis(); //$Rd = $redis->del("{$this->user_queue_key}"); $Rd = $redis->hDel("goods49",'用户id''); $a = $redis->hGet("goods_49_user", '用户id'); if(!$a){ dump($a); } if($Rd == 0){ exit("Redis队列已释放!"); } }
The above is the entire content of this article. I hope it will be helpful to everyone’s study. For more related content, please pay attention to the PHP Chinese website!
Related recommendations:
ThinkPHP and Ajax realize the drop-down menu of secondary linkage
##About the URL routing rules in thinkphp With static settings
The above is the detailed content of Code implemented by combining thinkphp with redis and queue. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










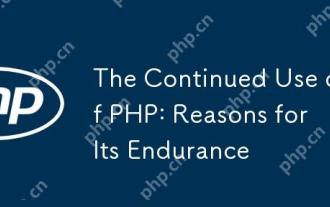
What’s still popular is the ease of use, flexibility and a strong ecosystem. 1) Ease of use and simple syntax make it the first choice for beginners. 2) Closely integrated with web development, excellent interaction with HTTP requests and database. 3) The huge ecosystem provides a wealth of tools and libraries. 4) Active community and open source nature adapts them to new needs and technology trends.
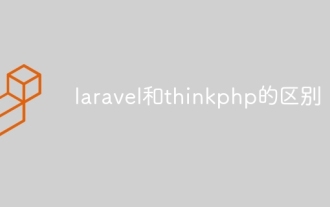
Laravel and ThinkPHP are both popular PHP frameworks and have their own advantages and disadvantages in development. This article will compare the two in depth, highlighting their architecture, features, and performance differences to help developers make informed choices based on their specific project needs.
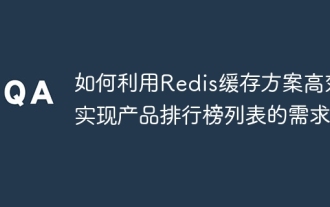
How does the Redis caching solution realize the requirements of product ranking list? During the development process, we often need to deal with the requirements of rankings, such as displaying a...
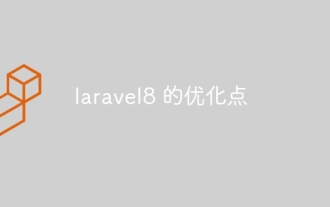
Laravel 8 provides the following options for performance optimization: Cache configuration: Use Redis to cache drivers, cache facades, cache views, and page snippets. Database optimization: establish indexing, use query scope, and use Eloquent relationships. JavaScript and CSS optimization: Use version control, merge and shrink assets, use CDN. Code optimization: Use Composer installation package, use Laravel helper functions, and follow PSR standards. Monitoring and analysis: Use Laravel Scout, use Telescope, monitor application metrics.
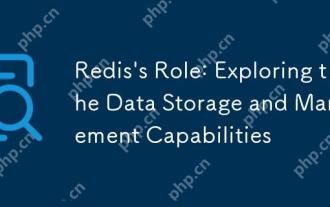
Redis plays a key role in data storage and management, and has become the core of modern applications through its multiple data structures and persistence mechanisms. 1) Redis supports data structures such as strings, lists, collections, ordered collections and hash tables, and is suitable for cache and complex business logic. 2) Through two persistence methods, RDB and AOF, Redis ensures reliable storage and rapid recovery of data.
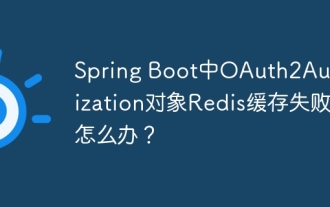
In SpringBoot, use Redis to cache OAuth2Authorization object. In SpringBoot application, use SpringSecurityOAuth2AuthorizationServer...

IIS and PHP are compatible and are implemented through FastCGI. 1.IIS forwards the .php file request to the FastCGI module through the configuration file. 2. The FastCGI module starts the PHP process to process requests to improve performance and stability. 3. In actual applications, you need to pay attention to configuration details, error debugging and performance optimization.
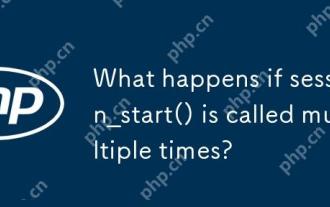
Multiple calls to session_start() will result in warning messages and possible data overwrites. 1) PHP will issue a warning, prompting that the session has been started. 2) It may cause unexpected overwriting of session data. 3) Use session_status() to check the session status to avoid repeated calls.
