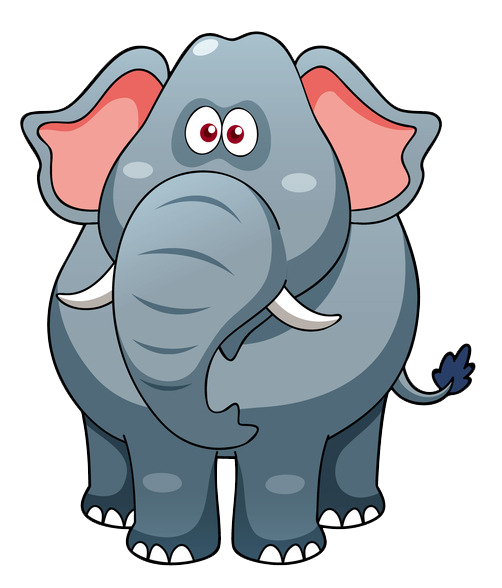
Correction status:qualified
Teacher's comments:
let arr = [1, 2, 3, 4, 5];
let index = 0;
while (index < arr.length) {//条件:index小于数组的长度
console.log(arr[index]);
index++;
}
for (let i = 0; i < arr.length; i++) {
console.log(arr[i]);
}
for (let value of arr) {
console.log(value);
}
试着用for-of循环遍历对象
const obj = {
id: 1,
item: "手机",
price: 5000,
};
for (let value of obj) {
console.log(value);
//obj is not iterable
}
//对象不能用for-of遍历
const obj = {
id: 1,
item: "手机",
price: 5000,
};
for (let value in obj) {
console.log(value);//for-in遍历对象,得到的是对象的属性,不是值
console.log(obj[key]);//得到对象的值
}
//forEach的回调函数有3个参数
//值,索引,数组
arr.forEach(function (item,index,arr) {
console.log(item,index,arr);
});
//通常我们只想得到数组的值可以如下
arr.forEach(function (item) {
console.log(item);
});
//回调函数可以箭头函数简写
arr.forEach((item) => console.log(item));
//数组解构赋值
let [item, price] = ["手机", 5000];
console.log(item, price); //手机 5000
//修改值
[item, price] = ["电脑", 15000];
console.log(item, price); //电脑 15000
//当变量多,值少时,应该给变量一个默认值
[item, price, color = "blue"] = ["电脑", 15000];
console.log(item, price, color);
//当变量少,值多时,增加变量 如 ...aaa
[item, price] = ["电脑", 15000, "blue", "17英寸"];
[item, price, ...other] = ["电脑", 15000, "blue", "17英寸"];
console.log(item, price, other);
console.log(item, price, ...other);
//解构对象
//当变量已经定义过,变量名会有冲突,可以使用别名,访问时用别名访问。
let { item: phone, price: jiaGe } = { item: "手机", price: 5000 };
console.log(phone, jiaGe);
//应用场景一:克隆对象
let obj = { id: 1, item: "手机", price: 5000 };
let { ...newObj } = obj;
console.log(newObj);
console.log(newObj.item);
//应用场景二:解构传参
let show = function (obj) {
return `${obj.item} : ${obj.price}`;
};
console.log(show(obj));
//参数简化
show = function ({ item, price }) {
return `${item} : ${price}`;
};
console.log(show(obj));