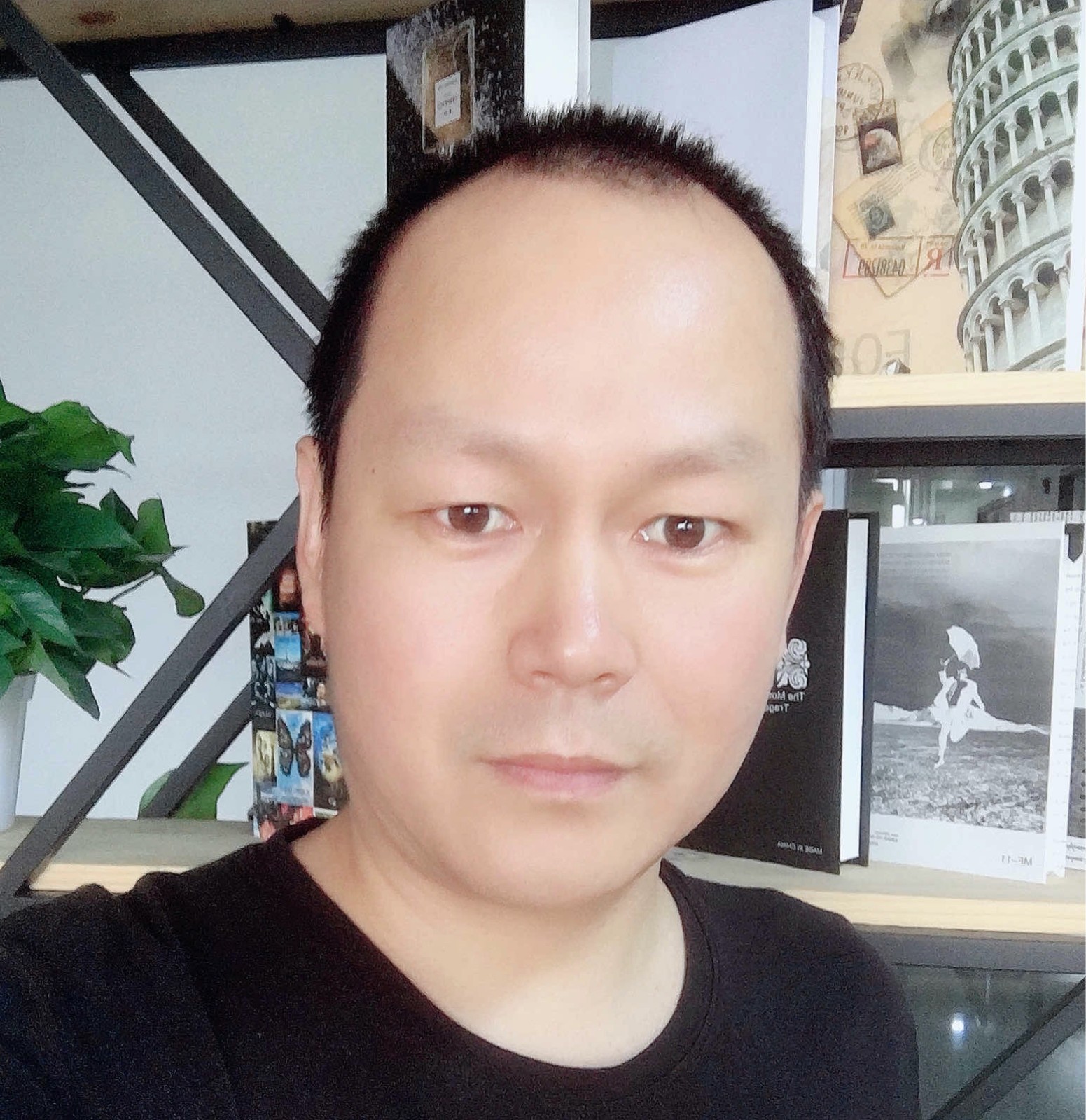
Correction status:qualified
Teacher's comments:有没有想过, 给这张表添加上编辑, 删除, 添加功能, 让它看上去更像是一个员工管理系统
controller/StaffsController.php
<?php
// 控制器
class StaffsController
{
//获取全部数据
public function listAll()
{
//实例化模型,获取数据
$sta = new StaffsModel();
$data = $sta->getAll();
require 'view/StaffslistAll.php';
}
//获取单条数据
public function info($id=1)
{
$id = isset($_GET['id']) ? $_GET['id'] : $id;
$sta = new StaffsModel();
$data = $sta->get($id);
require 'view/Staffslistinfo.php';
}
}
model/db.php
<?php
//数据库的基本操作
class Db
{
//数据库的基本参数
private $dbConfig=[
'type' => 'mysql', //数据库类型
'host' => 'localhost', //服务器地址
'dbname' => 'tj_ys', //数据库名称
'username' => 'root', //用户名
'password' => '123123' //密码
];
//单例模式,保存本类实例
private static $instance = null;
//保存数据库连接实例
private static $conn = null;
//新增ID
public $insertID = null;
//受影响的数量
public $num = 0;
//构造方法私有化,
private function __construct($params)
{
//初始化连接参数
$this->dbConfig = array_merge($this->dbConfig,$params);
//连接数据库
$this->connect();
}
//克隆方法私有化,
private function __clone()
{
}
//获取当前类的单一实例
public static function getInstance($params=[])
{
if(!self::$instance instanceof self){
self::$instance=new self($params);
}
return self::$instance;
}
//数据库连接
private function connect()
{
try {
//连接参数
$dsn = "{$this->dbConfig['type']}:host={$this->dbConfig['host']};dbname={$this->dbConfig['dbname']}";
//数据库连接
$this->conn = new PDO($dsn, $this->dbConfig['username'], $this->dbConfig['password']);
} catch (PDOException $e) {
die('连接失败' . $e->getMessage());
}
}
//数据表的写操作,新增、更新、删除
//返回受影响的记录数,如果是新增返回新增ID
public function exec($sql)
{
$num = $this->conn->exec($sql);
if ($num > 0){
// 如果是新增返回新增ID
if ($this->conn->lastInsertId() !== null){
$insertID = $this->conn->lastInsertId();
}
$this->num = $num; //返回受影响的数量
}else{
$error = $htis->conn->errorInfo(); //获取最后操作的错误信息的数组
//[0]:错误标识符;[1]:错误代码;[2]:错误信息
print '操作失败' . $error[0] . ': ' . $error[1] . ': ' . $error[2];
}
}
//查询操作:获取单条数据
public function fetch($sql)
{
return $this->conn->query($sql)->fetch(PDO::FETCH_ASSOC);
}
//查询操作:获取多条数据
public function fetchAll($sql)
{
return $this->conn->query($sql)->fetchAll(PDO::FETCH_ASSOC);
}
}
model/Model.php
<?php
//公共模型
class Model
{
protected $db = null; //数据库连接对象
public $data = null; //当前数据内容
public function __construct()
{
$this->init(); //完成数据连接
}
private function init()
{
$dbConfig=[
'dbname' => 'tj_ys', //数据库名称
'username' => 'root', //用户名
'password' => '123123'
];
$this->db = Db::getInstance($dbConfig);
}
//获取全部数据
public function getAll()
{
$sql="SELECT * FROM staffs";
return $this->data = $this->db->fetchAll($sql);
}
//获取全部数据
public function get($id)
{
$sql="SELECT * FROM staffs WHERE id = {$id}";
return $this->data = $this->db->fetch($sql);
}
}
model/StaffsModel.php
<?php
// 用户自定义模型
class StaffsModel extends Model
{
}
view/StaffslistAll.php
<!DOCTYPE html>
<html lang="zh">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<meta http-equiv="X-UA-Compatible" content="ie=edge">
<title></title>
<style>
table {border-collapse: collapse; border: 1px solid; width: 500px;height: 150px}
caption {font-size: 1.2rem; margin-bottom: 10px;}
tr:first-of-type { background-color:lightblue;}
td,th {border: 1px solid}
td:first-of-type {text-align: center}
</style>
</head>
<body>
<table>
<caption><h2>人物信息表</h2></caption>
<tr>
<th>ID</th>
<th>姓名</th>
<th>职位</th>
<th>操作</th>
</tr>
<?php foreach($data as $data_sta): ?>
<tr>
<td><?php echo $data_sta['id']; ?></td>
<td><?php echo $data_sta['name']; ?></td>
<td><?php echo $data_sta['position']; ?></td>
<td><a href="index.php?a=info&id=<?php echo $data_sta['id']; ?>">查看详情</a></td>
</tr>
<?php endforeach; ?>
</table>
总计:<?php echo count($data); ?>条记录
</body>
</html>
view/Staffslistinfo.php
<!DOCTYPE html>
<html lang="zh">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<meta http-equiv="X-UA-Compatible" content="ie=edge">
<title></title>
<style>
table {border-collapse: collapse; border: 1px solid; width: 500px;height: 150px}
caption {font-size: 1.2rem; margin-bottom: 10px;}
td,th {border: 1px solid}
td:first-of-type {text-align: center}
</style>
</head>
<body>
<table>
<caption><h2><?php echo $data['name']; ?>个人信息表</h2></caption>
<tr>
<th>ID</th>
<th><?php echo $data['id']; ?></th>
</tr>
<tr>
<th>姓名</th>
<th><?php echo $data['name']; ?></th>
</tr>
<tr>
<th>年龄</th>
<th><?php echo $data['age']; ?></th>
</tr>
<tr>
<th>性别</th>
<th><?php echo $data['sex']; ?></th>
</tr>
<tr>
<th>职位</th>
<th><?php echo $data['position']; ?></th>
</tr>
</table>
<a href="index.php?a=listAll">显示全部信息</a>
</body>
</html>
index.php
<?php
//入口文件
//加载模型类
require 'model/db.php';
require 'model/Model.php';
require 'model/StaffsModel.php';
//获取控制器名称
$controller = isset($_GET['c']) ? $_GET['c'] : 'Staffs';
//控制器加后缀
$controller .='Controller';
//加载控制器类
require 'controller/' . $controller . '.php';
// 获取方法
$action = isset($_GET['a']) ? $_GET['a'] : 'listAll';
$Sta = new $controller();
$Sta->$action();