


Detailed explanation of the difference between Python3.x and Python2.x
Python3.x is a revolutionary upgrade of Python, which abolishes many previous features and introduces new features. Not fully compatible with old code from 1.x and 2.x.
Python2.x is backward compatible, and the latest version is Python 2.7. Among them, versions 2.6 and 2.7 were released to allow Python to smoothly transition to 3.X, and also added some 3.X features.
Currently, many libraries do not support 3.x (for example: Django, wxPython), and most applications are still based on 2.x. Beginners are recommended to use version 2.7.
Here are some main differences between Python 3.X and 2.X
1.Performance
Py3.0 runs pystone benchmark 30% slower than Py2.5. Guido believes that Py3.0 has great room for optimization, and can
to achieve good optimization results.
The performance of Py3.1 is 15% slower than that of Py2.5, and there is still a lot of room for improvement.
2. Encoding
Py3.X source code files use utf-8 encoding by default, which makes the following code legal:
>>> China = 'china'
>>>print(China)
china
3. Grammar
1) Removed <> and replaced all with !=
2) Remove `` and use repr()
instead.
3) Add as and with keywords, as well as True, False, None
4) Integer division returns a floating point number. To get an integer result, please use //
5) Add nonlocal statement. Peripheral (non-global) variables can be assigned directly using noclocal x
6) Remove the print statement and add the print() function to achieve the same function. The same is true for the exec statement, which has been changed to the exec() function
For example:
2.X: print "The answer is", 2*2
3.X: print("The answer is", 2*2)
2.X: print x,
3.X: print(x, end=" ") # Use spaces to replace lines
2.X: print 2.X: print # Output new line
3.X: print()
2.X: print >>sys.stderr, "fatal error"
3.X: print("fatal error", file=sys.stderr)
2.X: print (x, y)
3.X: print((x, y)) # Different from print(x, y)!
7) Changed the behavior of sequential operators, such as x
2.X:guess = int(raw_input('Enter an integer : ')) # Method to read keyboard input
3.X:guess = int(input('Enter an integer : '))
9) Remove tuple parameter unpacking. You cannot define the function like def(a, (b, c)):pass
10) For the new octal word variable, the oct() function has been modified accordingly.
2.The method of X is as follows:
>>> 0666
438
>>> oct(438)
'0666'
3.X like this:
>>> 0666
SyntaxError: invalid token (
>>> 0o666
438
>>> oct(438)
'0o666'
11) Added binary literals and bin() function
>>> bin(438)
'0b110110110'
>>> _438 = '0b110110110'
>>> _438
'0b110110110'
12) Extended iterable unpacking. In Py3.X, a, b, *rest = seq and *rest, a = seq are legal, and only require two points: rest is a list
Objects and seq are iterable.
13) The new super() can no longer pass parameters to super(),
>>> class C(object):
def __init__(self, a):
print('C', a)
>>> class D(C):
def __init(self, a):
super().__init__(a) # Call super() without parameters
>>> D(8)
C 8
<__main__.D object at 0x00D7ED90>
14) New metaclass syntax:
Class Foo(*bases, **kwds):
Pass
15) Support class decorator. Usage is the same as function decorator:
>>> def foo(cls_a):
def print_func(self):
print('Hello, world!')
cls_a.print = print_func
return cls_a
>>> @foo
Class C(object):
Pass
>>> C().print()
Hello, world!
Class decorator can be used to play the trick of changing the civet cat into a prince. For more information please refer to PEP 3129
4. Strings and byte strings
1) Now there is only one type of string, str, but it is almost the same as the 2.x version of unicode.
2) Regarding byte strings, please refer to item 2 of "Data Type"
5.Data type
1) Py3.X has removed the long type, and now there is only one integer type - int, but it behaves like the 2.
2) A new bytes type is added, corresponding to the octet string of version 2.X. The method of defining a bytes literal is as follows:
>>> b = b'china'
>>> type(b)
str objects and bytes objects can be converted to each other using the .encode() (str -> bytes) or .decode() (bytes -> str) method.
>>> s = b.decode()
>>> s
'china'
>>> b1 = s.encode()
>>> b1
b'china'
3) The .keys(), .items and .values() methods of dict return iterators, while the previous iterkeys() and other functions have been abandoned. Also removed are
dict.has_key(), replace it with in
6. Object-oriented
1) Introduce abstract base classes (Abstract Base Classes, ABCs).
2) Container classes and iterator classes are ABCsized, so there are many more types in the cellections module than in Py2.5.
>>> import collections
>>> print('\n'.join(dir(collections)))
Callable
Container
Hashable
ItemsView
Iterable
Iterator
KeysView
Mapping
MappingView
MutableMapping
MutableSequence
MutableSet
NamedTuple
Sequence
Set
Sized
ValuesView
__all__
__builtins__
__doc__
__file__
__name__
_abcoll
_itemgetter
_sys
defaultdict
deque
In addition, numeric types are also ABCsized. On these two points, see PEP 3119 and PEP 3141.
3) The next() method of iterator is renamed to __next__(), and the built-in function next() is added to call the __next__() method of iterator
4) Two decorators, @abstractmethod and @abstractproperty, have been added to make it more convenient to write abstract methods (properties).
7.Exception
1) All exceptions inherit from BaseException and StardardError
is removed
2) Removed the sequence behavior and .message attribute of the exception class
3) Use raise Exception(args) instead of raise Exception, args syntax
4) The syntax for catching exceptions has changed, and the as keyword has been introduced to identify exception instances. In Py2.5:
>>> try:
... raise NotImplementedError('Error')
...except NotImplementedError, error:
... ... print error.message
...
Error
In Py3.0:
>>> try:
Raise NotImplementedError('Error')
except NotImplementedError as error: #Note this as
print(str(error))
Error
5) Exception chain, because __context__ is not implemented in version 3.0a1
8. Module changes
1) The cPickle module has been removed and can be replaced by the pickle module. Eventually we will have a transparent and efficient module.
2) Removed imageop module
3) Removed audiodev, Bastion, bsddb185, exceptions, linuxaudiodev, md5, MimeWriter, mimify, popen2,
rexec, sets, sha, stringold, strop, sunaudiodev, timing and xmllib modules
4) Removed the bsddb module (released separately, available from http://www.jcea.es/programacion/pybsddb.htm)
5) Removed new module
6) The os.tmpnam() and os.tmpfile() functions have been moved to the tmpfile module
7) The tokenize module now works with bytes. The main entry point is no longer generate_tokens, but tokenize.tokenize()
9. Others
1) xrange() is renamed to range(). If you want to use range() to obtain a list, you must explicitly call:
>>> list(range(10))
[0, 1, 2, 3, 4, 5, 6, 7, 8, 9]
2) The bytes object cannot hash, nor does it support the b.lower(), b.strip() and b.split() methods, but for the latter two, you can use b.strip(b’
\n\t\r \f’) and b.split(b’ ‘) to achieve the same purpose
3) zip(), map() and filter() all return iterators. And apply(), callable(), coerce(), execfile(), reduce() and reload
() functions have been removed
Now you can use hasattr() to replace callable(). The syntax of hasattr() is: hasattr(string, '__name__')
4) string.letters and related .lowercase and .uppercase have been removed, please use string.ascii_letters instead
5) If x < y cannot be compared, a TypeError exception is thrown. Version 2.x is to return a pseudo-random Boolean value
6) The __getslice__ series members are abandoned. a[i:j] is converted to a.__getitem__(slice(I, j)) or __setitem__ and
depending on the context
__delitem__ calls
7) The file class is abandoned, in Py2.5:
>>> file
In Py3.X:
>>> file
Traceback (most recent call last):
File "
file
NameError: name 'file' is not defined
The above is the detailed content of Detailed explanation of the difference between Python3.x and Python2.x. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










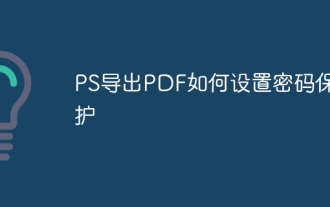
Export password-protected PDF in Photoshop: Open the image file. Click "File"> "Export"> "Export as PDF". Set the "Security" option and enter the same password twice. Click "Export" to generate a PDF file.
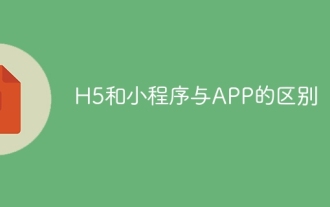
H5. The main difference between mini programs and APP is: technical architecture: H5 is based on web technology, and mini programs and APP are independent applications. Experience and functions: H5 is light and easy to use, with limited functions; mini programs are lightweight and have good interactiveness; APPs are powerful and have smooth experience. Compatibility: H5 is cross-platform compatible, applets and APPs are restricted by the platform. Development cost: H5 has low development cost, medium mini programs, and highest APP. Applicable scenarios: H5 is suitable for information display, applets are suitable for lightweight applications, and APPs are suitable for complex functions.
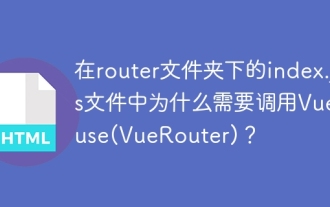
The necessity of registering VueRouter in the index.js file under the router folder When developing Vue applications, you often encounter problems with routing configuration. Special...
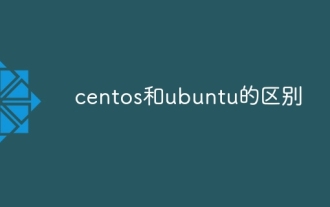
The key differences between CentOS and Ubuntu are: origin (CentOS originates from Red Hat, for enterprises; Ubuntu originates from Debian, for individuals), package management (CentOS uses yum, focusing on stability; Ubuntu uses apt, for high update frequency), support cycle (CentOS provides 10 years of support, Ubuntu provides 5 years of LTS support), community support (CentOS focuses on stability, Ubuntu provides a wide range of tutorials and documents), uses (CentOS is biased towards servers, Ubuntu is suitable for servers and desktops), other differences include installation simplicity (CentOS is thin)
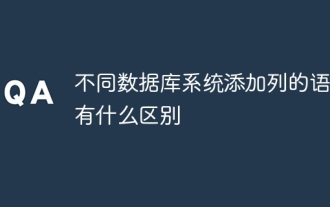
不同数据库系统添加列的语法为:MySQL:ALTER TABLE table_name ADD column_name data_type;PostgreSQL:ALTER TABLE table_name ADD COLUMN column_name data_type;Oracle:ALTER TABLE table_name ADD (column_name data_type);SQL Server:ALTER TABLE table_name ADD column_name data_
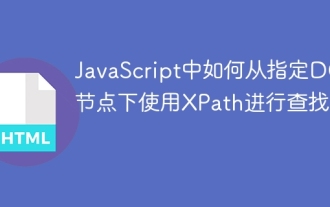
Detailed explanation of XPath search method under DOM nodes In JavaScript, we often need to find specific nodes from the DOM tree based on XPath expressions. If you need to...
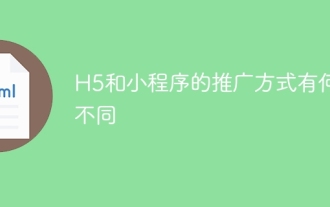
There are differences in the promotion methods of H5 and mini programs: platform dependence: H5 depends on the browser, and mini programs rely on specific platforms (such as WeChat). User experience: The H5 experience is poor, and the mini program provides a smooth experience similar to native applications. Communication method: H5 is spread through links, and mini programs are shared or searched through the platform. H5 promotion methods: social sharing, email marketing, QR code, SEO, paid advertising. Mini program promotion methods: platform promotion, social sharing, offline promotion, ASO, cooperation with other platforms.
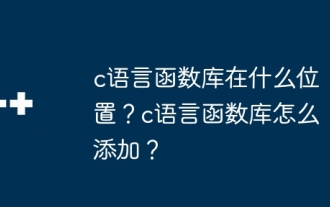
The C language function library is a toolbox containing various functions, which are organized in different library files. Adding a library requires specifying it through the compiler's command line options, for example, the GCC compiler uses the -l option followed by the abbreviation of the library name. If the library file is not under the default search path, you need to use the -L option to specify the library file path. Library can be divided into static libraries and dynamic libraries. Static libraries are directly linked to the program at compile time, while dynamic libraries are loaded at runtime.
