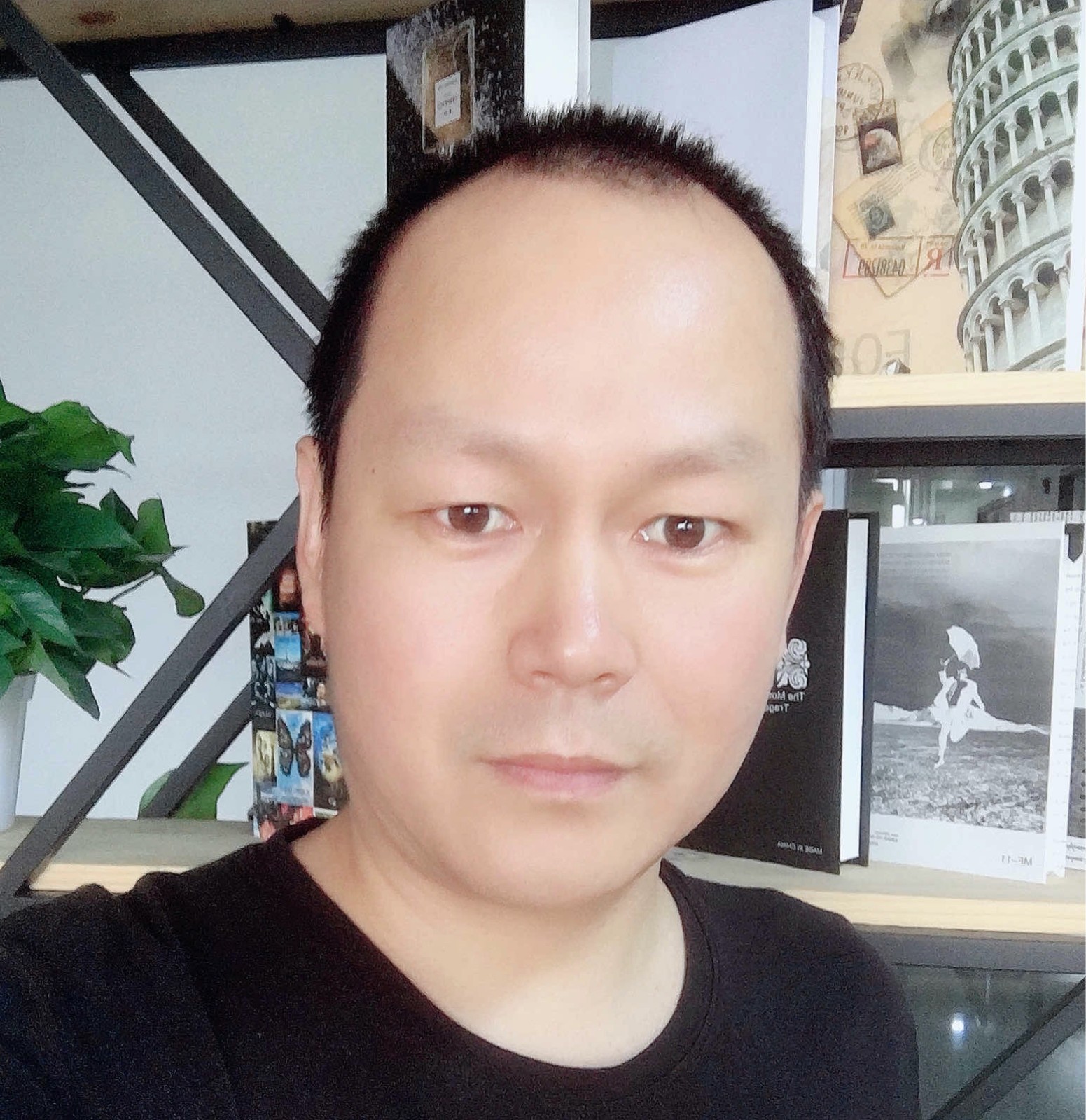
Correction status:qualified
Teacher's comments:完成的很认真
使用单独的变量名来存储一系列的值。
使用数组索引可以直接访问
例如:arr[i]
其中i可以是数字表示数组的顺序,也可以是一个数组中值得键名表示
for循环
来遍历for in
:遍历对象
ES5: forEach()
ES6中的箭头函数
借助的对象字面量的语法来创建的类数组,和数组大致相同。
1.成员的键名必须是0递增的正整数
2.对象必须要有一length
属性
下面是实验的过程
<!DOCTYPE html>
<html>
<head>
<title>数组遍历</title>
<meta charset="utf-8">
</head>
<body>
<!-- 1 将课程中的所有案例全部在按制台运行一遍
2 对于数组的声明,访问,遍历常用的方法全部全部熟练掌握
3 对于函数的声明,调用,参数,以及常用属性,arguments对象必须掌握
4 将数组的遍历与函数的调用与参数处理提交到博客中 -->
<script type="text/javascript">
var apho = ['apple','apple1','apple2','apple3'];
apho.name = 'daapp';
apho['user name'] = 'app';
//for循环遍历
for (var i = 1; i <= 1; i++) {
console.log(apho[i]);
}
//for in: 遍历对象
for (var i in apho) {
console.log(apho[i]);
}
// foreach遍历数组
apho.forEach(function(item, key, apho){
// console.log(item);
console.log(apho[key]);
});
//foreach箭头函数,遍历数组
apho.forEach(item=>console.log(item));
//类数组
var objArr = {
0: 'hello',
1: 'wrold',
2: {1:33.5, 2:44.5},
3: 'defind',
length: 4
};
// objArr.forEach(function (item){
// console.log(item);
// })
for (var i = 0; i < 3; i++) {
var p = document.createElement('p');
p.innerHTML='hello wrold';
document.body.appendChild(p);
console.log(p);
}
var eles = document.getElementsByTagName('p');
console.log(eles);
for (var i = 0; i < eles.length; i++) {
console.log(eles[i].innerHTML);
}
</script>
</body>
</html>
2.函数的声明,调用
function show1(value) {
console.log(value);
}
show1('what you name ');
构造函数
:构建JS语句大厦的基础
构造函数是用来创建对象的
构造函数可以由任何函数构成,但并不是都会创建出对象,除非用new
function show() {...}
js中的函数允许重复声明,以最后一个为准
es5中变量允许重复声明
一旦遇到return
返回给调用者后面的语句全部忽略
函数有name
, length
的属性console.log(add.name);
console.log(add.length);
toString()
, 返回函数源代码console.log(add.toString())
;
全局: global
: 顶层函数之外声明的,可以在函数内访问
局部: local
: 函数内部声明,仅限内部访问,外部不可见
函数内部声明的变量如果不加var
,就不能和当前的作用域绑定, 自动与全局绑定
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>函数</title>
</head>
<body>
<script>
//函数的声明
function show1(value) {
console.log(value);
}
//函数的调用
show1('what you name ');
// 函数表达式 / 匿名函数声明与调用,函数表达式后面没有‘;’
var show2 = function display(value) {
console.log(value);
}
show2('wo jiao da wei ');
console.log(show2.name);
//由于是函数表达式,所以函数未定义,无法传值,显示错误
// display(" How old are You");
//构造函数
var sum = Function('a','b', 'return a + b');
console.log(sum(11, 111));
//与上面的函数意义相同
// var sum = function (a, b) {return a + b;}
// console.log(sum(20, 90));
// 函数做为值的使用场景
function add (a, b, c) {
// 输出结果
return a + b +c;
}
// 赋值,由于还有一个c没传值,结果为NAN
var sum = add;
console.log(sum(12, 23));
// 函数当做对象属性
var obj = {};
obj.sum = add;
console.log(obj.sum(50, 90,13));
// 函数当参数: 回调
function huidiao(callback, a, b,c) {
return callback(a, b,c);
}
console.log(huidiao(add, 28, 49,123));
// 函数当返回值
function fun1() {
return add;
}
console.log(fun1()(39, 27,12) );
// es5: 不支持块作用域
if (true) {
var name = '测试1';
}
console.log(name);
// es6: 支持块作用域,显示未定义
// if (true) {
// let names = '测试2';
// }
// console.log(names);
// 函数的参数对象/参数类数组
function sum(...params) {
console.log(arguments);
for (var i = 0; i < arguments.length; i++) {
console.log(arguments[i]);
}
console.log(a + b);
}
sum(10,20,40,50);
console.log(sum.length);
// 闭包用来访问私有变量
function demo1() {
var email = '144682@qq.com';
// 子函数
return function hello(){
console.log(email);
}
hello;
}
// console.log(email);
// console.log(typeof demo1()());
console.log(demo1());
console.log(demo1()());
</script>
</body>
</html>