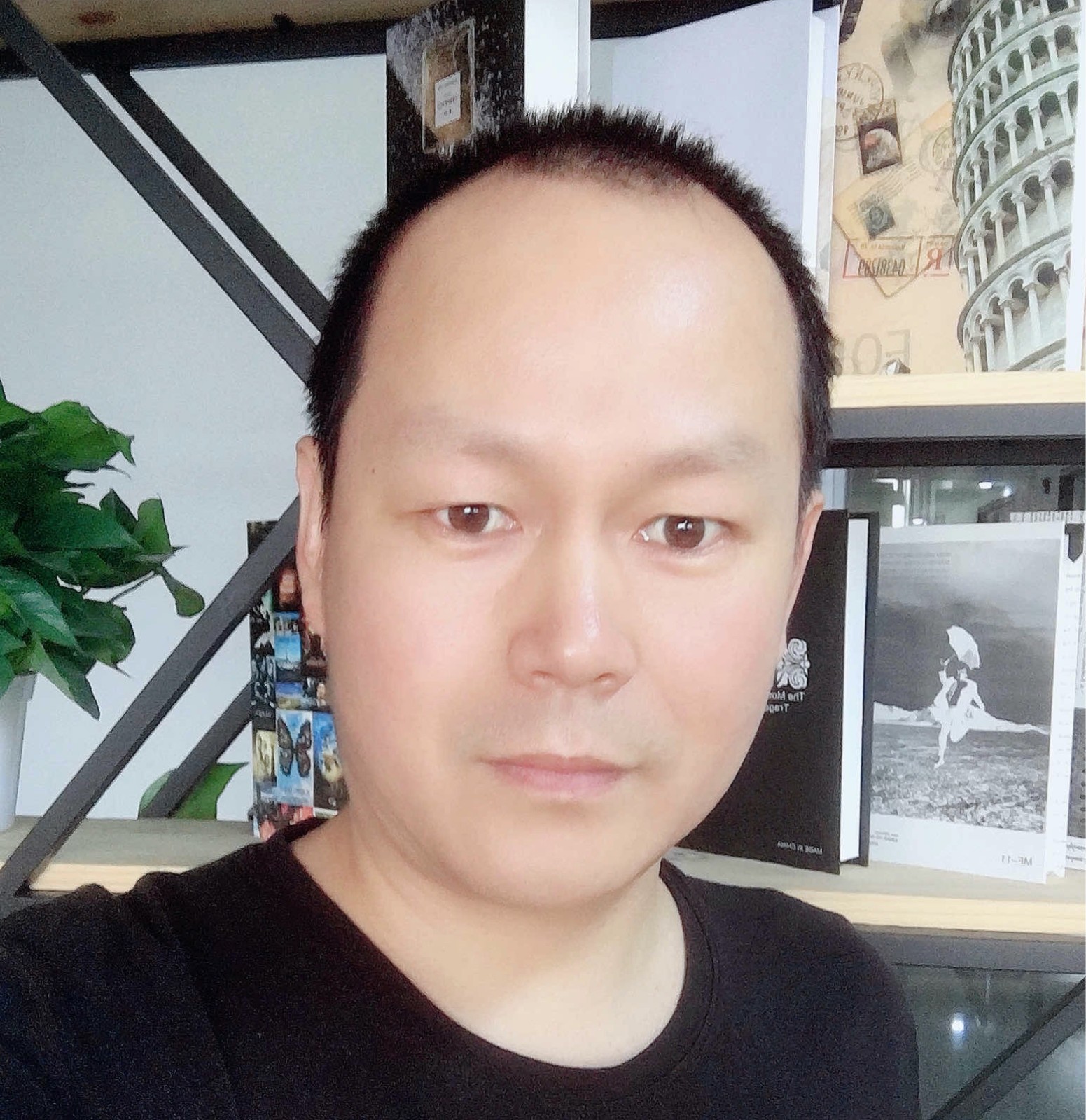
Correction status:qualified
Teacher's comments:这个作业 ,需要用到这么多的技术知识点吗? 你是不是搞得太复杂了
<?php
namespace config;
use PDO;
class Model{
//pdo
private static $pdo;
//数据表
private static $tabel;
public function __construct($dsn='mysql:host=localhost;dbname=film',$user='root',$password='root')
{
static::$pdo = new PDO($dsn,$user,$password);
}
//获取栏目表数据
private static function list($table='listinfo')
{
static::$tabel = $table;
$stmt = static::$pdo->prepare('SELECT * FROM ' . static::$tabel);
$stmt -> execute();
return $stmt -> fetchAll(PDO::FETCH_ASSOC);
}
//获取新闻内容页数据表数据
private static function article($table='article')
{
static::$tabel = $table;
$stmt = static::$pdo->prepare('SELECT * FROM ' . static::$tabel);
$stmt -> execute();
return $stmt -> fetchAll(PDO::FETCH_ASSOC);
}
//获取商品内容页表数据
private static function shop($table='shop')
{
static::$tabel = $table;
$stmt = static::$pdo->prepare('SELECT * FROM ' . static::$tabel);
$stmt -> execute();
return $stmt -> fetchAll(PDO::FETCH_ASSOC);
}
//所有数据整合到一个类方法中,方便mvc调用
public function getDate()
{
return [
'list' => static::list(),
'article' => static::article(),
'shop' => static::shop()
];
}
}
<?php
use \view\index;
use \config\Model;
use \view\article;
use \view\footer;
use \view\header;
use \view\list_article;
use \view\list_shop;
use \view\shop;
require __DIR__ . '/Autoload.php';
//容器类
class Container
{
//闭包数组
private static $binds = [];
//属性数组
private static $instance = [];
//依赖类或属性绑定到容器
public function bind($abstract,$concrete)
{
if($concrete instanceof Closure){
static::$binds[$abstract] = $concrete;
}else{
static::$instance[$abstract] = $concrete;
}
}
//将需要调用的依赖对象放出去
public function make($abstract, $parameters = [])
{
if(isset(static::$instance[$abstract])){
return static::$instance[$abstract];
}else{
return call_user_func_array(static::$binds[$abstract], $parameters);
}
}
}
//容器类实例化
$container = new Container();
//将数据库文件绑定到容器中
$container->bind('model', function(){return new Model();});
//将依赖的模板类绑定到容器中
$container->bind('header', function(){return new header();});
$container->bind('footer', function(){return new footer();});
$container->bind('index', function(){return new index();});
$container->bind('list_article', function(){return new list_article();});
$container->bind('list_shop', function(){return new list_shop();});
$container->bind('article', function(){return new article();});
$container->bind('shop', function(){return new shop();});
//Facade门面
class Facade
{
//设置容器数组
private static $container;
//设置数据库数组
private static $data;
//导入容器类
public static function insert(Container $container)
{
static::$container = $container;
}
//导入数据库
public static function data()
{
static::$data = static::$container->make('model')->getDate();
}
//首页视图模板静态化
public static function index()
{
return static::$container->make('index')->index(static::$data);
}
//header模板静态化
public static function header()
{
return static::$container->make('header')->header();
}
//footer模板静态化
public static function footer()
{
return static::$container->make('footer')->footer();
}
//list_article
public static function list_article()
{
return static::$container->make('list_article')->list_article(static::$data);
}
//article
public static function article()
{
return static::$container->make('article')->article(static::$data);
}
//list_shop
public static function list_shop()
{
return static::$container->make('list_shop')->list_shop(static::$data);
}
//shop
public static function shop()
{
return static::$container->make('shop')->shop(static::$data);
}
}
//控制器
class Controller
{
//构造方法,导入容器
public function __construct(Container $container)
{
Facade::insert($container);
}
public function index()
{
//导入数据库
Facade::data();
//渲染首页模板
Facade::header();
Facade::index();
Facade::footer();
}
public function list_article()
{
//导入数据库
Facade::data();
//渲染新闻列表模板
Facade::header();
Facade::list_article();
Facade::footer();
}
public function list_shop()
{
//导入数据库
Facade::data();
//渲染商品列表模板
Facade::header();
Facade::list_shop();
Facade::footer();
}
public function article()
{
//导入数据库
Facade::data();
//渲染新闻内容模板
Facade::header();
Facade::article();
Facade::footer();
}
public function shop()
{
//导入数据库
Facade::data();
//渲染商品内容模板
Facade::header();
Facade::shop();
Facade::footer();
}
}
//控制器实例化
$controller = new Controller($container);
<?php
spl_autoload_register(function (string $class) {
$file = str_replace('\\', '/', $class) . '.php';
if (!is_file($file)) {
throw new \Exception("file don't exists");
}
require $file;
});
//header.php
<?php
namespace view;
?>
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>首页</title>
<!-- <link rel="stylesheet" href="static/css/reset.css">-->
<link rel="stylesheet" href="static/font/iconfont.css">
<link rel="stylesheet" href="static/css/index.css">
</head>
<body>
<!--公共顶部导航区-->
<header>
<a href="/">网站首页</a>
<a href="/">专题</a>
<a href="/">网站导航</a>
<a href="/">二手商品</a>
<a href="/">讨论区</a>
<span>
<?php
if(isset($_COOKIE['name'])){
echo "<a><i class='iconfont icon-huiyuan2'></i>{$_COOKIE['name']}</a> <a href='dispatch.php?action=logout'>退出</a>";
}else{
echo '<a href="dispatch.php?action=login"><i class="iconfont icon-huiyuan2"></i>登陆</a><a href="dispatch.php?action=register">免费注册</a>';
}
?>
</span>
</header>
<?php
class header
{
public function header()
{
}
}
?>
*************************
*************************
//footer.php
<?php
namespace view;
class footer
{
public function footer()
{
}
}
?>
<!-- 页底部-->
<footer>
<div>
<a href="">简介</a>
<a href="">联系我们</a>
<a href="">招聘信息</a>
<a href="">友情链接</a>
<a href="">用户服务协议</a>
<a href="">隐私权声明</a>
<a href="">法律投诉声明</a>
</div>
<div><span>LOGO</span></div>
<div>
<p>2019 fengniao.com. All rights reserved . 安徽闹着玩有限公司(无聊网)版权所有</p>
<p>皖ICP证150110号 京ICP备14323013号-2 皖公网安备110108024357788号</p>
<p>违法和不良信息举报电话: 0551-1234567 举报邮箱: admin@baidu.com</p>
</div>
<div>
<p>关注公众号</p>
<img src="static/images/erwei-code.png" alt="">
</div>
</footer>
</body>
</html>
*************************
*************************
//index.php
<?php
namespace view;
?>
<!--logo+搜索框+快捷入口区-->
<?php
class index
{
public function index($data)
{
echo '
<link rel="stylesheet" href="static/css/base.css">
<main>
<section class="one w1220">
<a href=""><img src="img/logo.png" alt=""></a>
<div class="search">
<input type="text" value="">
<i class="icon icon-jinduchaxun"></i>
</div>
<div class="one-r">
<a href=""><i class="icon icon-huiyuan1"></i></a>
<a href=""><i class="icon icon-danmu1"></i></a>
<a href=""><i class="icon icon-duoxuankuang1"></i></a>
<a href=""><i class="icon icon-jishufuwu"></i></a>
<a href=""><i class="icon icon-peiwangyindao"></i></a>
<a href=""><i class="icon icon-wenjianjia"></i></a>
<a href=""><i class="icon icon-huiyuan1"></i></a>
</div>
</section>
<nav class="w1220">
<ul>
<span>
<i class="icon icon-renwujincheng"></i>
<a>资讯看学</a>
</span>
<li>器材</li>
<li>大师</li>
<li>学院</li>
<li>影赛</li>
<li>器材</li>
<li>大师</li>
<li>学院</li>
<li>影赛</li>
</ul>
<ul>
<span>
<i class="icon icon-renwujincheng"></i>
<a>资讯看学</a>
</span>
<li>器材</li>
<li>大师</li>
<li>学院</li>
<li>影赛</li>
</ul>
<ul>
<span>
<i class="icon icon-renwujincheng"></i>
<a>资讯看学</a>
</span>
<li>器材</li>
<li>大师</li>
<li>学院</li>
<li>影赛</li>
</ul>
<ul>
<span>
<i class="icon icon-renwujincheng"></i>
<a>资讯看学</a>
</span>
<li>器材</li>
<li>大师</li>
<li>学院</li>
<li>影赛</li>
<li>器材</li>
<li>大师</li>
<li>学院</li>
<li>影赛</li>
<li>器材</li>
<li>大师</li>
</ul>
</nav>
<section class="banner w1220">
<div><img src="/img/2.jpg" alt=""></div>
<div><img src="/img/banner-right.jpg" alt=""></div>
</section>
<article class="news w1220">
<section>
<h1>新闻资讯</h1>
<a href="">更多</a>
</section>
<article>
<aside>';
$route = array_slice($data['article'], 0, 1);
foreach ($route as $value) {
echo "<a href=/article.php?list={$value['list']}&id={$value['id']}><img src={$value['img']} width=380 height=160></a>";
}
$route = array_slice($data['article'], 10, 2);
foreach ($route as $value) {
echo "<a href=/article.php?list={$value['list']}&id={$value['id']}><img src={$value['img']} width=180 height=120><span>{$value['title']}</span></a>";
}
echo '
</aside>
<article>';
$route = array_slice($data['article'], 7, 1);
foreach ($route as $value) {
echo "<h3><a href=/article.php?list={$value['list']}&id={$value['id']}>{$value['title']}</a></h3>";
}
echo '
<ul>';
$route = array_slice($data['article'], 4, 8);
foreach ($route as $value) {
echo "<li><span>[新闻]</span><a href=/article.php?list={$value['list']}&id={$value['id']}>{$value['title']}</a></li>";
}
echo '
</ul>
</article>
<article>';
$route = array_slice($data['article'], 10, 1);
foreach ($route as $value) {
echo "<h3><a href=/article.php?list={$value['list']}&id={$value['id']}>{$value['title']}</a></h3>";
}
echo '
<ul>';
$route = array_slice(array_reverse($data['article']), 0, 8);
foreach ($route as $value) {
echo "<li><span>[新闻]</span><a href=/article.php?list={$value['list']}&id={$value['id']}>{$value['title']}</a></li>";
}
echo '
</ul>
</article>
</article>
</article>
<section class="bg">
<article class="picture w1220">
<h2><span>图片专区</span></h2>
<dl>';
$route = array_slice($data['list'], 2, 1);
foreach ($route as $value) {
echo "<dt>{$value['title']}<span>纵观摄影艺术</span></dt>";
}
$route = array_slice(array_reverse($data['article']), 0, 4);
foreach ($route as $value) {
echo "<dd><a href=/article.php?list={$value['list']}&id={$value['id']}><img src={$value['img']}>{$value['title']}</a></dd>";
}
echo '
</dl>
<dl>';
$route = array_slice($data['list'], 1, 1);
foreach ($route as $value) {
echo "<dt>{$value['title']}<span>纵观摄影艺术</span></dt>";
}
$route = array_slice(array_reverse($data['article']), 4, 4);
foreach ($route as $value) {
echo "<dd><a href=/article.php?list={$value['list']}&id={$value['id']}><img src={$value['img']}>{$value['title']}</a></dd>";
}
echo '
</dl>
<dl>';
$route = array_slice($data['list'], 0, 1);
foreach ($route as $value) {
echo "<dt>{$value['title']}<span>纵观摄影艺术</span></dt>";
}
$route = array_slice(array_reverse($data['article']), 8, 4);
foreach ($route as $value) {
echo "<dd><a href=/article.php?list={$value['list']}&id={$value['id']}><img src={$value['img']}>{$value['title']}</a></dd>";
}
echo '
</dl>
</article>
</section>
</main>';
echo '
<!--二手交易专区-->
<div class="title">
<span>二手交易</span>
</div>
<div class="second-hand">
<div>
<a href="">抢好货</a>
<span>0低价, 便捷,安全,快速</span>
</div>
<div>
<span>热门分类</span>
<a href="">美女写真</a>
<a href="">日本美女</a>
<a href="">美国美女</a>
<a href="">国内美女</a>
<a href="">AV美女</a>
</div>';
$route = array_slice(array_reverse($data['shop']),0, 4);
foreach ($route as $value) {
echo "<a href=/article.php?list={$value['list']}&id={$value['id']}><img src={$value['img']} width=176 height=120></a>";
}
$route = array_slice(array_reverse($data['shop']), 0, 4);
foreach ($route as $value) {
echo "<div class='detail'>";
echo "<a href=/article.php?list={$value['list']}&id={$value['id']}>{$value['title']}</a>";
echo "<div>";
echo " <a href=/article.php?list={$value['list']}&id={$value['id']}>";
echo " <span>¥ {$value['price']}</span>";
echo " <span>美女</span>";
echo " </a>
</div></div>";
}
$route = array_slice($data['shop'],0, 4);
foreach ($route as $value) {
echo "<a href=/article.php?list={$value['list']}&id={$value['id']}><img src={$value['img']} width=176 height=120></a>";
}
$route = array_slice($data['shop'],0, 4);
foreach ($route as $value) {
echo "<div class='detail'>";
echo "<a href=/article.php?list={$value['list']}&id={$value['id']}>{$value['title']}</a>";
echo "<div>";
echo " <a href=/article.php?list={$value['list']}&id={$value['id']}>";
echo " <span>¥ {$value['price']}</span>";
echo " <span>美女</span>";
echo " </a>
</div></div>";
}
echo '
<div>
<a href=""><img src="static/images/ad/1.png" alt="" width="180" height="112"></a>
<a href=""><img src="static/images/ad/2.png" alt="" width="180" height="112"></a>
<a href=""><img src="static/images/ad/3.png" alt="" width="180" height="112"></a>
<a href=""><img src="static/images/ad/4.png" alt="" width="180" height="112"></a>
<a href=""><img src="static/images/ad/image.png" alt="" width="393" height="56"></a>
<a href=""><img src="static/images/ad/ad2.jpg" alt="" width="393" height="56"></a>
</div>
</div>
<!--合作网站-->
<div class="title" style="background:#fff">
<span>合作网站</span>
</div>
<div class="my-links">
<a href="https://www.php.cn">php中文网</a>
<a href="https://www.html.cn">html中文网</a>
<a href="https://www.py.cn">python中文网</a>
<a href="https://www.php.cn">php中文网</a>
<a href="https://www.html.cn">html中文网</a>
<a href="https://www.py.cn">python中文网</a>
<a href="https://www.php.cn">php中文网</a>
<a href="https://www.html.cn">html中文网</a>
<a href="https://www.py.cn">python中文网</a>
<a href="https://www.php.cn">php中文网</a>
<a href="https://www.html.cn">html中文网</a>
<a href="https://www.py.cn">python中文网</a>
<a href="https://www.py.cn">python中文网</a>
<a href="https://www.php.cn">php中文网</a>
<a href="https://www.html.cn">html中文网</a>
<a href="https://www.py.cn">python中文网</a>
<a href="https://www.php.cn">php中文网</a>
<a href="https://www.html.cn">html中文网</a>
<a href="https://www.py.cn">python中文网</a>
<a href="https://www.php.cn">php中文网</a>
<a href="https://www.html.cn">html中文网</a>
<a href="https://www.py.cn">python中文网</a>
<a href="https://www.php.cn">php中文网</a>
<a href="https://www.html.cn">html中文网</a>
<a href="https://www.py.cn">python中文网</a>
<a href="https://www.py.cn">python中文网</a>
</div>
';
}
}
?>
######################################
######################################
//list_article.php
<?php
namespace view;
class list_article
{
public function list_article($data)
{
echo '
<link rel="stylesheet" href="static/css/article-list.css">
<div class="main">
<div class="top">
<img src="static/images/ar-logo.png" alt="">';
foreach ($data['list'] as $value) {
if($_GET['id'] === $value['id']){
echo "<a style='font-size:24px;margin-left:10px;' href=list.php?id={$value['id']}>{$value['title']}</a>";
}
}
echo '
<label><input type="search"><span class="iconfont icon-sousuo2"></span></label>
</div>
<article>
<div>';
foreach ($data['list'] as $value) {
if($_GET['id'] === $value['id']){
echo "<a href=list.php?id={$value['id']} id=active>{$value['title']}</a>";
}
}
foreach ($data['list'] as $value) {
if(!($_GET['id'] === $value['id'])){
echo "<a href=list.php?id={$value['id']}>{$value['title']}</a>";
}
}
echo '
</div>';
foreach (array_reverse($data['article']) as $value) {
if(!($_GET['id'] === $value['id'])){
echo "<div class='list1'>";
echo "<a href=article.php?list={$value['list']}&id={$value['id']}><img src={$value['img']} width='272' height='200'></a>";
echo "<div>";
echo "<a href=article.php?list={$value['list']}&id={$value['id']}>{$value['title']}</a>";
echo "<span>{$value['title']}</span>";
echo "</div>";
foreach($data['list'] as $v){
if($v['id'] === $value['list']){
echo "<a href=list.php?id={$v['id']}>{$v['title']} · 46 分钟前</a>";
}
}
echo "<span><i class='iconfont icon-icon_yulan'></i>2233</span>";
echo "</div>";
}
}
echo '
</article>
<!-- 右侧列表-->
<div class="list1">
<h3>网页评论</h3>
<ul>';
foreach (array_reverse($data['article']) as $value) {
echo "<li><a href=article.php?list={$value['list']}&id={$value['id']}>{$value['title']}</a></li>";
}
echo '
</ul>
</div>
<div class="list2">
<h3>网页评论</h3>
<ul>';
foreach ($data['article'] as $value) {
echo "<li><a href=article.php?list={$value['list']}&id={$value['id']}>{$value['title']}</a></li>";
}
echo '
</ul>
</div>
<div class="recommend">
<h3>推荐阅读</h3>';
$route = array_slice($data['article'],0,4);
foreach ($route as $value) {
echo "<a href=article.php?list={$value['list']}&id={$value['id']}><img src='{$value['img']}' width='195' height='130'></a>";
}
$route = array_slice($data['article'],0,4);
foreach ($route as $value) {
echo "<a href=article.php?list={$value['list']}&id={$value['id']}>{$value['title']}</a>";
}
$route = array_slice($data['article'],4,4);
foreach ($route as $value) {
echo "<a href=article.php?list={$value['list']}&id={$value['id']}><img src='{$value['img']}' width='195' height='130'></a>";
}
$route = array_slice($data['article'],4,4);
foreach ($route as $value) {
echo "<a href=article.php?list={$value['list']}&id={$value['id']}>{$value['title']}</a>";
}
echo '
</div>
</div>
';
}
}
######################################
######################################
//shop.php
<?php
namespace view;
class shop
{
public function shop($data)
{
echo '
<link rel="stylesheet" href="static/css/shop-content.css">
<main>
<!--导航-->
<nav>
<h3>所有产品分类 <span class="iconfont icon-liebiao"></span></h3>
<a href="\" class="active">首页</a>
<a href="">3C</a>
<a href="">生活用品</a>
<a href="">名字名画</a>
</nav>
<!-- 商品展示-->
<div class="goods">';
foreach($data['list'] as $value){
if($value['id'] === $_GET['list']){
echo "<div class='top-nav'>
<a href=\>首页</a>->>
<a href=list.php?id={$value['id']}>{$value['title']}</a>->> ";
foreach($data['shop'] as $vv){
if($_GET['id'] == $vv['id']){
echo "{$vv['title']}";
}
}
echo "</div>";
}
}
foreach($data['shop'] as $value){
if($value['id'] === $_GET['id'] && $_GET['list'] == 2){
echo "<img src={$value['img']}>
<h2>{$value['title']}</h2>
<p>本站特惠: <span>¥{$value['price']}</span></p>";
}
}
echo '
<p>
销量: <span>13</span> |
累积评价: <span>3</span> |
好评率: <span>199%</span>
</p>
<p>
<label for="num">购买数量:</label><input type="number" id="num" value="1">
</p>
<div>
<button>立即购买</button>
<button><i class="iconfont icon-icon_tianjia"></i>加入购物车</button>
</div>
<div>
<span><i class="iconfont icon-zhanghaoquanxianguanli"></i>本站保障</span>
<span><i class="iconfont icon-icon_safety"></i>企业认证</span>
<span><i class="iconfont icon-tianshenpi"></i>退款承诺</span>
<span><i class="iconfont icon-kuaisubianpai"></i>免费换货</span>
</div>
</div>
<!-- 商品详情-->
<div class="detail">
<aside>
<div><span>商品详情</span><span>案例演示</span></div>';
foreach ($data['shop'] as $value) {
echo "<div><a href=article.php?list={$value['lis']}&id={$value['id']}><img src={$value['img']} width=170></a>
<a href=article.php?list={$value['lis']}&id={$value['id']}>{$value['title']}</a>
<div><span>热销:11</span><span>¥{$value['price']}</span></div></div>";
}
echo '
</aside>
<article>
<div class="nav">
<a href="">商品详情</a>
<a href="">案例/演示</a>
<a href="">常见问题</a>
<a href="">累计评价</a>
<a href="">产品咨询</a>
</div>
<div class="content">';
foreach ($data['shop'] as $value) {
if($_GET['id'] == $value['id']){
echo $value['newstext'];
}
}
echo '
</div>
<div class="comment">
<h3>网页评论</h3>
<img src="static/images/user.png" alt="" width="60">
<textarea name="" id="" cols="30" rows="10"></textarea>
<button>发表评论</button>
</div>
<!-- 最新评论-->
<div>
<h3>最新评论</h3>
<div>
<img src="static/images/user.png" alt="" width="60" height="60">
<span>用户昵称</span>
<span>留言内容</span>
<div>
<span>2019-12-12 15:34:23发表</span>
<span><i class="iconfont icon-dianzan"></i>回复</span>
</div>
</div>
<div>
<img src="static/images/user.png" alt="" width="60" height="60">
<span>用户昵称</span>
<span>留言内容</span>
<div>
<span>2019-12-12 15:34:23发表</span>
<span><i class="iconfont icon-dianzan"></i>回复</span>
</div>
</div>
<div>
<img src="static/images/user.png" alt="" width="60" height="60">
<span>用户昵称</span>
<span>留言内容</span>
<div>
<span>2019-12-12 15:34:23发表</span>
<span><i class="iconfont icon-dianzan"></i>回复</span>
</div>
</div>
<div>
<img src="static/images/user.png" alt="" width="60" height="60">
<span>用户昵称</span>
<span>留言内容</span>
<div>
<span>2019-12-12 15:34:23发表</span>
<span><i class="iconfont icon-dianzan"></i>回复</span>
</div>
</div>
</div>
</article>
</div>
</main>
';
}
}
######################################
######################################
//article.php
<?php
namespace view;
class article
{
public function article($data)
{
echo '
<link rel="stylesheet" href="static/css/article.css">
<div class="main">
<div class="top">
<img src="static/images/ar-logo.png" alt="">';
foreach($data['list'] as $value){
if($value['id'] === $_GET['list']){
echo "<a style='font-size:27px;margin-left:15px;color:red' href=list.php?id={$value['id']}>{$value['title']}</a>";
}
}
echo '
<label><input type="search"><span class="iconfont icon-sousuo2"></span></label>
</div>
<!-- 正文-->
<article>';
foreach($data['article'] as $value){
if($value['id'] === $_GET['id']){
echo "<h1>{$value['title']}</h1>";
}
}
echo '
<div>
<span>发布时间:2019.8.8</span>
<span>来源:北京 青年报</span>
<span>阅读量:545</span>
<span>评论数:1545</span>
</div>
<div>';
foreach($data['article'] as $value){
if($value['id'] === $_GET['id']){
if($_GET['list'] == 1){
echo "<img src={$value['img']}><br>";
echo "{$value['newstext']}";
}else{
echo "{$value['newstext']}";
}
}
}
echo '
</div>
</article>
<!-- 右侧列表-->
<div class="list1">
<h3>网页评论</h3>
<ul>';
foreach (array_reverse($data['article']) as $value) {
echo "<li><a href=article.php?list={$value['list']}&id={$value['id']}>{$value['title']}</a></li>";
}
echo '
</ul>
</div>
<div class="list2">
<h3>网页评论</h3>
<ul>';
foreach ($data['article'] as $value) {
echo "<li><a href=article.php?list={$value['list']}&id={$value['id']}>{$value['title']}</a></li>";
}
echo '
</ul>
</div>
<div class="recommend">
<h3>推荐阅读</h3>';
$route = array_slice($data['article'],0,4);
foreach ($route as $value) {
echo "<a href=article.php?list={$value['list']}&id={$value['id']}><img src='{$value['img']}' width='195' height='130'></a>";
}
$route = array_slice($data['article'],0,4);
foreach ($route as $value) {
echo "<a href=article.php?list={$value['list']}&id={$value['id']}>{$value['title']}</a>";
}
$route = array_slice($data['article'],4,4);
foreach ($route as $value) {
echo "<a href=article.php?list={$value['list']}&id={$value['id']}><img src='{$value['img']}' width='195' height='130'></a>";
}
$route = array_slice($data['article'],4,4);
foreach ($route as $value) {
echo "<a href=article.php?list={$value['list']}&id={$value['id']}>{$value['title']}</a>";
}
echo '
</div>
</div>
';
}
}
//index.php
<?php
require __DIR__ . '/Controller.php';
$controller->index();
######################################
######################################
//article.php
<?php
require __DIR__ . '/Controller.php';
if($_GET['list'] == 2){
$controller->shop();
}else{
$controller->article();
}
######################################
######################################
//list.php
<?php
require __DIR__ . '/Controller.php';
if($_GET['id'] == 2){
$controller->list_shop();
}else{
$controller->list_article();
}
//派发器dispatch.php
<?php
$pdo = new PDO('mysql:host=localhost;dbname=film', 'root', 'root');
$action = isset($_GET['action']) ? $_GET['action'] : 'login';
$action = htmlentities(strtolower(trim($action)));
switch ($action){
case 'login' : require __DIR__ . '/login/login.php';//登录
break;
case 'check' : require __DIR__ . '/login/check.php';//登录验证
break;
case 'register' : require __DIR__ . '/login/register.php'; //注册
break;
case 'checker' : require __DIR__ . '/login/checker.php'; //注册验证
break;
case 'logout' : require __DIR__ . '/login/logout.php';//退出登录
break;
default : die('<script>location.assign("index.php");</script>');
}
######################################
######################################
//登录页面 login.php
<?php
if(isset($_COOKIE['name'])){
echo '<script>alert("不要重复登录");location.assign("index.php");</script>';
}
?>
<!doctype html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>用户登录</title>
</head>
<body>
<h3>用户登录</h3>
<form action="dispatch.php?action=check" method="post" onsubmit="return isEmpty();">
<p>
<label for="tel">手机号:</label>
<input type="tel" name="tel" id="tel">
</p>
<p>
<label for="password">密码:</label>
<input type="password" name="password" id="password">
</p>
<p>
<button>提交</button>
</p>
</form>
</body>
</html>
######################################
######################################
//登录验证 check.php
<?php
//验证是否通过post提交登陆
if($_SERVER['REQUEST_METHOD'] === 'POST'){
//从数据库获取账户密码
$stmt = $pdo->prepare('SELECT * FROM `user` WHERE `tel` = :tel AND `password` = :password LIMIT 1');
//验证提交登陆的账户和密码是否再服务器中有对应的账户密码
$stmt->execute( ['tel'=>$_POST['tel'], 'password'=>sha1($_POST['password']) ]);
$user = $stmt->fetch(PDO::FETCH_ASSOC);
if($user === false || empty($_POST['tel'])){
die('<script>alert("账户或密码错误,重新登陆");history.back();</script>');
}else{
setcookie('name',$user['name'],time() + 60 * 60 * 72); //表示3天,登陆成功后,cookie 3天有效,无需登陆
die('<script>alert("登录成功");location.assign("index.php");</script>');
}
}else{
die('<script>alert("非法操作");location.assign("/index.php");</script>');
}
######################################
######################################
//退出 logout.php
<?php
if(isset($_COOKIE['name'])){
setcookie('name',null,time() - 3600);
die('<script>alert("退出成功,返回首页");location.assign("index.php");</script>');
}else{
die('<script>alert("请先登录");location.assign("dispatch.php?action=login");</script>');
}
######################################
######################################
//注册 register.php
<?php
if(isset($_COOKIE['name'])){
echo '<script>alert("不要重复登录");location.assign("index.php");</script>';
}
?>
<!doctype html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>用户注册</title>
</head>
<body>
<h3>用户注册</h3>
<form action="dispatch.php?action=checker" method="post" onsubmit="return isEmpty();">
<p>
<label for="tel">手机号:</label>
<input type="tel" name="tel" id="tel">
</p>
<p>
<label for="name">用户名:</label>
<input type="name" name="name" id="name">
</p>
<p>
<label for="password">密码:</label>
<input type="password" name="password" id="password">
</p>
<p>
<button>提交</button>
</p>
</form>
<script>
function isEmpty() {
var phone = document.getElementById('phone').value;
var password = document.getElementById('password').value;
if (phone.length=== 0 || password.length===0) {
alert('手机和密码不能为空');
return false;
}
}
</script>
</body>
</html>
######################################
######################################
//注册验证 checker.php
<?php
if($_SERVER['REQUEST_METHOD'] === 'POST'){
//注册,新增用户
$stmt = $pdo->prepare('INSERT INTO `user`(`name`, `tel`, `password`) VALUES(:name, :tel, :password) ');
if( empty($_POST['tel']) || empty($_POST['password']) ){
die('<script>alert("账户或密码为空,重新登陆");history.back();</script>');
}elseif($stmt->execute(['name'=>$_POST['name'], 'tel'=>$_POST['tel'], 'password'=>sha1($_POST['password']) ]) ){
if($stmt->rowCount() > 0){
setcookie('name',$_POST['name'],time()+60*60*72);
die('<script>alert("注册成功");location.assign("/index.php");</script>');
}
}
}else{
die('<script>alert("非法操作");location.assign("/index.php");</script>');
}
.w1220{
width: 1220px;
margin: 10px auto;
}
main{
width: 100%;
}
main>.one{
height: 90px;
margin-top: 20px;
display: flex;
justify-content: space-between;
align-items: center;
}
main>.one>.one-r{
display: flex;
width: 400px;
justify-content: space-between;
}
main>.one>.search{
position: relative;
}
main>.one>.search>input{
width: 280px;
height: 30px;
border-radius: 15px;
padding-left: 20px;
}
main>.one>.search>i{
font-size: 26px;
position: absolute;
right: 10px;
top:5px;
cursor: pointer;
}
main>.one>.one-r i{
font-size: 30px;
}
/*首页导航区*/
main>nav{
display: flex;
justify-content: space-between;
font-size: 14px;
height: 50px;
}
main>nav>ul{
display: grid;
/* grid-template-rows: repeat(2, 1fr);
grid-template-columns: repeat(5, 1fr); */
grid-template: repeat(2, 1fr)/repeat(5,1fr);
align-items: center;
justify-items: center;
}
main>nav>ul>span{
grid-area: span 2;
/* grid-row-end: span 2; */
display: flex;
margin-left: 18px;
margin-top: 5px;
border-right: solid 1px lightgray;
}
main>nav>ul>span>i:first-of-type{
font-size: 40px;
color: red;
margin-right: 3px;
display: block;
}
main>nav>ul>span>a{
width: 40px;
height: 35px;
display: inline-block;
margin-left: 5px;
color: black;
}
main>nav>ul:nth-of-type(2),main>nav>ul:nth-of-type(3){
grid-template-columns:repeat(3,1fr);
width: 380px;
}
main>nav>ul:last-of-type{
grid-template-columns:repeat(7,1fr);
}
/*banner*/
main>.banner{
display: flex;
justify-content: space-between;
margin: 30px auto;
}
/*首页新闻资讯*/
main>.news>section{
display: flex;
justify-content: space-between;
align-items: center;
border-bottom: 1px solid rgba(156, 156, 156, 0.3);
}
main>.news>section>h1{
font-size: 28px;
padding-bottom: 10px;
border-bottom: 3px solid red;
}
main>.news>article{
display: grid;
grid-template-columns: 380px 1fr 1fr;
}
main>.news>article>aside{
display: grid;
grid-template: repeat(2, 1fr)/repeat(2, 1fr);
}
main>.news>article>aside>a:first-of-type{
grid-column-end: span 2;
margin-top: 25px;
}
main>.news>article>aside>a{
margin-top: 15px;
}
main>.news>article>aside img{
width: 100%;
display: block;
}
main>.news>article>aside>a:nth-of-type(2){
margin-right: 5px;
}
main>.news>article>aside>a:last-of-type{
margin-left: 5px;
}
main>.news>article>aside>a:nth-of-type(2)>img,
main>.news>article>aside>a:last-of-type>img{
width: 100%;
height: 120px;
}
main>.news>article>article{
margin-left: 30px;
}
main>.news>article>article>h3{
height: 70px;
line-height: 70px;
text-align: center;
white-space:nowrap;
width:380px;
overflow:hidden;
text-overflow:ellipsis;
}
main>.news>article>article h3 a{
color: red;
font-size: 22px;
}
main>.news>article>article>ul>li{
margin-top: 13px;
}
main>.news>article>article>ul>li:first-of-type{
margin-top: 0;
}
main>.news>article>article>ul>li:nth-of-type(5){
margin-top: 30px;
}
main>.news>article>article>ul a{
color: black;
}
main>.news>article>article>ul a:hover{
color: red;
}
main>.news>article>article>ul span{
color: #666;
}
main>.news>article>article>ul>li span{
margin-right: 10px;
}
.bg{
width: 100%;
background-color: rgba(156, 156, 156, 0.2);
}
main>.bg>.picture{
padding: 35px 0;
display: grid;
grid-template: 70px 1fr/repeat(3,1fr);
}
main>.bg>.picture>h2{
text-align: center;
grid-column-end: span 3;
}
main>.bg>.picture>h2>span{
padding-bottom: 5px;
border-bottom: 3px solid rgba(253, 0, 0, 0.7);
}
main>.bg>.picture>dl{
background-color: rgba(255, 255, 255, .7);
margin-right: 20px;
padding: 15px 10px;
box-sizing: border-box;
display: grid;
grid-template: 40px 1fr 1fr/repeat(2,1fr);
}
main>.bg>.picture>dl>dt{
grid-column: span 2;
margin-left: 10px;
font-size: 22px;
font-weight: 600;
border-bottom: 1px solid rgba(156, 156, 156, 0.2);
}
main>.bg>.picture>dl>dt>span{
font-size: 14px;
font-weight: 0;
margin-left: 20px;
}
main>.bg>.picture>dl:last-of-type{
margin-right: 0;
}
main>.bg>.picture>dl>dd{
width: 160px;
margin-top: 20px;
align-self: center;
justify-self:center;
}
main>.bg>.picture>dl>dd img{
width: 160px;
height: 120px;
display: block;
}
1.首页部分自己手写了css,用的grid和flex混搭,复习了一下前端
2.复习了$_COOKIE 使用它完成了会员登录设置
3.全手写MVC流程,感觉基本掌握了
4.目前的问题就是:接口视图层,model数据库,控制反转到容器层后,不知道怎么传数据库到视图层