Detailed explanation of sorting algorithm
The so-called sorting is the operation of arranging a string of records in increasing or decreasing order according to the size of one or some keywords in it. Sorting algorithm is how to arrange records as required. Sorting algorithms have received considerable attention in many fields, especially in the processing of large amounts of data. An excellent algorithm can save a lot of resources.
Simple Insertion Sort insertion sort
/** * 将位置p上的元素向左移动,直到它在前p+1个元素中的正确位置被找到的地方 * @param a an array of Comparable items */public static <AnyType extends Comparable<? super AnyType>> void insertionSort(AnyType[] a) { int j; for (int p = 1; p < a.length; p++) { AnyType tmp = a[p]; for (j = p; j > 0 && tmp.compareTo(a[j-1]) < 0; j--) { a[j] = a[j-1]; } a[j] = tmp; } System.out.println(Arrays.toString(a));}
Shell Sort Hill sort
/** * @param a an array of Comparable items */public static <AnyType extends Comparable<? super AnyType>> void shellSort(AnyType[] a) { int j; for (int gap = a.length / 2; gap > 0; gap /= 2) { for (int i = gap; i < a.length; i++) { AnyType tmp = a[i]; for (j = i; j >= gap && tmp.compareTo(a[j - gap]) < 0; j -= gap) { a[j] = a[j - gap]; } a[j] = tmp; } } System.out.println(Arrays.toString(a)); }
Binary Sort binary sort
/** * @param a an array of Comparable items */public static <AnyType extends Comparable<? super AnyType>> void binarySort(AnyType[] a) { Integer i,j; Integer low,high,mid; AnyType temp; for(i=1;i<a.length;i++){ temp=a[i]; low=0; high=i-1; while(low<=high){ mid=(low+high)/2; if(temp.compareTo(a[mid]) < 0) { high=mid-1; } else { low=mid+1; } } for(j=i-1;j>high;j--) a[j+1]=a[j]; a[high+1]=temp; } System.out.println(Arrays.toString(a)); }
Bubble Sort Bubble Sort
/** * @param a an array of Comparable items */public static <AnyType extends Comparable<? super AnyType>> void bubbleSort(AnyType[] a) { Integer i,j; AnyType temp; for(i=1;i<a.length;i++) { for(j=0;j<a.length-i;j++) { //循环找到下沉"气泡",每下沉一位,下次比较长度较小一位 if(a[j].compareTo(a[j+1]) > 0) { temp=a[j]; a[j]=a[j+1]; a[j+1]=temp; //将"气泡"下沉到当前比较的最后一位 } } } System.out.println(Arrays.toString(a)); }
Selection Sort Selection Sort
/** * @param a an array of Comparable items */public static <AnyType extends Comparable<? super AnyType>> void selectSort(AnyType[] a) { Integer i,j,min; AnyType temp; for(i=0;i<a.length-1;i++) { temp=a[i]; min=i; //将当前位置元素当作最小值元素(其实是要将最小值元素交换到当前) for(j=i+1;j<a.length;j++) { if(temp.compareTo(a[j]) > 0) { //用a[i]和后面所有元素逐个比较,找到最小指的下标并记录 temp=a[j]; //下一位小于前一位,则将下一位赋值给temp并继续往右移动比较 min=j; //最小值的下标,赋值给min } } a[min] = a[i]; //将最小值元素的和当前元素交换,使得当前元素为其后面所有元素中最小值 a[i] = temp; } System.out.println(Arrays.toString(a));
The above content is just a few kinds of sorting Algorithm tutorial, I hope it can help everyone.
Related recommendations:
Example analysis of basic commonly used sorting algorithms in JavaScript
Detailed explanation of PHP heap sorting algorithm examples
Detailed explanation of several sorting algorithm examples in php

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics




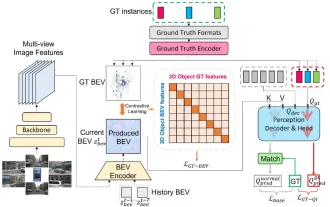
Written above & the author’s personal understanding: At present, in the entire autonomous driving system, the perception module plays a vital role. The autonomous vehicle driving on the road can only obtain accurate perception results through the perception module. The downstream regulation and control module in the autonomous driving system makes timely and correct judgments and behavioral decisions. Currently, cars with autonomous driving functions are usually equipped with a variety of data information sensors including surround-view camera sensors, lidar sensors, and millimeter-wave radar sensors to collect information in different modalities to achieve accurate perception tasks. The BEV perception algorithm based on pure vision is favored by the industry because of its low hardware cost and easy deployment, and its output results can be easily applied to various downstream tasks.
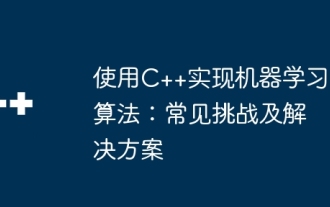
Common challenges faced by machine learning algorithms in C++ include memory management, multi-threading, performance optimization, and maintainability. Solutions include using smart pointers, modern threading libraries, SIMD instructions and third-party libraries, as well as following coding style guidelines and using automation tools. Practical cases show how to use the Eigen library to implement linear regression algorithms, effectively manage memory and use high-performance matrix operations.
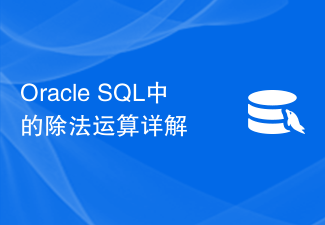
Detailed explanation of division operation in OracleSQL In OracleSQL, division operation is a common and important mathematical operation, used to calculate the result of dividing two numbers. Division is often used in database queries, so understanding the division operation and its usage in OracleSQL is one of the essential skills for database developers. This article will discuss the relevant knowledge of division operations in OracleSQL in detail and provide specific code examples for readers' reference. 1. Division operation in OracleSQL
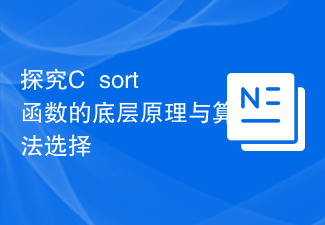
The bottom layer of the C++sort function uses merge sort, its complexity is O(nlogn), and provides different sorting algorithm choices, including quick sort, heap sort and stable sort.
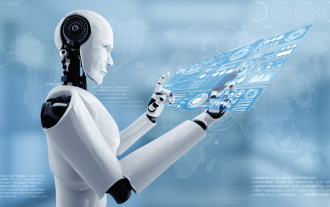
The convergence of artificial intelligence (AI) and law enforcement opens up new possibilities for crime prevention and detection. The predictive capabilities of artificial intelligence are widely used in systems such as CrimeGPT (Crime Prediction Technology) to predict criminal activities. This article explores the potential of artificial intelligence in crime prediction, its current applications, the challenges it faces, and the possible ethical implications of the technology. Artificial Intelligence and Crime Prediction: The Basics CrimeGPT uses machine learning algorithms to analyze large data sets, identifying patterns that can predict where and when crimes are likely to occur. These data sets include historical crime statistics, demographic information, economic indicators, weather patterns, and more. By identifying trends that human analysts might miss, artificial intelligence can empower law enforcement agencies

01 Outlook Summary Currently, it is difficult to achieve an appropriate balance between detection efficiency and detection results. We have developed an enhanced YOLOv5 algorithm for target detection in high-resolution optical remote sensing images, using multi-layer feature pyramids, multi-detection head strategies and hybrid attention modules to improve the effect of the target detection network in optical remote sensing images. According to the SIMD data set, the mAP of the new algorithm is 2.2% better than YOLOv5 and 8.48% better than YOLOX, achieving a better balance between detection results and speed. 02 Background & Motivation With the rapid development of remote sensing technology, high-resolution optical remote sensing images have been used to describe many objects on the earth’s surface, including aircraft, cars, buildings, etc. Object detection in the interpretation of remote sensing images
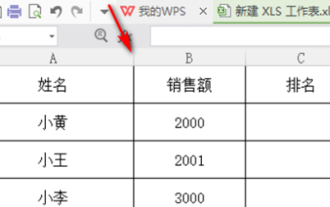
In our work, we often use wps software. There are many ways to process data in wps software, and the functions are also very powerful. We often use functions to find averages, summaries, etc. It can be said that as long as The methods that can be used for statistical data have been prepared for everyone in the WPS software library. Below we will introduce the steps of how to sort the scores in WPS. After reading this, you can learn from the experience. 1. First open the table that needs to be ranked. As shown below. 2. Then enter the formula =rank(B2, B2: B5, 0), and be sure to enter 0. As shown below. 3. After entering the formula, press the F4 key on the computer keyboard. This step is to change the relative reference into an absolute reference.
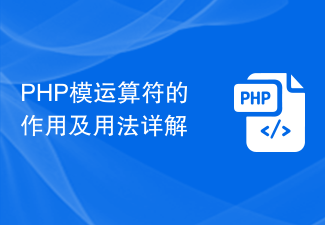
The modulo operator (%) in PHP is used to obtain the remainder of the division of two numbers. In this article, we will discuss the role and usage of the modulo operator in detail, and provide specific code examples to help readers better understand. 1. The role of the modulo operator In mathematics, when we divide an integer by another integer, we get a quotient and a remainder. For example, when we divide 10 by 3, the quotient is 3 and the remainder is 1. The modulo operator is used to obtain this remainder. 2. Usage of the modulo operator In PHP, use the % symbol to represent the modulus