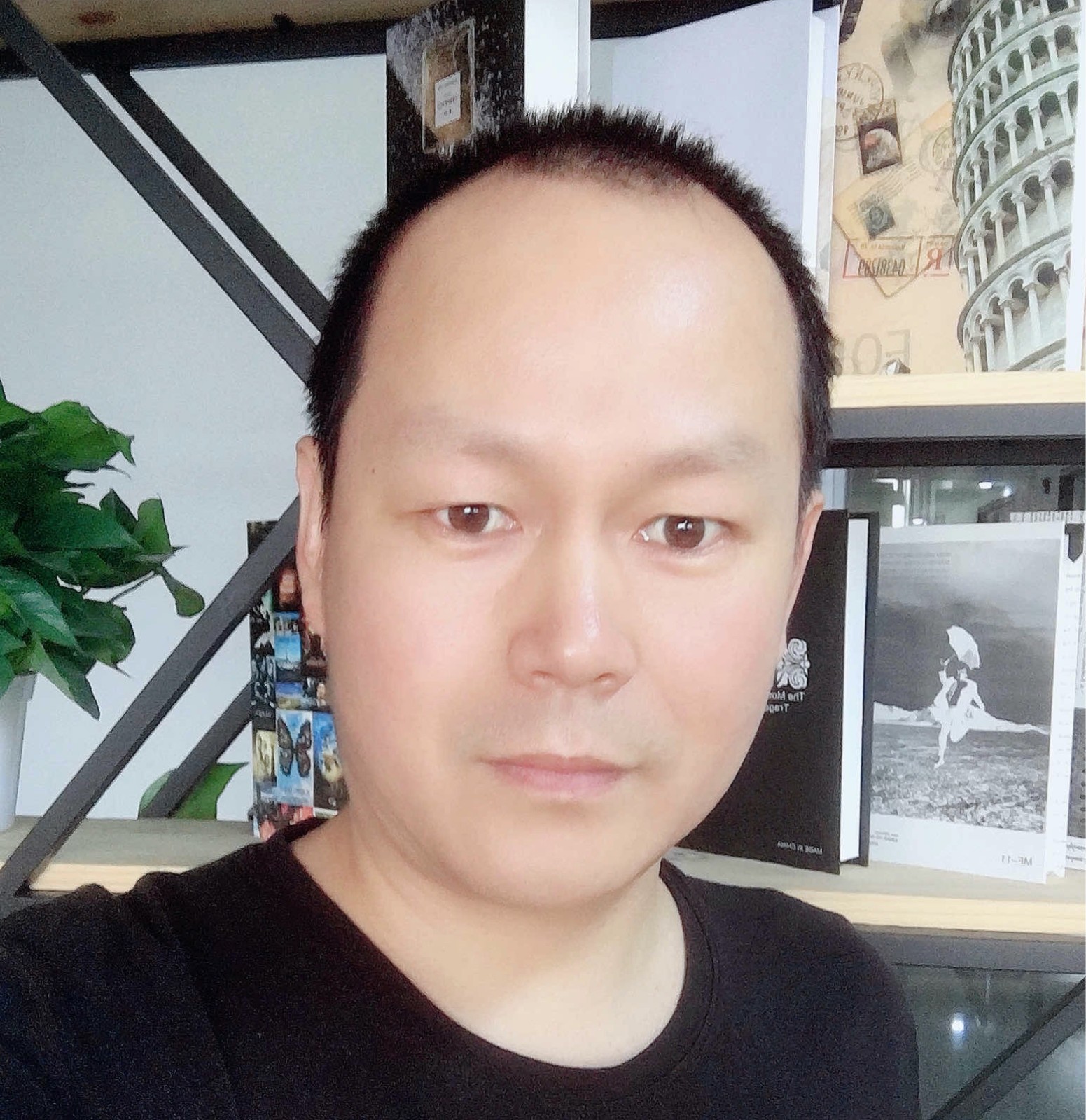
Correction status:qualified
Teacher's comments:如果欠的作业过多, 应该以当下为主, 否则二头都顾上不... 没有作业, 不必对我说抱歉, 学习在自己的事, 自己不上心, 没有帮得了你...
抱歉,朱老师,作业交的比较迟,本来想把大作业做了一起交,还是先把之前的作业交了!(#^.^#)
for与while循环之索引数组和关联数组的遍历
<?php
//for()循环遍历所以索引数组
$arr1 = ['吃饭','睡觉','打豆豆'];
$result = ' ' ;
for ($i = 0 ; $i < count($arr1) ; $i++ ) {
$result .= $arr1[$i] . '<br>';
}
echo $result. '<br>';
//die();
//while()循环遍历所以索引数组
//入口判断型
$i = 0 ;
$result = ' ' ;
while ($i < count($arr1) ) {
$result .= $arr1[$i] . '<br>';
$i++;
}
echo $result;
//出口判断型
$i = 0 ;
$result = ' ' ;
do{
$result .= $arr1[$i];
$i++;
}while( $i > count($arr1));
echo $result . '<br>';
echo '<hr>';
// for()循环遍历关联数组
$arr2 = [ 'id' =>111,'name'=>'小江','height'=>'180cm'];
$result = ' ' ;
reset($arr2);
for ($i = 0 ; $i < count($arr2) ; $i++ ) {
echo key($arr2).'=>'. current($arr2).'<br>';
next($arr2);
}
echo '<br>';
//while ()遍历关联数组
reset($arr2);
$i = 0 ;
while (current($arr2)){
echo key($arr2).'=>'. current($arr2);
next($arr2);
}
//foreach ()遍历数组
foreach ($arr2 as $key => $current) {
echo "$key => $current" . '<br>';
}
-在遍历关联数组的时候,一定要记住以下几个函数:
-1,reset($array)
:重置数组指针,指向第一个键
-2,next($array)
:将数组指针向后移一个
-3,prev($array)
:将数组指针向前移一个
-4,end($array)
:将数组指针移向末尾
-while循环的细节注意:
//初始条件在while外面
$i = 0 ;
$result = ' ' ;
while ($i < count($arr1) ) {
$result .= $arr1[$i] . '<br>';
//循环更新条件必须在这个位置
$i++;
}
echo $result;
源代码——HTML
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>注册表单</title>
<style>
* {
padding: 0;
margin: 0;
/*outline: 1px solid red;*/
}
body {
width: 1200px;
margin: auto;
display: flex;
/*align-content: center;*/
}
.login {
height: 300px;
width: 300px;
margin: 100px auto 0;
}
.login > h2 {
text-align: center;
}
.login > .form {
height: 100%;
display: flex;
flex-grow: 1;
flex-flow: column nowrap;
justify-content: space-evenly;
/*align-: space-evenly;*/
background-color: lightskyblue;
}
.login > .form {
border-radius: 10px;
}
.login > .form:hover {
box-shadow: 0 0 3px #333333;
}
</style>
</head>
<body>
<div class="login">
<h2>用户注册</h2>
<form action="demo2.php" method="post" class="form">
<section class="name">
<label for="name">姓名:</label>
<input type="text" name="name" id="name" placeholder="请输入您的真实姓名">
</section>
<section class="cellphone">
<label for="cellphone">电话:</label>
<input type="text" name="cellphone" id="cellphone" placeholder="请输入您的电话号码">
</section>
<section class="sex">
<label for="male">性别:</label>
<input type="radio" name="sex" id="male" value="male" checked><label for="male">男</label>
<input type="radio" name="sex" id="female" value="female"><label for="female">女</label>
</section>
<section>
爱好: <input type="checkbox" name="hobby" id="feed" value="feed"><label for="feed">吃饭</label>
<input type="checkbox" name="hobby" id="sleep" value="sleep" checked><label for="sleep">睡觉</label>
<input type="checkbox" name="" id="hobby" value="fight"><label for="fight">打豆豆</label><br>
</section>
<section>
<button>提交</button>
<button type="reset">重置</button>
</section>
</form>
</div>
</body>
</html>
<?php
//超全局变量
//$_REQUEST,里面保存的是用户所以的请求类型
//echo '<pre>' . print_r($_REQUEST,true) . '</pre>';
//判断用户的请求类型是否合法
//echo '请求类型:' . $_SERVER['REQUEST_METHOD'] . '<br>';
if ($_SERVER['REQUEST_METHOD'] === 'POST') {
// 判断请求变量是否设置,并且值不能为NULL
// empty()空字符串,0,NULL,false
if (!empty($_POST['name'])) $name = $_POST['name'];
if (!empty($_POST['cellphone'])) $cellphone = $_POST['cellphone'];
if (!empty($_POST['sex'])) $sex = $_POST['sex'];
if (!empty($_POST['hobby'])) $hobby = $_POST['hobby'];
// 将以上信息封装到字符串中
$login = compact('name' , 'cellphone' , 'sex' , 'hobby' );
echo '<pre>' . print_r($login,true) . '</pre>';
}else {
exit('<h3 style="color: red">请求的变量错误</h3>');
}
-1,验证HTML页面转过来的请求类型是否合法,请求类型是否是POST$_SERVER['REQUEST_METHOD']
-2,然后获取表单提交过来的信息$_POST['name']
-3,封装获取的信息compact()
有一张怎么都上传不起。。。。