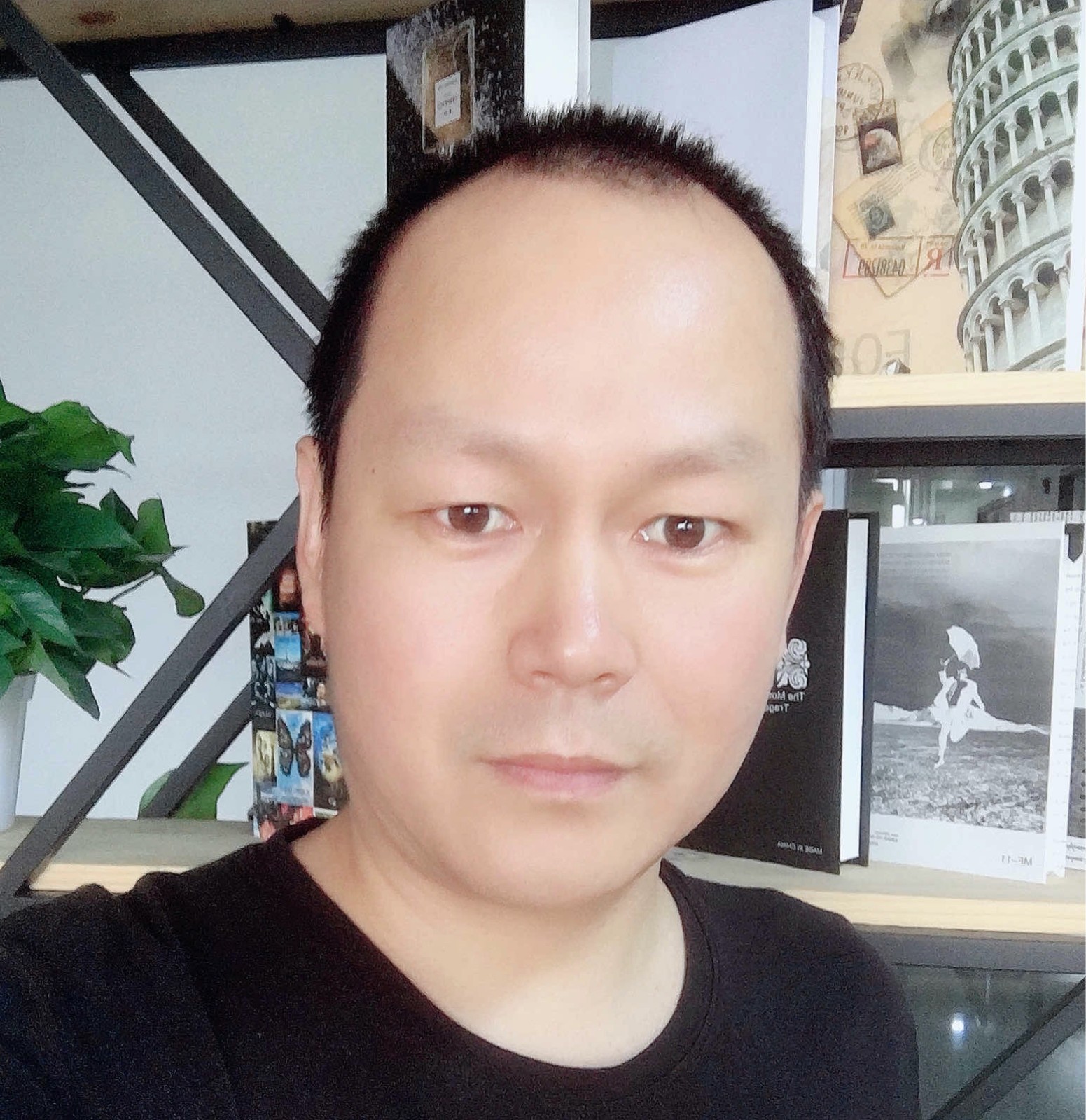
Correction status:qualified
Teacher's comments:听懂已经很不容易了, 毕竟我们是天天上课, 大家很辛苦
`<?php
//1、创建类
class Demo1{
//2、添加类成员
// 类成员分为
// 1、属性 对应外面的变量
// 2、方法 对应外面的函数
public $a = ‘张三’;
public function getInfo()
{
$obj = new Demo1();
return $obj -> a . ‘hello’;
}
}
//3、访问类成员
$obj = new Demo1();
echo $obj ->a;
echo ‘<br>‘;
echo $obj -> getInfo();
echo ‘<br>‘;
echo $obj ->a;
echo ‘<br>‘;
class Demo2{
//2、添加类成员
// 类成员分为
// 1、属性 对应外面的变量
// 2、方法 对应外面的函数
public $a = '李四';
public function getInfo1()
{
echo $this ->a;
}
}
$obj=new Demo2();
echo $obj->getInfo1();
`
`<?php
//1
class Demo2{
public $ab = ‘李四’;
public $bb =’讲师’;
public function getAb()
{
return $this -> ab ;
}
public function getBb()
{
return $this -> bb;
}
}
$obj = new Demo2();
echo $obj->getAb() . ‘<br>‘;
echo $obj->getBb() . ‘<br>‘;
`
`<?php
//1
class Demo3{
//2
public $aa;
public $bb;
public function __construct($aa,$bb)
{
$this ->aa =$aa;
$this ->bb =$bb;
}
public function getInfo()
{
return '我是: ' . $this->aa . $this->bb;
}
}
//3
$obj =new Demo3(‘李四’,’讲师’);
echo $obj->getInfo();
echo ‘<hr>‘;
class Demo4{
//2
public $aa;
public $bb;
public function __construct($aa,$bb)
{
$this ->aa =$aa;
$this ->bb =$bb;
echo $this->getInfo();
}
public function getInfo()
{
return '我是: ' . $this->aa . $this->bb;
}
}
//3
new Demo4(‘李四1’,’讲师’);
//echo $obj->getInfo();`
`<?php
//1
$_GET[‘username’]=’admin’;
echo $_GET[‘username’];
echo ‘<hr>‘;
class Demo4{
// 2
public $aa;
public $bb;
public function __construct($aa,$bb)
{
$this -> aa = $aa;
$this -> bb = $bb;
}
public function getInfo(){
return '我是: ' . $this->aa . $this->bb;
}
// public function get($name)
// {
// $username = $_GET[‘username’] ?? ‘’;
// if(isset($username)&& $username === ‘admin’){
// return isset($this->$name) ? $this -> $name: ‘属性未定义’;
// }else{
// return ‘无权访问’;
// }
// }
public function get($name)
{
// 仅允许用户名是’admin’的用户访问,其它访问返回: 无权访问
$username = $_GET[‘username’] ?? ‘’;
if (isset($username) && $username === ‘admin’) {
return isset($this->$name) ? $this->$name : ‘属性未定义’;
} else {
return ‘无权访问’;
}
}
}
$obj = new Demo4(‘www.php.cn’,’讲师’);
echo $obj->aa;
echo ‘<br>‘;
echo $obj->getInfo();
echo ‘<br>‘;
echo $obj->name;
`
`<?php
class Demo5
{
public $aa;
public $bb;
public function __construct($aa,$bb)
{
$this ->aa =$aa;
$this ->bb =$bb;
}
public function getInfo(){
return '我是: ' . $this->aa . '讲师: ' . $this->bb;
}
}
class Demo6 extends Demo5
{
private $cc;
public function construct($aa, $bb,$cc)
{
parent::construct($aa, $bb);
$this -> cc =$cc;
}
public function getInfo()
{
return parent::getInfo() . 'aaa' . $this->cc; // TODO: Change the autogenerated stub
}
}
$stu = new Demo5(‘gzg’,’aa’);
echo $stu -> getInfo();
echo ‘<hr>‘;
$obj = new Demo6(‘hyx’,’老师’,’php’);
echo $obj -> getInfo();`
`<?php
trait Test
{
public function getInfo()
{
return ‘我是: ‘ . $this->aa . ‘讲师: ‘ . $this->bb;
}
}
class Demo6
{
use Test;
public $aa;
public $bb;
public function __construct($aa,$bb)
{
$this->aa =$aa;
$this -> bb = $bb;
}
}
class Demo7 extends Demo6 {
private $cc;
public function __construct($aa, $bb,$cc)
{
parent::__construct($aa, $bb);
$this->cc =$cc;
}
}
$obj = new Demo6(‘hyx’,’111’);
echo $obj -> getInfo();
echo ‘<hr>‘;
$sub= new Demo7(‘gzg’,’222’,’abx’);
echo $sub -> getInfo();
`
`<?php
interface iDemo
{
public function getInfo();
public function hello();
}
//1
class Demo7 implements iDemo
{
// 2
public $aa;
public $bb;
public function __construct($aa,$bb)
{
$this ->aa =$aa;
$this -> bb =$bb;
}
public function getInfo()
{
return ‘我是: ‘ . $this->aa . ‘讲师: ‘ . $this->bb;
}
public function hello()
{
return 'Hello 大家晚上吃饱了吗?';
}
}
//3
$obj = new Demo7(‘hyx’,’111’ );
echo $obj ->getInfo() .’<br>‘;
echo $obj -> hello() .’<br>‘;`
`<?php
abstract class Demo
{
abstract public function getInfo();
public function hello()
{
return ‘Hello 大家晚上吃饱了吗?’;
}
}
class Demo8 extends Demo
{
public $aa;
public $bb;
public function __construct($aa,$bb)
{
$this->aa =$aa;
$this ->bb =$bb ;
}
public function getInfo()
{
return '我是: ' . $this->aa . '讲师: ' . $this->bb;
// TODO: Implement getInfo() method.
}
}
$obj = new Demo8('hyx','111');
echo $obj->getInfo() . ‘<br>‘;
echo $obj->hello() . ‘<br>‘;`
学习了 OOP编程,可以听懂,在简单案例中也能使用。希望在后面的案例中能学会灵活应用。