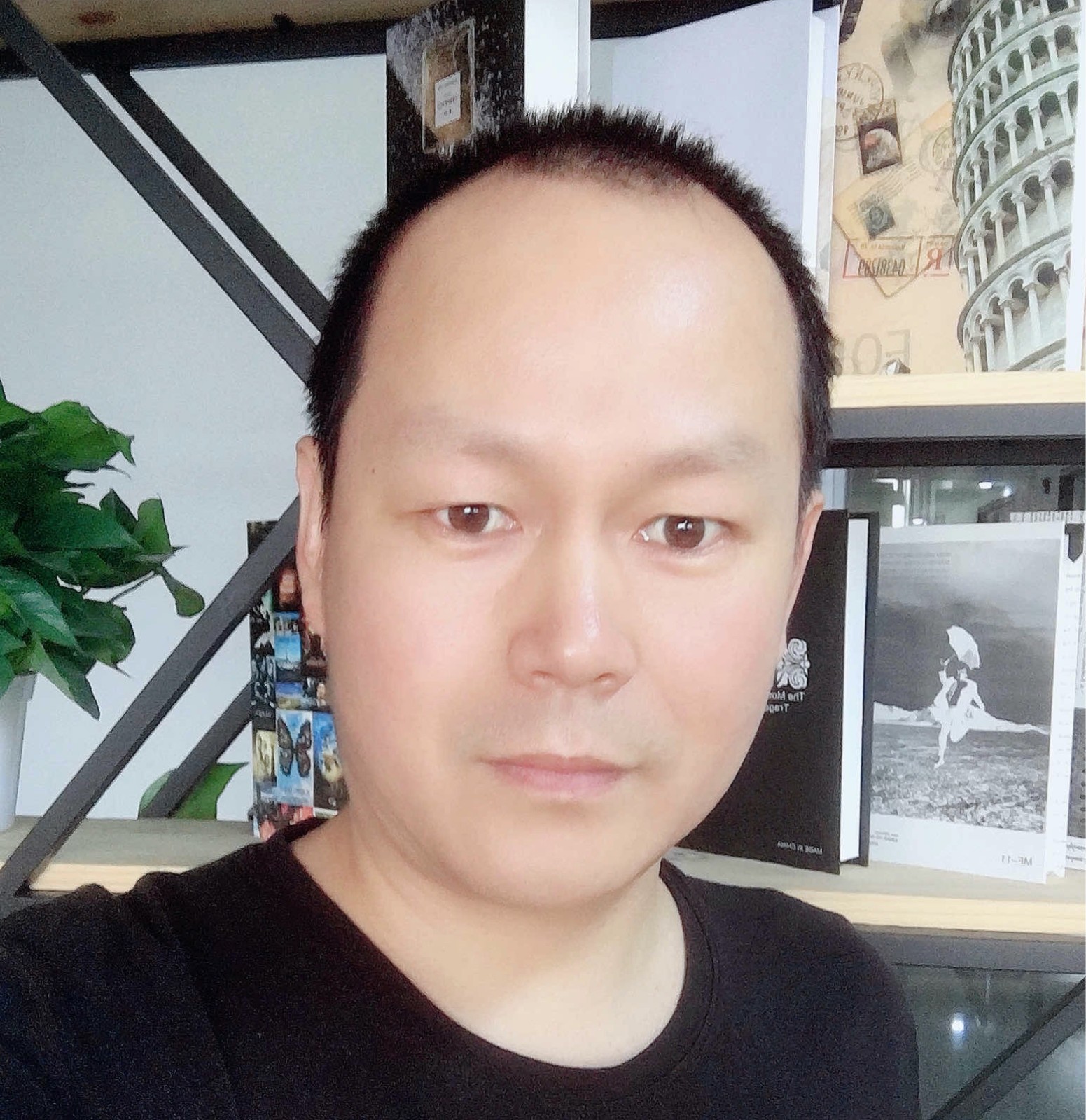
Correction status:qualified
Teacher's comments:OOP编程的核心, 咱们实际上都接触到了, 继续加油
/** 示例演示类继承的三大功能 */
// 基类:Animal
abstract class Animal
{
public $type = '哺乳动物';
protected $name = '';
/**
* 介绍自己
*/
public function adviceMyself()
{
return '我是' . $this->name;
}
}
/**
* 继承
* 实现类:Dog
*/
class Dog extends Animal
{
/**
* 属性重写
*/
public $name = '狗';
/**
* 扩展基类
*/
public function WagTail()
{
return '摇尾巴~~~';
}
}
/**
* 继承
* 实现类:Cat
*/
class Cat extends Animal
{
public $name = '猫';
/**
* 重写父类方法
*/
public function adviceMyself()
{
return '我是' . $this->name . ', 我属于' . $this->type . ', 我会' . $this->howl();
}
}
/* 汤姆 */
$tom = new Cat;
/* 史努比 */
$snoopy = new Dog;
// 介绍自己
/* 汤姆介绍自己,调用重写父类的方法 */
echobr($tom->adviceMyself());
/* 史努比介绍自己,调用继承自父类的方法 */
echobr($snoopy->adviceMyself());
// 史努比摇尾巴,扩展父类没有的方法
echobr($snoopy->WagTail());
/*
result:
我是猫, 我属于哺乳动物, 我会喵喵喵~~~
我是狗
喵喵喵~~~
汪汪汪~~~
摇尾巴~~~
*/
- - 抽象类的作用: 部分分离了声明与实现, 声明在抽象类中完成, 实现在工作类中完成.
/** 实例演示抽象类的作用与实现 */
// 基类:动物类
abstract class Animal
{
public $type = '哺乳动物';
protected $name = '';
// 不同动物叫的方式不一样,所以定义为抽象方法
abstract public function howl();
}
// 实现类
/* 狗 */
class Dog extends Animal
{
public $name = '狗';
public function howl()
{
return '汪汪汪~~~';
}
}
/* 猫 */
class Cat extends Animal
{
public $name = '猫';
public function howl()
{
return '喵喵喵~~~';
}
}
/* 史努比 */
$snoopy = new Dog;
echobr($snoopy->name . '的叫声是:' . $snoopy->howl());
/* 汤姆 */
$tom = new Cat;
echobr($tom->name . '的叫声是:' . $tom->howl());
/*
result:
狗的叫声是:汪汪汪~~~
猫的叫声是:喵喵喵~~~
*/
- 接口完全分离了声明与实现
- 接口使用关键字: interface
- 接口中定义的成员必须是public
- 接口允许多继承, 从而间接实现PHP的多继承
// 定义接口
interface Itf1
{
// 定义接口常量
const MAX = 65535;
// 定义抽象方法(不需要abstract关键字)
public function func1();
}
class Demo1 implements Itf1
{
// 实现接口的抽象方法
public function func1()
{
return __CLASS__ . '类的' . __METHOD__ . '方法';
}
}
// 使用接口中的常量
echobr(Itf1::MAX);
// 调用实现了接口的方法
echobr((new Demo1)->func1());
/*
result:
65535
Demo1类的Demo1::func1方法
*/