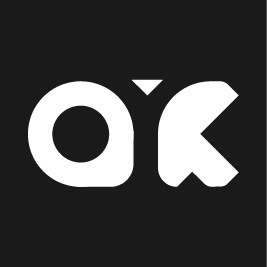
Correction status:qualified
Teacher's comments:完成的不错,继续加油
<?php
//数据库连接参数
$host = 'localhost';
$dbname = 'demo';
$username = 'root';
$pwd = 'root';
$dsn = "mysql:host=$host;dbname=$dbname";
try{
$pdo = new PDO($dsn,$username,$pwd);
}catch(PDOException $e){
die('连接失败:'.$e->getMessage());
}
<?php
header('content-type:text/html;charset=utf-8');
require 'connect.php';
$sql = 'INSERT INTO `student` (`name`,`phone`,`collegeId`) VALUES (:name,:phone,:collegeId)';
$stmt = $pdo->prepare($sql);
$name = '荆轲';
$phone = '10086';
$collegeId = 1;
$stmt->bindParam('name',$name,PDO::PARAM_STR);
$stmt->bindParam('phone',$phone,PDO::PARAM_STR);
$stmt->bindParam('collegeId',$collegeId,PDO::PARAM_INT);
if ($stmt->execute()){
if ($stmt->rowCount()>0){
echo '添加成功,返回主键ID是:'.$pdo->lastInsertId();
}else{
echo '添加失败';
}
}else{
die(print_r($stmt->errorInfo(),true));
}
$pdo = null;
<?php
header('content-type:text/html;charset=utf-8');
require 'connect.php';
$sql = 'UPDATE `student` SET `name`=:name,`phone`=:phone,`collegeId`=:collegeId WHERE `studentId`=:studentId';
$stmt = $pdo->prepare($sql);
$studentId = 5;
$name = '编辑后的名字';
$phone = '12345678';
$collegeId = 2;
$stmt->bindParam('studentId',$studentId,PDO::PARAM_INT);
$stmt->bindParam('name',$name,PDO::PARAM_STR);
$stmt->bindParam('phone',$phone,PDO::PARAM_STR);
$stmt->bindParam('collegeId',$collegeId,PDO::PARAM_INT);
if ($stmt->execute()){
if ($stmt->rowCount()>0){
echo '编辑成功';
}else{
echo '编辑失败';
}
}else{
die(print_r($stmt->errorInfo(),true));
}
$pdo = null;
<?php
header('content-type:text/html;charset=utf-8');
require 'connect.php';
$sql = 'DELETE FROM `student` WHERE `studentId`=:studentId';
$stmt = $pdo->prepare($sql);
$studentId = 6;
$stmt->bindParam('studentId',$studentId,PDO::PARAM_INT);
if ($stmt->execute()){
if ($stmt->rowCount()>0){
echo '删除成功';
}else{
echo '删除失败';
}
}else{
die(print_r($stmt->errorInfo(),true));
}
$pdo = null;
<?php
header('content-type:text/html;charset=utf-8');
require 'connect.php';
$sql = 'SELECT * FROM `student`';
$stmt = $pdo->prepare($sql);
$stmt->execute();
$cates = $stmt->fetchAll(PDO::FETCH_ASSOC);
foreach($cates as $cate){
echo '学生信息:'.$cate['studentId'].'-'.$cate['name'].'-'.$cate['phone'].'-'.$cate['collegeId'];
echo '<br>';
}
<?php
header('content-type:text/html;charset=utf-8');
require 'connect.php';
$sql = 'SELECT * FROM `student` WHERE `studentId`=:studentId';
$stmt = $pdo->prepare($sql);
$studentId = 2;
$stmt->bindParam('studentId',$studentId,PDO::PARAM_INT);
$stmt->execute();
while($cate = $stmt->fetch(PDO::FETCH_ASSOC)){
echo '学生信息:'.$cate['studentId'].'-'.$cate['name'].'-'.$cate['phone'].'-'.$cate['collegeId'];
}
$pdo = null;