


A brief discussion of PHP source code 31: Basics of the heap layer in the PHP memory pool
This article mainly introduces about PHP source code 31: The foundation of the heap layer in the PHP memory pool, which has a certain reference value. Now I share it with you. Friends in need can refer to it
A brief discussion on PHP source code 31: The basics of the heap layer in the PHP memory pool
[Overview]
PHP’s memory manager is hierarchical . This manager has three layers: storage layer, heap layer and emalloc/efree layer. The storage layer is introduced in PHP source code reading notes 30: Storage layer in PHP memory pool. The storage layer actually applies for memory to the system through functions such as malloc() and mmap(), and releases the requested memory through the free() function. Memory. The storage layer usually applies for relatively large memory blocks. The large memory applied here does not mean the memory required by the storage layer structure. It is just that when the heap layer calls the allocation method of the storage layer, the memory it applies for in the segment format is relatively large. , the role of the storage layer is to make the memory allocation method transparent to the heap layer.
Above the storage layer is the heap layer we want to learn about today. The heap layer is a scheduling layer that interacts with the emalloc/efree layer above to split the large blocks of memory applied for through the storage layer and provide them on demand. There is a set of memory scheduling strategies in the heap layer, which is the core area of the entire PHP memory allocation management.
All the following sharing is based on the situation that ZEND_DEBUG has not been opened.
First look at the structures involved in the heap layer:
[Structure]
/* mm block type */typedef struct _zend_mm_block_info { size_t _size;/* block的大小*/ size_t _prev;/* 计算前一个块有用到*/} zend_mm_block_info; typedef struct _zend_mm_block { zend_mm_block_info info;} zend_mm_block; typedef struct _zend_mm_small_free_block {/* 双向链表 */ zend_mm_block_info info; struct _zend_mm_free_block *prev_free_block;/* 前一个块 */ struct _zend_mm_free_block *next_free_block;/* 后一个块 */} zend_mm_small_free_block;/* 小的空闲块*/ typedef struct _zend_mm_free_block {/* 双向链表 + 树结构 */ zend_mm_block_info info; struct _zend_mm_free_block *prev_free_block;/* 前一个块 */ struct _zend_mm_free_block *next_free_block;/* 后一个块 */ struct _zend_mm_free_block **parent;/* 父结点 */ struct _zend_mm_free_block *child[2];/* 两个子结点*/} zend_mm_free_block; struct _zend_mm_heap { int use_zend_alloc;/* 是否使用zend内存管理器 */ void *(*_malloc)(size_t);/* 内存分配函数*/ void (*_free)(void*);/* 内存释放函数*/ void *(*_realloc)(void*, size_t); size_t free_bitmap;/* 小块空闲内存标识 */ size_t large_free_bitmap; /* 大块空闲内存标识*/ size_t block_size;/* 一次内存分配的段大小,即ZEND_MM_SEG_SIZE指定的大小,默认为ZEND_MM_SEG_SIZE (256 * 1024)*/ size_t compact_size;/* 压缩操作边界值,为ZEND_MM_COMPACT指定大小,默认为 2 * 1024 * 1024*/ zend_mm_segment *segments_list;/* 段指针列表 */ zend_mm_storage *storage;/* 所调用的存储层 */ size_t real_size;/* 堆的真实大小 */ size_t real_peak;/* 堆真实大小的峰值 */ size_t limit;/* 堆的内存边界 */ size_t size;/* 堆大小 */ size_t peak;/* 堆大小的峰值*/ size_t reserve_size;/* 备用堆大小*/ void *reserve;/* 备用堆 */ int overflow;/* 内存溢出数*/ int internal;#if ZEND_MM_CACHE unsigned int cached;/* 已缓存大小 */ zend_mm_free_block *cache[ZEND_MM_NUM_BUCKETS];/* 缓存数组/ #endif zend_mm_free_block *free_buckets[ZEND_MM_NUM_BUCKETS*2];/* 小块空闲内存数组 */ zend_mm_free_block *large_free_buckets[ZEND_MM_NUM_BUCKETS];/* 大块空闲内存数组*/ zend_mm_free_block *rest_buckets[2];/* 剩余内存数组 */ };
For the memory operation function in the heap structure, if use_zend_alloc is no, malloc-type memory allocation is used, this At this time, all operations do not go through the memory management in the heap layer, and directly use functions such as malloc.
The default size of compact_size is 2 * 1024 * 1024 (2M). If the variable ZEND_MM_COMPACT is set, the size is specified. If the peak memory exceeds this value, the compact function of storage will be called, just this function The current implementation is empty and may be added in subsequent versions.
reserve_size is the size of the reserve heap, by default it is ZEND_MM_RESERVE_SIZE, its size is (8*1024)
*reserve is the reserve heap, its size is reserve_size, it is used to report errors when memory overflows .
[About USE_ZEND_ALLOC]
The environment variable USE_ZEND_ALLOC can be used to allow selection of malloc or emalloc memory allocation at runtime. Using malloc-type memory allocation will allow external debuggers to observe memory usage, while emalloc allocation will use the Zend memory manager abstraction, requiring internal debugging.
[zend_startup() -> start_memory_manager() -> alloc_globals_ctor()]
static void alloc_globals_ctor(zend_alloc_globals *alloc_globals TSRMLS_DC){ char *tmp; alloc_globals->mm_heap = zend_mm_startup(); tmp = getenv("USE_ZEND_ALLOC"); if (tmp) { alloc_globals->mm_heap->use_zend_alloc = zend_atoi(tmp, 0); if (!alloc_globals->mm_heap->use_zend_alloc) {/* 如果不使用zend的内存管理器,同直接使用malloc函数*/ alloc_globals->mm_heap->_malloc = malloc; alloc_globals->mm_heap->_free = free; alloc_globals->mm_heap->_realloc = realloc; } }}
[Initialization]
[zend_mm_startup()]
Initialize the allocation plan of the storage layer , initialize the segment size, compression boundary value, and call zend_mm_startup_ex() to initialize the heap layer.
[zend_mm_startup() -> zend_mm_startup_ex()]
[Memory Alignment]
Memory alignment is used in PHP’s memory allocation. The memory alignment calculation obviously has two goals: one is to reduce The number of CPU memory accesses; the second is to keep the storage space efficient enough.
# define ZEND_MM_ALIGNMENT 8 #define ZEND_MM_ALIGNMENT_MASK ~(ZEND_MM_ALIGNMENT-1) #define ZEND_MM_ALIGNED_SIZE(size)(((size) + ZEND_MM_ALIGNMENT - 1) & ZEND_MM_ALIGNMENT_MASK) #define ZEND_MM_ALIGNED_HEADER_SIZEZEND_MM_ALIGNED_SIZE(sizeof(zend_mm_block)) #define ZEND_MM_ALIGNED_FREE_HEADER_SIZEZEND_MM_ALIGNED_SIZE(sizeof(zend_mm_small_free_block))
PHP uses memory alignment in the memory of allocated blocks. If the lower three digits of the required memory size are not 0 (not divisible by 8), add 7 to the lower digits. And ~7 performs an AND operation, that is, for memory sizes that are not an integer multiple of 8, the memory size is completed to be divisible by 8.
On win32 machines, the numerical sizes corresponding to some macros are:
ZEND_MM_MIN_SIZE=8
ZEND_MM_MAX_SMALL_SIZE=272
ZEND_MM_ALIGNED_HEADER_SIZE=8
ZEND_MM_ALIGNED_FREE_HEADER_SIZE=16
ZEND_MM_MIN_ALLOC_BLOCK_SIZE=8
ZEND_MM_ALIGNED_MIN_HEADER_SIZE =16
ZEND_MM_ALIGNED_SEGMENT_SIZE=8
If you want to allocate a block with a size of 9 bytes, the actual allocated size is ZEND_MM_ALIGNED_SIZE(9 8)=24
[of the block Positioning】
The two right digits of the allocated memory are used to mark the type of memory.
The definition of its size is #define ZEND_MM_TYPE_MASK ZEND_MM_LONG_CONST(0×3)
The code shown below is the positioning of the block
#define ZEND_MM_NEXT_BLOCK(b)ZEND_MM_BLOCK_AT(b, ZEND_MM_BLOCK_SIZE(b)) #define ZEND_MM_PREV_BLOCK(b)ZEND_MM_BLOCK_AT(b, -(int)((b)->info._prev & ~ZEND_MM_TYPE_MASK)) #define ZEND_MM_BLOCK_AT(blk, offset)((zend_mm_block *) (((char *) (blk))+(offset))) #define ZEND_MM_BLOCK_SIZE(b)((b)->info._size & ~ZEND_MM_TYPE_MASK)#define ZEND_MM_TYPE_MASKZEND_MM_LONG_CONST(0x3)
The next element of the current block is the current block The head position plus the length of the entire block (minus the length of the type).
The previous element of the current block is the head position of the current block minus the length of the previous block (removing the length of the type).
Regarding the length of the previous block, it is set to the result of the OR operation of the size of the current block and the block type during the initialization of the block.
The above is the entire content of this article. I hope it will be helpful to everyone's study. For more related content, please pay attention to the PHP Chinese website!
Related recommendations:
A brief discussion of PHP source code 30: The storage layer in the PHP memory pool
A brief discussion of PHP Source code twenty-nine: About the inheritance of interfaces
## A brief discussion of PHP source code twenty-eight: About the class structure and inheritance
The above is the detailed content of A brief discussion of PHP source code 31: Basics of the heap layer in the PHP memory pool. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










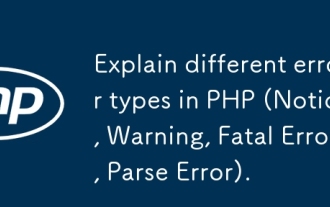
There are four main error types in PHP: 1.Notice: the slightest, will not interrupt the program, such as accessing undefined variables; 2. Warning: serious than Notice, will not terminate the program, such as containing no files; 3. FatalError: the most serious, will terminate the program, such as calling no function; 4. ParseError: syntax error, will prevent the program from being executed, such as forgetting to add the end tag.
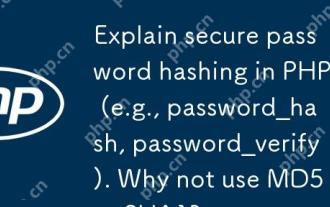
In PHP, password_hash and password_verify functions should be used to implement secure password hashing, and MD5 or SHA1 should not be used. 1) password_hash generates a hash containing salt values to enhance security. 2) Password_verify verify password and ensure security by comparing hash values. 3) MD5 and SHA1 are vulnerable and lack salt values, and are not suitable for modern password security.
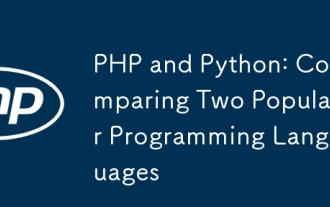
PHP and Python each have their own advantages, and choose according to project requirements. 1.PHP is suitable for web development, especially for rapid development and maintenance of websites. 2. Python is suitable for data science, machine learning and artificial intelligence, with concise syntax and suitable for beginners.
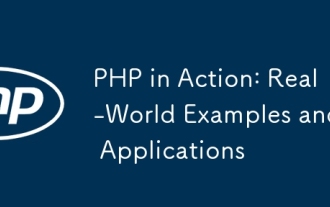
PHP is widely used in e-commerce, content management systems and API development. 1) E-commerce: used for shopping cart function and payment processing. 2) Content management system: used for dynamic content generation and user management. 3) API development: used for RESTful API development and API security. Through performance optimization and best practices, the efficiency and maintainability of PHP applications are improved.
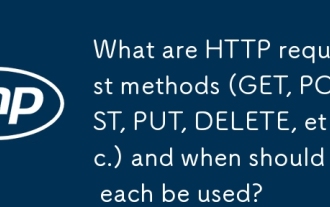
HTTP request methods include GET, POST, PUT and DELETE, which are used to obtain, submit, update and delete resources respectively. 1. The GET method is used to obtain resources and is suitable for read operations. 2. The POST method is used to submit data and is often used to create new resources. 3. The PUT method is used to update resources and is suitable for complete updates. 4. The DELETE method is used to delete resources and is suitable for deletion operations.
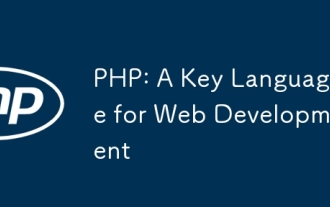
PHP is a scripting language widely used on the server side, especially suitable for web development. 1.PHP can embed HTML, process HTTP requests and responses, and supports a variety of databases. 2.PHP is used to generate dynamic web content, process form data, access databases, etc., with strong community support and open source resources. 3. PHP is an interpreted language, and the execution process includes lexical analysis, grammatical analysis, compilation and execution. 4.PHP can be combined with MySQL for advanced applications such as user registration systems. 5. When debugging PHP, you can use functions such as error_reporting() and var_dump(). 6. Optimize PHP code to use caching mechanisms, optimize database queries and use built-in functions. 7
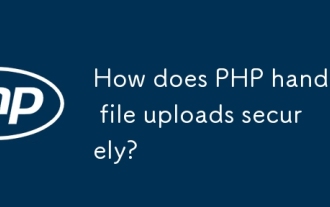
PHP handles file uploads through the $\_FILES variable. The methods to ensure security include: 1. Check upload errors, 2. Verify file type and size, 3. Prevent file overwriting, 4. Move files to a permanent storage location.
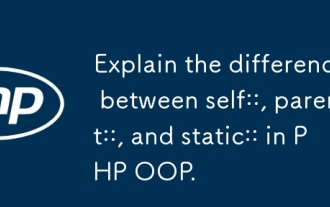
In PHPOOP, self:: refers to the current class, parent:: refers to the parent class, static:: is used for late static binding. 1.self:: is used for static method and constant calls, but does not support late static binding. 2.parent:: is used for subclasses to call parent class methods, and private methods cannot be accessed. 3.static:: supports late static binding, suitable for inheritance and polymorphism, but may affect the readability of the code.
