


Detailed graphic explanation of the LZ77 compression algorithm coding Python implementation principle
Preface
The LZ77 algorithm is a lossless compression algorithm published by Israeli Abraham Lempel in 1977. LZ77 is a typical dictionary-based compression algorithm, and many current compression technologies are based on LZ77. In view of its status in the field of data compression, this article will introduce its principles in detail with pictures and source code.
Principle introduction:
First introduce a few professional terms.
1.lookahead buffer (I don’t know how to express it in Chinese, temporarily called the area to be encoded):
Area waiting for encoding
2. search buffer:
Already encoded area, search buffer
3. Sliding window:
Specified size window, including "search buffer" (left) + "area to be encoded" (right )
Next, the specific encoding process is introduced:
In order to encode the area to be encoded, the encoder searches in the search buffer of the sliding window until it finds a matching string . The distance between the starting string of the matching string and the buffer to be encoded is called the "offset value", and the length of the matching string is called the "matching length". When encoding, the encoder will keep searching in the search area until it finds the maximum matching string and outputs (o, l), where o is the offset value and l is the matching length. Then the window slides l and continues coding. If no matching string is found, (0, 0, c) is output, where c is the next character waiting to be encoded in the area to be encoded, and the window slides "1". The algorithm implementation will be similar to the following:
while( lookAheadBuffer not empty ) { get a pointer (position, match) to the longest match in the window for the lookAheadBuffer; output a (position, length, char()); shift the window length+1 characters along; }
The main steps are:
1. Set the encoding position to the beginning of the input stream
2. Search in the area to be encoded in the sliding window The maximum matching string in the search area
3. If the string is found, output (offset value, matching length), and the window slides forward "matching length"
4. If not found , output (0, 0, the first character of the area to be encoded), and the window slides forward one unit
5. If the area to be encoded is not empty, return to step 2
Description It's really too complicated, let's explain it with examples
Example:
Now there is the string "AABCBBABC", now encode it.
At the beginning, the window slides into the position shown in the picture
As can be seen from the picture, there are three characters "AAB" in the buffer to be encoded. At this time, the buffer is searched The area is still empty. Therefore, when encoding the first character, since the search area is empty, no matching string can be found, (0,0, A) is output, and the window moves one unit to the right, as shown below
At this time, there is "ABC" in the area to be encoded. Start coding. First encode "A" and find "A" in the search area. Since the area to be encoded has not been exceeded, "AB" starts to be encoded, but no matching string is found in the search area, so it cannot be encoded. Therefore only "A" can be encoded.
Output(1, 1). That is, it is offset by one unit relative to the area to be encoded, and the matching length is 1. Slide the window to the right to match the length, that is, move 1 unit. As shown in the picture below
, if not found, output (0, 0, B), move 1 odd number to the right, as shown in the picture below
Output (0, 0, C), shift 1 unit to the right, as shown below
Output (2, 1), shift 1 unit to the right, As shown below
Output (3, 1), move 1 unit to the right, as shown below
开始编码”A”,在搜索缓冲区查找到匹配字符串。由于待编码缓冲区没有超过,继续编码。开始编码”AB”,也搜索到。不要停止,继续编码“ABC”,找到匹配字符串。由于继续编码,则超过了窗口,故只编码“ABC”,输出(5, 3),偏移5,长度3。右移3个单位,如下图
此时待编码缓冲区为空,停止编码。
最终输出结果如下
python代码实现:
class Lz77: def init(self, inputStr): self.inputStr = inputStr #输入流 self.searchSize = 5 #搜索缓冲区(已编码区)大小 self.aheadSize = 3 #lookAhead缓冲区(待编码区)大小 self.windSpiltIndex = 0 #lookHead缓冲区开始的索引 self.move = 0 self.notFind = -1 #没有找到匹配字符串 #得到滑动窗口的末端索引 def getWinEndIndex(self): return self.windSpiltIndex + self.aheadSize #得到滑动窗口的始端索引 def getWinStartIndex(self): return self.windSpiltIndex - self.searchSize #判断lookHead缓冲区是否为空 def isLookHeadEmpty(self): return True if self.windSpiltIndex + self.move> len(self.inputStr) - 1 else False def encoding(self): step = 0 print("Step Position Match Output") while not self.isLookHeadEmpty(): #1.滑动窗口 self.winMove() #2. 得到最大匹配串的偏移值和长度 (offset, matchLen) = self.findMaxMatch() #3.设置窗口下一步需要滑动的距离 self.setMoveSteps(matchLen) if matchLen == 0: #匹配为0,说明无字符串匹配,输出下一个需要编码的字母 nextChar = self.inputStr[self.windSpiltIndex] result = (step, self.windSpiltIndex, '-', '(0,0)' + nextChar) else: result = (step, self.windSpiltIndex, self.inputStr[self.windSpiltIndex - offset: self.windSpiltIndex - offset + matchLen], '(' + str(offset) + ',' + str(matchLen) + ')') #4.输出结果 self.output(result) step = step + 1 #仅用来设置第几步 #滑动窗口(移动分界点) def winMove(self): self.windSpiltIndex = self.windSpiltIndex + self.move #寻找最大匹配字符并返回相对于窗口分界点的偏移值和匹配长度 def findMaxMatch(self): matchLen = 0 offset = 0 minEdge = self.minEdge() + 1 #得到编码区域的右边界 #遍历待编码区,寻找最大匹配串 for i in range(self.windSpiltIndex + 1, minEdge): #print("i: %d" %i) offsetTemp = self.searchBufferOffest(i) if offsetTemp == self.notFind: return (offset, matchLen) offset = offsetTemp #偏移值 matchLen = matchLen + 1 #每找到一个匹配串,加1 return (offset, matchLen) #入参字符串是否存在于搜索缓冲区,如果存在,返回匹配字符串的起始索引 def searchBufferOffest(self, i): searchStart = self.getWinStartIndex() searchEnd = self.windSpiltIndex #下面几个if是处理开始时的特殊情况 if searchEnd < 1: return self.notFind if searchStart < 0: searchStart = 0 if searchEnd == 0: searchEnd = 1 searchStr = self.inputStr[searchStart : searchEnd] #搜索区字符串 findIndex = searchStr.find(self.inputStr[self.windSpiltIndex : i]) if findIndex == -1: return -1 return len(searchStr) - findIndex #设置下一次窗口需要滑动的步数 def setMoveSteps(self, matchLen): if matchLen == 0: self.move = 1 else: self.move = matchLen def minEdge(self): return len(self.inputStr) if len(self.inputStr) - 1 < self.getWinEndIndex() else self.getWinEndIndex() + 1 def output(self, touple): print("%d %d %s %s" % touple) if name == "main": lz77 = Lz77("AABCBBABC") lz77.encoding()
只是简单的写了下,没有过多考虑细节,请注意,这不是最终的代码,只是用来阐述原理,仅供参考。输出结果就是上面的输出(格式由于坑爹的博客园固定样式,代码位置有偏移,请注意
The above is the detailed content of Detailed graphic explanation of the LZ77 compression algorithm coding Python implementation principle. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










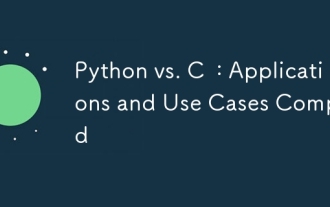
Python is suitable for data science, web development and automation tasks, while C is suitable for system programming, game development and embedded systems. Python is known for its simplicity and powerful ecosystem, while C is known for its high performance and underlying control capabilities.
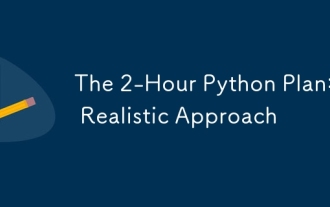
You can learn basic programming concepts and skills of Python within 2 hours. 1. Learn variables and data types, 2. Master control flow (conditional statements and loops), 3. Understand the definition and use of functions, 4. Quickly get started with Python programming through simple examples and code snippets.
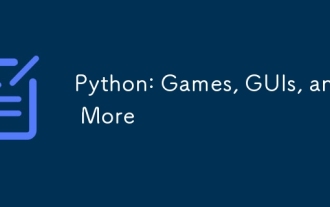
Python excels in gaming and GUI development. 1) Game development uses Pygame, providing drawing, audio and other functions, which are suitable for creating 2D games. 2) GUI development can choose Tkinter or PyQt. Tkinter is simple and easy to use, PyQt has rich functions and is suitable for professional development.
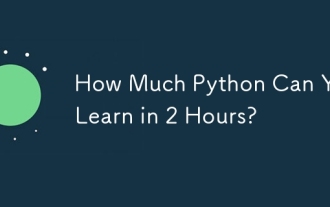
You can learn the basics of Python within two hours. 1. Learn variables and data types, 2. Master control structures such as if statements and loops, 3. Understand the definition and use of functions. These will help you start writing simple Python programs.
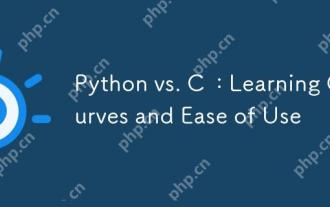
Python is easier to learn and use, while C is more powerful but complex. 1. Python syntax is concise and suitable for beginners. Dynamic typing and automatic memory management make it easy to use, but may cause runtime errors. 2.C provides low-level control and advanced features, suitable for high-performance applications, but has a high learning threshold and requires manual memory and type safety management.
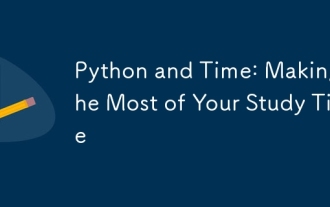
To maximize the efficiency of learning Python in a limited time, you can use Python's datetime, time, and schedule modules. 1. The datetime module is used to record and plan learning time. 2. The time module helps to set study and rest time. 3. The schedule module automatically arranges weekly learning tasks.
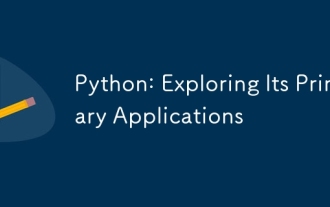
Python is widely used in the fields of web development, data science, machine learning, automation and scripting. 1) In web development, Django and Flask frameworks simplify the development process. 2) In the fields of data science and machine learning, NumPy, Pandas, Scikit-learn and TensorFlow libraries provide strong support. 3) In terms of automation and scripting, Python is suitable for tasks such as automated testing and system management.
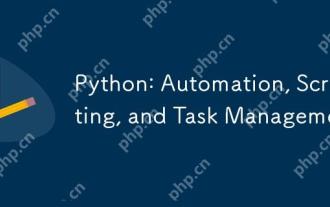
Python excels in automation, scripting, and task management. 1) Automation: File backup is realized through standard libraries such as os and shutil. 2) Script writing: Use the psutil library to monitor system resources. 3) Task management: Use the schedule library to schedule tasks. Python's ease of use and rich library support makes it the preferred tool in these areas.
