Super calling details in multiple inheritance
Note: Python 3 is used as the operating environment here, and the example is taken from Chapter 8 of "python cookbook".
In python, if a subclass wants to initialize the parent class, there are two main methods. The first is to directly pass the parent class name, and the second is to use the super method. There is no difference between the two in single inheritance, but there are some subtle differences that need to be paid attention to in multiple inheritance. Example explanation is the last word!
1. The case of using the parent class name:
Python code
class Base:
def __init__(self):
print('Base.__init__')
class A (Base):
def __init__(self):
use using using using use using ‐ ‐ use using out out out out out of ’s to def __init__(self)’s to be __init__(self’ be-to-be--
Base.__init__(self) " B.__init__(self)
print( 'C.__init__')
At this time, instantiating class C will output the following:Python code
>>> c = C()
Base.__init__
A.__init__
Base .__init__
B.__init__
C.__init__
>>>
It can be seen that the Base class is called twice. This must not be the result we want in many cases, so we can consider using the super method at this time.
Python code
class Base:
def __init__(self):
print('Base.__init__')
class A (Base):
def __init__(self):
super().__init__( )
) to super() here
At this time, the output of Class C is instantiated at this time:
python code & & gt; & gt; & gt; c = c () base .____ B. __init__ A.__init__ C.__init__ >>>It can be seen that the Base class is only called once! But unfortunately, this is not what prompted me to write this blog record, because if you observe carefully, although the Base class is indeed called only once as expected, have you noticed that "B.__init__" is output first and then Just output "A.__init__"? And why does this cause Base to be initialized only once? You must be a little confused too, right? In fact, all this is "blamed" on the calling process of super during multiple inheritance. When python implements a class (not just inheritance), it will generate a method to generate a parsing order list, which can be viewed through the class attribute __mro__, as in this example:
Python code >> ;> C.__mro__ (
So when searching for a property or method, it will iterate through each class according to this list until it finds the first class that matches this property or method. When using super in inheritance, the interpreter will search for the next class on the list every time it encounters super until it no longer encounters super or the list is traversed, and then returns layer by layer similar to recursion. Therefore, the search process in this example is: encounter super in C --> search for the next class in the list, that is, A --> encounter super again in A, search for B --> super reappears in B, search Base --> Initialize the Base class and return recursively.
In order to better explain the process, please comment out the super line of class B now:
Python code
class B(Base):
def __init__(self):
#super().__init__( )
) to super() here
'Print (' C.__ init__ ')Class C again, output as follows:
pythonn code & & gt; & gt; c = c () b .__
C.__init__
Base class no longer produces output! Why? Because there is no super in B, the list is blocked from searching for the Base class, so Base is not initialized!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










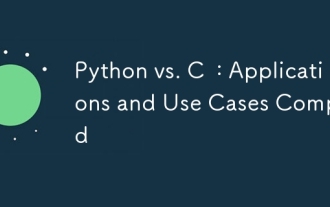
Python is suitable for data science, web development and automation tasks, while C is suitable for system programming, game development and embedded systems. Python is known for its simplicity and powerful ecosystem, while C is known for its high performance and underlying control capabilities.
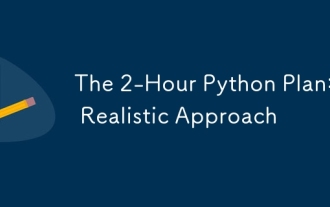
You can learn basic programming concepts and skills of Python within 2 hours. 1. Learn variables and data types, 2. Master control flow (conditional statements and loops), 3. Understand the definition and use of functions, 4. Quickly get started with Python programming through simple examples and code snippets.
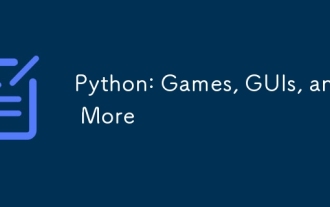
Python excels in gaming and GUI development. 1) Game development uses Pygame, providing drawing, audio and other functions, which are suitable for creating 2D games. 2) GUI development can choose Tkinter or PyQt. Tkinter is simple and easy to use, PyQt has rich functions and is suitable for professional development.
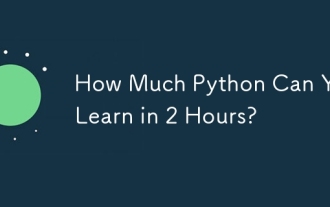
You can learn the basics of Python within two hours. 1. Learn variables and data types, 2. Master control structures such as if statements and loops, 3. Understand the definition and use of functions. These will help you start writing simple Python programs.
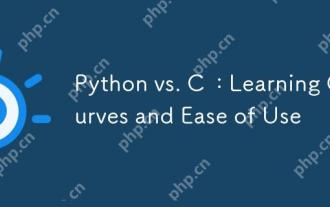
Python is easier to learn and use, while C is more powerful but complex. 1. Python syntax is concise and suitable for beginners. Dynamic typing and automatic memory management make it easy to use, but may cause runtime errors. 2.C provides low-level control and advanced features, suitable for high-performance applications, but has a high learning threshold and requires manual memory and type safety management.
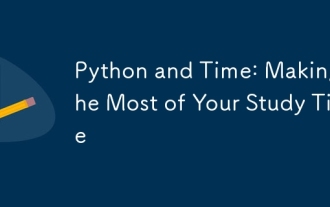
To maximize the efficiency of learning Python in a limited time, you can use Python's datetime, time, and schedule modules. 1. The datetime module is used to record and plan learning time. 2. The time module helps to set study and rest time. 3. The schedule module automatically arranges weekly learning tasks.
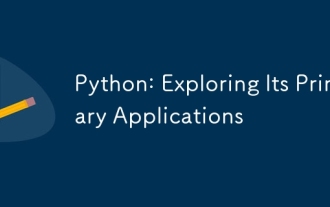
Python is widely used in the fields of web development, data science, machine learning, automation and scripting. 1) In web development, Django and Flask frameworks simplify the development process. 2) In the fields of data science and machine learning, NumPy, Pandas, Scikit-learn and TensorFlow libraries provide strong support. 3) In terms of automation and scripting, Python is suitable for tasks such as automated testing and system management.
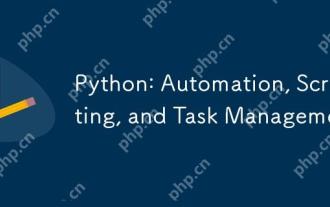
Python excels in automation, scripting, and task management. 1) Automation: File backup is realized through standard libraries such as os and shutil. 2) Script writing: Use the psutil library to monitor system resources. 3) Task management: Use the schedule library to schedule tasks. Python's ease of use and rich library support makes it the preferred tool in these areas.
