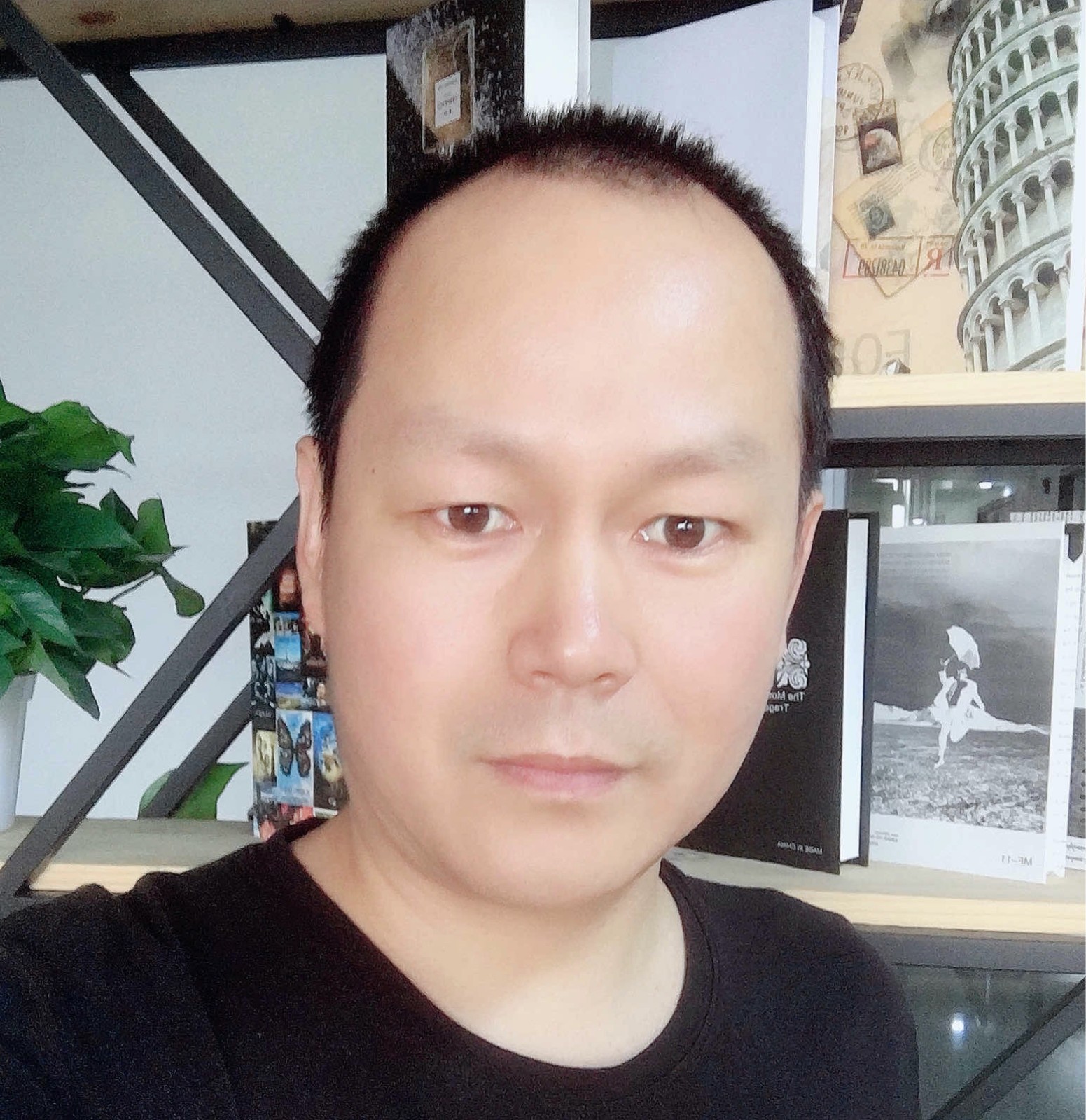
Correction status:qualified
Teacher's comments:完成的相当OK, 复习还能这么认真, 不错
// 1.创建类class Demo1{ // 2.添加类成员 // 在类中的变量和函数,和程序中的变量与函数有区别,添加了访问限制符 // 变量=>类的属性,函数=>类的方法,定义:访问限制符 成员 public $name='Ricky'; public function getName(){// 第一步,类的实例化 $obj=new Demo1; return 'Hi, ' . $obj->name; }}// 3.访问类成员$obj = new Demo1;//访问属性:对象成员访问符 " -> "echo $obj->name . '<br>';echo $obj->getName() . '<br>';//创建类class Demo2{// 2.添加类成员 public $name = 'Ricky'; public $occupation='Programmer'; public function getInfo(){// 类的实例化引用 $obj = new self 等同于 $this; return 'Name:'.$this->name.' Occupancy:'.$this->occupation; } public function getOccupation(){ return 'Your occupation is ' . $this->occupation; }}$obj2 = new Demo2;echo $obj2->getInfo().'<br>';echo $obj2->getOccupation().'<br>';// 构造函数class Demo3{ public $name; public $occupation; public function getInfo(){ return 'My name is ' . $this->name . ' and occupation is ' . $this->occupation. '<br>'; } public function __construct($name,$occupation) { $this->name = $name; $this->occupation = $occupation; echo $this->getInfo(); }}$obj3 = new Demo3('Ricky','Programmer');// __get()魔术方法,检查匹配类中属性class Demo4{ public $name; protected $occupation; public function getInfo(){ return 'My name is ' . $this->name . ' and occupation is ' . $this->occupation.'<br>'; } public function __construct($name,$occupation) { $this->name = $name; $this->occupation = $occupation; } public function __get($name){ // 匹配类中的属性 $userRole = $_GET['role'] ?? ''; if(isset($userRole) && $userRole === 'admin'){ return isset($this->$name) ? $this->$name : 'Undefined'; }else{ return 'Forbidden Visit'; } }}$obj4 = new Demo4('Ricky','Programmer');echo $obj4->rold; // 访问不存在属性//类的继承class Demo5{ public $name; protected $occupation; public function getInfo(){ return 'My name is ' . $this->name . ' and occupation is ' . $this->occupation.', '; } public function __construct($name,$occupation){ $this->name = $name; $this->occupation = $occupation; }}class Demo5_1 extends Demo5{ private $age; public function __construct($name, $occupation,$age) { parent::__construct($name, $occupation); $this->age = $age; } public function getInfo() { return parent::getInfo() . 'I\'m '.$this->age.' years old.'; }}// 访问类成员$obj5 = new Demo5_1('Ricky','Programmer','28');echo '<br>'.$obj5->getInfo();// trait: 代码复用方式,用来扩展当前类的功能// trait: 当成一个公共方法库trait First{ public function getInfo(){ return 'My name is ' . $this->name . ' and occupation is ' . $this->occupation.'<br>'; }}class Demo6{// 导入trait类库 use First; public $name; public $occupation; public function __construct($name,$occupation){ $this->name = $name; $this->occupation = $occupation; }}class Demo6_1 extends Demo6{ private $age; public function __construct($name, $occupation,$age) { parent::__construct($name, $occupation); $this->age = $age; } public function getInfo() { return parent::getInfo().'I\'m ' . $this->age . 'years old'; }}$obj6 = new Demo6_1('Ri1cky','Programmer','28');$obj6->getInfo();
// trait: 代码复用方式,用来扩展当前类的功能// trait: 当成一个公共方法库trait First{ public function getInfo(){ return 'My name is ' . $this->name . ' and occupation is ' . $this->occupation.'<br>'; }}class Demo6{// 导入trait类库 use First; public $name; public $occupation; public function __construct($name,$occupation){ $this->name = $name; $this->occupation = $occupation; }}class Demo6_1 extends Demo6{ private $age; public function __construct($name, $occupation,$age) { parent::__construct($name, $occupation); $this->age = $age; } public function getInfo() { return parent::getInfo().'I\'m ' . $this->age . 'years old'; }}$obj6 = new Demo6_1('Ri1cky','Programmer','28');echo $obj6->getInfo();
//1.创建类interface iDemo{ //接口方法 public function getInfo(); public function hello();}//工作类class Demo7 implements iDemo{ public $name; protected $occupation; public function getInfo(){ return 'My name is ' . $this->name . ' and occupation is ' . $this->occupation.'<br>'; } public function hello(){ return 'Hello everyone.'; } public function __construct($name,$occupation) { $this->name = $name; $this->occupation = $occupation; }}$obj = new Demo7('Ricky','Programmer');echo $obj->getInfo();echo $obj->hello();
// 抽象类:有抽象方法,也是已实现的方法// 接口全是抽象方法// 共同之处:统统不能实例化,原因是内部有抽象方法//1.创建类interface iDemo{ //接口方法 public function getInfo(); public function hello();}abstract class AbsClass{ // 抽象方法 abstract public function getInfo(); // 已实现方法 public function hello(){ return 'Hello everyone.'; }}//工作类class Demo8 extends AbsClass { public $name; protected $occupation; public function getInfo(){ return 'My name is ' . $this->name . ' and occupation is ' . $this->occupation.'<br>'; } public function hello(){ return 'Hello everyone.'; } public function __construct($name,$occupation) { $this->name = $name; $this->occupation = $occupation; }}$obj = new Demo8('Ricky','Programmer');echo $obj->getInfo();echo $obj->hello();
1.抽象类与接口,都是定义执行方法,具体实现代码写在工作类中,扩展抽象类使用extends
,扩展接口使用implements
,通常使用抽象类来实现接口,用工作类实现抽象类,抽象类一旦被继承,其中的所有抽象方法必须在子类中全部实现。
2.Trait主要解决了继承代码复用的问题,比如多个子类要继承多个父类的方法,就可以使用Trait把方法写入,在子类中使用use Trait
来调用该方法。