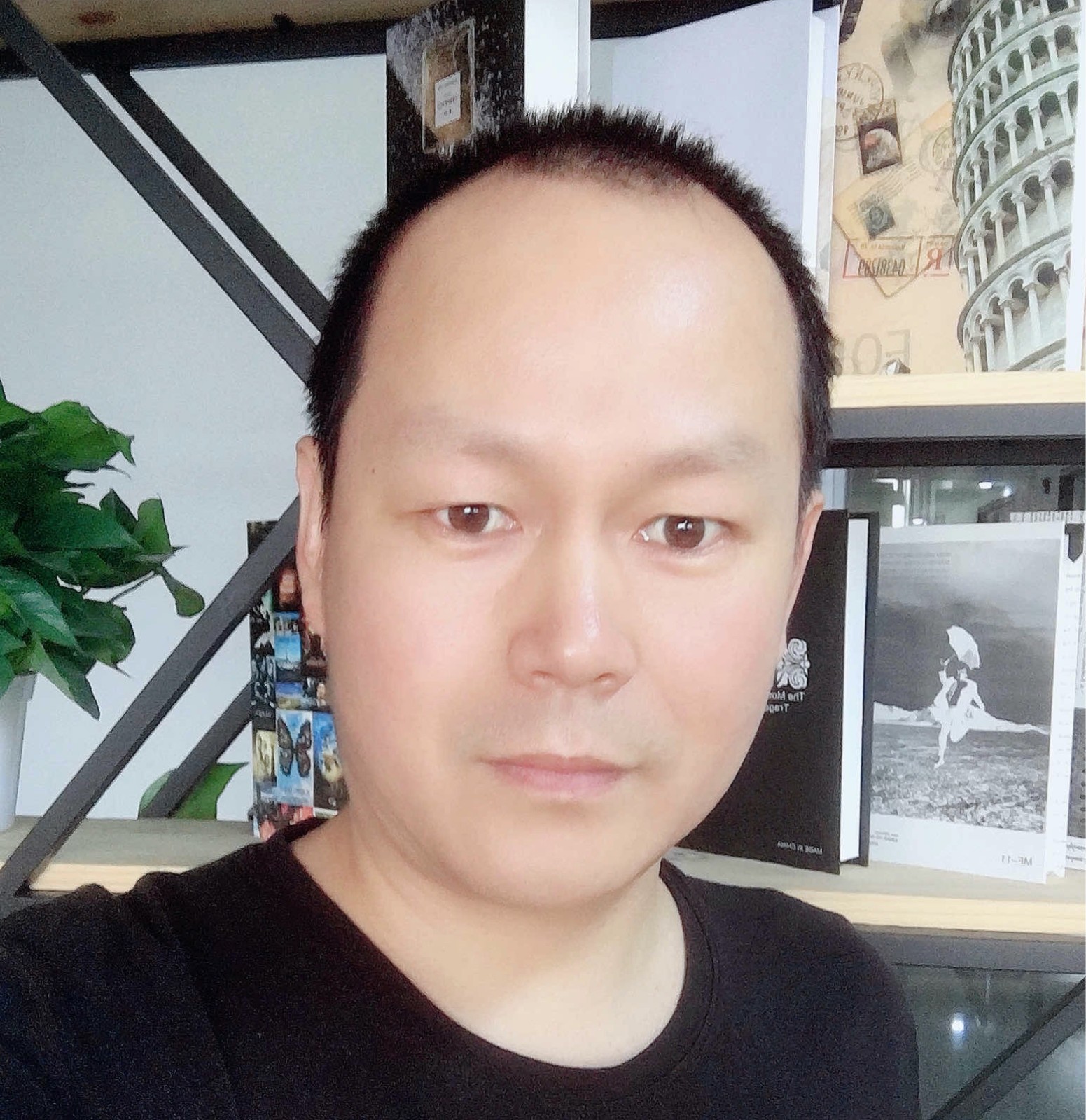
Correction status:qualified
Teacher's comments:作业干净整齐, 在面向如此灾难(原作业被清空)之下, 仍能保持一颗平和的心态认真学习.不错的.... 之前的作业, 可以抽空再写上一遍,这是老天逼你成功, 逼你学习. 作业没有其实是好事, 不是吗?
MVC 模式:Model-View-Controller(模型-视图-控制器)。
class Model
{
public function getData()
{
// 用二维数组来模拟从表中获取到的商品数据
return [
['id'=>1, 'name'=>'苹果电脑', 'model'=>'MacBook Pro', 'price'=>25800],
['id'=>2, 'name'=>'华为手机','model'=>'P30 Pro','price'=>4988],
['id'=>3, 'name'=>'小爱同学','model'=>'AI音箱','price'=>299],
];
}
}
class View
{
public function fetch($data)
{
$table = '';
$table .= '<table>';
$table .= '<caption>商品信息表</caption>';
$table .= '<tr><th>ID</th><th>品名</th><th>型号</th><th>价格</th></tr>';
foreach ($data as $item){
$table .= '<tr>';
$table .= "<td>{$item['id']}</td>";
$table .= "<td>{$item['name']}</td>";
$table .= "<td>{$item['model']}</td>";
$table .= "<td>{$item['price']}</td>";
$table .= '</tr>';
}
$table .= '</table>';
return $table;
}
}
echo '<style>
table {border-collapse: collapse; border: 1px solid; width: 500px;height: 150px}
caption {font-size: 1.2rem; margin-bottom: 10px;}
tr:first-of-type { background-color:lightblue;}
td,th {border: 1px solid}
td:first-of-type {text-align: center}
</style>';
require 'Model.php';
require 'View.php';
// 1、服务容器
class Container
{
//装载对象方法的容器
protected $instance = [];
/**
* 将类的实例化过程绑定到容器中
* @param $alias:类实例的别名
* @param Closure $process 闭包类/匿名函数
*/
public function bind($alias, Closure $process)
{
$this->instance[$alias] = $process;
}
/**
* @param $alias 类实例别名
* @param array $params 数组参数
* @return mixed 实例/对象
*/
public function make($alias, $params = [])
{
return call_user_func_array($this->instance[$alias], []);
}
}
//实例化容器
$container = new Container();
//将模型对象、视图对象保存到容器中
$container->bind('model', function () {
return new Model();
});
$container->bind('view', function () {
return new View();
});
// 2、添加Facade门面类, 规范/统一对外部对象的调用方式(静态)
class Facade
{
protected static $container;
protected static $data = [];
/**
* @param Container $container 服务容器
*/
public static function initialize(Container $container)
{
static::$container = $container;
}
// 将模型中的 getData() 方法静态化
public static function getData()
{
static::$data = static::$container->make('model')->getData();
}
// 将模型中的 fetch() 方法静态化
public static function fetch()
{
return static::$container->make('view')->fetch(static::$data);
}
}
// 3、创建控制器
class Controller
{
public function __construct(Container $container)
{
// 调用 Facade里面的初始化方法
Facade::initialize($container);
}
public function index()
{
// 获取数据
Facade::getData();
// 渲染模板
return Facade::fetch();
}
}
//客户端调用
$controller = new Controller($container);
echo $controller->index();
代码效果:
手写:
总结:
1、类的静态成员:使用static
声明,内部访问使用self::属性名/方法名
,类外访问使用类名::属性名/方法名
。
2、静态延迟绑定:使用static::属性名/方法名
绑定,普通成员属性/方法在编译时被调用,静态成员属性/方法在运行时被调用。最终谁调用它,它就属于哪个类的成员。
3、mvc模式:使用mvc模式编程,极大的提高开发效率,降低耦合度。M
代表模型,V
代表视图,C
代表控制器。
控制器通过使用容器(Container)
分别将Model
、View
的实例放入到Container
中,再使用Facade
类将Model
中的getData()
和View
中的fetch()
方法静态化,然后在Controller控制器中分别使用Facade::getData()
和Facade::fetch()
完成数据获取和模板的渲染。
最后客户端实例化控制器并调用index()
方法。
THE END !