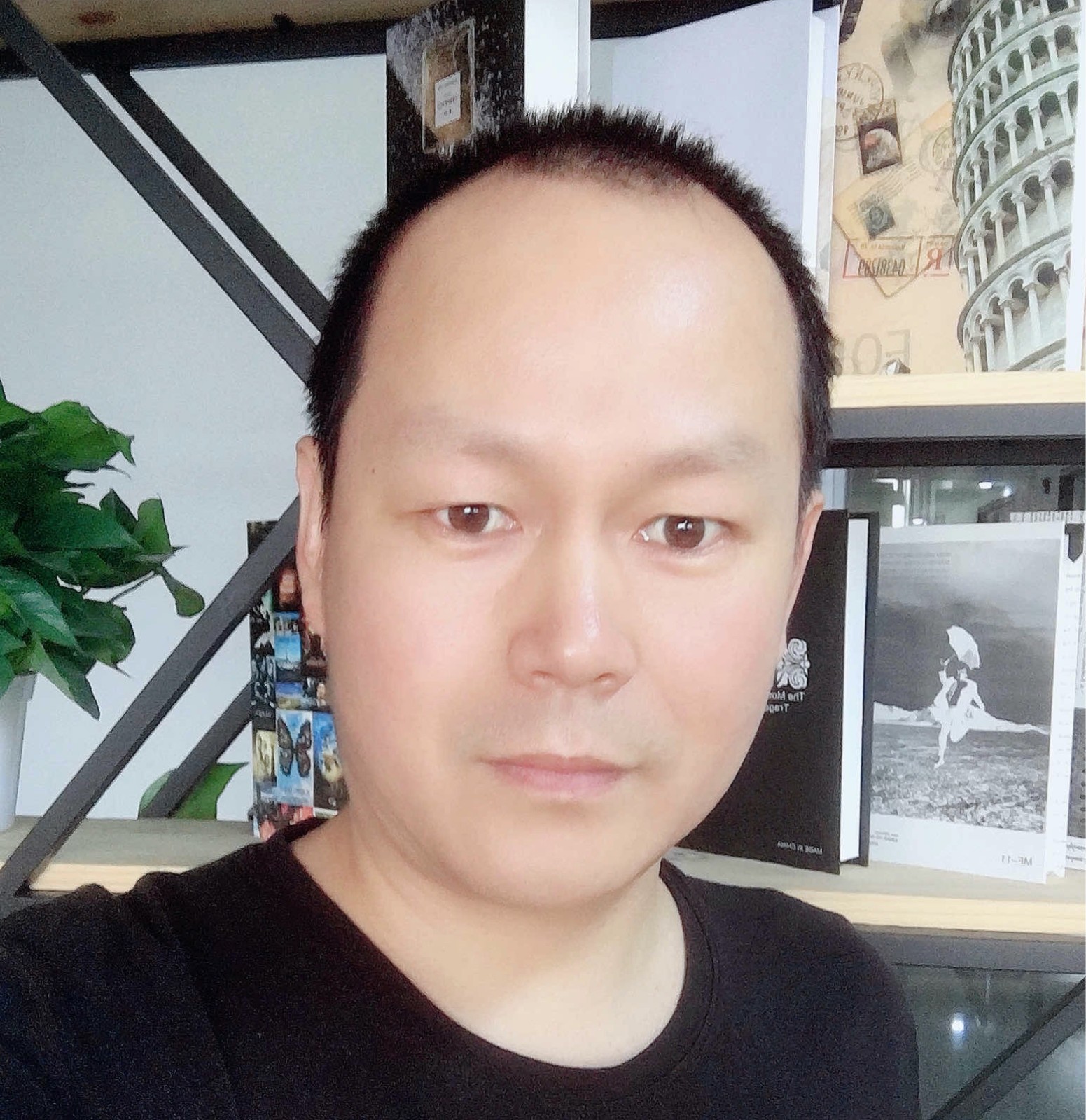
Correction status:qualified
Teacher's comments:不必重复内容
<?php
//重载:访问拦截器(属性,方法拦截器)
//访问不存在或无权限属性/方法自动调用
// 属性拦截器 __set() , __get(), __isset() ,__unset()
//// 构造方法: __construct(), 是类的实例化过程中被自动调用, new 的时候
//再次注意:构造函数不能是静态
class Demo
{
private $brand;
private $model;
protected $price;
public function __construct($brand,$model,$price)
{
$this->brand=$brand;
$this->model=$model;
$this->price=$price;
}
//创建属性拦截器
public function __get($accept)
{
$attri='get'.ucfirst($accept);
return method_exists($this,$attri) ? $this->$attri() : null;
}
private function getBrand()
{
return "品-牌:".mb_substr($this->brand,0,1).'*';
}
private function getModel()
{
return "<br>型-号:".substr($this->model,0,3).'*';
}
private function getPrice()
{
return "价-格:".$this->price;;
}
//设置拦截器
public function __set($accept,$val)
{
$attri='set'.ucfirst($accept);
return method_exists($this,$attri) ? $this->$attri($val):null;
}
private function setBrand($val)
{
$this->brand=trim($val);
}
private function setModel($val)
{
$this->model=trim($val);
}
private function setPrice($val)
{
$this->price=$val;
// var_dump($val);
}
public function __isset($accept)
{
$attri='isset'.ucfirst($accept);
return method_exists($this,$attri) ? $this->$attri() : null;
}
private function issetBrand()
{
return $this->brand;
}
private function issetModel()
{
return $this->model;
}
private function issetPrice()
{
return isset($this->price);
}
//删除拦截器
public function __unset($accept)
{
if($accept==='brand')
{
$attri='set'.ucfirst($accept);
if(method_exists($this,$attri)) return $this->$attri(null);
}
}
}
//属性拦截器
$demo=new Demo('啊为','pro20','请 ->商——家');
echo $demo->barnd;
?>
<html>
<body>
<style>
table{border:0px;cellspacing:2px;cellpadding:2px;background:#FF00FF;width:200px}
TR{ BACKGROUND:#ccd}
TD{padding:0;margin:0;text-align:center}
</style>
<table >
<tr>
<td style="background:#FFF">属性拦截器</td>
</tr>
<tr>
<td><?php echo $demo->brand;?></td>
</tr>
<tr>
<td><?php echo $demo->model;?></td>
</tr>
<tr>
<td><?php echo $demo->price; ?></td>
</tr>
<?php
$demo->brand="米米";
$demo->model="Note 3";
$demo->price="999";
?>
<tr>
<td style="background:#FFF">设置拦截器</td>
</tr>
<tr>
<td><?php echo $demo->brand; ?></td>
</tr>
<tr>
<td><?php echo $demo->model; ?></td>
</tr>
<tr>
<td><?php echo $demo->price; ?></td>
</tr>
<tr>
<td style="background:#FFF">属性检测拦截器</td>
</tr>
<tr>
<td><?php echo isset($demo->brand) ? '品-牌:存在':'品-牌:不存在'; ?></td>
</tr>
<tr>
<td><?php echo isset($demo->moddel) ? '型-号:存在':'型-号:不存在'; ?></td>
</tr>
<tr>
<td><?php echo isset($demo->pricde) ? '价-格:存在':'价-格:不存在'; ?></td>
</tr>
<?php
unset($demo->brand);
?>
<tr>
<td style="background:#FFF">删除属性截器</td>
</tr>
<tr>
<td><?php echo $demo->brand; ?></td>
</tr>
<tr>
<td><?php echo $demo->model; ?></td>
</tr>
<tr>
<td><?php echo $demo->price; ?></td>
</tr>
</table>
</body>
</html>