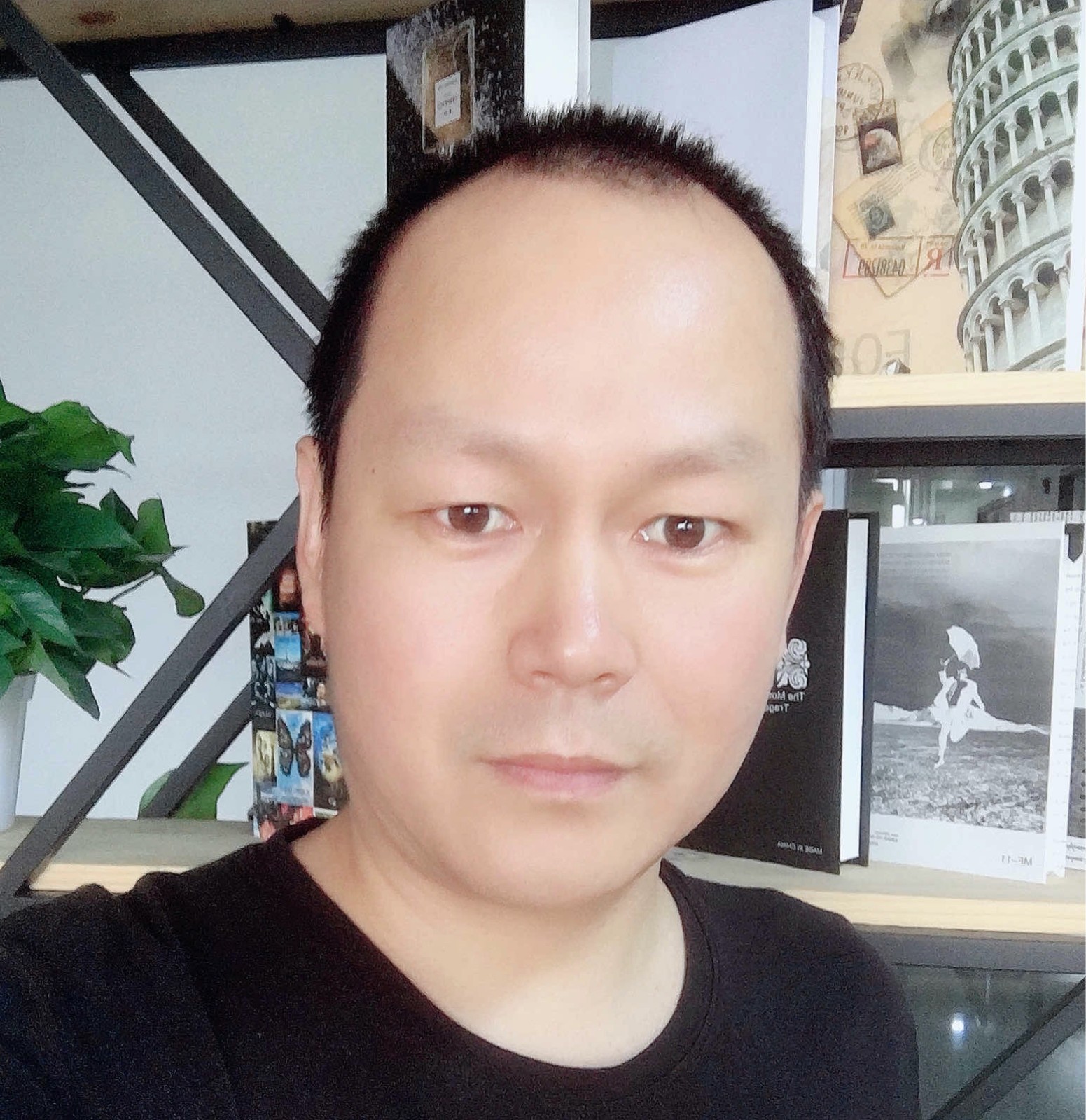
Correction status:qualified
Teacher's comments:接口, 像是宪法, 是其它法律的参考与依据
就如同老师给同学们出了一道开放题,这个题目是抽象的,解答的方法有很多
<?php
interface Fruit
{
const MAX_WEIGHT = 5; //静态常量(接口的常量)
function setName($name);
function getName();
}
//实现接口
class Apple implements Fruit
{
private $name;
function getName()
{
return $this->name;
}
function setName($_name)
{
$this->name = $_name;
}
}
$apple = new Apple(); //创建对象
$apple->setName("苹果");
echo "创建了一个" . $apple->getName();
echo "<br />";
echo "MAX_GRADE is " . Apple::MAX_WEIGHT; //静态常量
接口是一堆方法的说明,不能加属性(成员变量)
接口就是供组装成类用的,方法只能用 public;
<?php
interface iNicola
{
//接口常量
const NATION = '中国广东梅州';
}
interface iNicola1 extends iNicola
{
//接口常量
const USER_NAME = '尼古拉';
}
//接口允许多继承
interface iNicola2 extends iNicola, iNicola1
{
//接口方法
public function write();
}
//实现类
class Nicola implements iNicola, iNicola1, iNicola2
{
//必须实现接口中的抽象方法
public function write()
{
return iNicola1::USER_NAME . '家乡是:' . iNicola::NATION;
}
}
echo (new Nicola)->write();
代码复用
在继承上下文环境中,具有优先级,通过优先级别的设置,降低单继承的影响
子类 > trait > 父类
<?php
// trait php5.4+ 代码复用
// 与抽象类和接口一样,不能被实例化,只能嵌入到宿主类使用
// trait是一个特殊类:1.常规属性,2.静态,3.抽象,不能有类常量
trait tDemo
{
//常规
public $nicola = '尼古拉';
public function get()
{
return $this->nicola;
}
//静态
public static $nico = '搬运工/Boss';
public static function set()
{
return '职业是:' . self::$nico;
}
//抽象
public static $age;
//抽象方法
abstract public static function set1();
}
// 客户端
class Nicola
{
use tDemo;
public static function set1()
{
return self::$age;
}
}
$nicola = new Nicola;
Nicola::$age = '18';
echo Nicola::set1();
<?php
trait Dog{
public $name="小狗";
public function bark(){
echo "这是一只旺财";
}
}
class Animal{
public function eat(){
echo "旺财正在吃东西";
}
}
class Cat extends Animal{
use Dog;
public function drive(){
echo "旺财在开车";
}
}
$cat = new Cat();
$cat->drive();
echo "<br/>";
$cat->eat();
echo "<br/>";
$cat->bark();
关于 trait 的理解,php 从以前到现在一直都是单继承的语言,无法同时从两个基类/父类中继承属性和方法,为了解决这个问题,php 出了 Trait 这个特性。通过在类中使用 use 关键字,声明要组合的 Trait 名称,具体的 Trait 的声明使用 Trait 关键词,Trait 不能实例化。