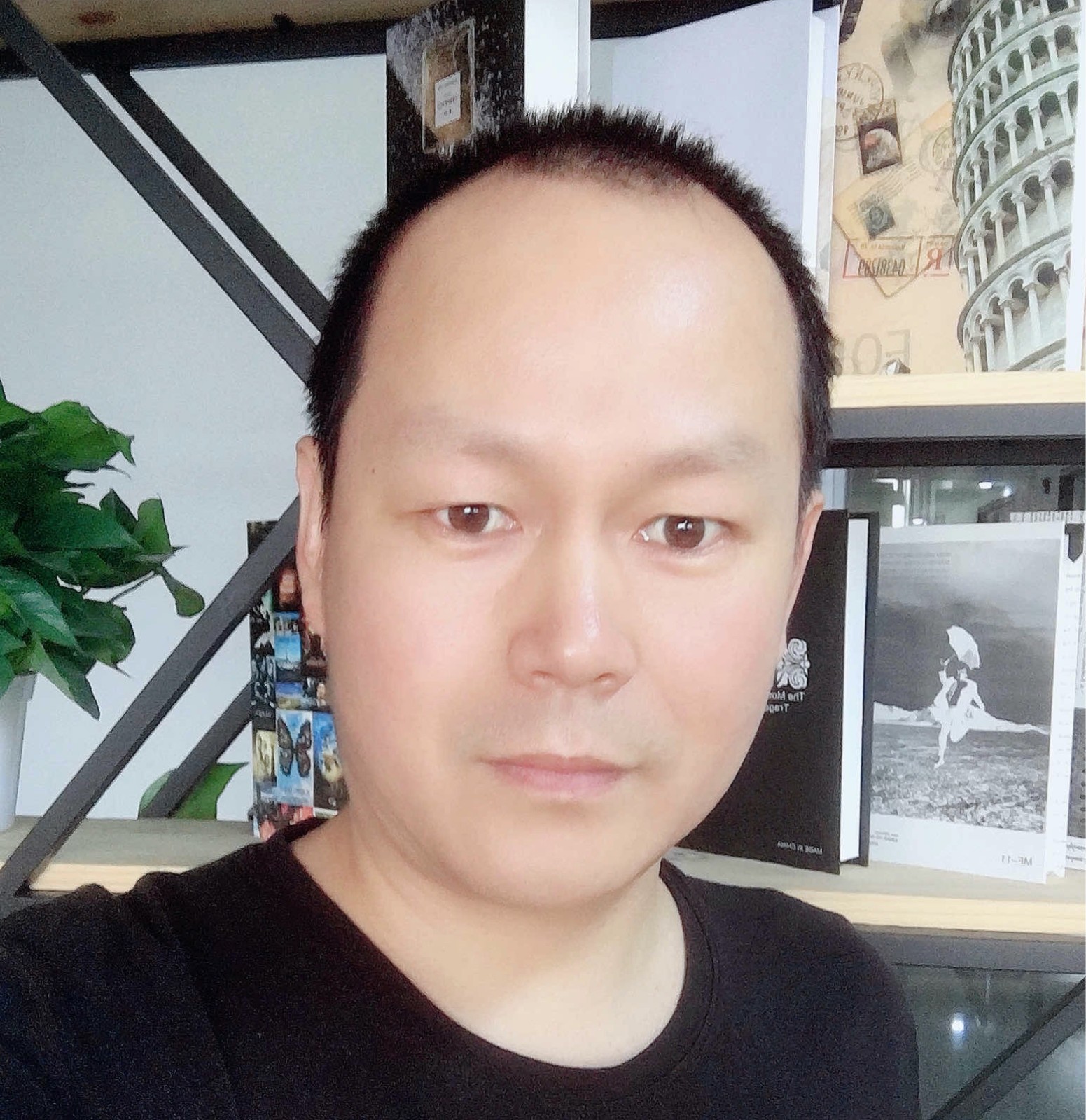
Correction status:qualified
Teacher's comments:听讲你的博客被清空了, 正好趁这个时机, 把前面的作业再重写一遍, 会大有收获的
// 1. 创建类
class Demo1
{
// 2. 添加类成员
// 在类中的变量和函数,和程序中的变量与函数有区别, 添加了访问限制符
// 变量=>属性, 函数=>方法, 访问限制符 成员
// 创建属性
public $site = 'php中文网';
// 创建方法: 输出当前类的属性$site
public function getSite()
{
// 第一步:类的实例化
$obj = new Demo1;
// 第二步: 返回类属性
return $obj->site . ' 欢迎您~~';
}
}
// 3. 访问类成员
$obj = new Demo1;
// 访问属性: 对象成员访问符: ->
echo $obj->site . '<br>';
echo $obj->getSite();
self
: 引用当前类$this
: 引用当前类的实例/对象
class Demo
{
private $name;
private $age;
public function __construct($name, $age)
{
//$obj = new self();
$this->name = $name;
$this->age = $age;
}
public function getInfo()
{
return $this->name . '--' . $this->age;
}
}
$obj = new Demo('唐僧', 30);
echo $obj->getInfo(); //唐僧--30
__construct()
: 类实例化自动调用,完成对象的初始化new
: 当用new调用一个类名称函数时会触发类中的构造方法
<?php
class Demo
{
private $name;
private $age;
//当类被实例化,构造方法就会自动完成方法内的所有操作
public function __construct($name, $age)
{
$this->name = $name;
$this->age = $age;
$this->getInfo();
}
public function getInfo()
{
return $this->name.'--'.$this->age;
}
}
echo new Demo('唐僧', 30); //唐僧--30
public
: 本类, 类外, 子类均可访问private
: 仅本类可访问protected
: 本类,子类可访问,类外不可见
class Demo{
private $name;
private $age;
public function __construct($name, $age)
{
$this->name = $name;
$this->age = $age;
}
//使用成员方法来访问 私有化成员属性
public function getInfo(){
return $this->name.'--'.$this->age;
}
// 魔术方法: __get($name), 属性重载
public function __get($name)
{
// 仅允许用户名是'admin'的用户访问,其它访问返回: 无权访问
$username = $_GET['username'] ?? '';
if (isset($username) && $username === 'admin') {
return isset($this->$name) ? $this->$name : '属性未定义';
} else {
return '无权访问';
}
}
}
$obj = new Demo('唐僧',30);
echo $obj->getInfo(); //唐僧--30
echo $obj->sex; //属性未定义
protected
受保护成员,可以子类中访问extends
设置子类继承的父类parent
子类中引用父类成员代码
class Demo{
public $name;
public $age;
public function __construct($name, $age)
{
$this->name = $name;
$this->age = $age;
}
}
class Child extends Demo {
public $sex;
/**
* Child constructor.
* @param $sex
*/
public function __construct($name, $age, $sex)
{
parent::__construct($name,$age);
$this->sex = $sex;
}
public function getInfo(){
return $this->name.'--'.$this->age.'--'.$this->sex;
}
}
$obj = new Child('唐僧',30);
echo $obj->getInfo(); //唐僧--30--男
trait
声明trait类,实现类的多继承与方法复用use
在类中插入trait类代码
trait Common{
public function getInfo(){
return $this->name.'--'.$this->age.'--'.$this->sex;
}
}
class Demo{
public $name;
public $age;
public function __construct($name, $age)
{
$this->name = $name;
$this->age = $age;
}
}
class Child extends Demo {
//使用trait技术,实现了Child类继承多累的需求
use Common;
public $sex;
/**
* Child constructor.
* @param $sex
*/
public function __construct($name, $age, $sex)
{
parent::__construct($name,$age);
$this->sex = $sex;
}
}
$obj = new Child('唐僧',30,'男');
echo $obj->getInfo(); //唐僧--30--男
interface
定义接口implements
实现接口
interface iCommon{
public function hello();
public function say();
}
class Demo implements iCommon {
public $name;
public $age;
public function __construct($name, $age)
{
$this->name = $name;
$this->age = $age;
}
function hello()
{
return 'hello '.$this->name;
}
function say()
{
return 'say '.$this->name;
}
}
$obj = new Demo('唐僧',30);
echo $obj->hello(); //hello 唐僧
echo $obj->say(); //say 唐僧
abstract class Common{
abstract function hello();
public function say(){
return 'say goodbye! '.$this->name;
}
}
class Demo extends Common {
public $name;
public $age;
public function __construct($name, $age)
{
$this->name = $name;
$this->age = $age;
}
function hello()
{
return 'hello '.$this->name;
}
}
$obj = new Demo('唐僧',30);
echo $obj->hello(); //hello 唐僧
echo $obj->say(); //say goodbye! 唐僧
手写:
THE END !