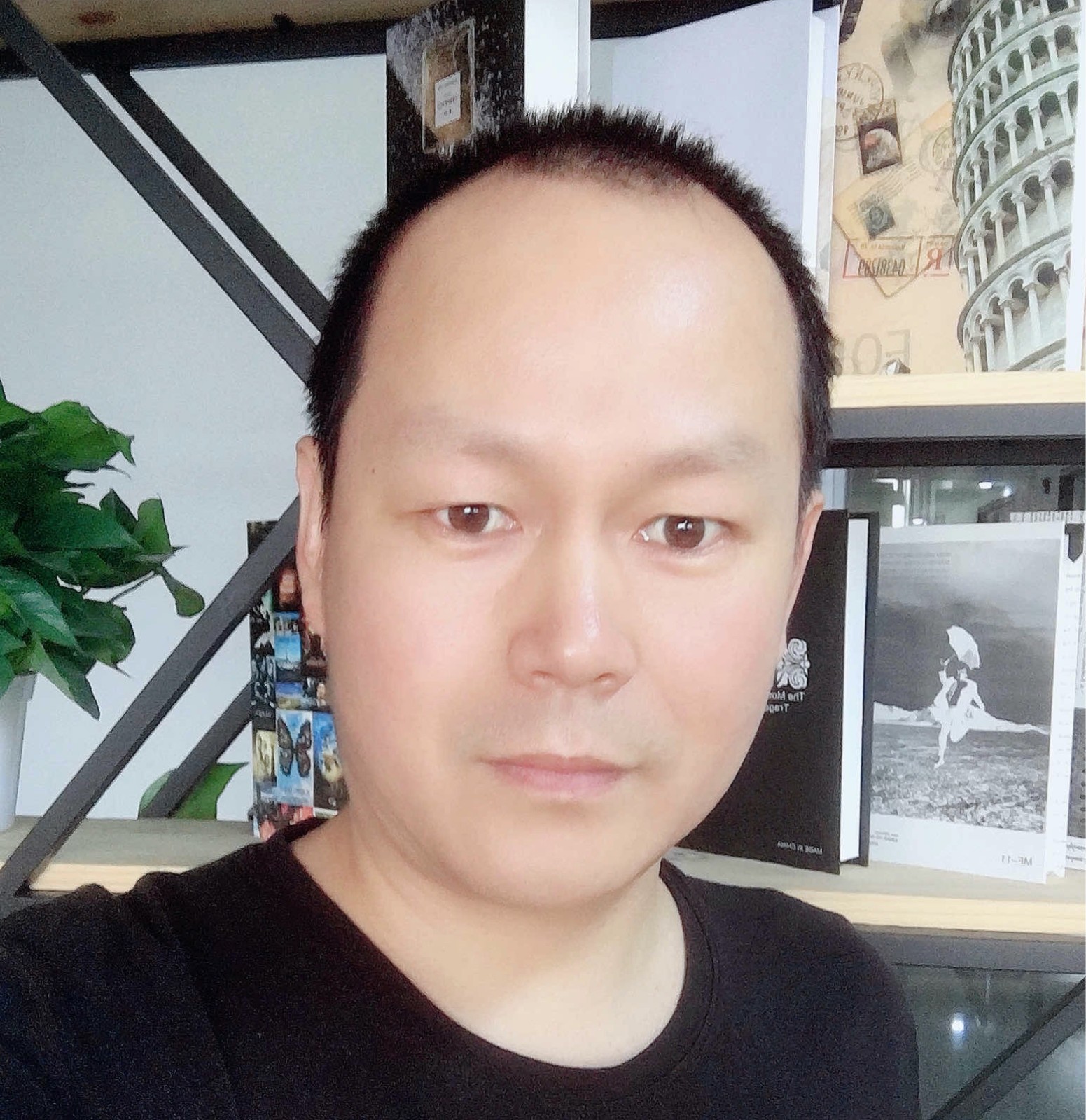
Correction status:qualified
Teacher's comments:复习课程 的作业 , 还能这样认真的完成, 相当棒
<?php//添加类class Demo1{ //添加类成员 public $site ='php中文网'; public function getsite() { }}//访问类成员$obj= new Demo1;echo $obj->site;
<?php//添加类class Demo2{ //添加类成员 public $site ='php中文网'; public $role='讲师'; public function getsite() { //访问类成员// $obj= new self;// return $obj->site; return $this->site; } public function getrole() { //访问类成员// $obj= new self;// echo $obj->role; //$this是当前类的实例的引用,它始终与当前类的实例绑定// self绑定当前类,$this是绑定当前类的实例。 return $this->role; }}//访问类成员$obj= new Demo2(); //函数调用。$obj= new Demo2;echo $obj->site;echo $obj->getrole();
<?php//添加类class Demo3{ //添加类成员 public $site; public $role; public function getinfo() { return '我是:'.$this->site . '来自'. $this->role ; } //构建方法:1.类实例的初始化;2.自动完成在类实例创建过程中的操作; public function __construct($site,$role) { //1.初始化类成员 $this->site = $site; $this->role = $role;// //2.创建类实例// $obj= new self;////// //3.添加类成员// $obj->site =$this->site;// $obj->role =$this->role; echo $this->getinfo(); //4.返回类实例// return $obj; }}//访问类成员 new Demo3('朱老师','php中文网');echo '<hr>';class Demo33{ public $site; public $role; public function getsite(){ return '我是:'. $this->site . '来自' . $this->role; } public function __construct($site,$role) { $this->site = $site; $this->role = $role; echo $this->getsite(); }}new Demo33('朱老师','PHP中文网');
<?php //添加类 class Demo4{ //添加类成员...private私有成员。protected类内子类可访问。 public $site; protected $role; public function getinfo() { return '我是:'.$this->site . '来自'. $this->role ; } //构建方法:1.类实例的初始化;2.自动完成在类实例创建过程中的操作; public function __construct($site,$role) { $this->site = $site; $this->role = $role; }// public function getrole()// {// $username = $_GET['username'] ?? '';// if (isset($username)&& $username ==='admin')// {// return $this->role;// }else// {// return '无权访问';// }// }// public function __get($name)// {// return isset($this->$name) ? $this->$name :'未定义属性';// } public function __get($name) { $username = $_GET['username'] ?? ''; if (isset($username)&& $username ==='admin') { return isset($this->$name) ? $this->$name :'未定义属性'; }else { return '无权访问'; } }}//访问类成员$obj =new Demo4('www.php.cn','PHP中文网');// echo $obj->getrole($name);// echo $obj->name;echo $obj->role;echo $obj->name;
<?php //添加类 class Demo5 { //添加类成员...private私有成员。protected类内子类可访问。 public $site; protected $role; public function getinfo() { return '这里是:'.$this->site . ' 讲师是:'. $this->role ; } //构建方法:1.类实例的初始化;2.自动完成在类实例创建过程中的操作; public function __construct($site,$role) { $this->site = $site; $this->role = $role; } } class Demo5_1 extends Demo5 { private $course; public function __construct($site, $role,$course) { parent::__construct($site, $role); $this->course = $course; } public function getinfo() { return parent::getinfo() .',负责的课程是:'.$this->course; // TODO: Change the autogenerated stub } }//访问类成员$sub =new Demo5_1('php中文网','神奇朱老师','php编程'); echo $sub->getinfo();
<?php//trait不能实例化,不是类,当做公共方法库。trait Test{ public function getinfo() { return '这里是:'.$this->site . ' 讲师是:'. $this->role; }} //添加类 class Demo6 { //添加类成员...private私有成员。protected类内子类可访问。 public $site; protected $role; use Test;// public function getinfo()// {// return '这里是:'.$this->site . ' 讲师是:'. $this->role ;// } //构建方法:1.类实例的初始化;2.自动完成在类实例创建过程中的操作; public function __construct($site,$role) { $this->site = $site; $this->role = $role; } }// trait:还可以用在类的父子类关系中 class Demo6_1 extends Demo6 { private $course; public function __construct($site, $role,$course) { parent::__construct($site, $role); $this->course = $course; } public function getinfo() { return parent::getinfo() .',负责的课程是:'.$this->course; // TODO: Change the autogenerated stub } }//访问类成员$sub =new Demo6('php中文网','Peter zhu'); echo $sub->getinfo(); echo '<hr>';$sub2 =new Demo6_1('php中文网','Peter zhu','子类加第三个');echo $sub2->getinfo();
<?php//接口//对象的模板是类,类的模板是接口。//object——class--interface//面向接口编程是最重要的面向对象编程思想之一。//接口中没有方法的具体实现,接口不能实例化。interface iDemo{ //接口方法 public function getinfo(); public function hello();} //添加类// Demo7工作类 class Demo7 implements iDemo { //添加类成员...private私有成员。protected类内子类可访问。 public $site; protected $role; public function getinfo() { return '这里是:'.$this->site . ' 讲师是:'. $this->role ; } public function hello() { return'hello,大家吃饱了吧'; // TODO: Implement hello() method. } //构建方法:1.类实例的初始化;2.自动完成在类实例创建过程中的操作; public function __construct($site,$role) { $this->site = $site; $this->role = $role; } }//访问类成员$obj=new Demo7('Demo7第一个参数','demo7---2参数');echo $obj->getinfo().'<br>';echo $obj->hello();
<?php //抽象类:不能实例化,做父类。 //接口中有抽象方法,抽象中有抽象方法也有已实现的方法。 abstract class Chouxiang { abstract public function getinfo(); public function hello() { return'hello,大家吃饱了吧'; } } //添加类// Demo8工作类 class Demo8 extends Chouxiang { //添加类成员...private私有成员。protected类内子类可访问。 public $site; protected $role; public function getinfo() { return '这里是:'.$this->site . ' 讲师是:'. $this->role ; }// public function hello()// {// return'hello,大家吃饱了吧'; // TODO: Implement hello() method.// } //构建方法:1.类实例的初始化;2.自动完成在类实例创建过程中的操作; public function __construct($site,$role) { $this->site = $site; $this->role = $role; } }//访问类成员$obj=new Demo8('Demo8第一个参数','demo8---2参数');echo $obj->getinfo().'<br>';echo $obj->hello();