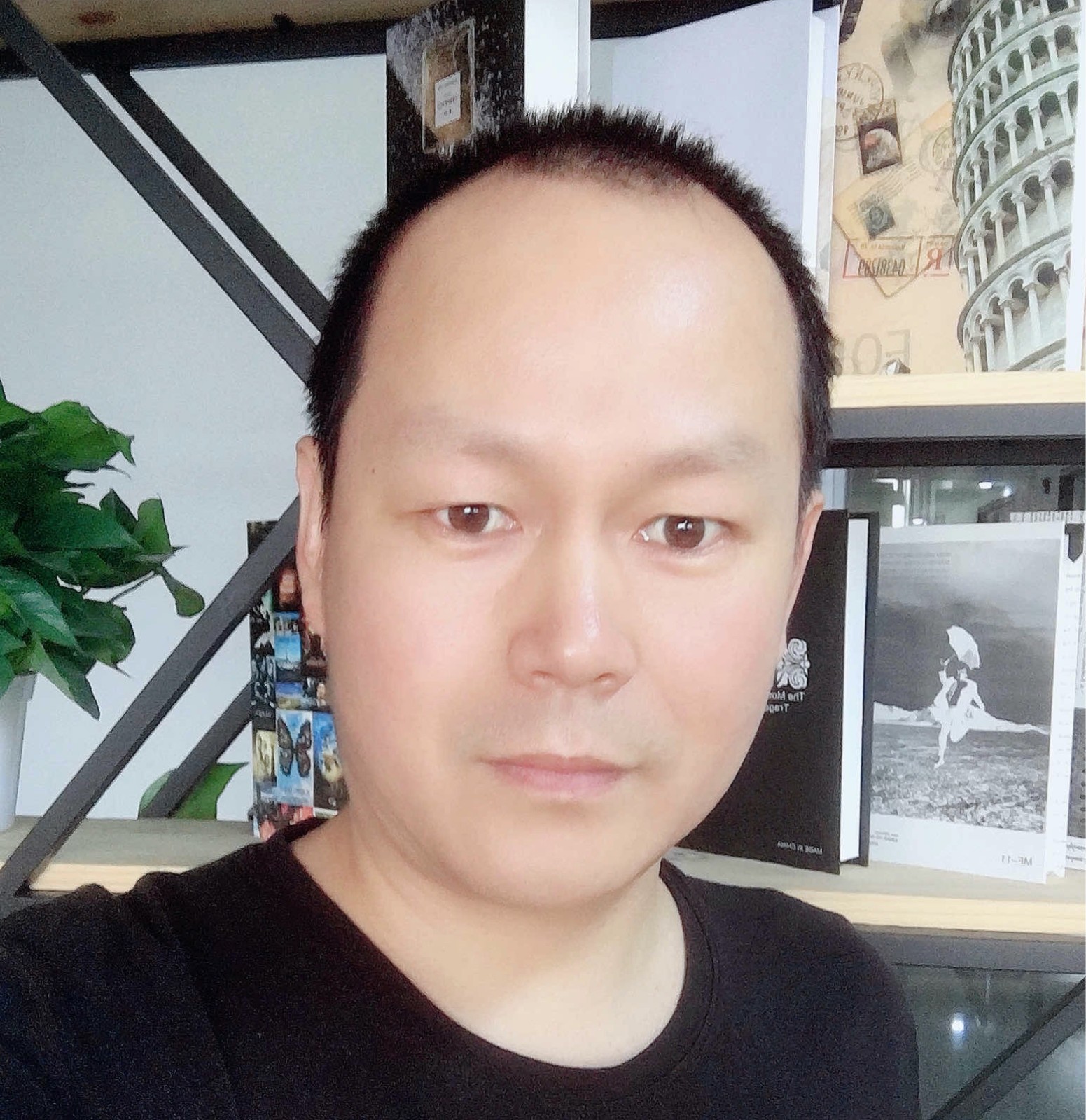
Correction status:qualified
Teacher's comments:写代码之前, 可以画个业务草图
<?php
namespace mvc;
use PDO;
class Model{
public function __construct($a='mysql:host=localhost;dbname=film', $b='root', $c='root', $table='user')
{
$this->pdo = new PDO($a,$b,$c);
$this->table = $table;
}
public function select(){
$sql = "SELECT id,name,tel FROM {$this->table}";
$stmt = $this->pdo->prepare($sql);
$stmt->execute();
return $stmt->fetchAll(PDO::FETCH_ASSOC);
}
}
<?php
namespace mvc;
class View{
public function fetch($data){
$table = '<table>';
$table .= '<caption>商品列表</caption>';
$table .= '<tr><th>ID</th><th>品名</th><th>型号</th></tr>';
foreach($data as $v){
$table .= '<tr>';
$table .= '<td>' . $v['id'] . '</td>';
$table .= '<td>' . $v['name'] . '</td>';
$table .= '<td>' . $v['tel'] . '</td>';
$table .= '</tr>';
}
$table .= '</table>';
return $table;
}
}
echo '<style>
table {border-collapse: collapse; border: 1px solid; width: 500px;height: 170px}
caption {font-size: 1.2rem; margin-bottom: 10px;}
tr:first-of-type { background-color:lightblue;}
td,th {border: 1px solid}
td{text-align: center}
</style>';
<?php
namespace mvc;
use Closure;
require __DIR__ . '/model.php';
require __DIR__ . '/view.php';
//添加服务容器层
class Container{
//容器属性时一个空数组,里面装创建对象的方法
protected $contt = [];
// 放进去,把实例化过程绑定到容器中
// $alias 类实类的别名 可以自定义;Closure用于代表 匿名函数 的类
public function bind($alias, Closure $process){
//将类实例化的方法 存储到容器中
$this->contt[$alias] = $process;
}
//取出来,执行容器中的实例方法
public function make($alias, $arr = []){
//call_user_func_array 回调函数,第一个是回调函数,第二个时索引数组
//如call_user_func_array($a, $b) 把($a)作为回调函数调用,把参数数组作($b)为回调函数的参数传入。
return call_user_func_array($this->contt[$alias], $arr);
}
}
$container = new Container();
//把model的实例化过程,绑定到容器中
$container->bind('model', function (){return new Model();});
//把view的实例化过程,绑定到容器中
$container->bind('view', function (){return new View();});
//添加facade门面类
class facade{
protected static $container;
protected static $data = [];
// 导入服务器容器,并静态化
public static function initialize(Container $container){
static::$container = $container;
}
// 导入model,并静态化
public static function getData(){
static::$data = static::$container->make('model')->select();
}
// 导出view,并静态化
public static function fetch(){
return static::$container->make('view')->fetch(static::$data);
}
}
//控制器
class demo{
public function __construct(Container $container)
{
facade::initialize($container);
}
public function getOut(){
//获取数据
facade::getData();
//渲染模板
return facade::fetch();
}
}
//客户端
$demo = new demo($container);
echo $demo->getOut();
mvc对我来说,很有难度,做了几遍,流程基本懂了,但还是手生,主要问题在容器层,facade门面。。本周末好好练习