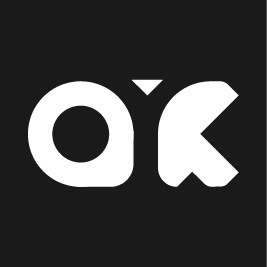
Correction status:qualified
Teacher's comments:完成的不错,继续加油。
用到两个文件
<?php
class con{
// 需要用到的属性
public $host;
public $user;
public $pass;
public $name;
public $type;
public $port;
public $charset;
public $dsn;
// 创建链接数据库的方法construct
public function construct($host,$user,$pass,$name,$type='mysql',$port=3306,$charset='utf8' ){
$this->host=$host;
$this->user=$user;
$this->pass=$pass;
$this->name=$name;
$this->type=$type;
$this->port=$port;
$this->charset=$charset;
$db = array(
'charset' => $this->charset,
'port' => $this->port,
'type' => $this->type,
'host' => $this->host,
'user' => $this->user,
'pass' => $this->pass,
'name' => $this->name
);
$dsn = "{$db['type']}:host={$db['host']}; dbname={$db['name']}; charset={$db['charset']}; port={$db['port']}";//数据源
try {
//实例化PDO类,创建PDO对象
$pdo = new PDO($dsn,$db['user'],$db['pass']);
} catch (PDOException $e) {
die('数据库错误:'.$e->getMessage());
}
return $pdo;
}
}
//实例化父类的对象
$c1=new con();
// 链接数据库
$pdo=$c1->construct('127.0.0.1','root','root','dedecmsv57');
<?php
//引入connect数据库链接文件中的con类
require __DIR__.'/connect.php';
//创建con的子类find
class find extends con{
public $table;
public $fields;
public $where;
public $order;
public $limit;
//创建子类的查询find
public function select($table,$fields, $where='', $order='',$limit=''){
$this->table = $table;
$this->fields=$fields;
$this->where=$where;
$this->order=$order;
$this->limit=$limit;
//实例化父类的对象
$c1=new con();
// 链接数据库
$pdo=$c1->construct('127.0.0.1','root','root','dedecmsv57');
//创建SQL语句
$sql = 'SELECT ';
if (is_array($fields)) {
foreach ($fields as $field) {
$sql .= $field.', ';
}
} else {
$sql .= $fields;
}
$sql = rtrim(trim($sql),',');
$sql .= ' FROM '.$table;
//查询条件
if(!empty($where)){
$sql .= ' WHERE '.$where;
}
//排序条件
if(!empty($order)) {
$sql .= ' order by '.$order;
}
//分页条件
if(!empty($limit)) {
$sql .= ' limit '.$limit;
}
$sql .= ';';
//创建PDO预处理对象
$stmt = $pdo->prepare($sql);
//执行查询操作
if($stmt->execute()){
if($stmt->rowCount()>0){
$stmt->setFetchMode(PDO::FETCH_ASSOC);
//返回一个二维数组
return $stmt->fetchAll();
}
} else {
return false;
}
}
// 查询单条记录
public function find2($table,$fields,$where=''){
//实例化父类的对象
$c1=new con();
// 链接数据库
$pdo=$c1->construct('127.0.0.1','root','root','dedecmsv57');
$sql = 'SELECT ';
if (is_array($fields)) {
foreach ($fields as $field) {
$sql .= $field.', ';
}
} else {
$sql .= $fields;
}
$sql = rtrim(trim($sql),',');
$sql .= ' FROM '.$table;
//查询条件
if(!empty($where)){
$sql .= ' WHERE '.$where;
}
$sql .= ' LIMIT 1;';
//创建PDO预处理对象
$stmt = $pdo->prepare($sql);
//执行查询操作
if($stmt->execute()){
if($stmt->rowCount()>0){
$stmt->setFetchMode(PDO::FETCH_ASSOC);
return $stmt->fetch();
}
} else {
return false;
}
}
//* 新增数据
public function insert($table,$data=[]){
//实例化父类的对象
$c1=new con();
// 链接数据库
$pdo=$c1->construct('127.0.0.1','root','root','dedecmsv57');
$sql = "INSERT INTO {$table} SET ";
//组装插入语句
if(is_array($data)){
foreach ($data as $k=>$v) {
$sql .= $k.'="'.$v.'", ';
}
}else{
return false;
}
//去掉尾部逗号,并添加分号结束
$sql = rtrim(trim($sql),',').';';
//创建PDO预处理对象
$stmt = $pdo->prepare($sql);
//执行新增操作
if($stmt->execute()){
if($stmt->rowCount()>0){
return true;
}
} else {
return false;
}
}
//* 统计数量
public function count_num($table,$where=''){
//实例化父类的对象
$c1=new con();
// 链接数据库
$pdo=$c1->construct('127.0.0.1','root','root','dedecmsv57');
$sql = 'SELECT count(*) as count_number FROM '.$table;
//查询条件
if(!empty($where)){
$sql .= ' WHERE '.$where;
}
//创建PDO预处理对象
$stmt = $pdo->prepare($sql);
//执行查询操作
if($stmt->execute()){
if($stmt->rowCount()>0){
$row = $stmt->fetch(PDO::FETCH_ASSOC);
$rows = $row['count_number'];
return $rows;
}
} else {
return false;
}
}
}
//实例化对象
$s1=new find();
//查询到的数据是一个二维数组
$find1=$s1->select('dede_addonarticle','aid,typeid,userip,body');
//输出二维数组的body字段
foreach ($find1 as $v){
echo $v['userip'].'<br>';
echo $v['body'].'<br>';
}
// 查询单条记录
$value=$s1->find2('dede_addonarticle','aid,typeid,userip,body');
echo $value['body'];
//* 更新数据
$s1->insert('dede_addonarticle','[1,20,"body里的文章内容比较多","我也不知道这是啥","这也不知道","2"]');
//* 统计数量
$a=$s1->count_num('dede_addonarticle');
echo $a;