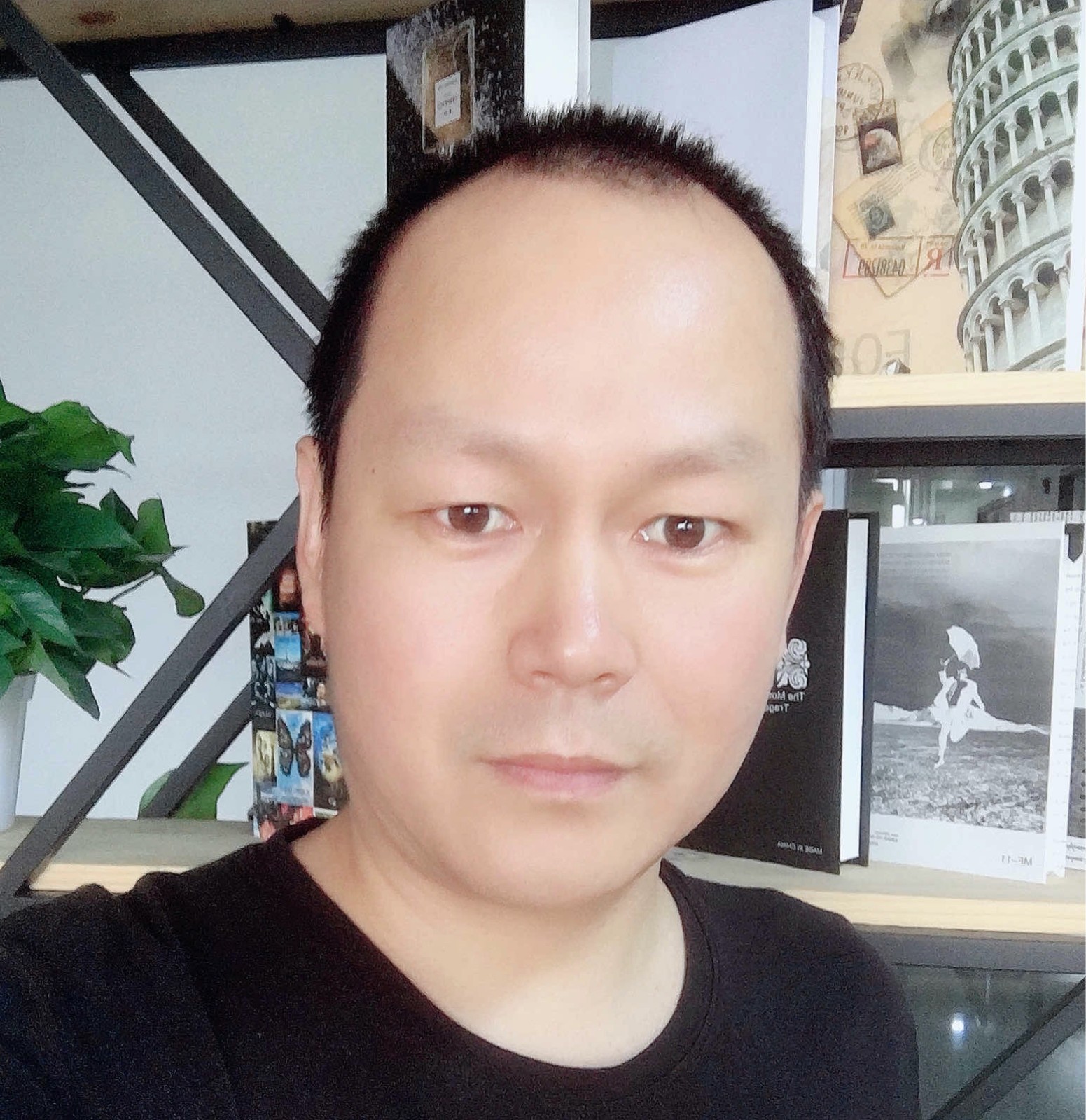
Correction status:qualified
Teacher's comments:接口的用法, 大家以后还要多多练习
// 父类
class Book
{
public $title = '三字经';
protected $price = '20';
private $isbn = '123-456';
public function show()
{
echo "《 {$this->title} 》,售价:{$this->price} 元,ISBN:{$this->isbn}。";
}
}
// 继承
class Book1 extends Book
{
// 重写属性
public $title = '千字文';
protected $price = 30;
private $isbn = '456-123';
// 属性扩展
// 静态属性不能通过已实例化的对象访问,静态方法可以访问
public static $author = '周兴嗣';
// 重写方法
public function show()
{
// 引用父类中已有的方法
echo parent::show() . '作者:' . self::$author;
}
// 扩展方法,增加父类的功能
public static function show1()
{
echo ' 《千字文》作者是 ' . self::$author;
}
}
$book = new Book();
echo $book->show() . '<br><hr>';
$book1 = new Book1();
echo $book1->show() . '<hr>';
Book1::show1();
echo '<hr>';
输出结果:
// 抽象类(设计)不能被实例化,至少要有一个抽象方法
abstract class Phone
{
protected $system = 'ios';
protected $brand = 'apple';
// 抽象方法,没有方法体,其功能在子类中实现
abstract protected function display();
}
// 工作类(实现)
class IPhone extends Phone
{
protected $network = '5G';
public function display()
{
return "{$this->brand} 的网络制式是 {$this->network}";
}
}
$iphone = new IPhone();
echo $iphone->display(); // apple 的网络制式是 5G
// 接口语法
interface User
{
// 接口属性
const USERTYPE = 'VIP';
// 接口方法,必须是抽象方法
public function getDiscount();
}
// 工作类
class VipUser implements User
{
private $discount = 0.8;
// 必须实现接口中的方法
public function getDiscount()
{
return " 用户的折扣率是:{$this->discount}";
}
}
$vipuser = new VipUser();
echo User::USERTYPE . $vipuser->getDiscount();
输出结果: