


Learn the three major characteristics of PHP object-oriented
PHP’s three major characteristics of object-oriented learning Learning goals: Fully understand abstraction, encapsulation, inheritance, and polymorphism
The three major characteristics of object-oriented: encapsulation, inheritance, and polymorphism First, simply understand Let’s talk about abstraction:
When we defined a class earlier, we actually extracted the common attributes and behaviors of a class of things to form a physical model (template). This method of studying problems is called abstraction
1. Encapsulation
Encapsulation is to encapsulate the extracted data and operations on the data together. The data is protected internally, and other parts of the program only have authorized operations. (Method) to operate on data.
php provides three access control modifiers
public means global, accessible inside this class, outside the class, and subclasses
protected means protected, only this class or subclasses can access
private means private and can only be accessed within this class
The above three modifiers can modify both methods and properties (variables). If the method does not have access modifiers, it defaults to public. Member properties must specify access modifiers. , there is also this way of writing var $name in PHP4, which means public attributes. This way of writing is not recommended.
Example:
Copy code The code is as follows:
<?php class Person{ public $name; protected $age; private $salary; function __construct($name,$age,$salary){ $this->name=$name; $this->age=$age; $this->salary=$salary; } public function showinfo(){ //这表示三个修饰符都可以在本类内部使用 echo $this->name."||".$this->age."||".$this->salary; } } $p1=new Person('张三',20,3000); //这里属于类外部,那么如果用下面的方法访问age和salary都会报错 // echo $p1->age; echo$p1->salary; ?>
So what should I do if I want to access protected and private elements and methods externally? The usual approach is to access these variable formats through public functions:
public function setxxxx($val){
$this->xxxx=$val;
}
public function getxxxx(){
return $this->xxxx;
}
The set and get here are just for the convenience of identification, not necessary.
For example:
public function getsalary(){
return $this- >salary; //Extension: You can call some methods here, such as judging the user name, etc., and only give access if it is correct
}
You can use echo from the outside $p1->getsalary();
If To access protected and private, you can also use the following methods, but they are not recommended, as long as you understand them
__set() and __get()
__set() assigns values to protected or private attributes
__set( $name,$val);
__get() gets the value of protected or private
__get($name);
For example:
Copy code The code is as follows:
<?php class testa{ protected $name; //使用__set()来管理所有属性 public function __set($pro_name,$pro_val){ //上面$pro_name和$pro_val可自定义 //下面$this->pro_name为既定,不可更改 $this->pro_name=$pro_val; } //使用__get()来获取所有属性值 public function __get($pro_name){ if(isset($pro_name)){ return $this->pro_name; } else { return null; } } } $n1=new testa(); //正常情况,类外部是不能访问protected属性的,但是用了上面的方法就可以对它们进行操作 $n1->name='小三'; echo $n1->name; ?>
//Just understand the above code, it is not recommended to use
2. Inheritance
Let’s look at an example first:
Copy code The code is as follows:
<?php class Pupil{ public $name; protected $age; public function getinfo(){ echo $this->name.'||'.$this->age; } public function testing(){ echo 'this is pupil'; } } class Graduate{ public $name; protected $age; public function getinfo(){ echo $this->name.'||'.$this->age; } public function testing(){ echo 'this is Graduate'; } } ?>
As can be seen from the above example, when multiple classes have many common properties and methods, the reusability of the code is not high. Code redundancy, think about the processing method in css
Solution: Inherit
Copy code The code is as follows:
<?php class Students{ public $name; public $age; public function __construct($name,$age){ $this->name=$name; $this->age=$age; } public function showinfo(){ echo $this->name.'||'.$this->age; } } class Pupil extends Students{ function testing(){ echo 'Pupil '.$this->name.' is testing'; } } class Graduate extends Students{ function testing(){ echo 'Graduate '.$this->name.' is testing'; } } $stu1=new Pupil('张三',20); $stu1->showinfo(); echo '<br/>'; $stu1->testing(); ?>
As can be seen from the above, Inheritance means that a subclass (Subclass) inherits the public and protected attributes and methods of the parent class (BaseClass) through extends the parent class. Private attributes and methods cannot be inherited.
Syntax structure:
class parent class name { }
class subclass name extends parent class name{}
Details:
1. A subclass can only inherit one parent class (here refers to direct inheritance); if you want to inherit the properties and methods of multiple classes , you can use multi-layer inheritance
Example:
Copy code The code is as follows:
<?php class A{ public $name='AAA'; } class B extends A{ public $age=30; } class C extends B{} $p=new C(); echo $p->name;//这里会输出AAA ?>
2. When creating a subclass object, by default The constructor of its parent class will not be automatically called
Example:
class A{
public function __construct(){
echo 'A';
}
}
class B extends A{
public function __construct(){
echo 'B';
}
}
$b=new B();//Here B will be output first Constructor, if there is no constructor in B,
in A will be output. 3. If you need to access the method of the parent class in the subclass (the modifier of the constructor and member method is protected or private), you can use Parent class::method name or parent::method name to complete [Here parent and the previously mentioned self are both lowercase, and an error is reported in uppercase]
class A{
public function test(){
echo 'a_test';
}
}
class B extends A{
public function __construct(){
//Both methods are OK
A::test();
parent::test();
}
}
$b=new B();
5. If the method of a subclass (derived class) is exactly the same as the method of the parent class (public, protected), we call it method coverage or method override (override), see the following polymorphism
3. Polymorphism
Example:
Copy code The code is as follows:
<?php class Animal{ public $name; public $price; function cry(){ echo 'i don\'t know'; } } class Dog extends Animal{ //覆盖、重写 function cry(){ echo 'Wang Wang!'; Animal::cry();//这里不会报错,能正确执行父类的cry(); } } $dog1=new Dog(); $dog1->cry(); ?>
小结:
1、当一个父类知道所有的子类都有一个方法,但是父类不能确定该方法如何写,可以让子类去覆盖它的方法,方法覆盖(重写),必须要求子类的方法名和参数个数完全一致
2、如果子类要去调用父类的某个方法(protected/public),可以使用 父类名::方法名 或者 parent::方法名
3、在实现方法重写的时候,访问修饰符可以不一样,但是子类方法的访问权限必须大于等于父类方法的访问权限(即不能缩小父类方法的访问权限)
如 父类public function cry(){} 子类 protected function cry(){} 则会报错
但是子类的访问权限可以放大,如:
父类private function cry(){} 子类 protected function cry(){} 可以正确执行
扩展:
方法重载(overload)
基本概念:函数名相同,但参数的个数或参数的类型不同,达到调用同一个函数,可以区分不同的函数
在PHP5中虽然也支持重载,但是和其它语言还是有很大区别的,php中不能定义多个同名函数
PHP5中提供了强大的“魔术”函数,使用这些魔术函数,我们可以做到函数重载,
这里我们要到到 __call,当一个对象调一个方法时,而该方法不存在,则程序会自动调用__call
【官方不推荐使用】
PHP中有以下几个魔术常量:__LINE__ __FILE__ __DIR__ __FUNCTION__ __CLASS__ 等
例:
复制代码 代码如下:
<?php class A{ function test1($p){ echo 'test1<br/>'; } function test2($p){ echo 'test2<br/>'; } function __call($method,$p){ //这里$p为数组,上面两个变量名可自定义 if($method == 'test'){ if(count($p)==1){ $this->test1($p); } else if(count($p)==2){ $this->test2($p); } } } } $a=new A(); $a->test(5); $a->test(3,5); ?>
相关推荐:
PHP面向对象五大原则之依赖倒置原则(DIP)详解_php技巧
The above is the detailed content of Learn the three major characteristics of PHP object-oriented. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










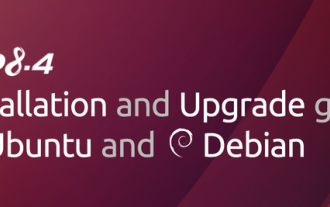
PHP 8.4 brings several new features, security improvements, and performance improvements with healthy amounts of feature deprecations and removals. This guide explains how to install PHP 8.4 or upgrade to PHP 8.4 on Ubuntu, Debian, or their derivati
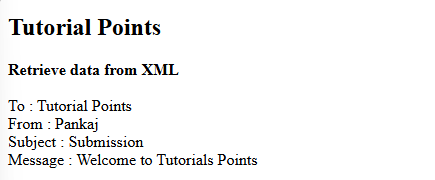
This tutorial demonstrates how to efficiently process XML documents using PHP. XML (eXtensible Markup Language) is a versatile text-based markup language designed for both human readability and machine parsing. It's commonly used for data storage an
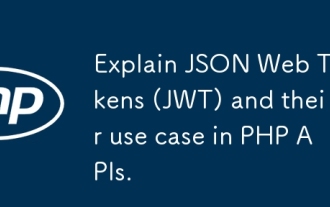
JWT is an open standard based on JSON, used to securely transmit information between parties, mainly for identity authentication and information exchange. 1. JWT consists of three parts: Header, Payload and Signature. 2. The working principle of JWT includes three steps: generating JWT, verifying JWT and parsing Payload. 3. When using JWT for authentication in PHP, JWT can be generated and verified, and user role and permission information can be included in advanced usage. 4. Common errors include signature verification failure, token expiration, and payload oversized. Debugging skills include using debugging tools and logging. 5. Performance optimization and best practices include using appropriate signature algorithms, setting validity periods reasonably,
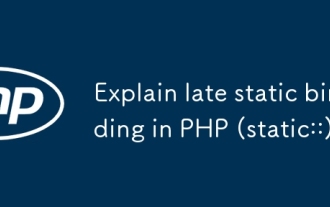
Static binding (static::) implements late static binding (LSB) in PHP, allowing calling classes to be referenced in static contexts rather than defining classes. 1) The parsing process is performed at runtime, 2) Look up the call class in the inheritance relationship, 3) It may bring performance overhead.
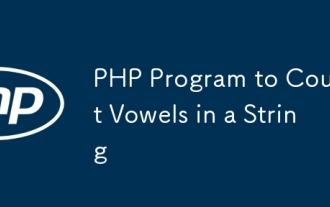
A string is a sequence of characters, including letters, numbers, and symbols. This tutorial will learn how to calculate the number of vowels in a given string in PHP using different methods. The vowels in English are a, e, i, o, u, and they can be uppercase or lowercase. What is a vowel? Vowels are alphabetic characters that represent a specific pronunciation. There are five vowels in English, including uppercase and lowercase: a, e, i, o, u Example 1 Input: String = "Tutorialspoint" Output: 6 explain The vowels in the string "Tutorialspoint" are u, o, i, a, o, i. There are 6 yuan in total

What are the magic methods of PHP? PHP's magic methods include: 1.\_\_construct, used to initialize objects; 2.\_\_destruct, used to clean up resources; 3.\_\_call, handle non-existent method calls; 4.\_\_get, implement dynamic attribute access; 5.\_\_set, implement dynamic attribute settings. These methods are automatically called in certain situations, improving code flexibility and efficiency.
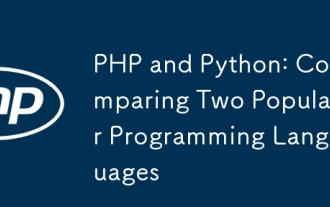
PHP and Python each have their own advantages, and choose according to project requirements. 1.PHP is suitable for web development, especially for rapid development and maintenance of websites. 2. Python is suitable for data science, machine learning and artificial intelligence, with concise syntax and suitable for beginners.
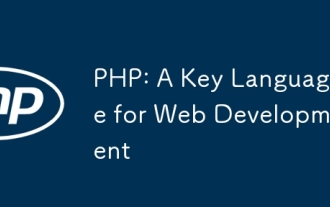
PHP is a scripting language widely used on the server side, especially suitable for web development. 1.PHP can embed HTML, process HTTP requests and responses, and supports a variety of databases. 2.PHP is used to generate dynamic web content, process form data, access databases, etc., with strong community support and open source resources. 3. PHP is an interpreted language, and the execution process includes lexical analysis, grammatical analysis, compilation and execution. 4.PHP can be combined with MySQL for advanced applications such as user registration systems. 5. When debugging PHP, you can use functions such as error_reporting() and var_dump(). 6. Optimize PHP code to use caching mechanisms, optimize database queries and use built-in functions. 7
