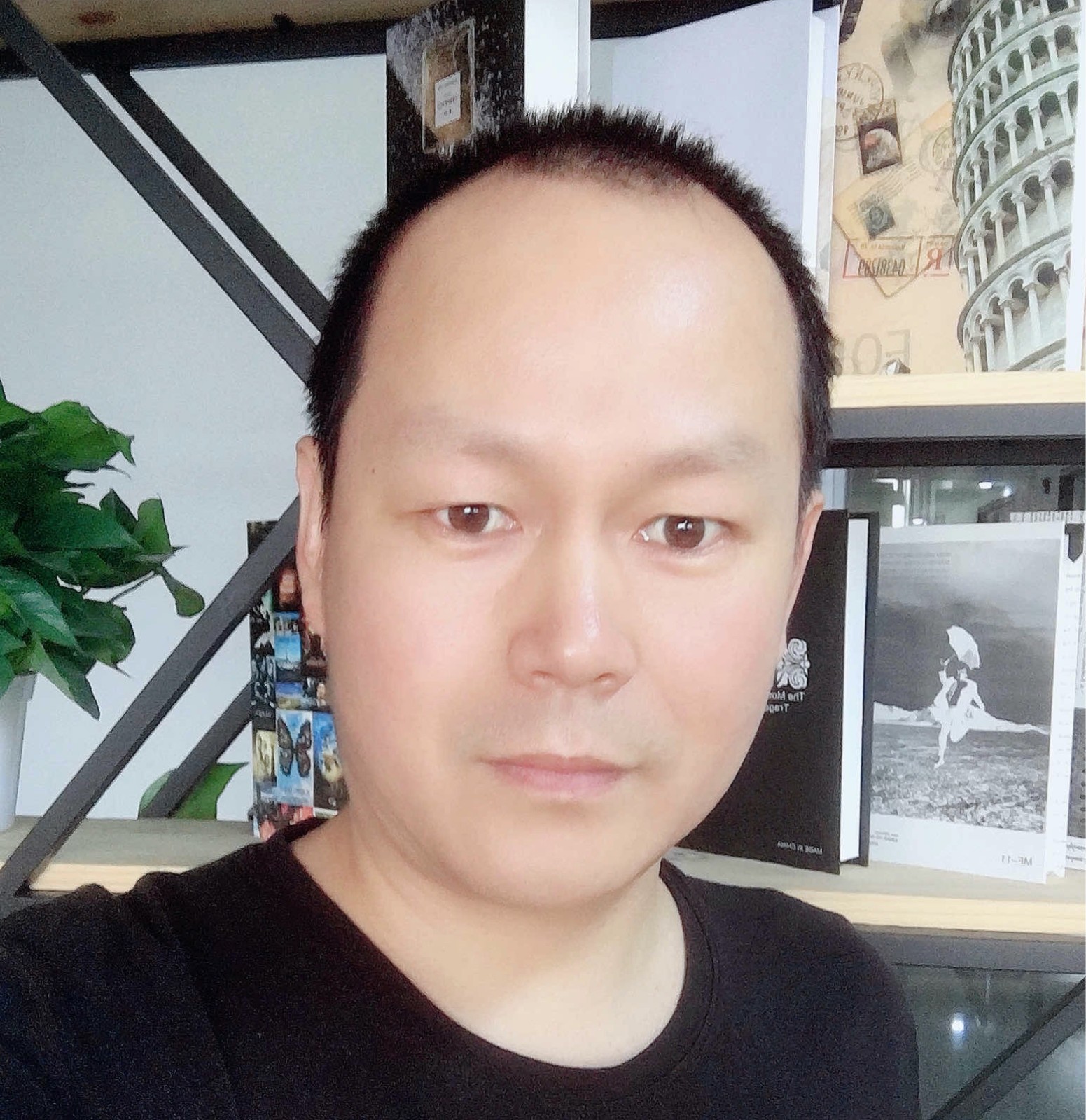
Correction status:qualified
Teacher's comments:希望能在最后的一节课还能见到你, 继续努力,坚持到底
常量简单的说就是开发过程中需要的一个固定的不轻易去改变它的一个值,去创建这样一个值就叫常量(目前我的理解),常量名不需要使用“$”符号,但是命名必须遵循PHP命名规范,推荐使用大写,以方便和变量进行区别。
常量可以用函数define("常量名","常量值")
或者用关键字const
创建。
define()创建:
<?php
$country = '中国' ;
define("WLC" , $country . "欢迎您");
效果图
const创建:
<?php
const A = '努力学习';
echo A ;
效果图
从上面的例子可以看出define
和const
创建常量是有区别的,define
可以用表达式创建常量,const
只能用字面量
单引号不会解析转义符、变量。
例
<?php
$country = '中国';
echo '我出生在\t . $country';
图
上面的特殊字符与变量名并没有被解析出来,只会按照字面量进行输出。
双引号
<?php
$country = '中国';
echo "我出生在\t$country";
图
上面的特殊字符与变量名被解析出来
数组就是把多个元素(可以理解为变量的值)放在一个数组(可以理解为变量)里面,由多个变量值组成的合集。
索引数组:存放元素的房间号为数值的是索引数组。
<?php
$arr = [10,20,30];
echo '<pre>', print_r($arr,true) ,'<pre>';
echo '<hr>';
echo $arr[0];
图1
图2
多个元素统一创建
<?php
$arr = [10,20,30];
echo '<pre>', print_r($arr,true) ,'<pre>';
echo '<hr>';
逐个创建
<?php
$arr2[] = 30;
$arr2[] = 40;
$arr2[] = 50;
echo '<pre>', print_r($arr2,true) ,'<pre>';
从效果图可以看出,创建方式对最终效果没有影响
关联数组:存放元素房间号,有具体语义化定义的为关联数组
<?php
$arr2 = ['id'=>'101' , 'name'=>'张飞' , 'force'=>90];
echo '<pre>', print_r($arr2,true) ,'<pre>';
echo '<hr>';
echo $arr2['name'];
图1
图2
关联数组与索引数组一样分为统一创建,与逐个创建
<?php
// 统一创建
$arr2 = ['id'=>'101' , 'name'=>'张飞' , 'force'=>90];
echo '<pre>', print_r($arr2,true) ,'<pre>';
echo '<hr>';
// 逐个创建
$arr3['id']='102';
$arr3['name']='关羽';
$arr3['force']=95;
echo '<pre>', print_r($arr3,true) ,'<pre>';
图
多维数组:可以理解为数组的嵌套,一般嵌套两层(索引数组里面嵌套关联数组),太多了会混乱。
<?php
// 创建二维数组
$arr4 = [
['id'=>'101' , 'name'=>'张飞' , 'force'=>90],
['id'=>'102' , 'name'=>'关羽' , 'force'=>95],
];
echo '<pre>', print_r($arr4,true) ,'<pre>';
echo '<hr>';
// 访问0号房间(索引数组)里面的元素(关联数组)中‘name’房间号里面的具体元素。
echo $arr4[0]['name'] , '<br>';
// 访问1号房间(索引数组)里面的元素(关联数组)中‘name’房间号里面的具体元素。
echo $arr4[1]['name'] , '<br>';
图1
图2
implode
函数。格式为‘接收转换后的变量名’ = implode(‘分隔符’,需要转换的数组变量名)。
例
<?php
$arr = [10,20,30];
// 把数组转为字符串
$str = implode('+' , $arr);
// 获取转换后的变量类型
echo '转换后的类型为:'. gettype($str);
// 转换后的变量输出结果
echo '<br>';
echo '转换后str的值为:'. $str ;
图
explode
函数实现。格式为‘接收转换后的变量名’ = explode(‘分隔符必须和字符串使用的分隔符相同’,需要转换的字符串变量名)。
例
<?php
$arr = [10,20,30];
// 把数组转为字符串
$str = implode('+' , $arr);
// 获取转换后的变量类型
echo '转换后的类型为:'. gettype($str);
// 转换后的变量输出结果
echo '<br>';
echo '转换后str的值为:'. $str .'<hr>';
// 把字符串转为数组,分隔符必须和字符串使用的分隔符相同
$arr1 = explode('+',$str);
// 获取转换后的变量类型
echo '转换后的类型为:'. gettype($arr1);
// 转换后的变量输出结果
echo '<br>';
echo '<pre>', print_r($arr1,true) ,'<pre>';
图
extract
把关联数组元素转换为变量格式为:extract(需要转换的数组名)
<?php
$animal = ['D'=>'Dog', 'C'=>'Cat','H'=>'Horse'];
// 把关联数组$animal里面的元素转为变量,其房间号就为变量名,里面具体元素值为变量值
extract($animal);
// 输出转为变量后的值
echo "\$D的值为$D<br>\$C的值为$C<br>\$H的值为$H";
图1
图2
compact
把多个变量转为关联数组格式为:接收转换后的关联数组名称 = compact('需要转换的变量名不需要加$符号','需要转换的变量名不需要加$符号')
<?php
$R = 'Rabbit' ; $L = 'Lion' ; $T = 'Tiger' ;
// 把对应变量转为关联数组
$animal2 = compact('R','L' ,'T');
echo $R.'<br>'.$L.'<br>'.$T;
echo '<pre>', print_r($animal2,true) ,'<pre>';
图1
图2
好啦,关于今天的知识就学习到这里了,下次提交关于流程控制与循环的知识。
为了生活最近太忙了,好不容易有时间可以补一下课程和作业,选择了就不要放弃,再累都要努力。