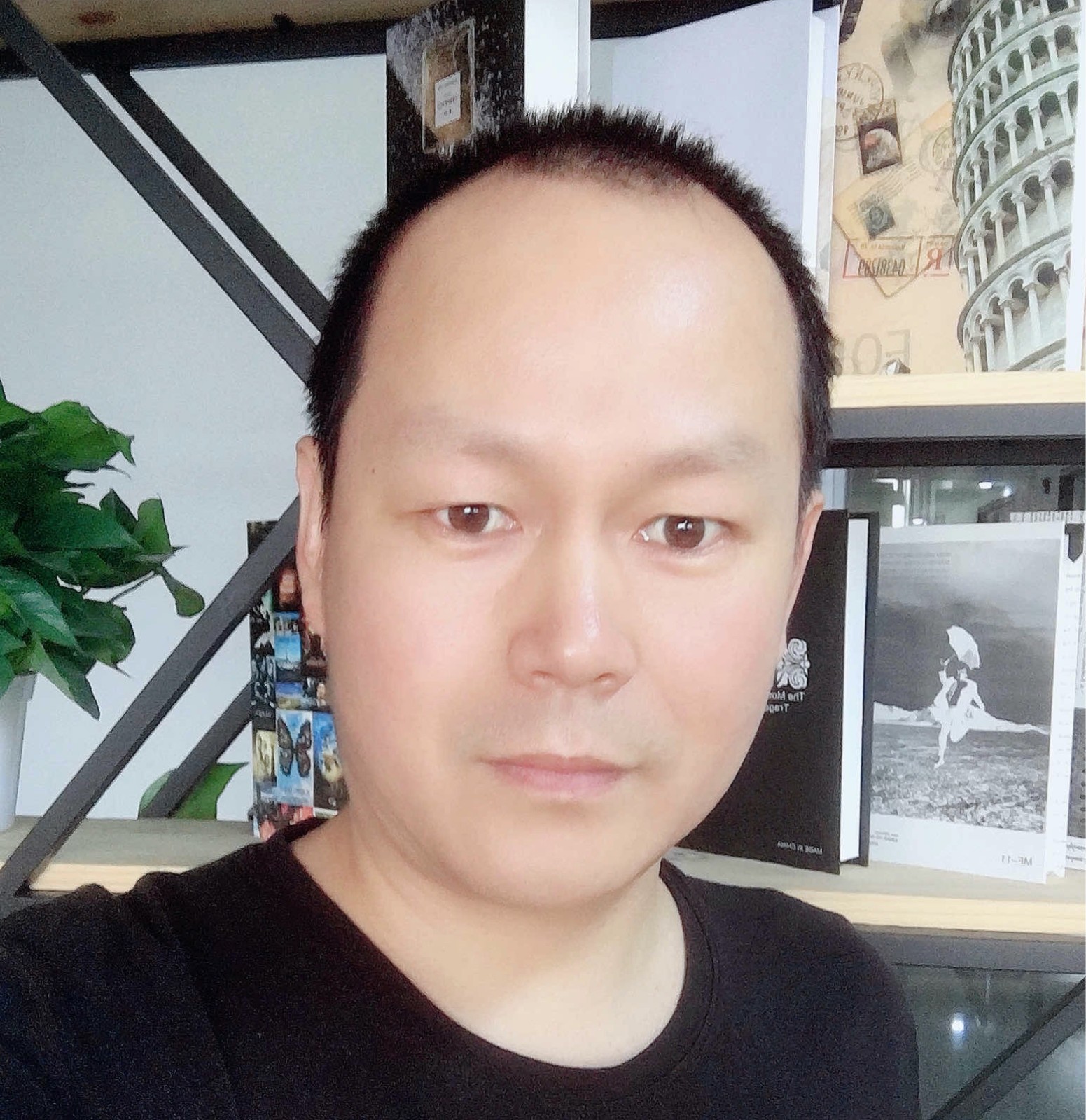
Correction status:qualified
Teacher's comments:觉得不难, 有二种可能, 一是你学得不错, 二是你可能被假象骗了... 多写多看, 不论做什么事都会有收获的, 加油吧
<?php
/** 变量配置 **/
define('DB_NAME', 'dedecmsv57');
define('DB_USER', 'root');
define('DB_PASSWORD', '123456');
define('DB_HOST', 'localhost');
//创建链接数据库的类和数据库操作
class Sql_db
{
public $pdo;
// 链接数据库
public function conect($host,$dbname,$user,$pass)
{
$dsn = "mysql:host=$host; dbname=$dbname;";
try{
$pdo=new PDO($dsn,$user,$pass);
}catch (PDOException $e){
die('数据库错误:'.$e->getMessage());
}
return $pdo;
}
}
<?php
// 连接数据库:$pdo
include 'connect.php';
//创建一个Sql_db的子类
class select extends Sql_db{
public $pdo;
public function __construct()
{
// 调用父类方法链接数据库
$this->pdo= parent::conect(DB_HOST,DB_NAME,DB_USER,DB_PASSWORD);
}
// 查询栏目信息和链接
public function selHead(){
$sql = 'select * from `coumn` ';
$stmt = $this->pdo->prepare($sql);
$stmt->execute();
$cates = $stmt->fetchAll(PDO::FETCH_ASSOC);
foreach ($cates as $cate){
echo ' <li><a href="'. $cate['url'].'.php'.'?id='.$cate['id'].'">'.$cate['cname'].'</a></li>';
}
}
// 首页换灯图片
public function selPic(){
$sql = 'select `titlename`,`imageurl` from `images` ';
$stmt = $this->pdo->prepare($sql);
$stmt->execute();
$cates = $stmt->fetchAll(PDO::FETCH_ASSOC);
// 图片路径
$imgpath='static/images/';
foreach ($cates as $cate){
if ($cate['imageurl']===$cate['titlename'])
{
echo '<div><img src= '.$imgpath.$cate['titlename'].'></div>';
}
}
}
// 查询首页h3红色标题图片
public function selTitle(){
$sql = 'select `*` from `article` limit 1 ';
$stmt = $this->pdo->prepare($sql);
$stmt->execute();
$article = $stmt->fetchAll(PDO::FETCH_ASSOC);
foreach ($article as $art)
{
echo '<h3><a href="article.php?id='.$art['id'].'" >'.$art['title'].'</a></h3>';
}
}
//首页新闻资讯
public function selNew($a){
// 变量$limit控制显示的条数
$limit='limit '.$a;
$sql = 'select `*` from `article` '. $limit;
$stmt = $this->pdo->prepare($sql);
$stmt->execute();
$article = $stmt->fetchAll(PDO::FETCH_ASSOC);
foreach ($article as $art)
{
echo '<li>
<span>[新闻]</span>
<a href="article.php?id='.$art['id'].'" >'.$art['title'].'</a>
</li>';
}
}
//首页新闻频道图片
public function selImage($a){
// 变量$limit控制显示的行数
$limit='limit '.$a;
// 图片路径
$imgPath='static/images/';
$sql = 'select `*` from `article` '.$limit;
$stmt = $this->pdo->prepare($sql);
$stmt->execute();
$article = $stmt->fetchAll(PDO::FETCH_ASSOC);
foreach ($article as $art)
{
echo '<dd class="phpcn-col-md6">
<a href="article.php?id='.$art['id'].'" ><img src='.$imgPath.$art['img'].'>
<span>'.$art['title'].'</span>
</a>
</dd>';
}
}
//首页图片频道图片
public function imageChan($a,$b){
if ($b==0){
$limit='limit '.$a;
}else{
$limit='limit '.$a.','.$b;
}
// 图片频道图片路径
$imgpath='static/images/shop/';
$sql = 'select `*` from `images`'.$limit ;
$stmt = $this->pdo->prepare($sql);
$stmt->execute();
$pic = $stmt->fetchAll(PDO::FETCH_ASSOC);
foreach ($pic as $p)
{
echo '<li class="phpcn-col-md6">
<a href="images-content.php?id='.$p['id'].'"><img src='.$imgpath.$p['imageurl'].'>
<span>'.$p['titlename'].'</span>
</a>
</dd></li>';
}
}
// 首页商品
public function selShop(){
$sql = 'select `*` from `shop` ';
$stmt = $this->pdo->prepare($sql);
$stmt->execute();
$pic = $stmt->fetchAll(PDO::FETCH_ASSOC);
foreach ($pic as $p)
{
echo '<dd class="phpcn-col-md3">
<a href="shop-content.php?id='.$p['id'].'"><img src="static/images/shop/'.$p['img'].'"></a>
<a href="shop-content.php?id='.$p['id'].'">'.$p['title'].'<a href="" class="phpcn-mt-10">
<span class="price">'.$p['price'].'</span>
<span class="tags">美女</span></a></dd>';
}
}
//**************************************************************************************
//列表页面
public function listSel($a=10){
// 变量$limit控制显示的行数默认10行
$limit='limit '.$a;
$sql = 'select `*` from `article` '. $limit;
$stmt = $this->pdo->prepare($sql);
$stmt->execute();
$article = $stmt->fetchAll(PDO::FETCH_ASSOC);
foreach ($article as $cate)
{
echo ' <div class="phpcn-clear phpcn-mt-30">
<div class="phpcn-col-md4"><img src="static/images/'. $cate['img']. '"> </div>
<div class="phpcn-col-md8">
<h2><a href="article.php?id='.$cate['id'].'">'.$cate['title'].'</a></h2>
<div class="info phpcn-mt-10">'.$cate['title'].'
</div>
<div>
<a href="article.php?id='.$cate['id'].'">区块链头条 '.$cate['times'].'</a>
<span>
<i class="phpcn-icon phpcn-icon-icon_yulan phpcn-r phpcn-mr-20">'.$cate['click'].'</i>
</span>
</div>
</div>
</div>' ;
}
}
//列表页推荐阅读
public function reading ($limit){
$limit='limit'.$limit;
$sql = 'select `*` from `article` '. $limit;
$stmt = $this->pdo->prepare($sql);
$stmt->execute();
$article = $stmt->fetchAll(PDO::FETCH_ASSOC);
foreach ($article as $cate) {
echo '<li class="phpcn-col-md3">
<div>
<a href="article.php?id='.$cate['id'].'"> <img src="static/images/'.$cate['img'].'"> </a>
<a href="article.php?id='.$cate['id'].'">'.$cate['title'].'</a>
</div>
</li>';
}
}
//网页评论
public function comments($limit){
$limit='limit '.$limit;
$sql = 'select `*` from `article` '. $limit;
$stmt = $this->pdo->prepare($sql);
$stmt->execute();
$article = $stmt->fetchAll(PDO::FETCH_ASSOC);
$i=1;
foreach ($article as $cate) {
echo '<li>
<span class="hot">'.$i++.'</span>
<a href="article.php?id='.$cate['id'].'">'.$cate['title'].'</a>
</li>';
}
}
}
//实例化查询对象
$selHead=new select();
?>
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<link rel="stylesheet" href="static/css/style.css?ddd" media="all">
<link rel="stylesheet" href="static/css/home.css?ddd" media="all">
<script src="static/js/jquery3.4.1.js"></script>
<title>php中文网后台</title>
</head>
<body>
<div class="home-top phpcn-clear">
<ul class='phpcn-col-md10'>
<li ><a href="index.php">首页</a></li>
<?php $selHead->selHead(); ?>
</ul>
<dl class='phpcn-col-md2'>
<dd>
<a href="">免费注册</a>
</dd>
<dd>
<a href=""><i class="phpcn-icon phpcn-icon-huiyuan2"></i>登陆</a>
</dd>
</dl>
</div>
<?php
include __DIR__ . "/head.php";
?>
<!DOCTYPE html>
<!--头部结束--->
<!--LOGO与搜索--->
<div class="phpcn-main phpcn-mt-60">
<div class="logo-top phpcn-clear">
<div class="phpcn-col-md4">
<a href="">
<img src="static/images/logo.png">
</a>
</div>
<div class="phpcn-col-md4">
<input type="" name="">
<i class="phpcn-icon phpcn-icon-jinduchaxun"></i>
</div>
<div class="phpcn-col-md4">
<dl class="erwei-code">
<dd>
<a href=""><i class="phpcn-icon phpcn-icon-huiyuan1"></i></a>
</dd>
<dd>
<a href=""><i class="phpcn-icon phpcn-icon-danmu1"></i></a>
</dd>
<dd>
<a href=""><i class="phpcn-icon phpcn-icon-duoxuankuang1"></i></a>
</dd>
<dd>
<a href=""><i class="phpcn-icon phpcn-icon-jishufuwu"></i></a>
</dd>
<dd>
<a href=""><i class="phpcn-icon phpcn-icon-peiwangyindao"></i></a>
</dd>
<dd>
<a href=""><i class="phpcn-icon phpcn-icon-wenjianjia"></i></a>
</dd>
<dd>
<a href=""><i class="phpcn-icon phpcn-icon-huiyuan1"></i></a>
</dd>
</dl>
</div>
</div>
<!--LOGO与搜索结束--->
<!--菜单 开始 --->
<div class="menu phpcn-mt-30 phpcn-clear">
<div >
<dl class="phpcn-col-md3">
<dt>
<i class="phpcn-icon phpcn-icon-gongdan"></i>
<span>资讯看学</span>
</dt>
<dd>
<a href="">器材</a>
<a href="">大师</a>
<a href="">学院</a>
<a href="">影赛</a>
<a href="">器材</a>
<a href="">大师</a>
<a href="">学院</a>
<a href="">影赛</a>
</dd>
</dl>
<dl class="phpcn-col-md2">
<dt>
<i class="phpcn-icon phpcn-icon-renwujincheng"></i>
<span>资讯看学</span>
</dt>
<dd>
<a href="">器材</a>
<a href="">大师</a>
<a href="">学院</a>
<a href="">影赛</a>
</dd>
</dl>
<dl class="phpcn-col-md2">
<dt>
<i class="phpcn-icon phpcn-icon-gongdan"></i>
<span>资讯看学</span>
</dt>
<dd>
<a href="">器材</a>
<a href="">大师</a>
<a href="">学院</a>
<a href="">影赛</a>
</dd>
</dl>
<dl class="phpcn-col-md4">
<dt>
<i class="phpcn-icon phpcn-icon-DOC"></i>
<span>资讯看学</span>
</dt>
<dd>
<a href="">器材</a>
<a href="">大师</a>
<a href="">学院</a>
<a href="">影赛</a>
<a href="">器材</a>
<a href="">大师</a>
<a href="">学院</a>
<a href="">影赛</a>
<a href="">器材</a>
<a href="">大师</a>
<a href="">学院</a>
<a href="">影赛</a>
</dd>
</dl>
</div>
</div>
<!--菜单 结束--->
<!--幻灯片-->
<div class="phpcn-clear banner phpcn-mt-30 ">
<div class="phpcn-col-md9 " >
<div class="pi">
<div class="pike">
<!-- // 换灯图片-->
<?php $selHead->selPic(); ?>
</div>
<div class="pike_prev"></div>
<div class="pike_next"></div>
<div class="pike_spot"></div>
</div>
</div>
<div class="phpcn-col-md3" >
<img class="phpcn-r banner-right" src="static/images/banner-right.jpg">
</div>
</div>
<!--幻灯片结束-->
<!--新聞開始-->
<div class="home-news phpcn-mt-30">
<div class="title phpcn-mb-20">
<a href="" class="tit">新闻资讯</a>
<span class="phpcn-r"><a href="">更多</a></span>
</div>
<div class="phpcn-clear ">
<div class="phpcn-col-md4">
<img src="static/images/news.jpg">
</div>
<div class="phpcn-col-md4">
<!-- 查询首页h3红色标题图片-->
<?php $selHead->selTitle(); ?>
<ul>
<!-- //首页新闻资讯-->
<!-- 显示4行-->
<?php $selHead->selNew(4); ?>
</ul>
</div>
<div class="phpcn-col-md4">
<!-- 查询首页h3红色标题图片-->
<?php $selHead->selTitle(); ?>
<ul>
<!-- //首页新闻资讯-->
<!-- 显示4行-->
<?php $selHead->selNew(4); ?>
</ul>
</div>
</div>
<div class="phpcn-clear phpcn-mt-30">
<div class="phpcn-col-md4">
<dl class="pic">
<!-- //首页新闻频道图片-->
<?php $selHead->selImage(2); ?>
</dl>
</div>
<div class="phpcn-col-md4">
<ul>
<!-- 显示5行-->
<?php $selHead->selNew(5); ?>
</ul>
</div>
<div class="phpcn-col-md4">
<ul>
<!-- 显示5行-->
<?php $selHead->selNew(5); ?>
</ul>
</div>
</div>
</div>
<!--新聞開始結束-->
</div>
<!--LOGO与搜索结束--->
<!--图片开始-->
<div class="phpcn-clear phpcn-mt-30" style='background:#eee;'>
<div class="phpcn-main">
<div class="phpcn-clear home-img">
<div class="title-header phpcn-mb-40 phpcn-mt-20"><span>图片专区</span></div>
<div class=" phpcn-clear phpcn-col-space15">
<div class="phpcn-col-md4">
<div class="bg">
<div class="title "><a href="">美女</a> <span>纵观摄影艺术</span></div>
<ul class="phpcn-clear">
<!-- 根据传递的参数不同调用不同的数据-->
<?php $selHead->imageChan(0,4); ?>
</ul>
</div>
</div>
<div class="phpcn-col-md4">
<div class="bg">
<div class="title "><a href="">美女</a> <span>纵观摄影艺术</span></div>
<ul class="phpcn-clear">
<!-- 根据传递的参数不同调用不同的数据-->
<?php $selHead->imageChan(0,4); ?>
</ul>
</div>
</div>
<div class="phpcn-col-md4">
<div class="bg">
<div class="title "><a href="">美女</a> <span>纵观摄影艺术</span></div>
<ul class="phpcn-clear">
<!--根据传递的参数不同调用不同的数据-->
<?php $selHead->imageChan(0,4); ?>
</ul>
</div>
</div>
</div>
</div>
<!--图片开始结束-->
<!--商城开始-->
<div class="phpcn-clear">
<div class="title-header phpcn-mb-40 phpcn-mt-20"><span>二手交易</span></div>
<div class="home-shop phpcn-clear">
<div class="title "><a href="">抢好货</a> <span>0低价、便捷、安全、快速</span></div>
<div class='head-tags'>
<span>热门分类:</span>
<a href="">美女写真</a>
<a href="">日本美女</a>
<a href="">美国美女</a>
<a href="">国内美女</a>
<a href="">AV美女</a>
</div>
<div class="phpcn-clear">
<div class="phpcn-col-md8">
<dl>
<!-- 商品调用-->
<?php $selHead->selShop(); ?>
</dl>
</div>
<div class="phpcn-col-md4 home-ad">
<ul class="phpcn-clear">
<li class="phpcn-col-md6">
<a href="">
<img src="static/images/ad/1.png">
</a>
</li>
<li class="phpcn-col-md6">
<a href="">
<img src="static/images/ad/2.png">
</a>
</li>
<li class="phpcn-col-md6">
<a href="">
<img src="static/images/ad/3.png">
</a>
</li>
<li class="phpcn-col-md6">
<a href="">
<img src="static/images/ad/4.png">
</a>
</li>
</ul>
<div class="phpcn-mt-10">
<a href="">
<img src="static/images/ad/image.png" class="slogan">
</a>
</div>
<div class="phpcn-mt-10" >
<a href="">
<img src="static/images/ad/ad2.jpg" class="slogan ">
</a>
</div>
</div>
</div>
</div>
</div>
<!--商城开始结束-->
</div>
</div>
<!--友情链接 -->
<div class="phpcn-clear phpcn-mt-30">
<div class="phpcn-main links">
<div class="title-header phpcn-mb-40 phpcn-mt-20"><span>合作网站</span></div>
<div>
<a href="">php中文网</a>
<a href="">百度中国</a>
<a href="">phpstudy</a>
<a href="">php中文网</a>
<a href="">百度中国</a>
<a href="">phpstudy</a>
<a href="">php中文网</a>
<a href="">百度中国</a>
<a href="">phpstudy</a>
<a href="">php中文网</a>
<a href="">百度中国</a>
<a href="">phpstudy</a>
<a href="">php中文网</a>
<a href="">百度中国</a>
<a href="">phpstudy</a>
<a href="">php中文网</a>
<a href="">百度中国</a>
<a href="">phpstudy</a>
<a href="">php中文网</a>
<a href="">百度中国</a>
<a href="">phpstudy</a>
<a href="">php中文网</a>
<a href="">百度中国</a>
<a href="">phpstudy</a>
</div>
</div>
</div>
<!--友情链接结束-->
<!--网站底部-->
<?php include __DIR__ . '/foot.php'; ?>
<!--网站底部-->
<script src="static/js/pin.js"></script>
<script>
var myPi = new Xpcms(".pi", {
type: 1, // 轮播的类型(1渐隐)
automatic: true, //是否自动轮播 (默认false)
autoplay: 2000, //自动轮播毫秒 (默认3000)
hover: true, //鼠标悬停轮播 (默认false)
arrowColor: "yellow", //箭头颜色 (默认绿色)
arrowBackgroundType: 2, //箭头背景类型 (1: 方形, 2:圆形)
arrowBackground: 1, //箭头背景色 (1:白色,2:黑色, 默认:无颜色)
arrowTransparent: 0.2, //箭头背景透明度 (默认: 0.5)
spotColor: "white",//圆点颜色 (默认: 白色)
spotType: 1, //圆点的形状 (默认: 圆形, 1:圆形, 2.矩形)
spotSelectColor: "red", //圆点选中颜色 (默认绿色)
spotTransparent: 0.8, //圆点透明度 (默认0.8)
mousewheel: true, //是否开启鼠标滚动轮播(默认false)
drag: false, //是否开启鼠标拖动 (默认为: true, 如不需要拖动设置false即可)
loop: true, //是否循环轮播 (默认为: false)
});
var progress = $('.phpcn-progress .phpcn-row');
jQuery.each(progress, function(){
//console.log($(this).find('.phpcn-progress-percent').attr('class'));
$(this).find('.phpcn-progress-bor').css('width',$(this).find('.phpcn-progress-percent').html());
});
$('.phpcn-dl dl').last().css('border-bottom','1px solid #C9C9C9');
$('#admin-select').mouseover(function(){
//alert($(this).find('dl').css('display'));
$(this).find('dl').show();
});
$('#admin-select').find('dl').mouseout(function(){
$(this).hide();
});
$('.phpcn-button').mouseover(function(){
$(this).addClass('phpcn-button-hover');
});$('.phpcn-button').mouseout(function(){
$(this).removeClass('phpcn-button-hover');
});
$('.tree').css('min-height',$(document).height()-160);
$('.tree').find('li').click(function(){
//alert($('.tree').find('dl').css('display'));
//$('.tree').find('dl').hide();
if($(this).find('dl').css('display')=='none'){
$(this).find('i').removeClass('phpcn-icon-down');
$(this).find('i').addClass('phpcn-icon-up');
$('.tree').find('dl').hide();
$(this).find('dl').show();
}else{
$(this).find('i').removeClass('phpcn-icon-up');
$(this).find('i').addClass('phpcn-icon-down');
$(this).find('dl').hide();
}
});
//table窗口
$('.tree').find('a').click(function(){
//alert($('.tree').find('dl').css('display'));
//$('.tree').find('dl').hide();
var tabs=$('.phpcn-tab-title a');
tree=$(this);
jQuery.each(tabs, function(){
$('.phpcn-tab-title li').removeClass('on');
if($(this).attr('data')!=tree.attr('data')){
var tabs=$('.phpcn-tab-title ul');
$(this).removeClass('on');
var html='<li class="on"><i></i><dd>'+tree.html()+' <a class="phpcn-icon phpcn-icon-guanbi" data='+tree.attr('data')+' href="javascript:;"></a></dd></li>';
tabs.append(html);
return false;
}
});
});
//table窗口
$('.phpcn-tab-title').find('.phpcn-icon-guanbi').click(function(){
alert();
$(this).parent().parent('li').remove();
});
$('.phpcn-tab-title').find('li').mouseover(function(){
var i=$(this).find('i');
$('.phpcn-tab-title').find('li').find('i').css('width','0%');
if($(this).css('background')!='#f6f6f6'){
$('.phpcn-tab-title').find('li').css('background','none');
$(this).css('background','#f6f6f6');
}
if(i.css('width')!='100%'){
i.css('width','100%');
}
});
</script>
</body>
</html>
<?php
include __DIR__ . "/head.php";
class shop extends select
{
public function shopList($limit=8){
// 变量$limit控制显示的行数默认10行
$limit='limit '.$limit;
// 图片路径
$imagePath='static/images/shop/';
$sql = 'select `*` from `shop` '. $limit;
$stmt = $this->pdo->prepare($sql);
$stmt->execute();
$article = $stmt->fetchAll(PDO::FETCH_ASSOC);
foreach ($article as $cate)
{
echo '<dd class="phpcn-col-md2">
<a href="shop-content.php?id='.$cate['id'].'"><img src="'.$imagePath.$cate['img'].'"></a>
<a href="shop-content.php?id='.$cate['id'].'">'.$cate['title'].'</a>
<a href="" class="phpcn-mt-10">
<span class="price">'.$cate['price'].'</span>
<span class="tags">美女</span>
</a>
</dd>' ;
}
}
}
$shop1=new shop();
?>
<div class="phpcn-clear category phpcn-p-20">
<div class="phpcn-main">
<div class="phpcn-col-md12 search">
<div class="phpcn-col-md3">
<a href="">
<img src="static/images/logo.png" class="logo">
</a>
</div>
<div class="phpcn-col-md6">
<ul>
<li>
<a href="" class="on">商品</a>
<a href="">服务商</a>
</li>
<li class="phpcn-clear">
<input type="" name=""><button class="phpcn-button s-btn">搜索</button>
</li>
</ul>
</div>
<div class="phpcn-col-md3">
<button class="phpcn-button phpcn-bg-red">免费入驻</button>
</div>
</div>
<div class="phpcn-col-md12 phpcn-mt-60">
<div class="phpcn-col-md3 all-cat">
所有产品分类 <i class="phpcn-icon phpcn-icon-liebiao phpcn-r"></i>
</div>
<div class="phpcn-col-md9">
<div class="cat-nav">
<a href="" class="on">首页</a>
<a href="">3c数码</a>
<a href="">生活用品 <span class='phpcn-badge'>新</span></a>
<a href="">名字名画</a>
</div>
</div>
</div>
<div class="phpcn-col-md3 cat-left">
<ul>
<li>
<h2><a href="">企业软件</a></h2>
<span>
<a href="">微信推广</a>
<a href=""> QQ推广 </a>
<a href=""> 公众号推广 </a>
<a href=""> 淘客软件 </a>
<a href=""> 网络推广</a>
<a href=""> SEO软件</a>
</span>
</li>
<li>
<h2><a href="">企业软件</a></h2>
<span>
<a href="">微信推广</a>
<a href=""> QQ推广 </a>
<a href=""> 公众号推广 </a>
<a href=""> 淘客软件 </a>
<a href=""> 网络推广</a>
<a href=""> SEO软件</a>
</span>
</li>
<li>
<h2><a href="">企业软件</a></h2>
<span>
<a href="">微信推广</a>
<a href=""> QQ推广 </a>
<a href=""> 公众号推广 </a>
<a href=""> 淘客软件 </a>
<a href=""> 网络推广</a>
<a href=""> SEO软件</a>
</span>
</li>
</ul>
</div>
<div class="phpcn-col-md9">
<div class="shop-banner">
<img src="static/images/4.jpg">
</div>
</div>
</div>
</div>
<div class="phpcn-main">
<!--商城开始-->
<div class="phpcn-clear">
<div class="title-header phpcn-mb-40 phpcn-mt-20"><span>名画</span></div>
<div class="home-shop phpcn-clear">
<div class="title "><a href="">抢好货</a> <span>0低价、便捷、安全、快速</span></div>
<div class='head-tags'>
<span>热门分类:</span>
<a href="">美女写真</a>
<a href="">日本美女</a>
<a href="">美国美女</a>
<a href="">国内美女</a>
<a href="">AV美女</a>
</div>
<div class="phpcn-clear">
<div class="phpcn-col-md8">
<dl>
<?php $shop1->shopList(8);?>
</dl>
</div>
<div class="phpcn-col-md4 home-ad">
<ul class="phpcn-clear">
<li class="phpcn-col-md6">
<a href="">
<img src="static/images/ad/1.png">
</a>
</li>
<li class="phpcn-col-md6">
<a href="">
<img src="static/images/ad/2.png">
</a>
</li>
<li class="phpcn-col-md6">
<a href="">
<img src="static/images/ad/3.png">
</a>
</li>
<li class="phpcn-col-md6">
<a href="">
<img src="static/images/ad/4.png">
</a>
</li>
</ul>
<div class="phpcn-mt-10">
<a href="">
<img src="static/images/ad/image.png" class="slogan">
</a>
</div>
<div class="phpcn-mt-10" >
<a href="">
<img src="static/images/ad/ad2.jpg" class="slogan ">
</a>
</div>
</div>
</div>
</div>
<!--商品分类-->
<div class="title-header phpcn-mb-40 phpcn-mt-20"><span>真写</span></div>
<div class="home-shop phpcn-clear">
<div class="title "><a href="">抢好货</a> <span>0低价、便捷、安全、快速</span></div>
<div class='head-tags'>
<span>热门分类:</span>
<a href="">美女写真</a>
<a href="">日本美女</a>
<a href="">美国美女</a>
<a href="">国内美女</a>
<a href="">AV美女</a>
</div>
<div class="phpcn-clear">
<div class="phpcn-col-md12">
<dl>
<?php $shop1->shopList(12);?>
</dl>
</div>
</div>
</div>
<!--商品分类结束--><!--商品分类-->
<div class="title-header phpcn-mb-40 phpcn-mt-20"><span>真写</span></div>
<div class="home-shop phpcn-clear">
<div class="title "><a href="">抢好货</a> <span>0低价、便捷、安全、快速</span></div>
<div class='head-tags'>
<span>热门分类:</span>
<a href="">美女写真</a>
<a href="">日本美女</a>
<a href="">美国美女</a>
<a href="">国内美女</a>
<a href="">AV美女</a>
</div>
<div class="phpcn-clear">
<div class="phpcn-col-md12">
<dl>
<?php $shop1->shopList(12);?>
</dl>
</div>
</div>
</div>
<!--商品分类结束-->
</div>
<!--商城开始结束-->
</div>
</div>
</div>
<!--网站底部-->
<?php include __DIR__ . '/foot.php'; ?>
<!--网站底部-->
<script src="static/js/pin.js"></script>
<script>
var myPi = new Pike(".pi", {
type: 1, // 轮播的类型(1渐隐)
automatic: true, //是否自动轮播 (默认false)
autoplay: 2000, //自动轮播毫秒 (默认3000)
hover: true, //鼠标悬停轮播 (默认false)
arrowColor: "yellow", //箭头颜色 (默认绿色)
arrowBackgroundType: 2, //箭头背景类型 (1: 方形, 2:圆形)
arrowBackground: 1, //箭头背景色 (1:白色,2:黑色, 默认:无颜色)
arrowTransparent: 0.2, //箭头背景透明度 (默认: 0.5)
spotColor: "white",//圆点颜色 (默认: 白色)
spotType: 1, //圆点的形状 (默认: 圆形, 1:圆形, 2.矩形)
spotSelectColor: "red", //圆点选中颜色 (默认绿色)
spotTransparent: 0.8, //圆点透明度 (默认0.8)
mousewheel: true, //是否开启鼠标滚动轮播(默认false)
drag: false, //是否开启鼠标拖动 (默认为: true, 如不需要拖动设置false即可)
loop: true, //是否循环轮播 (默认为: false)
});
var progress = $('.phpcn-progress .phpcn-row');
jQuery.each(progress, function(){
//console.log($(this).find('.phpcn-progress-percent').attr('class'));
$(this).find('.phpcn-progress-bor').css('width',$(this).find('.phpcn-progress-percent').html());
});
$('.phpcn-dl dl').last().css('border-bottom','1px solid #C9C9C9');
$('#admin-select').mouseover(function(){
//alert($(this).find('dl').css('display'));
$(this).find('dl').show();
});
$('#admin-select').find('dl').mouseout(function(){
$(this).hide();
});
$('.phpcn-button').mouseover(function(){
$(this).addClass('phpcn-button-hover');
});$('.phpcn-button').mouseout(function(){
$(this).removeClass('phpcn-button-hover');
});
$('.tree').css('min-height',$(document).height()-160);
$('.tree').find('li').click(function(){
//alert($('.tree').find('dl').css('display'));
//$('.tree').find('dl').hide();
if($(this).find('dl').css('display')=='none'){
$(this).find('i').removeClass('phpcn-icon-down');
$(this).find('i').addClass('phpcn-icon-up');
$('.tree').find('dl').hide();
$(this).find('dl').show();
}else{
$(this).find('i').removeClass('phpcn-icon-up');
$(this).find('i').addClass('phpcn-icon-down');
$(this).find('dl').hide();
}
});
//table窗口
$('.tree').find('a').click(function(){
//alert($('.tree').find('dl').css('display'));
//$('.tree').find('dl').hide();
var tabs=$('.phpcn-tab-title a');
tree=$(this);
jQuery.each(tabs, function(){
$('.phpcn-tab-title li').removeClass('on');
if($(this).attr('data')!=tree.attr('data')){
var tabs=$('.phpcn-tab-title ul');
$(this).removeClass('on');
var html='<li class="on"><i></i><dd>'+tree.html()+' <a class="phpcn-icon phpcn-icon-guanbi" data='+tree.attr('data')+' href="javascript:;"></a></dd></li>';
tabs.append(html);
return false;
}
});
});
//table窗口
$('.phpcn-tab-title').find('.phpcn-icon-guanbi').click(function(){
alert();
$(this).parent().parent('li').remove();
});
$('.phpcn-tab-title').find('li').mouseover(function(){
var i=$(this).find('i');
$('.phpcn-tab-title').find('li').find('i').css('width','0%');
if($(this).css('background')!='#f6f6f6'){
$('.phpcn-tab-title').find('li').css('background','none');
$(this).css('background','#f6f6f6');
}
if(i.css('width')!='100%'){
i.css('width','100%');
}
});
</script>
</body>
</html>
<?php
include __DIR__ . "/head.php";
//接受url中专来的参数
if (empty($_GET['id'])){
die('参数错误');
}
$a=$_GET['id'];
class shop_content extends Sql_db
{
public $title;
public $img;
public $time;
public $sel;
public $body;
public $price;
public $imgPath='static/images/shop/';
public function __construct()
{
// 链接数据库
$this->pdo= parent::conect(DB_HOST,DB_NAME,DB_USER,DB_PASSWORD);
}
public function shopContent($a=1){
$a='`id`='.$a;
$sql = 'select `*` from `shop` where '.$a;
$stmt = $this->pdo->prepare($sql);
$stmt->execute();
$article = $stmt->fetchAll(PDO::FETCH_ASSOC);
foreach ($article as $cate){
$this->title=$cate['title'];
$this->time=$cate['times'];
$this->sel=$cate['sel'];
$this->body=$cate['body'];
$this->price=$cate['price'];
$this->img=$cate['img'];
}
}
//热销商品
public function hot($limit){
$limit='limit '.$limit;
$sql = 'select `*` from `shop` '.$limit;
$stmt = $this->pdo->prepare($sql);
$stmt->execute();
$article = $stmt->fetchAll(PDO::FETCH_ASSOC);
foreach ($article as $cate){
echo '<li><a href="shop-content.php?id='.$cate['id'].'" ><div class="phpcn-ps-r"> <img src="static/images/shop/'.$cate['img'].'">
<h3>'.$cate['title'].'</span></h3></div><div class="attribute">
<span class="phpcn-l">热销11</span>
<span class="phpcn-r">'.$cate['price'].'</span>
</div>
</a>
</li>';
}
}
}
$shop=new shop_content();
$shop->shopContent($a);
?>
<div class="phpcn-clear category phpcn-p-20 phpcn-bg-fff" >
<div class="phpcn-main ">
<div class="phpcn-col-md12 search ">
<div class="phpcn-col-md3">
<a href="">
<img src="static/images/logo.png" class="logo">
</a>
</div>
<div class="phpcn-col-md6">
<ul>
<li>
<a href="" class="on">商品</a>
<a href="">服务商</a>
</li>
<li class="phpcn-clear">
<input type="" name=""><button class="phpcn-button s-btn">搜索</button>
</li>
</ul>
</div>
<div class="phpcn-col-md3">
<button class="phpcn-button phpcn-bg-red">免费入驻</button>
</div>
</div>
<div class="phpcn-col-md12 phpcn-mt-60 ">
<div class="phpcn-col-md3 all-cat borb">
所有产品分类 <i class="phpcn-icon phpcn-icon-liebiao phpcn-r"></i>
</div>
<div class="phpcn-col-md9 ">
<div class="cat-nav borb">
<a href="" class="on">首页</a>
<a href="">3c数码</a>
<a href="">生活用品 <span class='phpcn-badge'>新</span></a>
<a href="">名字名画</a>
</div>
</div>
</div>
</div>
</div>
<div class="phpcn-clear phpcn-mt-20">
<div class="phpcn-main phpcn-bg-fff ">
<!--商品详情-->
<div class="shop phpcn-p-20">
<div class="path phpcn-clear">
<a href="">首页</a>
<span>->></span>
<a href="">3C数码</a>
<span>->></span>
<a href="">笔记本电脑</a>
<span>->></span>
</div>
<div class="detail phpcn-clear phpcn-mt-30">
<div class="phpcn-col-md4">
<img src="<?php echo $shop->imgPath.$shop->img;?>" class="buy-img">
</div>
<div class="phpcn-col-md8">
<h1><?php echo $shop->title; ?></h1>
<div class="price">
<span>本站特惠</span><strong >¥<?php echo $shop->price; ?></strong>
</div>
<div class="buy-lieval">
<ul>
<li class="br">销量:<span><?php echo $shop->sel; ?></span></li>
<li class="br">累积评价:<span>0</span></li>
<li>好评率:<span>100%</span></li>
</ul>
</div>
<div class="buy-nums phpcn-clear">
<ul>
<li>购买数量</li>
<li class="phpcn-ps-r">
<input type="text" name="" id="quantity" value="1" size="3" maxlength="6" class="fl" style="border-radius:0;">
<i class="phpcn-icon phpcn-icon-up"></i>
<i class="phpcn-icon phpcn-icon-down"></i>
</li>
</ul>
</div>
<div class="buy-btn phpcn-clear phpcn-mt-20">
<button class="phpcn-button phpcn-bg-red">立即购买</button>
<button class="phpcn-button "><i class="phpcn-icon phpcn-icon-icon_tianjia"></i>加入购物车</button>
</div>
<div class="buy-guarantee phpcn-clear phpcn-mt-20">
<ul>
<li> <i class="phpcn-icon phpcn-icon-zhanghaoquanxianguanli"></i> 本站保障</li>
<li> <i class="phpcn-icon phpcn-icon-icon_safety"></i>企业认证</li>
<li><i class="phpcn-icon phpcn-icon-tianshenpi"></i>退款承诺</li>
<li><i class="phpcn-icon phpcn-icon-kuaisubianpai"></i>免费换货</li>
</ul>
</div>
</div>
</div>
</div>
<!--商品详情结束-->
</div>
</div>
<div class="phpcn-clear phpcn-mt-20">
<div class="phpcn-main shop-conten">
<div class="phpcn-col-md2 ">
<div class="categorymenu phpcn-clear cat-width">
<ul>
<li class="phpcn-col-md6"><a href="">商品详情</a></li>
<li class="phpcn-col-md6"><a href="">案例/演示</a></li>
</ul>
</div>
<div class="shop-left-list">
<ul>
<!-- 根据传递的参数不同可调用不用的商品-->
<?php $shop->hot(5); ?>
</ul>
</div>
</div>
<div class="phpcn-col-md10 ">
<div class="content ">
<div class="categorymenu cat-nav-width">
<ul class="phpcn-clear">
<li class="phpcn-col-md1"><a href="" class="on">商品详情</a></li>
<li class="phpcn-col-md1"><a href="">案例/演示</a></li>
<li class="phpcn-col-md1"><a href="">常见问题</a></li>
<li class="phpcn-col-md1"><a href="">累计评价</a></li>
<li class="phpcn-col-md1"><a href="">产品咨询</a></li>
</ul>
</div>
<article class="phpcn-clear">
<?php echo $shop->body; ?>
</article>
<!--评论--->
<div class="comment phpcn-mt-30 ">
<div class="title phpcn-mb-30"><span>网页评论</span></div>
<div class="phpcn-clear">
<div class="phpcn-col-md1"><img class="user" src="static/images/user.png"> </div>
<div class="phpcn-col-md11">
<textarea>我来评论两句</textarea>
<button class="phpcn-button phpcn-bg-red phpcn-button-hover phpcn-mt-10 phpcn-mb-10 phpcn-r">发表评论</button>
</div>
</div>
<div class="title phpcn-mb-30"><span>最新评论</span></div>
<div class="phpcn-clear">
<div class="phpcn-clear border">
<div class="phpcn-col-md1">
<img class="user" src="static/images/user.png">
</div>
<div class="phpcn-col-md11">
<ul class="phpcn-clear">
<li class="user-naem">
用户昵称
</li>
<li class="cont">留言内容</li>
<li>
<span>2019-08-08 14:46:05发表</span>
<span class="phpcn-r"><i class="phpcn-icon phpcn-icon-dianzan"></i> <a href="">回复 </a></span>
</li>
</ul>
</div>
</div>
<div class="phpcn-clear border">
<div class="phpcn-col-md1">
<img class="user" src="static/images/user.png">
</div>
<div class="phpcn-col-md11">
<ul class="phpcn-clear">
<li class="user-naem">
用户昵称
</li>
<li class="cont">留言内容</li>
<li>
<span>2019-08-08 14:46:05发表</span>
<span class="phpcn-r"><i class="phpcn-icon phpcn-icon-dianzan"></i> <a href="">回复 </a></span>
</li>
</ul>
</div>
</div>
<div class="phpcn-clear border">
<div class="phpcn-col-md1">
<img class="user" src="static/images/user.png">
</div>
<div class="phpcn-col-md11">
<ul class="phpcn-clear">
<li class="user-naem">
用户昵称
</li>
<li class="cont">留言内容</li>
<li>
<span>2019-08-08 14:46:05发表</span>
<span class="phpcn-r"><i class="phpcn-icon phpcn-icon-dianzan"></i> <a href="">回复 </a></span>
</li>
</ul>
</div>
</div>
</div>
<div class="phpcn-clear border">
<div class="phpcn-col-md1">
<img class="user" src="static/images/user.png">
</div>
<div class="phpcn-col-md11">
<ul class="phpcn-clear">
<li class="user-naem">
用户昵称
</li>
<li class="cont">留言内容</li>
<li>
<span>2019-08-08 14:46:05发表</span>
<span class="phpcn-r"><i class="phpcn-icon phpcn-icon-dianzan"></i> <a href="">回复 </a></span>
</li>
</ul>
</div>
</div>
</div>
<!--评论结束--->
</div>
</div>
</div>
</div>
<!--网站底部-->
<?php include __DIR__ . '/foot.php'; ?>
<!--网站底部-->
<script src="static/js/pin.js"></script>
<script>
var myPi = new Pike(".pi", {
type: 1, // 轮播的类型(1渐隐)
automatic: true, //是否自动轮播 (默认false)
autoplay: 2000, //自动轮播毫秒 (默认3000)
hover: true, //鼠标悬停轮播 (默认false)
arrowColor: "yellow", //箭头颜色 (默认绿色)
arrowBackgroundType: 2, //箭头背景类型 (1: 方形, 2:圆形)
arrowBackground: 1, //箭头背景色 (1:白色,2:黑色, 默认:无颜色)
arrowTransparent: 0.2, //箭头背景透明度 (默认: 0.5)
spotColor: "white",//圆点颜色 (默认: 白色)
spotType: 1, //圆点的形状 (默认: 圆形, 1:圆形, 2.矩形)
spotSelectColor: "red", //圆点选中颜色 (默认绿色)
spotTransparent: 0.8, //圆点透明度 (默认0.8)
mousewheel: true, //是否开启鼠标滚动轮播(默认false)
drag: false, //是否开启鼠标拖动 (默认为: true, 如不需要拖动设置false即可)
loop: true, //是否循环轮播 (默认为: false)
});
var progress = $('.phpcn-progress .phpcn-row');
jQuery.each(progress, function(){
//console.log($(this).find('.phpcn-progress-percent').attr('class'));
$(this).find('.phpcn-progress-bor').css('width',$(this).find('.phpcn-progress-percent').html());
});
$('.phpcn-dl dl').last().css('border-bottom','1px solid #C9C9C9');
$('#admin-select').mouseover(function(){
//alert($(this).find('dl').css('display'));
$(this).find('dl').show();
});
$('#admin-select').find('dl').mouseout(function(){
$(this).hide();
});
$('.phpcn-button').mouseover(function(){
$(this).addClass('phpcn-button-hover');
});$('.phpcn-button').mouseout(function(){
$(this).removeClass('phpcn-button-hover');
});
$('.tree').css('min-height',$(document).height()-160);
$('.tree').find('li').click(function(){
//alert($('.tree').find('dl').css('display'));
//$('.tree').find('dl').hide();
if($(this).find('dl').css('display')=='none'){
$(this).find('i').removeClass('phpcn-icon-down');
$(this).find('i').addClass('phpcn-icon-up');
$('.tree').find('dl').hide();
$(this).find('dl').show();
}else{
$(this).find('i').removeClass('phpcn-icon-up');
$(this).find('i').addClass('phpcn-icon-down');
$(this).find('dl').hide();
}
});
//table窗口
$('.tree').find('a').click(function(){
//alert($('.tree').find('dl').css('display'));
//$('.tree').find('dl').hide();
var tabs=$('.phpcn-tab-title a');
tree=$(this);
jQuery.each(tabs, function(){
$('.phpcn-tab-title li').removeClass('on');
if($(this).attr('data')!=tree.attr('data')){
var tabs=$('.phpcn-tab-title ul');
$(this).removeClass('on');
var html='<li class="on"><i></i><dd>'+tree.html()+' <a class="phpcn-icon phpcn-icon-guanbi" data='+tree.attr('data')+' href="javascript:;"></a></dd></li>';
tabs.append(html);
return false;
}
});
});
//table窗口
$('.phpcn-tab-title').find('.phpcn-icon-guanbi').click(function(){
alert();
$(this).parent().parent('li').remove();
});
$('.phpcn-tab-title').find('li').mouseover(function(){
var i=$(this).find('i');
$('.phpcn-tab-title').find('li').find('i').css('width','0%');
if($(this).css('background')!='#f6f6f6'){
$('.phpcn-tab-title').find('li').css('background','none');
$(this).css('background','#f6f6f6');
}
if(i.css('width')!='100%'){
i.css('width','100%');
}
});
</script>
</body>
</html>
<?php
include __DIR__ . "/head.php";
?>
<!--顶部结束-->
<style>
.pi{
width: 100%;
height: 310px;
}
</style>
<div class="web-top phpcn-box-s phpcn-clear phpcn-mb-30">
<div class="phpcn-main">
<div class="phpcn-col-md7">
<span class="web-tit">php中文网</span>
<span class="web-name">图片站</span>
</div>
<div class="phpcn-col-md5">
<input type="" name=""> <i class="phpcn-icon phpcn-icon-sousuo2" placeholder='关键字搜索'></i>
</div>
</div>
</div>
<div class="phpcn-main">
<div class="phpcn-clear">
<div class="phpcn-col-md8">
<div class="pi">
<div class="pike">
<?php $selHead->selPic(); ?>
</div>
<div class="pike_prev"></div>
<div class="pike_next"></div>
<div class="pike_spot"></div>
</div>
</div>
<div class="phpcn-col-md4">
<div class="image-right">
<div class="hot-article">
<div class="title"><span>r热闹推荐</span></div>
<ul>
<!-- 根据参数可调用不同的图片-->
<?php $selHead->comments(6); ?>
</ul>
</div>
</div>
</div>
</div>
</div>
<!--图片开始-->
<div class="phpcn-clear phpcn-mt-30" style='background:#eee;'>
<div class="phpcn-main">
<div class="phpcn-clear home-img">
<div class="title-header phpcn-mb-40 phpcn-mt-20"><span>图片专区</span></div>
<div class=" phpcn-clear phpcn-col-space15">
<div class="phpcn-col-md4">
<div class="bg">
<div class="title "><a href="">美女</a> <span>纵观摄影艺术</span></div>
<ul class="phpcn-clear">
<!-- 根据参数可调用不同的图片-->
<?php $selHead->imageChan(1,8); ?>
</ul>
</div>
</div>
<div class="phpcn-col-md4">
<div class="bg">
<div class="title "><a href="">美女</a> <span>纵观摄影艺术</span></div>
<ul class="phpcn-clear">
<!-- 根据参数可调用不同的图片-->
<?php $selHead->imageChan(2,8); ?>
</ul>
</div>
</div>
<div class="phpcn-col-md4">
<div class="bg">
<div class="title "><a href="">美女</a> <span>纵观摄影艺术</span></div>
<ul class="phpcn-clear">
<!-- 根据参数可调用不同的图片-->
<?php $selHead->imageChan(1,8); ?>
</ul>
</div>
</div>
</div>
</div>
</div>
<!--图片开始结束-->
<!--图片开始-->
<div class="phpcn-clear phpcn-mt-30" style='background:#eee;'>
<div class="phpcn-main">
<div class="phpcn-clear home-img">
<div class="title-header phpcn-mb-40 phpcn-mt-20"><span>图片专区</span></div>
<div class=" phpcn-clear phpcn-col-space15">
<div class="phpcn-col-md4">
<div class="bg">
<div class="title "><a href="">美女</a> <span>纵观摄影艺术</span></div>
<ul class="phpcn-clear">
<!-- 根据参数可调用不同的图片-->
<?php $selHead->imageChan(1,4); ?>
</ul>
</div>
</div>
<div class="phpcn-col-md4">
<div class="bg">
<div class="title "><a href="">美女</a> <span>纵观摄影艺术</span></div>
<ul class="phpcn-clear">
<!-- 根据参数可调用不同的图片-->
<?php $selHead->imageChan(2,4); ?>
</ul>
</div>
</div>
<div class="phpcn-col-md4">
<div class="bg">
<div class="title "><a href="">美女</a> <span>纵观摄影艺术</span></div>
<ul class="phpcn-clear">
<!-- 根据参数可调用不同的图片-->
<?php $selHead->imageChan(3,4); ?>
</ul>
</div>
</div>
</div>
</div>
</div>
<!--图片开始结束-->
<!--网站底部-->
<?php include __DIR__ . '/foot.php'; ?>
<!--网站底部-->
<script src="static/js/pin.js"></script>
<script>
var myPi = new Pike(".pi", {
type: 1, // 轮播的类型(1渐隐)
automatic: true, //是否自动轮播 (默认false)
autoplay: 2000, //自动轮播毫秒 (默认3000)
hover: true, //鼠标悬停轮播 (默认false)
arrowColor: "yellow", //箭头颜色 (默认绿色)
arrowBackgroundType: 2, //箭头背景类型 (1: 方形, 2:圆形)
arrowBackground: 1, //箭头背景色 (1:白色,2:黑色, 默认:无颜色)
arrowTransparent: 0.2, //箭头背景透明度 (默认: 0.5)
spotColor: "white",//圆点颜色 (默认: 白色)
spotType: 1, //圆点的形状 (默认: 圆形, 1:圆形, 2.矩形)
spotSelectColor: "red", //圆点选中颜色 (默认绿色)
spotTransparent: 0.8, //圆点透明度 (默认0.8)
mousewheel: true, //是否开启鼠标滚动轮播(默认false)
drag: false, //是否开启鼠标拖动 (默认为: true, 如不需要拖动设置false即可)
loop: true, //是否循环轮播 (默认为: false)
});
var progress = $('.phpcn-progress .phpcn-row');
jQuery.each(progress, function(){
//console.log($(this).find('.phpcn-progress-percent').attr('class'));
$(this).find('.phpcn-progress-bor').css('width',$(this).find('.phpcn-progress-percent').html());
});
$('.phpcn-dl dl').last().css('border-bottom','1px solid #C9C9C9');
$('#admin-select').mouseover(function(){
//alert($(this).find('dl').css('display'));
$(this).find('dl').show();
});
$('#admin-select').find('dl').mouseout(function(){
$(this).hide();
});
$('.phpcn-button').mouseover(function(){
$(this).addClass('phpcn-button-hover');
});$('.phpcn-button').mouseout(function(){
$(this).removeClass('phpcn-button-hover');
});
$('.tree').css('min-height',$(document).height()-160);
$('.tree').find('li').click(function(){
//alert($('.tree').find('dl').css('display'));
//$('.tree').find('dl').hide();
if($(this).find('dl').css('display')=='none'){
$(this).find('i').removeClass('phpcn-icon-down');
$(this).find('i').addClass('phpcn-icon-up');
$('.tree').find('dl').hide();
$(this).find('dl').show();
}else{
$(this).find('i').removeClass('phpcn-icon-up');
$(this).find('i').addClass('phpcn-icon-down');
$(this).find('dl').hide();
}
});
//table窗口
$('.tree').find('a').click(function(){
//alert($('.tree').find('dl').css('display'));
//$('.tree').find('dl').hide();
var tabs=$('.phpcn-tab-title a');
tree=$(this);
jQuery.each(tabs, function(){
$('.phpcn-tab-title li').removeClass('on');
if($(this).attr('data')!=tree.attr('data')){
var tabs=$('.phpcn-tab-title ul');
$(this).removeClass('on');
var html='<li class="on"><i></i><dd>'+tree.html()+' <a class="phpcn-icon phpcn-icon-guanbi" data='+tree.attr('data')+' href="javascript:;"></a></dd></li>';
tabs.append(html);
return false;
}
});
});
//table窗口
$('.phpcn-tab-title').find('.phpcn-icon-guanbi').click(function(){
alert();
$(this).parent().parent('li').remove();
});
$('.phpcn-tab-title').find('li').mouseover(function(){
var i=$(this).find('i');
$('.phpcn-tab-title').find('li').find('i').css('width','0%');
if($(this).css('background')!='#f6f6f6'){
$('.phpcn-tab-title').find('li').css('background','none');
$(this).css('background','#f6f6f6');
}
if(i.css('width')!='100%'){
i.css('width','100%');
}
});
</script>
</body>
</html>
<?php
include __DIR__ . "/head.php";
if (empty($_GET['id'])){
die('参数错误');
}
$a=$_GET['id'];
class shopImg extends select{
public $title;
public $img;
public function __construct()
{
// 调用父类方法链接数据库
$this->pdo= parent::conect(DB_HOST,DB_NAME,DB_USER,DB_PASSWORD);
}
public function selImg($a=1){
$a='`id`='.$a;
$sql = 'select `*` from `images` where '.$a;
$stmt = $this->pdo->prepare($sql);
$stmt->execute();
$article = $stmt->fetchAll(PDO::FETCH_ASSOC);
foreach ($article as $cate){
$this->title=$cate['titlename'];
$this->img=$cate['imageurl'];
}
}
}
$art=new shopImg();
$art->selImg($a);
?>
<!--顶部结束-->
<style>
.pi{
width: 100%;
height: 310px;
}
</style>
<div class="web-top phpcn-box-s phpcn-clear phpcn-mb-30">
<div class="phpcn-main">
<div class="phpcn-col-md7">
<span class="web-tit">php中文网</span>
<span class="web-name">图片站</span>
</div>
<div class="phpcn-col-md5">
<input type="" name=""> <i class="phpcn-icon phpcn-icon-sousuo2" placeholder='关键字搜索'></i>
</div>
</div>
</div>
<div class="phpcn-main ">
<div class="images">
<h1><?php echo $art->title;?></h1>
<div class="cont">
<div class="box">
<!-- 存放大图的容器-->
<div class="all">
<div class="top-img">
<div class="activeimg">
<?php echo ' <img src="static/images/'. $art->img.'">';?>
</div>
<div class="left"><img src="static/images/left.png"> </div>
<div class="right"><img src="static/images/right.png"></div>
</div>
<!-- 存放缩略图的容器-->
<div class="bot-img">
<ul>
<li class="active"><img src="static/images/1.jpg"> </li>
<li><img src="static/images/2.jpg"> </li>
<li><img src="static/images/3.jpg"> </li>
<li><img src="static/images/4.jpg"> </li>
</ul>
</div>
</div>
</div>
</div>
</div>
<!--评论--->
<div class="comment phpcn-mt-30 ">
<div class="title phpcn-mb-30"><span>网页评论</span></div>
<div class="phpcn-clear">
<div class="phpcn-col-md1"><img class="user" src="static/images/user.png"> </div>
<div class="phpcn-col-md11">
<textarea>我来评论两句</textarea>
<button class="phpcn-button phpcn-bg-red phpcn-button-hover phpcn-mt-10 phpcn-mb-10 phpcn-r">发表评论</button>
</div>
</div>
<div class="title phpcn-mb-30"><span>最新评论</span></div>
<div class="phpcn-clear">
<div class="phpcn-clear border">
<div class="phpcn-col-md1">
<img class="user" src="static/images/user.png">
</div>
<div class="phpcn-col-md11">
<ul class="phpcn-clear">
<li class="user-naem">
用户昵称
</li>
<li class="cont">留言内容</li>
<li>
<span>2019-08-08 14:46:05发表</span>
<span class="phpcn-r"><i class="phpcn-icon phpcn-icon-dianzan"></i> <a href="">回复 </a></span>
</li>
</ul>
</div>
</div>
<div class="phpcn-clear border">
<div class="phpcn-col-md1">
<img class="user" src="static/images/user.png">
</div>
<div class="phpcn-col-md11">
<ul class="phpcn-clear">
<li class="user-naem">
用户昵称
</li>
<li class="cont">留言内容</li>
<li>
<span>2019-08-08 14:46:05发表</span>
<span class="phpcn-r"><i class="phpcn-icon phpcn-icon-dianzan"></i> <a href="">回复 </a></span>
</li>
</ul>
</div>
</div>
<div class="phpcn-clear border">
<div class="phpcn-col-md1">
<img class="user" src="static/images/user.png">
</div>
<div class="phpcn-col-md11">
<ul class="phpcn-clear">
<li class="user-naem">
用户昵称
</li>
<li class="cont">留言内容</li>
<li>
<span>2019-08-08 14:46:05发表</span>
<span class="phpcn-r"><i class="phpcn-icon phpcn-icon-dianzan"></i> <a href="">回复 </a></span>
</li>
</ul>
</div>
</div>
</div>
<div class="phpcn-clear border">
<div class="phpcn-col-md1">
<img class="user" src="static/images/user.png">
</div>
<div class="phpcn-col-md11">
<ul class="phpcn-clear">
<li class="user-naem">
用户昵称
</li>
<li class="cont">留言内容</li>
<li>
<span>2019-08-08 14:46:05发表</span>
<span class="phpcn-r"><i class="phpcn-icon phpcn-icon-dianzan"></i> <a href="">回复 </a></span>
</li>
</ul>
</div>
</div>
</div>
<!--评论结束--->
</div>
<!--网站底部-->
<script src="static/js/pin.js"></script>
<script>
$(function(){
$('.bot-img ul li').click(function(){
var _this=$(this);
_this.addClass('active').siblings('li').removeClass('active');
var int=_this.index();
$('.activeimg').animate({left:int*-500},"slow");
});
var list=$('.bot-img ul li').length;
$('.activeimg').css({
width:list*500,
});
$('.right').click(function(){
next(list)
})
$('.left').click(function(){
prev(list)
});
//自动播放 2秒播放一次 无限循环
var timer='';
var num=0;
timer=setInterval(function(){ //打开定时器
num++;
if(num>parseFloat(list)-1){
num=0;
$('.activeimg').animate({left:num*-500},"slow");
}else{
$('.activeimg').animate({left:num*-500},"slow");
}
},2000);
})
var index=0;
//下一张
function next(list){
if(index<list-1){
index++;
$('.activeimg').animate({left:index*-500},"slow");
$('.bot-img ul li').eq(index).addClass('active').siblings('li').removeClass('active')
}else{
index=0;
$('.activeimg').animate({left:index*-522},"slow");
$('.bot-img ul li').eq(index).addClass('active').siblings('li').removeClass('active')
}
}
// 上一张
function prev(list){
index--;
if(index<0){
index=list-1;
$('.activeimg').animate({left:index*-500},"slow");
$('.bot-img ul li').eq(index).addClass('active').siblings('li').removeClass('active')
}else{
$('.activeimg').animate({left:index*-500},"slow");
$('.bot-img ul li').eq(index).addClass('active').siblings('li').removeClass('active')
}
}
var myPi = new Pike(".pi", {
type: 1, // 轮播的类型(1渐隐)
automatic: true, //是否自动轮播 (默认false)
autoplay: 2000, //自动轮播毫秒 (默认3000)
hover: true, //鼠标悬停轮播 (默认false)
arrowColor: "yellow", //箭头颜色 (默认绿色)
arrowBackgroundType: 2, //箭头背景类型 (1: 方形, 2:圆形)
arrowBackground: 1, //箭头背景色 (1:白色,2:黑色, 默认:无颜色)
arrowTransparent: 0.2, //箭头背景透明度 (默认: 0.5)
spotColor: "white",//圆点颜色 (默认: 白色)
spotType: 1, //圆点的形状 (默认: 圆形, 1:圆形, 2.矩形)
spotSelectColor: "red", //圆点选中颜色 (默认绿色)
spotTransparent: 0.8, //圆点透明度 (默认0.8)
mousewheel: true, //是否开启鼠标滚动轮播(默认false)
drag: false, //是否开启鼠标拖动 (默认为: true, 如不需要拖动设置false即可)
loop: true, //是否循环轮播 (默认为: false)
});
</script>
</body>
</html>
<?php
include __DIR__ . "/head.php";
?>
<!--新闻内容头部分类-->
<div class="ar-head phpcn-main">
<div class='phpcn-col-md10 path'>
<a href=""><img src="static/images/ar-logo.png"></a>
<a href="">财经</a>
<span>></span>
<span>列表</span>
</div>
<div class='phpcn-col-md2'>
<input type="" name=""> <i class="phpcn-icon phpcn-icon-sousuo2" placeholder='关键字搜索'></i>
</div>
<div class="phpcn-clear">
<div class="phpcn-col-md9">
<div class="article-content">
<!--列表开始-->
<div class="alist">
<div class="nav phpcn-mt-20">
<a href="">头条 </a>
<a href="">热文 </a>
<a href="">直播 </a>
<a href="" class="on">新闻 </a>
<a href="">政策地图 </a>
<a href="">相对论 </a>
<a href="">人物 </a>
<a href="">行情 </a>
<a href="">投研</a>
<a href="">技术</a>
<a href="">百科</a>
</div>
<div class="aritcle-list">
<!-- 文章资讯页列表-->
<?php $selHead->listSel(); ?>
</div>
</div>
<!--列表开始结束-->
<!--推荐阅读 --->
<div class="about-read phpcn-mt-30 phpcn-clear">
<div class="title"><span>推荐阅读</span></div>
<ul>
<!--推荐阅读-->
<?php $selHead->reading(6); ?>
</ul>
</div>
<!--推荐阅读 结束--->
</div>
</div>
<div class="phpcn-col-md3">
<div class="hot-article">
<div class="title"><span>网页评论</span></div>
<ul>
<!-- 文章右侧内容-->
<?php $selHead->comments(6) ?>
</ul>
</div>
<div class="hot-article phpcn-mt-30">
<div class="title"><span>网页评论</span></div>
<ul>
<!-- 文章右侧内容-->
<?php $selHead->comments(6) ?>
</ul>
</div>
</div>
</div>
</div>
<!--新闻内容头部分类结束-->
<!--网站底部-->
<?php include __DIR__ . '/foot.php'; ?>
<!--网站底部-->
<script src="static/js/pin.js"></script>
<script>
var myPi = new Pike(".pi", {
type: 1, // 轮播的类型(1渐隐)
automatic: true, //是否自动轮播 (默认false)
autoplay: 2000, //自动轮播毫秒 (默认3000)
hover: true, //鼠标悬停轮播 (默认false)
arrowColor: "yellow", //箭头颜色 (默认绿色)
arrowBackgroundType: 2, //箭头背景类型 (1: 方形, 2:圆形)
arrowBackground: 1, //箭头背景色 (1:白色,2:黑色, 默认:无颜色)
arrowTransparent: 0.2, //箭头背景透明度 (默认: 0.5)
spotColor: "white",//圆点颜色 (默认: 白色)
spotType: 1, //圆点的形状 (默认: 圆形, 1:圆形, 2.矩形)
spotSelectColor: "red", //圆点选中颜色 (默认绿色)
spotTransparent: 0.8, //圆点透明度 (默认0.8)
mousewheel: true, //是否开启鼠标滚动轮播(默认false)
drag: false, //是否开启鼠标拖动 (默认为: true, 如不需要拖动设置false即可)
loop: true, //是否循环轮播 (默认为: false)
});
var progress = $('.phpcn-progress .phpcn-row');
jQuery.each(progress, function(){
//console.log($(this).find('.phpcn-progress-percent').attr('class'));
$(this).find('.phpcn-progress-bor').css('width',$(this).find('.phpcn-progress-percent').html());
});
$('.phpcn-dl dl').last().css('border-bottom','1px solid #C9C9C9');
$('#admin-select').mouseover(function(){
//alert($(this).find('dl').css('display'));
$(this).find('dl').show();
});
$('#admin-select').find('dl').mouseout(function(){
$(this).hide();
});
$('.phpcn-button').mouseover(function(){
$(this).addClass('phpcn-button-hover');
});$('.phpcn-button').mouseout(function(){
$(this).removeClass('phpcn-button-hover');
});
$('.tree').css('min-height',$(document).height()-160);
$('.tree').find('li').click(function(){
//alert($('.tree').find('dl').css('display'));
//$('.tree').find('dl').hide();
if($(this).find('dl').css('display')=='none'){
$(this).find('i').removeClass('phpcn-icon-down');
$(this).find('i').addClass('phpcn-icon-up');
$('.tree').find('dl').hide();
$(this).find('dl').show();
}else{
$(this).find('i').removeClass('phpcn-icon-up');
$(this).find('i').addClass('phpcn-icon-down');
$(this).find('dl').hide();
}
});
//table窗口
$('.tree').find('a').click(function(){
//alert($('.tree').find('dl').css('display'));
//$('.tree').find('dl').hide();
var tabs=$('.phpcn-tab-title a');
tree=$(this);
jQuery.each(tabs, function(){
$('.phpcn-tab-title li').removeClass('on');
if($(this).attr('data')!=tree.attr('data')){
var tabs=$('.phpcn-tab-title ul');
$(this).removeClass('on');
var html='<li class="on"><i></i><dd>'+tree.html()+' <a class="phpcn-icon phpcn-icon-guanbi" data='+tree.attr('data')+' href="javascript:;"></a></dd></li>';
tabs.append(html);
return false;
}
});
});
//table窗口
$('.phpcn-tab-title').find('.phpcn-icon-guanbi').click(function(){
alert();
$(this).parent().parent('li').remove();
});
$('.phpcn-tab-title').find('li').mouseover(function(){
var i=$(this).find('i');
$('.phpcn-tab-title').find('li').find('i').css('width','0%');
if($(this).css('background')!='#f6f6f6'){
$('.phpcn-tab-title').find('li').css('background','none');
$(this).css('background','#f6f6f6');
}
if(i.css('width')!='100%'){
i.css('width','100%');
}
});
</script>
</body>
</html>
<?php
include __DIR__ . "/head.php";
if (empty($_GET['id'])){
die('参数错误');
}
$a=$_GET['id'];
//文章内容页
class article extends select{
public $title;
public $time;
public $read;
public $body;
public function __construct()
{
// 调用父类方法链接数据库
$this->pdo= parent::conect(DB_HOST,DB_NAME,DB_USER,DB_PASSWORD);
}
public function article($a=1){
$a='`id`='.$a;
$sql = 'select `*` from `article` where '.$a;
$stmt = $this->pdo->prepare($sql);
$stmt->execute();
$article = $stmt->fetchAll(PDO::FETCH_ASSOC);
// 得到文章的基本信息本赋值给相应变量,在内容中直接访问变量就行
foreach ($article as $cate){
$this->title=$cate['title'];
$this->time=$cate['times'];
$this->read=$cate['click'];
$this->body=$cate['body'];
}
}
}
$art=new article();
$art->article($a);
?>
<!--新闻内容头部分类-->
<div class="ar-head phpcn-main">
<div class='phpcn-col-md10 path'>
<a href=""><img src="static/images/ar-logo.png"></a>
<a href="">财经</a>
<span>></span>
<span>正文</span>
</div>
<div class='phpcn-col-md2'>
<input type="" name=""> <i class="phpcn-icon phpcn-icon-sousuo2" placeholder='关键字搜索'></i>
</div>
<div class="phpcn-clear">
<div class="phpcn-col-md9">
<div class="article-content">
<!-- 标题-->
<h1> <?php echo $art->title; ?></h1>
<div class="attribute">
<span>发布时间:<?php echo $art->time;?></span>
<span>来源:北京 青年报</span>
<span>阅读量:<?php echo $art->read;?></span>
<span>评论数:1545</span>
</div>
<article >
<!-- 内容-->
<?php echo $art->body; ?>
</article>
<div class="suggest">
<button class="phpcn-button phpcn-bg-red phpcn-button-hover">赞</button>
<button class="phpcn-button phpcn-color-grayphpcn-button-hover">踩</button>
</div>
<!--评论--->
<div class="comment phpcn-mt-30">
<div class="title phpcn-mb-30"><span>网页评论</span></div>
<div class="phpcn-clear">
<div class="phpcn-col-md1"><img class="user" src="static/images/user.png"> </div>
<div class="phpcn-col-md11">
<textarea>
我来评论两句
</textarea>
<button class="phpcn-button phpcn-bg-red phpcn-button-hover phpcn-mt-10 phpcn-mb-10 phpcn-r">发表评论</button>
</div>
</div>
</div>
<!--评论结束--->
<!--推荐阅读 --->
<div class="about-read phpcn-mt-30">
<div class="title"><span>推荐阅读</span></div>
<ul>
<?php $selHead->reading(6); ?>
</ul>
</div>
<!--推荐阅读 结束--->
</div>
</div>
<div class="phpcn-col-md3">
<div class="hot-article">
<div class="title"><span>网页评论</span></div>
<ul>
<?php $selHead->comments(6) ?>
</ul>
</div>
<div class="hot-article phpcn-mt-30">
<div class="title"><span>网页评论</span></div>
<ul>
<?php $selHead->comments(6) ?>
</ul>
</div>
</div>
</div>
</div>
<!--新闻内容头部分类结束-->
<!--网站底部-->
<?php include __DIR__ . '/foot.php'; ?>
<!--网站底部-->
<script src="static/js/pin.js"></script>
<script>
var myPi = new Pike(".pi", {
type: 1, // 轮播的类型(1渐隐)
automatic: true, //是否自动轮播 (默认false)
autoplay: 2000, //自动轮播毫秒 (默认3000)
hover: true, //鼠标悬停轮播 (默认false)
arrowColor: "yellow", //箭头颜色 (默认绿色)
arrowBackgroundType: 2, //箭头背景类型 (1: 方形, 2:圆形)
arrowBackground: 1, //箭头背景色 (1:白色,2:黑色, 默认:无颜色)
arrowTransparent: 0.2, //箭头背景透明度 (默认: 0.5)
spotColor: "white",//圆点颜色 (默认: 白色)
spotType: 1, //圆点的形状 (默认: 圆形, 1:圆形, 2.矩形)
spotSelectColor: "red", //圆点选中颜色 (默认绿色)
spotTransparent: 0.8, //圆点透明度 (默认0.8)
mousewheel: true, //是否开启鼠标滚动轮播(默认false)
drag: false, //是否开启鼠标拖动 (默认为: true, 如不需要拖动设置false即可)
loop: true, //是否循环轮播 (默认为: false)
});
var progress = $('.phpcn-progress .phpcn-row');
jQuery.each(progress, function(){
//console.log($(this).find('.phpcn-progress-percent').attr('class'));
$(this).find('.phpcn-progress-bor').css('width',$(this).find('.phpcn-progress-percent').html());
});
$('.phpcn-dl dl').last().css('border-bottom','1px solid #C9C9C9');
$('#admin-select').mouseover(function(){
//alert($(this).find('dl').css('display'));
$(this).find('dl').show();
});
$('#admin-select').find('dl').mouseout(function(){
$(this).hide();
});
$('.phpcn-button').mouseover(function(){
$(this).addClass('phpcn-button-hover');
});$('.phpcn-button').mouseout(function(){
$(this).removeClass('phpcn-button-hover');
});
$('.tree').css('min-height',$(document).height()-160);
$('.tree').find('li').click(function(){
//alert($('.tree').find('dl').css('display'));
//$('.tree').find('dl').hide();
if($(this).find('dl').css('display')=='none'){
$(this).find('i').removeClass('phpcn-icon-down');
$(this).find('i').addClass('phpcn-icon-up');
$('.tree').find('dl').hide();
$(this).find('dl').show();
}else{
$(this).find('i').removeClass('phpcn-icon-up');
$(this).find('i').addClass('phpcn-icon-down');
$(this).find('dl').hide();
}
});
//table窗口
$('.tree').find('a').click(function(){
//alert($('.tree').find('dl').css('display'));
//$('.tree').find('dl').hide();
var tabs=$('.phpcn-tab-title a');
tree=$(this);
jQuery.each(tabs, function(){
$('.phpcn-tab-title li').removeClass('on');
if($(this).attr('data')!=tree.attr('data')){
var tabs=$('.phpcn-tab-title ul');
$(this).removeClass('on');
var html='<li class="on"><i></i><dd>'+tree.html()+' <a class="phpcn-icon phpcn-icon-guanbi" data='+tree.attr('data')+' href="javascript:;"></a></dd></li>';
tabs.append(html);
return false;
}
});
});
//table窗口
$('.phpcn-tab-title').find('.phpcn-icon-guanbi').click(function(){
alert();
$(this).parent().parent('li').remove();
});
$('.phpcn-tab-title').find('li').mouseover(function(){
var i=$(this).find('i');
$('.phpcn-tab-title').find('li').find('i').css('width','0%');
if($(this).css('background')!='#f6f6f6'){
$('.phpcn-tab-title').find('li').css('background','none');
$(this).css('background','#f6f6f6');
}
if(i.css('width')!='100%'){
i.css('width','100%');
}
});
</script>
</body>
</html>