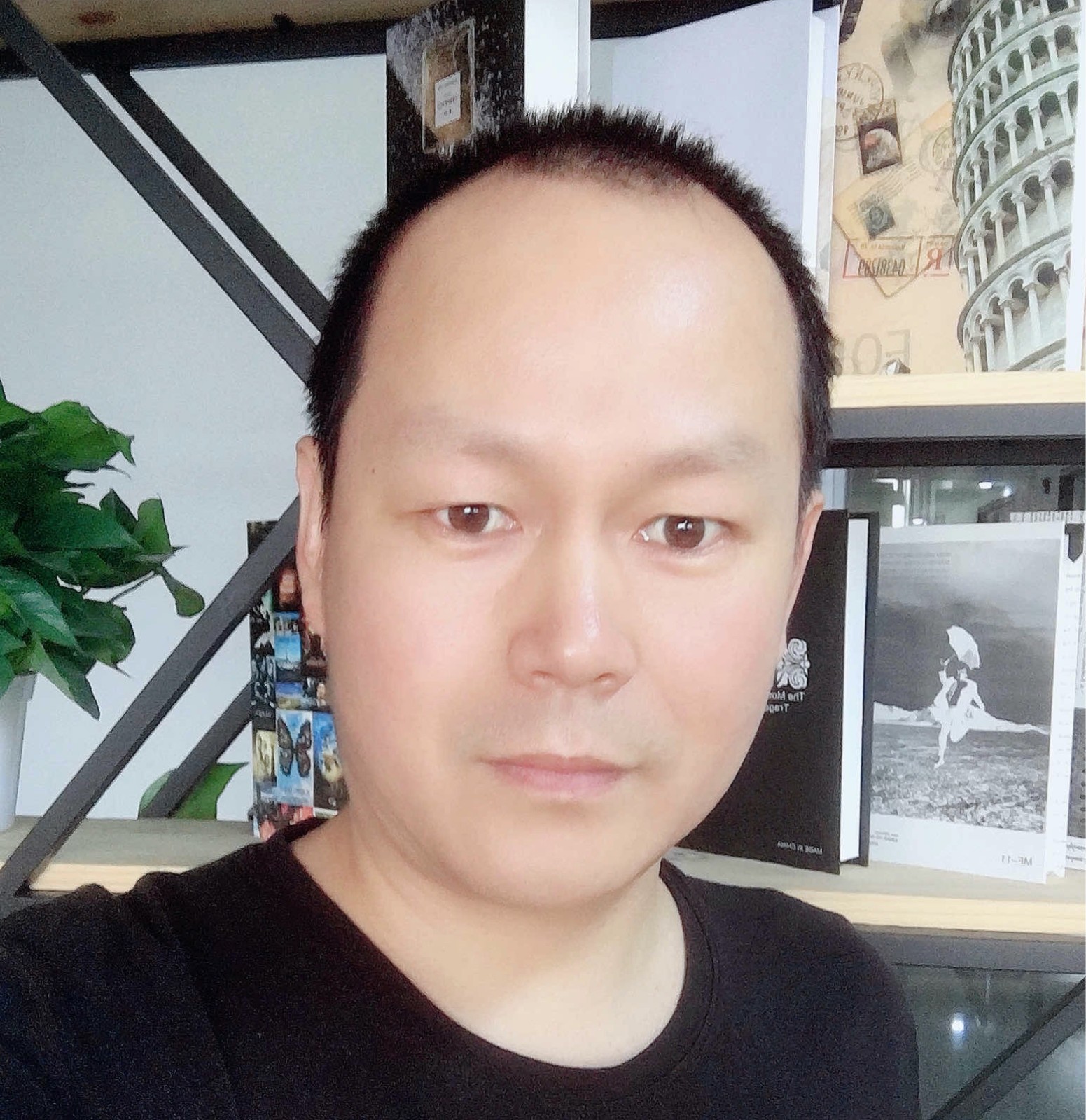
Correction status:qualified
Teacher's comments:完成的挺不错, 对于类中的一些成员的基本功能都能明白
<?php
// 把pdo写到类中
class Db
{
public $dsn;
public $username;
public $password;
public $pdo;
public function __construct($dsn, $username, $password)
{
$this->dsn = $dsn;
$this->username = $username;
$this->password = $password;
// return new PDO($this->dsn,$this->username,$this->password);
$this->connect();
}
public function connect()
{
try {
$this->pdo = new PDO($this->dsn, $this->username, $this->password);
} catch (PDOException $e) {
die(print_r($e->getMessage(), true));
}
return $this->pdo;
}
//新增操作
public function insert($table, $data = [])
{
//连接sql数据库
//组装sql语句
$sql = "INSERT INTO {$table} SET ";
// print_r($data);
if (is_array($data) && !empty($data)) {
foreach ($data as $k => $v) {
$sql .= '`' . $k . '`="' . $v . '",';
}
} else {
return false;
}
$sql = rtrim(trim($sql), ',') . ';';
// print_r($sql);exit();
//创建预处理对象
$stmt = $this->pdo->prepare($sql);
//执行sql操作
$res = $stmt->execute();
//返回操作结果
if ($res) {
if ($stmt->rowCount() > 0) {
return '成功添加了' . $stmt->rowCount() . '条数据';
} else {
return '没有新增数据';
}
} else {
return false;
}
//关闭sql链接
}
//修改操作
public function update($table, $data = [], $where = '')
{
$sql = "UPDATE {$table} SET ";
if (is_array($data) && !empty($data)) {
foreach ($data as $k => $v) {
$sql .= '`' . $k . '`="' . $v . '",';
}
} else {
return false;
}
$sql = rtrim(trim($sql), ',') . ' ';
if (!empty($where)) {
$sql .= 'WHERE ' . $where . ';';
} else {
return false;
}
// print_r($sql);exit();
//创建预处理对象
$stmt = $this->pdo->prepare($sql);
//执行SQL操作
if ($stmt->execute()) {
if ($stmt->rowCount() > 0) {
return '成功更新了' . $stmt->rowCount() . '条数据';
} else {
return '没有数据被更新';
}
} else {
return false;
}
}
//删除操作
public function delete($table, $where = '')
{
$sql = "DELETE FROM {$table} ";
if (!empty($where)) {
$sql .= 'WHERE ' . $where . ';';
} else {
die('删除必须加条件');
}
// print_r($sql); exit();
$stmt = $this->pdo->prepare($sql);
if ($stmt->execute()) {
if ($stmt->rowCount() > 0) {
return '成功删除了' . $stmt->rowCount() . '条数据';
} else {
return '找不到要删除的数据';
}
} else {
return false;
}
}
//查询操作
//查询单条数据
public function find($table, $fields, $where = '')
{
$sql = "SELECT ";
if (is_array($fields)) {
foreach ($fields as $v) {
$sql .= $v . ',';
}
} else {
$sql .= $fields;
}
$sql = rtrim(trim($sql), ',');
$sql .= ' FROM ' . $table;
if (!empty($where)) {
$sql .= ' WHERE ' . $where;
}
$sql .= ' LIMIT 1;';
$stmt = $this->pdo->prepare($sql);
$find = $stmt->execute();
if ($find) {
return $stmt->fetch(PDO::FETCH_ASSOC);
} else {
return false;
}
}
//查询多条数据
public function select($table, $fields, $where = '', $order = '', $limit = '')
{
$sql = "SELECT ";
if (is_array($fields)) {
foreach ($fields as $field) {
$sql .= $field . ',';
}
} else {
$sql .= $fields;
}
$sql = rtrim(trim($sql), ',');
$sql .= ' FROM ' . $table;
if (!empty($where)){
$sql .= ' WHERE '. $where;
}
if (!empty($order)){
$sql .= ' ORDER BY '.$order;
}
if (!empty($limit)){
$sql .= ' LIMIT '.$limit . ';';
}
// print_r($sql);
$stmt = $this->pdo -> prepare($sql);
if($stmt->execute()){
return $stmt->fetchAll(PDO::FETCH_ASSOC);
}
}
//析构函数
public function __destruct()
{
$this->pdo = null;
}
}
$db = new Db("mysql:host=localhost;dbname=movies", 'root', 'root');
//print_r($db->pdo);
//print_r($db->pdo);
//if($db->pdo){
// echo '连接成功';
//}
//插入操作
//$insert = $db->insert('category',['name'=>'ds','alias'=>'都市生活剧']);
//echo $insert;
//更新操作
//$update = $db->update('category', ['name' => 'xj', 'alias' => '喜剧片'], '`cate_id`=23');
//echo $update;
//删除操作
//$del = $db->delete('category', '`cate_id` = 24');
//echo $del;
//查找单条记录
//$res = $db->find('category','*','cate_id = 25');
//print_r($res);
//查找多条记录
$sel = $db->select('category', '*','name="gz"','cate_id DESC','0,10');
print_r($sel);