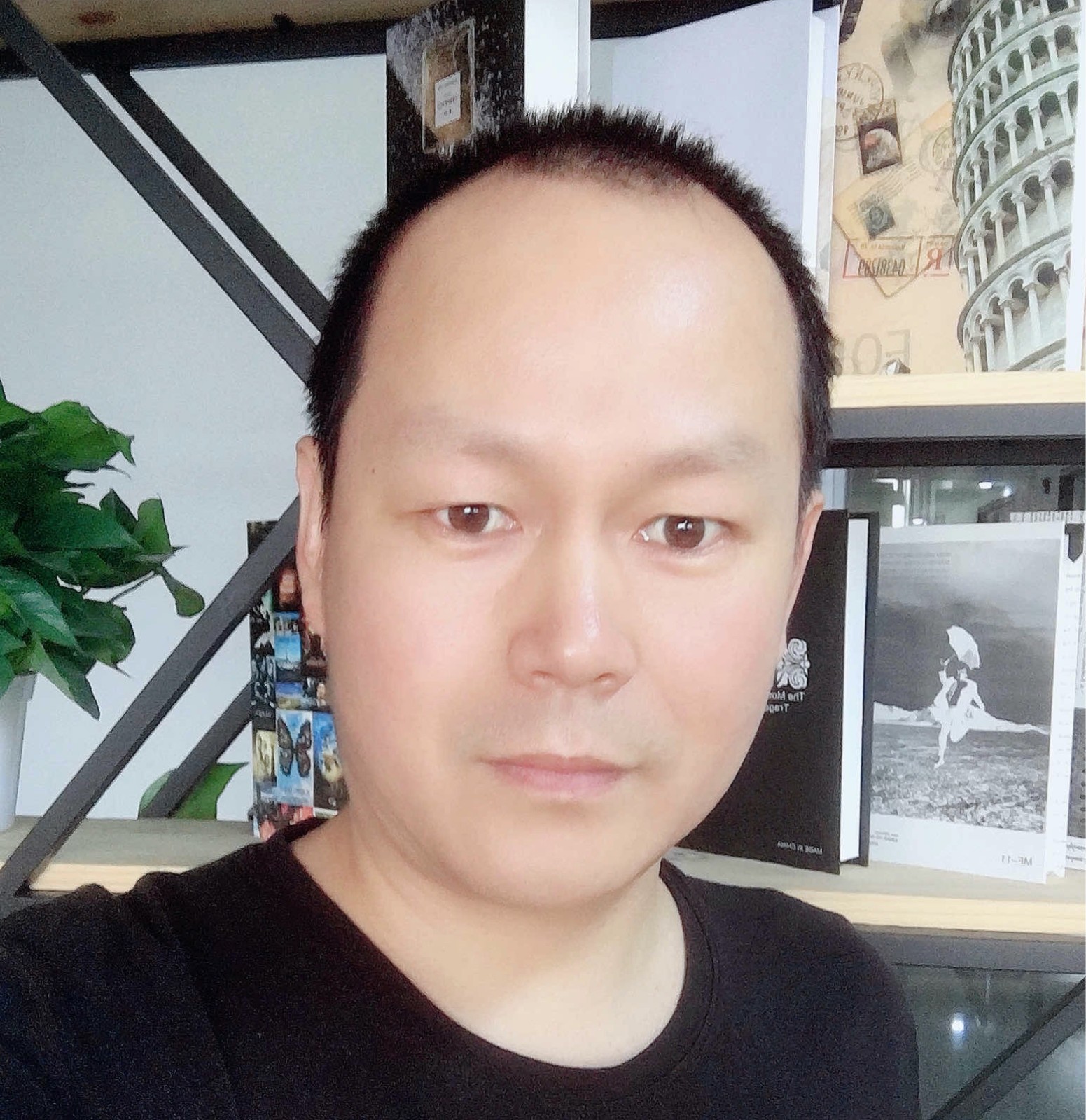
Correction status:qualified
Teacher's comments:完成的很好
// 流程控制之循环
$arr1 = ['html', 'css', 'js', 'php', 'laraval'];
// 几个使用函数
// count() 计算数组元素的数量
echo '数组的数量是:'. count($arr1). '<br>';
//strlen() 计算字符串长度
echo '字符串的长度是:'. strlen('php.cn'). '<br>';
//trim(), rtrim(), ltrim() 是从字符串两边 右边 左边 删除指定的字符,默认删除的是空格
$str = ' php.cn ';
echo '字符串原始长度是:'. strlen($str) . '<br>';
$str = trim($str);
echo '字符串当前长度是:'. strlen($str) . '<br>';
// mt_rand(min, max) 产生指定范围的随机数
$randcolor = 'rgb('. mt_rand(0,255). ','. mt_rand(0,255). ','. mt_rand(0,255). ')';
echo $randcolor. '<br>';
echo '<h3 style="color:'.$randcolor.'";>自学<h3>';
//for() 计数循环
// for(循环变量的初始化,循环条件,更新循环条件)
$result = '';
for ($i=0; $i < count($arr1); $i++) {
// $result = '<span style="color:'.$randcolor.'">'. $result. $arr1[$i]. '</span>'. ',';
$result = $result. $arr1[$i];
}
echo rtrim($result,','). '<br>';
// while() : 根据循环条件,只要条件满足 就一直执行循环体中的语句
// while() : 根据循环条件的位置,分为两种,入口判断,出口判断
// 循环变量必须写在while外部
echo '<hr>';
//入口判断
$i = 0;
$result ='';
while ($i < count($arr1)) {
$result = '<span style="color:'.$randcolor.'">'. $result. $arr1[$i]. '</span>'. ',';
$i++;
}
echo rtrim($result,','). '<br>';
// 出口判断
$i = 0;
$result ='';
do {
$result = '<span style="color:'.$randcolor.'">'. $result. $arr1[$i]. '</span>'. ',';
$i++;
}while ($i > count($arr1));
echo rtrim($result,','). '<br>';
// ======================================================
echo '<hr>';
$arr2 = ['id'=>1002, 'name'=>'小明', 'pirce'=>222];
foreach ($arr2 as $key => $value) {
echo $key . '='. $value. '<br>';
}
echo '<hr>';
// for 遍历 关联数组
// key() current() 分别返回当前元素的键和值
// next() 将指针只想数组当前元素的下一个元素位置 reset()进行指针复位
for ($i=0; $i < count($arr2); $i++) {
echo key($arr2). '===>'. current($arr2). '<br>';
next($arr2);
}
// while () 遍历 关联数组
reset($arr2);
$i = 0;
while ($i < count($arr2)) {
echo key($arr2). '===>'. current($arr2). '<br>';
next($arr2);
$i++;
}
// 循环表单的应用
$months = range(1,12);
$select = '月份: <select name="months">';
foreach ($months as $month) {
$select = $select .'<option value="'.$month.'">' .$month .'月'.'</option>';
}
$select = $select.'</select>';
echo $select;
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>用户注册</title>
<style type="text/css">
.item{
width: 800px;
height: 500px;
margin: auto;
}
.item > h3{
display: flex;
justify-content: center;
color:red;
border-bottom:1px red solid;
box-sizing: border-box;
}
.item > form{
display: flex;
flex-direction: column;
align-items: center;
border: 1px #8888 solid;
background-color: #d9d9d9;
}
.item > form > span{
margin-top: 8px;
}
.item > form > span:hover{
background-color: blank;
}
.item > form > span > button{
border: none;
background-color: #fff;
width: 80px;
height: 30px;
}
</style>
</head>
<body>
<div class="item">
<h3>用户注册</h3>
<form action="demo3.php" method="post">
<span>
<label for="username">用户名</label>
<input type="text" name="username" id="username" required>
</span>
<span>
<label for="password1">密码</label>
<input type="password" name="password1" id="password1" required>
</span>
<span>
<label for="password2">重复密码</label>
<input type="password" name="password2" id="password2" required>
</span>
<span>
<label for="email">邮箱</label>
<input type="email" name="email" id="email">
</span>
<span>
<label for="passwor">性别</label>
<input type="radio" name="gender" value="male" id="male" required>
<label for="male">男</label>
<input type="radio" name="gender" value="female" id="female" required>
<label for="female">女</label>
</span>
<span>
<button>提交注册</button>
</span>
</form>
</div>
</body>
</html>
// 超全局变量:
// 1是指每一个php程序(就是一个动态的php页面)都已经存在的变量,不需要用户区主动创建
// 2它的值,是由系统根据每个php程序自动设置初始值,大部分反应程序状态
// 3它的值,大多是允许用户更新,但不能删除它,否则会引起致命错误。
// 4 他没有作用域显示,无论是全局,还是函数中,都直接用,不需要声明
// $_REQUEST 请求数据的超全局变量,里面保存的是用户所有的请求数据
// echo '<pre>'. print_r($_REQUEST ,true). '</pre>';
// echo '<pre>'. print_r($_POST ,true). '</pre>';
// echo '用户名:'. $_POST['username'] . '<br>';
// 判断用户的请求类型是否合法
// echo '请求类型是:'. $_SERVER['REQUEST_METHOD'] . '<br>';
if ($_SERVER['REQUEST_METHOD'] === 'POST') {
//检查请求变量是否设置,并且不能为NULL,isset()
// if (isset($_POST['username'])) {
// echo '有';
// }else{
// echo '无';
// }
//empty() 空字符串, 0 NULL false
// if (!empty($_POST['username'])) {
// echo '有';
// }else{
// echo '无';
// }
if (!empty($_POST['username'])) $username = $_POST['username'];
if (!empty($_POST['password1'])) $password1 = $_POST['password1'];
if (!empty($_POST['password2'])) $password2 = $_POST['password2'];
if (!empty($_POST['email'])) $email = $_POST['email'];
if (!empty($_POST['gender'])) $gender = $_POST['gender'];
//两次密码必须一致
if ($password1 === $password2) {
$password = $password1;
}else{
exit('<script>alert("两次密码不一致");history.back();</script>');
}
$data = compact('username', 'password', 'email', 'gender');
echo '<per>'. print_r($data, true). '</per>';
}else{
exit('<h3 style="color:red">请求类型错误</h3>');
}