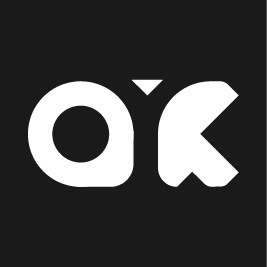
Correction status:qualified
Teacher's comments:完成的不错,继续努力
<?php
//post请求
//使用$_POST数组接收
print_r($_POST);
echo '<br>用户输入的邮箱:'.$_POST['email'];
echo '<br>用户输入的密码:'.$_POST['password'];
?>
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>POST传值</title>
</head>
<body>
<form action="" method="post">
<label for="email">email:</label>
<input type="text" name="email" id="email">
<label for="password">password:</label>
<input type="password" name="password" id="password">
<button>登陆</button>
</form>
</body>
</html>
<?php
//php系统函数
//一、字符串函数
//1.strtolower() 将字符串转换成小写
$a = 'ABcDE';
var_dump(strtolower($a)); //string(5) "abcde"
echo '<br>';
//2.strtoupper()将字符串转换成大写
$string = 'abcdE';
var_dump(strtoupper($string)); //string(5) "ABCDE"
echo '<br>';
//3.strlen() 获取字符串长度
$string1 = 'afuiabceankjfdsa';
var_dump(strlen($string1)); //int(16)
echo '<br>';
//4.trim() 去除字符串首尾空白字符(或其他字符)
$string = " 123123 ";
echo trim($string); //123123
echo '<br>';
var_dump(trim($string)); //string(6) "123123"
echo '<br>';
//5.ltrim() 去除字符串左边的空白字符(或其他字符)
$string = "1234321";
var_dump(ltrim($string,'1')); //string(6) "234321"
echo '<br>';
//6.rtrim() 去除字符串右边的空白字符(或其他字符)
$string = "1234321";
var_dump(rtrim($string,'1')); //string(6) "123432"
echo '<br>';
//7.str_replace() 字符串替换
$string = "123aaa123";
var_dump(str_replace('aaa', '123', $string)); //string(9) "123123123"
echo '<br>';
//8.strpbrk() 字符串中查找一组字符是否存在...
$string = '13701796255';
var_dump(strpbrk($string, '18')); //string(11) "13701796255"
echo '<br>';
//9.explode() 把字符串拆分成数组。
$stringArr = 'a b c d e';
var_dump(explode(' ', $stringArr)); //array(5) { [0]=> string(1) "a" [1]=> string(1) "b" [2]=> string(1) "c" [3]=> string(1) "d" [4]=> string(1) "e" }
echo '<br>';
//10.implode() 把数组组合为字符串。
$array = ['a','b','c','d','e'];
var_dump(implode('', $array)); //string(5) "abcde"
echo '<br>';
//11.md5() 对字符串进行md5加密
$password = '123456';
var_dump(md5($password)); //string(32) "e10adc3949ba59abbe56e057f20f883e"
echo '<br>';
//12.count() 计算数组中元素的数量
$arr = ['1','2','3','4','5'];
var_dump(count($arr)); //int(5)
echo '<br>';
//13.array_merge() 合并两个数组
$arr2 = ['a','b','c'];
var_dump(array_merge($arr,$arr2)); //array(8) { [0]=> string(1) "1" [1]=> string(1) "2" [2]=> string(1) "3" [3]=> string(1) "4" [4]=> string(1) "5" [5]=> string(1) "a" [6]=> string(1) "b" [7]=> string(1) "c" }
echo '<br>';
//14.in_array() 查询数组中是否存在某值
var_dump(in_array('a', $arr)); //bool(false)
echo '<br>';
var_dump(in_array('a', $arr2)); //bool(true)
echo '<br>';
//15.sort() 数组的升序排序 对索引数组 成功返回1 失败返回0
$arr_sort = ['16','25','32','12.5'];
sort($arr_sort);
print_r($arr_sort); //Array ( [0] => 12.5 [1] => 16 [2] => 25 [3] => 32 )
echo '<br>';
//16.rsort() 数组的降序排序
rsort($arr_sort);
print_r($arr_sort); //Array ( [0] => 32 [1] => 25 [2] => 16 [3] => 12.5 )
echo '<br>';
//17.array_unique() 移除数组中重复的值 去除之后下标保持不变
$array = ['6','6','6','5','4'];
$array_unique=array_unique($array);
print_r($array_unique); //Array ( [0] => 6 [3] => 5 [4] => 4 )
echo '<br>';
//18.array_push() 将元素添加到数组的末尾 返回添加后的数组长度
array_push($array,'3','2','1');
print_r($array); //Array ( [0] => 6 [1] => 6 [2] => 6 [3] => 5 [4] => 4 [5] => 3 [6] => 2 [7] => 1 )
echo '<br>';
//19.array_pop() 将数组末尾最后一个元素移除 返回被删除元素
array_pop($array);
print_r($array); //Array ( [0] => 6 [1] => 6 [2] => 6 [3] => 5 [4] => 4 [5] => 3 [6] => 2 )
echo '<br>';
//20.array_shift() 删除数组开头的元素
array_shift($array);
print_r($array);
echo '<br>';
//21.array_unshift() 将元素添加到数组开头
array_unshift($array, '0');
print_r($array); //Array ( [0] => 0 [1] => 6 [2] => 6 [3] => 5 [4] => 4 [5] => 3 [6] => 2 )
手写:
总结:php中函数有很大作用,需要能灵活运用处理解决问题。函数众多,记是记不完也记不住的,功能具体分为几大类,可以按照功能分类记住一些常用的以提高开发效率。