A brief discussion on Python object memory usage
Everything is an object
Everything in Python is an object, including all types of constants and variables, integers, booleans, and even functions. See a question on stackoverflow Is everything an object in python like ruby
You can verify it in the code:
# everythin in python is object def fuction(): return print isinstance(True, object) print isinstance(0, object) print isinstance('a', object) print isinstance(fuction, object)
How to calculate
Python provides the function getsizeof in the sys module to calculate the size of Python objects.
sys.getsizeof(object[, default]) 以字节(byte)为单位返回对象大小。 这个对象可以是任何类型的对象。 所以内置对象都能返回正确的结果 但不保证对第三方扩展有效,因为和具体实现相关。 ...... getsizeof() 调用对象的 __sizeof__ 方法, 如果对象由垃圾收集器管理, 则会加上额外的垃圾收集器开销。
Of course, the object memory usage is closely related to the Python version and operating system version. The code and test results in this article are based on the windows7 32-bit operating system.
import sys print sys.version
<font color="#000000" face="NSimsun">2.7.2 (default, Jun 24 2011, 12:21:10) [MSC v.1500 32 bit (Intel)]</font>
Basic types
•Boolean
print 'size of True: %d' % (sys.getsizeof(True)) print 'size of False: %d' % (sys.getsizeof(False))
Output:
size of True: 12 size of False: 12
•Plastic surgery
# normal integer print 'size of integer: %d' % (sys.getsizeof(1)) # long print 'size of long integer: %d' % (sys.getsizeof(1L)) print 'size of big long integer : %d' % (sys.getsizeof(100000L)) Output:
size of integer: 12x size of long integer 1L: 14 size of long integer 100000L: 16
It can be seen that the integer type occupies 12 bytes, and the long integer type occupies at least 14 bytes, and the occupied space will become larger as the number of digits increases. In version 2.x, if the value of the integer type exceeds sys.maxint, it is automatically expanded to a long integer. After Python 3.0, integers and long integers are unified into one type.
•Floating point
print 'size of float: %d' % (sys.getsizeof(1.0))
Output:
size of float: 16
Floating point type occupies 16 bytes. Exceeding a certain precision will be rounded off.
Refer to the following code:
print 1.00000000003 print 1.000000000005
Output:
1.00000000003 1.00000000001
•String
# size of string type print 'rn'.join(["size of string with %d chars: %d" % (len(elem), sys.getsizeof(elem)) for elem in ["", "a" , "ab"]]) # size of unicode string print 'rn'.join(["size of unicode string with %d chars: %d" % (len(elem), sys.getsizeof(elem)) for elem in [u"", u"a", u"ab"]])
Output:
size of string with 0 chars: 21 size of string with 1 chars: 22 size of string with 2 chars: 23 size of unicode string with 0 chars: 26 size of unicode string with 1 chars: 28 size of unicode string with 2 chars : 30
An ordinary empty string occupies 21 bytes, and each additional character occupies 1 more byte. Unicode strings occupy a minimum of 26 bytes, and each additional character occupies an additional 2 bytes.
Collection type
•List
# size of list type print 'rn'.join(["size of list with %d elements: %d" % (len(elem), sys.getsizeof(elem)) for elem in [[], [0] , [0,2], [0,1,2]]])
Output:
size of list with 0 elements: 36 size of list with 1 elements: 40 size of list with 2 elements: 44 size of list with 3 elements: 48
The visible list occupies at least 36 bytes, and each additional element increases by 4 bytes. But be aware that the sys.getsizeof function does not calculate the element size of the container type. For example:
print 'size of list with 3 integers %d' % (sys.getsizeof([0,1,2])) print 'size of list with 3 strings %d' % (sys.getsizeof(['0',' 1','2']))
Output:
size of list with 3 integers 48 size of list with 3 strings 48
What is stored in the container should be a reference to the element. If you want to accurately calculate the container, you can refer to the recursive sizeof recipe. Use the total_size function it gives:
print 'total size of list with 3 integers %d' % (total_size([0,1,2])) print 'total size of list with 3 strings %d' % (total_size(['0','1' ,'2']))
The output is:
total size of list with 3 integers 84 total size of list with 3 strings 114
It can be seen that the space occupied by the list is basic space 36 + (object reference 4 + object size) * number of elements.
Also note that if you declare a list variable, it will pre-allocate some space to increase efficiency when adding elements:
li = [] for i in range(0, 101): print 'list with %d integers size: %d, total_size: %d' % (i, getsizeof(li), total_size(li)) li.append( i)
•Tuple
Basically similar to a list, but it occupies at least 28 bytes.
•Dictionary
The situation of dictionaries is relatively complicated. Of course, you should refer to the code dictobject.c for details. In addition, NOTES ON OPTIMIZING DICTIONARIES is worth reading carefully.
For the basic situation, you can refer to some answers in [stackoverflow] question Python's underlying hash data structure for dictionaries:
•The dictionary has a minimum space of 8 entries (PyDict_MINSIZE);
•When the number of entries is less than 50,000, it will increase 4 times each time;
•When the number of entries is greater than 50,000, it will increase by 2 times each time;
•The hash value of the key is cached in the dictionary and will not be recalculated after the dictionary is resized;
The dictionary will resize every time it approaches 2/3.
The above article briefly discussing the memory usage of Python objects is all the content shared by the editor. I hope it can give you a reference, and I hope you will support Script Home.

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










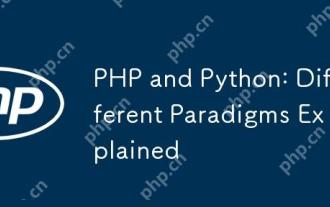
PHP is mainly procedural programming, but also supports object-oriented programming (OOP); Python supports a variety of paradigms, including OOP, functional and procedural programming. PHP is suitable for web development, and Python is suitable for a variety of applications such as data analysis and machine learning.
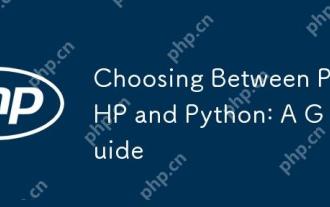
PHP is suitable for web development and rapid prototyping, and Python is suitable for data science and machine learning. 1.PHP is used for dynamic web development, with simple syntax and suitable for rapid development. 2. Python has concise syntax, is suitable for multiple fields, and has a strong library ecosystem.
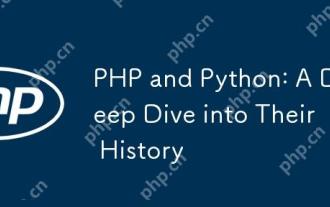
PHP originated in 1994 and was developed by RasmusLerdorf. It was originally used to track website visitors and gradually evolved into a server-side scripting language and was widely used in web development. Python was developed by Guidovan Rossum in the late 1980s and was first released in 1991. It emphasizes code readability and simplicity, and is suitable for scientific computing, data analysis and other fields.
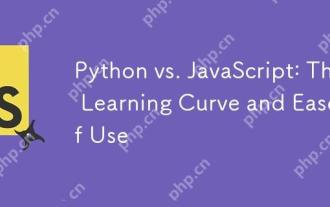
Python is more suitable for beginners, with a smooth learning curve and concise syntax; JavaScript is suitable for front-end development, with a steep learning curve and flexible syntax. 1. Python syntax is intuitive and suitable for data science and back-end development. 2. JavaScript is flexible and widely used in front-end and server-side programming.
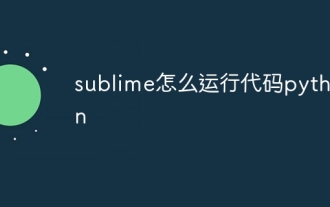
To run Python code in Sublime Text, you need to install the Python plug-in first, then create a .py file and write the code, and finally press Ctrl B to run the code, and the output will be displayed in the console.
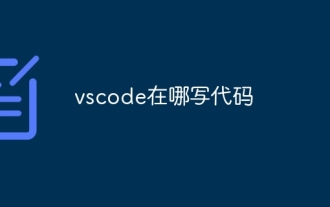
Writing code in Visual Studio Code (VSCode) is simple and easy to use. Just install VSCode, create a project, select a language, create a file, write code, save and run it. The advantages of VSCode include cross-platform, free and open source, powerful features, rich extensions, and lightweight and fast.
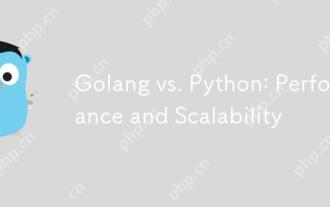
Golang is better than Python in terms of performance and scalability. 1) Golang's compilation-type characteristics and efficient concurrency model make it perform well in high concurrency scenarios. 2) Python, as an interpreted language, executes slowly, but can optimize performance through tools such as Cython.
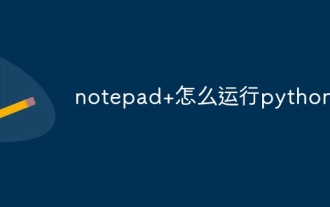
Running Python code in Notepad requires the Python executable and NppExec plug-in to be installed. After installing Python and adding PATH to it, configure the command "python" and the parameter "{CURRENT_DIRECTORY}{FILE_NAME}" in the NppExec plug-in to run Python code in Notepad through the shortcut key "F6".
