Summary of Exceptions in Python
Exceptions refer to exceptions and violations in the program. The exception mechanism refers to the program's handling method after an error occurs in the program. When an error occurs, the execution flow of the program changes and the control of the program is transferred to exception handling. The following article mainly summarizes relevant information about exceptions in Python. Friends in need can refer to it.
Preface
Exception class is a commonly used exception class, which includes StandardError, StopIteration, GeneratorExit, Warning and other exception classes. Exceptions in Python are created using inheritance structures. Base class exceptions can be captured in the exception handler, or various subclass exceptions can be captured. Exceptions are captured using the try...except statement in Python, and the exception clause is defined after the try clause. .
Exception handling in Python
The statement structure of exception handling
try: <statements> #运行try语句块,并试图捕获异常 except <name1>: <statements> #如果name1异常发现,那么执行该语句块。 except (name2, name3): <statements> #如果元组内的任意异常发生,那么捕获它 except <name4> as <variable>: <statements> #如果name4异常发生,那么进入该语句块,并把异常实例命名为variable except: <statements> #发生了以上所有列出的异常之外的异常 else: <statements> #如果没有异常发生,那么执行该语句块 finally: <statement> #无论是否有异常发生,均会执行该语句块。
Explanation
- ##else and finally are optional, there may be 0 or more except, but if an else occurs, there must be at least one except.
- #No matter how you specify the exception, the exception is always identified by the instance object and most of the time is activated at any given moment. Once an exception is caught by an except clause somewhere in the program, it is dead unless it is reraised by another raise statement or an error.
raise statement
- raise #The instance can be created before the raise statement or in the raise statement.
- #raise #Python will implicitly create an instance of the class
- raise name(value) #Provide additional information while throwing an exception value
- #raise # Rethrow the latest exception
- raise exception from E
ValueError with additional information: raise ValueError('we can only accept positive values')
__cause__ attribute that raised the exception. If the raised exception is not caught, Python will print the exception as part of the standard error message:
try: 1/0 except Exception as E: raise TypeError('bad input') from E
The execution result is as follows:
Traceback (most recent call last): File "hh.py", line 2, in <module> 1/0 ZeropisionError: pision by zero The above exception was the direct cause of the following exception: Traceback (most recent call last): File "hh.py", line 4, in <module> raise TypeError('bad input') from E TypeError: bad input
assert statement
with...as statement
- The environment manager must have
__enter__
and
__exit__method.
##
The method will be run during initialization. If there is an ass, the return value of the __enter__
function will be assigned to The variables in the as clause, otherwise, are discarded directly.
The code nested in the code block will be executed.
If the with code block raises an exception, the
method will be called (with exception details). These are also the same values returned by sys.exc_info. If this method returns false, the exception is re-raised. Otherwise, it terminates abnormally. Under normal circumstances, exceptions should be re-raised so that they can be passed outside the with statement.
If the with code block does not throw an exception, the
method will still be called, and its type, value and traceback parameters will be passed as None.
The following is a simple custom context management class.
class Block: def __enter__(self): print('entering to the block') return self def prt(self, args): print('this is the block we do %s' % args) def __exit__(self,exc_type, exc_value, exc_tb): if exc_type is None: print('exit normally without exception') else: print('found exception: %s, and detailed info is %s' % (exc_type, exc_value)) return False with Block() as b: b.prt('actual work!') raise ValueError('wrong')
If you log out to the raise statement above, it will exit normally.
Without canceling the raise statement, the running result is as follows:
entering to the block this is the block we do actual work! found exception: <class 'ValueError'>, and detailed info is wrong Traceback (most recent call last): File "hh.py", line 18, in <module> raise ValueError('wrong') ValueError: wrong
If an exception occurs, a tuple containing 3 elements can be returned by calling the
function. The first element is the class that raised the exception, and the second is the instance that was actually raised. The third element, the traceback object, represents the stack of calls when the exception originally occurred. If everything is normal, 3 None will be returned.
|Exception Name|Description| |BaseException|Root class for all exceptions| | SystemExit|Request termination of Python interpreter| |KeyboardInterrupt|User interrupted execution (usually by pressing Ctrl+C)| |Exception|Root class for regular exceptions| | StopIteration|Iteration has no further values| | GeneratorExit|Exception sent to generator to tell it to quit| | SystemExit|Request termination of Python interpreter| | StandardError|Base class for all standard built-in exceptions| | ArithmeticError|Base class for all numeric calculation errors| | FloatingPointError|Error in floating point calculation| | OverflowError|Calculation exceeded maximum limit for numerical type| | ZeropisionError|pision (or modulus) by zero error (all numeric types)| | AssertionError|Failure of assert statement| | AttributeError|No such object attribute| | EOFError|End-of-file marker reached without input from built-in| | EnvironmentError|Base class for operating system environment errors| | IOError|Failure of input/output operation| | OSError|Operating system error| | WindowsError|MS Windows system call failure| | ImportError|Failure to import module or object| | KeyboardInterrupt|User interrupted execution (usually by pressing Ctrl+C)| | LookupError|Base class for invalid data lookup errors| | IndexError|No such index in sequence| | KeyError|No such key in mapping| | MemoryError|Out-of-memory error (non-fatal to Python interpreter)| | NameError|Undeclared/uninitialized object(non-attribute)| | UnboundLocalError|Access of an uninitialized local variable| | ReferenceError|Weak reference tried to access a garbage collected object| | RuntimeError|Generic default error during execution| | NotImplementedError|Unimplemented method| | SyntaxError|Error in Python syntax| | IndentationError|Improper indentation| | TabErrorg|Improper mixture of TABs and spaces| | SystemError|Generic interpreter system error| | TypeError|Invalid operation for type| | ValueError|Invalid argument given| | UnicodeError|Unicode-related error| | UnicodeDecodeError|Unicode error during decoding| | UnicodeEncodeError|Unicode error during encoding| | UnicodeTranslate Error|Unicode error during translation| | Warning|Root class for all warnings| | DeprecationWarning|Warning about deprecated features| | FutureWarning|Warning about constructs that will change semantically in the future| | OverflowWarning|Old warning for auto-long upgrade| | PendingDeprecation Warning|Warning about features that will be deprecated in the future| | RuntimeWarning|Warning about dubious runtime behavior| | SyntaxWarning|Warning about dubious syntax| | UserWarning|Warning generated by user code|
More about exceptions in Python (Exception ), please pay attention to the PHP Chinese website for related articles!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
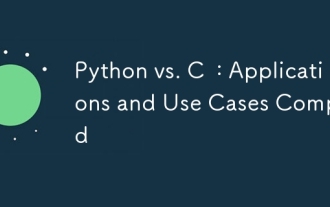
Python is suitable for data science, web development and automation tasks, while C is suitable for system programming, game development and embedded systems. Python is known for its simplicity and powerful ecosystem, while C is known for its high performance and underlying control capabilities.
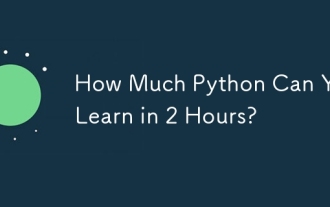
You can learn the basics of Python within two hours. 1. Learn variables and data types, 2. Master control structures such as if statements and loops, 3. Understand the definition and use of functions. These will help you start writing simple Python programs.
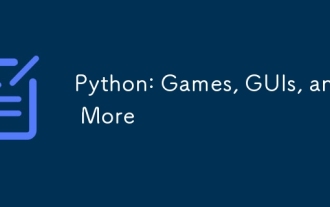
Python excels in gaming and GUI development. 1) Game development uses Pygame, providing drawing, audio and other functions, which are suitable for creating 2D games. 2) GUI development can choose Tkinter or PyQt. Tkinter is simple and easy to use, PyQt has rich functions and is suitable for professional development.
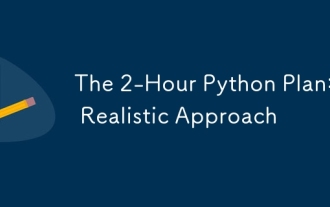
You can learn basic programming concepts and skills of Python within 2 hours. 1. Learn variables and data types, 2. Master control flow (conditional statements and loops), 3. Understand the definition and use of functions, 4. Quickly get started with Python programming through simple examples and code snippets.
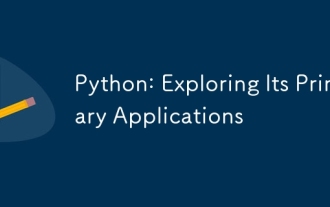
Python is widely used in the fields of web development, data science, machine learning, automation and scripting. 1) In web development, Django and Flask frameworks simplify the development process. 2) In the fields of data science and machine learning, NumPy, Pandas, Scikit-learn and TensorFlow libraries provide strong support. 3) In terms of automation and scripting, Python is suitable for tasks such as automated testing and system management.
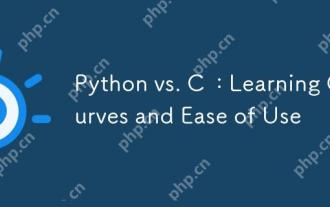
Python is easier to learn and use, while C is more powerful but complex. 1. Python syntax is concise and suitable for beginners. Dynamic typing and automatic memory management make it easy to use, but may cause runtime errors. 2.C provides low-level control and advanced features, suitable for high-performance applications, but has a high learning threshold and requires manual memory and type safety management.
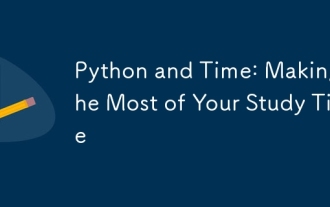
To maximize the efficiency of learning Python in a limited time, you can use Python's datetime, time, and schedule modules. 1. The datetime module is used to record and plan learning time. 2. The time module helps to set study and rest time. 3. The schedule module automatically arranges weekly learning tasks.
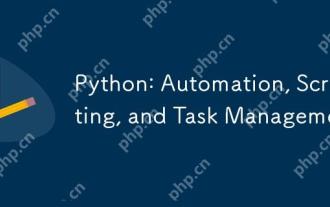
Python excels in automation, scripting, and task management. 1) Automation: File backup is realized through standard libraries such as os and shutil. 2) Script writing: Use the psutil library to monitor system resources. 3) Task management: Use the schedule library to schedule tasks. Python's ease of use and rich library support makes it the preferred tool in these areas.
