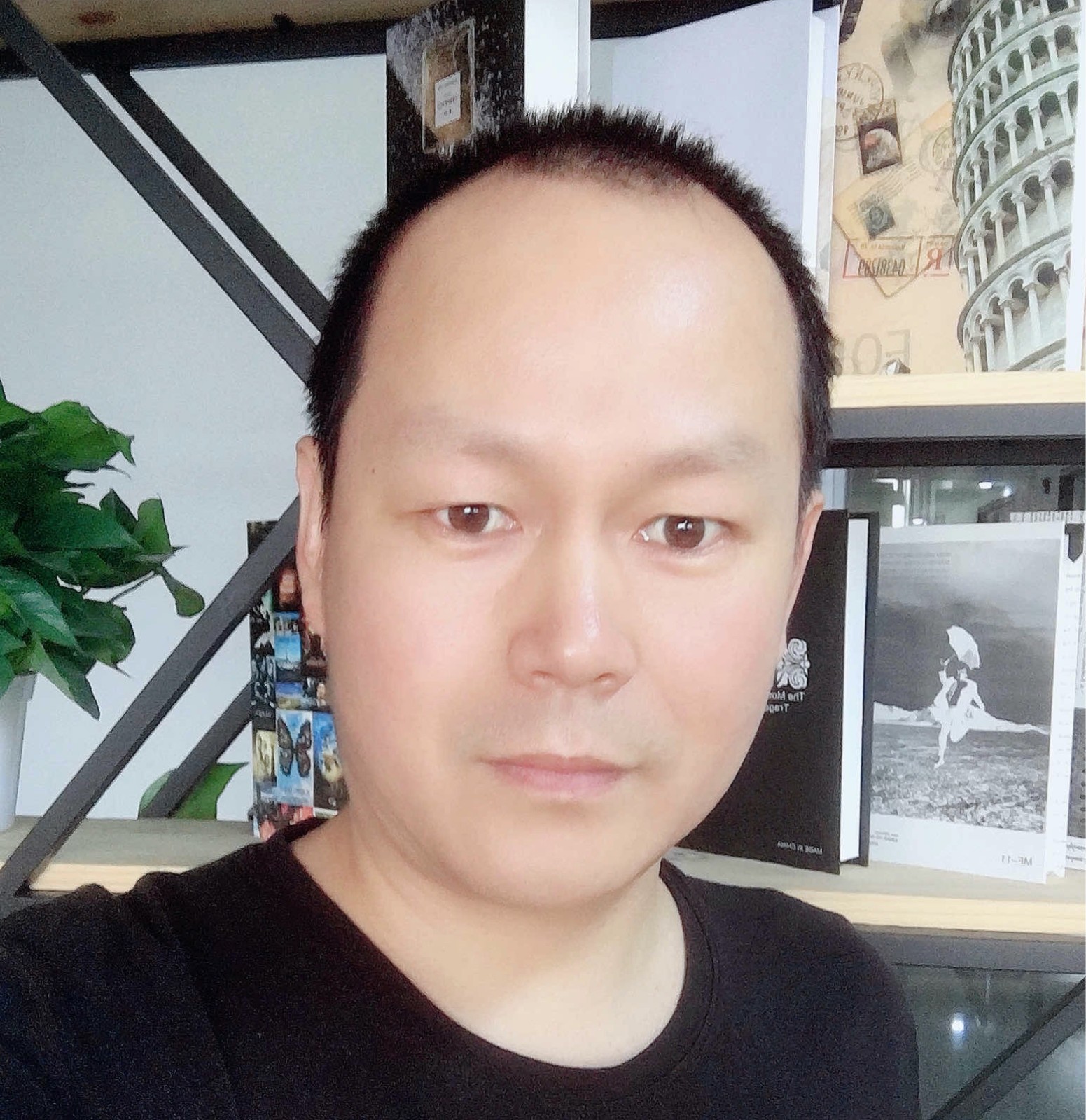
Correction status:qualified
Teacher's comments:总结的不错, 到时就大胆的在项目中去用它们吧
abstract class A
{
//当实现类中2个函数功能相同时,可以写在抽象类中
//抽象类不能实例化
//将类的定义与类的调用分离开
//用后期静态绑定,使用关键字static将self替换
//self 始终与定义它的类绑定
//static始终与调用它的类绑定
public function write()
{
//return new self;
return new static();
}
public static function amount($price,$sum)
{
return '价格是:'.$amount = $price * $sum;
}
public static function index($price,$sum)
{
//return self::amount($price,$sum);
return static::amount($price,$sum);
}
}
class Base extends A
{
// public function write():self
// {
// return new self();
// }
public static function amount($price, $sum)
{
return '优惠后价格是:'.$amount = $price * $sum *0.8;
}
}
class C extends A
{
// public function write():self
// {
// return new self();
// }
}
//当子类改写父类的方法 由于是self关键字,在调用index()时 还是绑定定义它的父类的方法,所以子类改写没有效果
echo Base::index(100,2); //输出结果是 价格是:200
//如果把class A中的index()方法中的self改成static,那么调用Base的index() 就与Base绑定
//输出的结果是 优惠后价格是:160
<?php //构造方法:__construct(),在类的实例化中被调用,功能就是生成一个新对象;
class Product
{
private $name;
private $price;
public function __construct($name,$price,$sum)
{
//1.生成一个新对象,类实例
//2.初始化对象
$this->name = $name;
$this->price = $price;
$this->sum = $sum;
$this->write();
//返回值是隐式返回 返回当前新实例
}
public function write()
{
$count = $this->price * $this->sum;
echo "$this->name:数量为:$this->sum,单价:$this->price 元,总价为$count 元";
}
}
$product = new Product('手机',500,6);
//PHP重载:又称拦截器,分属性拦截器,方法拦截器
//使用场景:当用户访问一个不存在或无权访问的属性或者方法时自动调用
//属性拦截器:__get(),__set(),__unset(),__isset()
class Person
{
private $name;
private $age;
private $profession=12;
public function __construct($name,$age)
{
$this->name = $name;
$this->age = $age;
}
//访问拦截器
public function __get($property)
{
$methed = 'get'.ucfirst($property);
return method_exists($this,$methed) ? $this->$methed() : '无权访问';
}
private function getName()
{
return $this->name;
}
private function getAge()
{
return $this->age;
}
private function getProfession()
{
return $this->profession;
}
//设置拦截器
public function __set($property,$value)
{
$methed = 'set'.ucfirst($property);
return method_exists($this,$methed) ? $this->$methed($value) : null;
}
private function setAge($value)
{
return $this->age = $value;
}
//属性检测拦截器
public function __isset($property)
{
return $property === 'name' ? true :false;
}
//属性销毁拦截器
public function __unset($property)
{
if ($property==='profession'){
unset($this->profession);
}
}
}
$person = new Person('张三',35);
echo $person->name; //输出张三
echo $person->age; //输出35
echo '<hr>';
$person->age = '40';
echo $person->age; //输出40
echo isset($person->name) ?'存在':'不存在';
unset($person->profession);
echo $person->profession; //销毁了profession
//方法拦截器:__call(),__callstatic
class Product
{
public function __call($name, $arguments)
{
if($name === 'write')
if(is_int($arguments[0]))
{
$this->doForint();
}elseif(is_string($arguments[0])){
$this->doForstr();
}
}
public function doForint()
{
echo __METHOD__.'方法';
}
public function doForstr()
{
echo __METHOD__.'方法';
}
}
$product = new Product;
echo $product->write('1'); //输出Product::doForstr方法
这节课学习后期静态绑定与拦截器,后期静态绑定不再被解析为定义当前方法所在的类,而是可以在子类绑定。拦截器可以对用户的一些非法设置进行处理,带来不必要的麻烦。