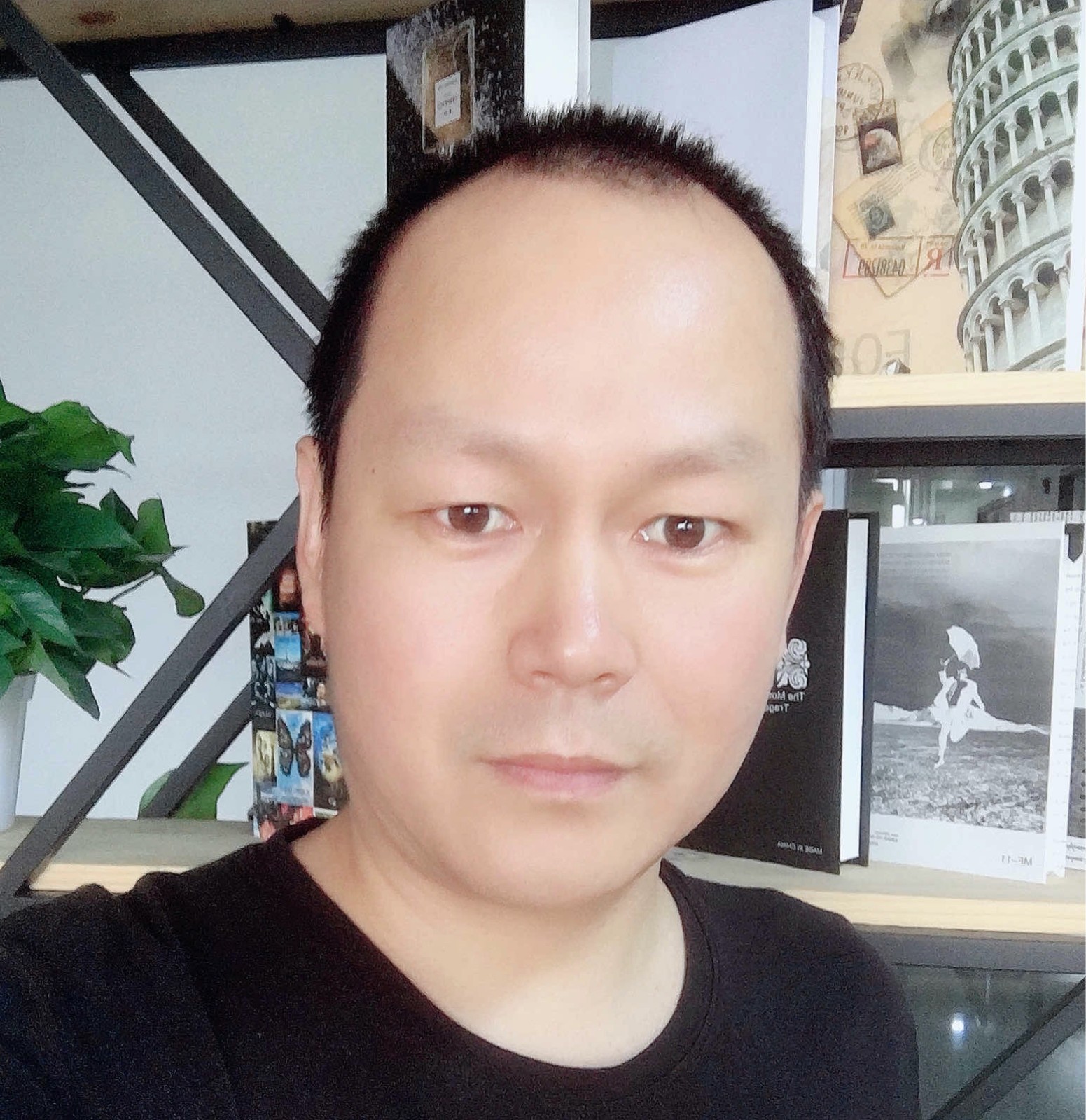
Correction status:qualified
Teacher's comments:总结的非常棒, 也很完整, 字不多, 但精彩
//1,创建类
class Demo
{
//2,添加类成员
public $site = 'PHP中文网';
public $role = '讲师';
public function getSite(){
return $this->site.' 欢迎您';
}
public function getRole(){
return $this->role;
}
}
//3,访问类成员
$obj = new Demo();
echo $obj->site .'<br>';
echo $obj->getRole() . '<br>';
echo $obj->getSite() . '<br>';
运行效果:
class Demo2
{
public $site;
public $role;
public function getInfo()
{
return '我是:' . $this->site . $this->role;
}
//构造方法:实例化即执行
public function __construct($site,$role){
$this->site = $site;
$this->role = $role;
echo $this->getInfo();
}
}
new Demo2('PHP中文网','老师');
运行效果:
class Demo3
{
public $site;
protected $role;
public function getInfo()
{
return '我是:' . $this->site . $this->role;
}
//构造方法
public function __construct($site, $role)
{
$this->site = $site;
$this->role = $role;
}
//魔术方法:__get($name) ,属性重载
public function __get($name)
{
$username = isset($_GET['username']) ? $_GET['username'] : '';
if (isset($username) && $username == 'admin'){
return isset($this->$name) ? $this->$name : '属性未定义';
}else{
return '无权访问';
}
}
}
$obj = new Demo3('PHP中文网','讲师');
echo $obj->role;
echo '<hr>';
echo $obj->name;
//$this->name 这是访问类中的一个名为name的成员变量
//$this->$name 这是访问对象中的可变变量,可以理解成动态变量,变量名由$name的值决定,
//如果$name = 'site' 那么 $this->$name 就等于$this->site 再查找当前类中是否有属性为site的成员变量
运行效果:
class Demo4
{
public $site;
protected $role;
public function getInfo(){
return '我是:' . $this->site . ' 讲师:' . $this->role;
}
public function __construct($site,$role)
{
$this->site = $site;
$this->role = $role;
}
}
class Demo4_1 extends Demo4
{
private $course;
public function __construct($site, $role, $course)
{
parent::__construct($site, $role);
$this->course = $course;
}
public function getInfo()
{
return parent::getInfo() . ',负责的课程是:' . $this->course;
}
}
$obj = new Demo4_1('php.cn','朱老师','php');
echo $obj->getInfo();
运行效果:
//trait 当成一个公共方法库,不能实例化
trait Test
{
public function getInfo(){
return '我是:'. $this->site . ' 讲师:' . $this->role;
}
}
class Demo5
{
//导入trait类库
use Test;
public $site;
protected $role;
public function __construct($site,$role)
{
$this->site = $site;
$this->role = $role;
}
}
class Demo5_1 extends Demo5{
private $course;
public function __construct($site, $role,$course)
{
parent::__construct($site, $role);
$this->course = $course;
}
}
// 优先级: 当前类中的同名方法 > trait类中的同名方法 > 父类中的同名方法
$demo5 = new Demo5('PHP中文网','朱老师');
echo $demo5->getInfo();
echo '<hr>';
$obj = new Demo5_1('php.cn','zhulaoshi','PHP');
echo $obj->getInfo();
运行效果:
//接口:定义工作类中的方法原型
interface iDemo{
public function getInfo();
public function hello();
}
class Demo6 implements iDemo
{
public $site;
protected $role;
public function getInfo()
{
return '我是:' . $this->site . '讲师:'. $this->role;
}
public function hello(){
return 'Hello Word!';
}
public function __construct($site,$role)
{
$this->site = $site;
$this->role = $role;
}
}
$obj = new Demo6('PHP中文网','朱老师');
echo $obj->getInfo();
echo '<hr>';
echo $obj->hello();
运行效果:
//抽象类
abstract class Cx
{
abstract public function getInfo();
public function hello(){
return 'Hello Word!';
}
}
class Demo7 extends Cx
{
public $site;
protected $role;
public function getInfo()
{
return '我是:' . $this->site . '讲师' . $this->role;
}
public function __construct($site,$role)
{
$this->site = $site;
$this->role = $role;
}
}
$obj = new Demo7('php中文网','朱老师');
echo $obj->getInfo() . '<br>';
运行效果:
手写:
1, 面向对象编程的基本流程:创建类,添加类成员,访问类成员
2, self
引用当前类,$this
引用当前类的实例/对象
3, __construct
构造方法,实例化类时就会同步执行
4, 访问控制符punlic
类内、类外、子类均可见,protected
类内、子类可见,类外不可见,private
仅本类内可访问
5, $this->name
这是访问类中的一个名为name的成员变量
6, $this->$name
这是访问对象中的可变变量,可以理解成动态变量,变量名由$name
的值决定, 如果$name = 'site'
那么 $this->$name
就等于$this->site
再查找当前类中是否有属性为site的成员变量
7, trait
: 当成一个公共方法库,使用了类的定义的语法,但不是类,所以不能实例化,用use
导入到类中使用
8, 优先级: 当前类中的同名方法 > trait
类中的同名方法 > 父类中的同名方法
9, interface
接口只允许出现方法和常量,可见性必须为public
,不能有方法体,接口中的方法必须都为抽象方法
10, 接口是一种约定, 定义了实现它的类中必须实现的方法,没有方法的具体实现, 所以不能实例化
11, implements
类实现接口的关键字
12, abstract
定义抽象方法、抽象类
13, 抽象类只能被继承,不能实例化,且抽象方法都必须在子类复写
14, 抽象方法不能有方法体,不能有{}