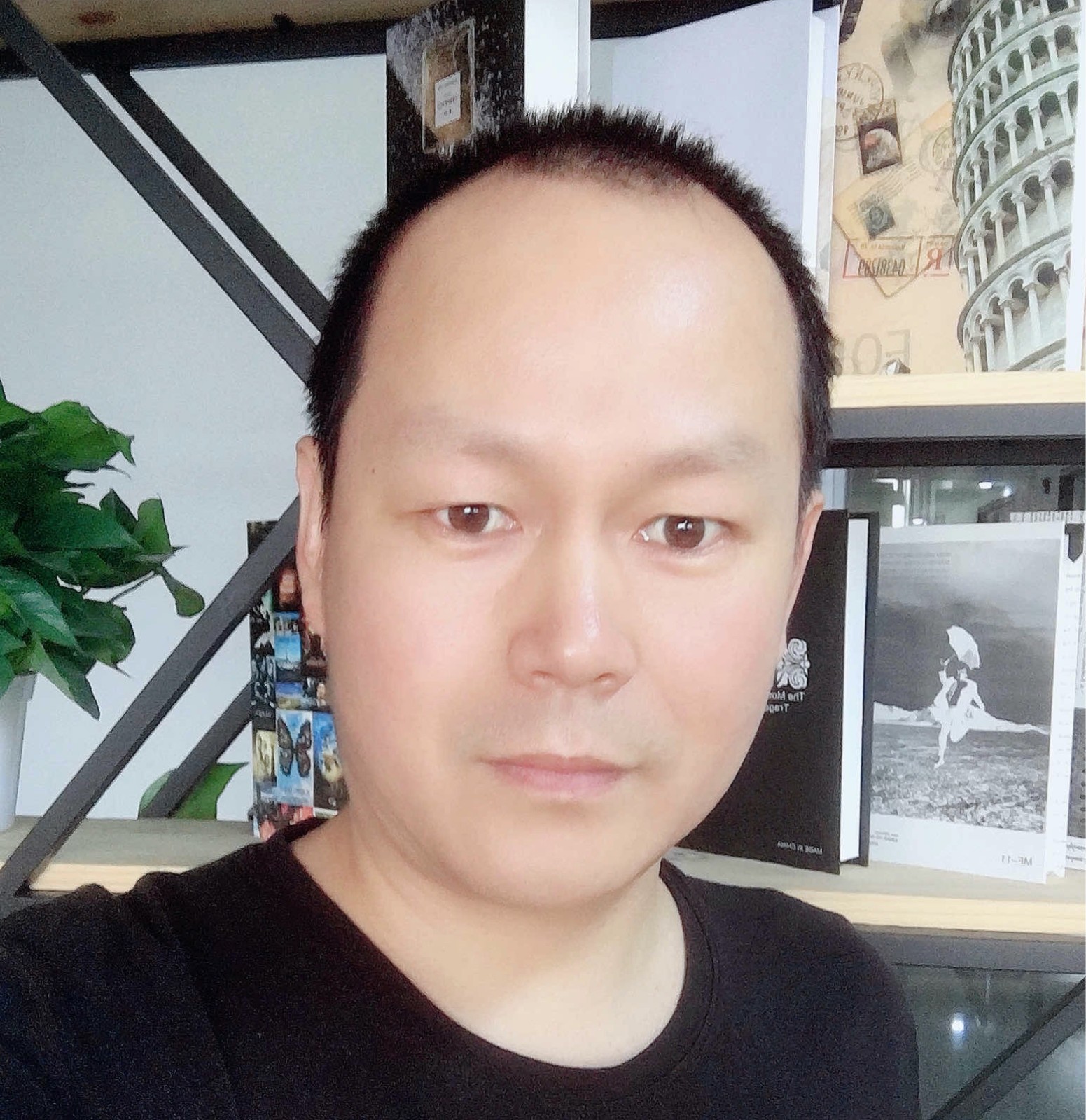
Correction status:qualified
Teacher's comments:思维很清楚,流程很明白, 相信你对这几个最简单的设计 模式是掌握了
单例模式
:创建类的唯一实例,不允许多次实例化一个类,例如数据库的连接有最大上限1000,NEW一次PDO就会多一个类实例,前面的连接如果没释放,就会增加一个,达到最大连接数以后,后面的就不无法再连接数据库了。
将类中的构造方式私有化private
, 防止从外部通过new实例化这个类; 转为从类的内部将它实例化——>创建一个公共的静态方法,返回当前类的唯一实例
克隆方法私有化,防止克隆当前对象: private function __clone()
<?php
namespace chapter7;
// 一般模式:每new一次就会创建一个对象
class Temp
{
//...
}
// 实例化
$obj1 = new Temp;
$obj2 = new Temp;
var_dump($obj1);
echo '<br>';
var_dump($obj2);
echo '<br>';
// 创建了二个完全不同的对象
var_dump($obj1 === $obj2);
echo '<hr>';
//单例模式: 创建类的唯一实例
class Demo1
{
// 将类中的构造方式私有化, 防止从外部通过new实例化这个类
private function __construct()
{
}
// 既然外部不能实例化, 那么只能从为类的内部将它实例化
// 创建一个公共的静态方法,返回当前类的唯一实例
// 当前类实例
private static $instance = null;
public static function getInstance()
{
if (is_null(self::$instance)) {
self::$instance = new self();
}
// 当前类已经实例化过了, 就不要重复实例化,直接返回
return self::$instance;
}
// 克隆方法私有化,防止克隆当前对象
private function __clone()
{
}
}
// 在类的外部不能用对象访问类成员,只能用类,意味着只能访问静态成员
// 获取当前类的唯一实例
$obj1 = Demo1::getInstance();
$obj2 = Demo1::getInstance();
var_dump($obj1 === $obj2);
echo '<br>';
var_dump($obj1);
echo '<br>';
var_dump($obj2);
echo '<hr>';
//vung1类
<?php
namespace base\inc1;
// Vung1类
class Vung1
{
public function sanxuat()
{
return 'soi tho';
}
}
//soicon类
<?php
namespace base\inc1;
// Soicon类
class Soicon
{
public function sanxuat()
{
return 'soi con';
}
}
//danho类
<?php
namespace base\inc1;
// Danho类
class Danho
{
public function sanxuat()
{
return 'thanh pham';
}
}
//工厂模式:将原来依赖对象通过一个工厂来实例化
class Xuong
{
private static $instance = null;
public static function getInstance($sanxuat)
{
switch (strtolower($sanxuat)) {
case 'vung1':
self::$instance = new Vung1();
break;
case 'soicon':
self::$instance = new Soicon();
break;
case 'danho':
self::$instance = new Danho();
break;
}
// 返回具体的类实例
return self::$instance;
}
}
// Sanxuat类
class Sanxuat2
{
// Sanxuat车间
private $sanxuat;
// 将原构造方法中实例化依赖对象的过程,交给工厂类完成了
public function __construct($sanxuat = null)
{
// 将原来依赖三个类, 变成了依赖一个工厂类
$this->sanxuat = Xuong::getInstance($sanxuat);
}
// 调用外部依赖的对象的方法
public function sanxuatMode()
{
return '产品是:===> '. $this->sanxuat->sanxuat();
}
}
// 客户端调用
echo (new Sanxuat2('vung1'))->sanxuatMode() . '<br>';
echo (new Sanxuat2('soicon'))->sanxuatMode() . '<br>';
echo (new Sanxuat2('danho'))->sanxuatMode() . '<br>';
<?php
namespace chapter7;
// 导入刚才创建的三个类
use base\inc2\iVehicle1;
use base\inc2\Vung1;
use base\inc2\Soicon;
use base\inc2\Danho;
// 自动加载器
require __DIR__ . '/autoload.php';
// Sanxuat类
class Sanxuat3
{
// Sanxuat车间
private $sanxuat;
// 将原构造方法中实例化依赖对象的过程,交给工厂类完成了
public function __construct(iVehicle1 $sanxuat = null)
{
// 将原来依赖三个类, 变成了依赖一个工厂类
$this->sanxuat = $sanxuat;
}
// 调用外部依赖的对象的方法
public function sanxuatMode()
{
return '产品是:+++> '. $this->sanxuat->sanxuat();
}
}
// 客户端调用
echo (new Sanxuat3(new Vung1()))->sanxuatMode() . '<br>';
echo (new Sanxuat3(new Soicon()))->sanxuatMode() . '<br>';
echo (new Sanxuat3(new Danho()))->sanxuatMode() . '<br>';