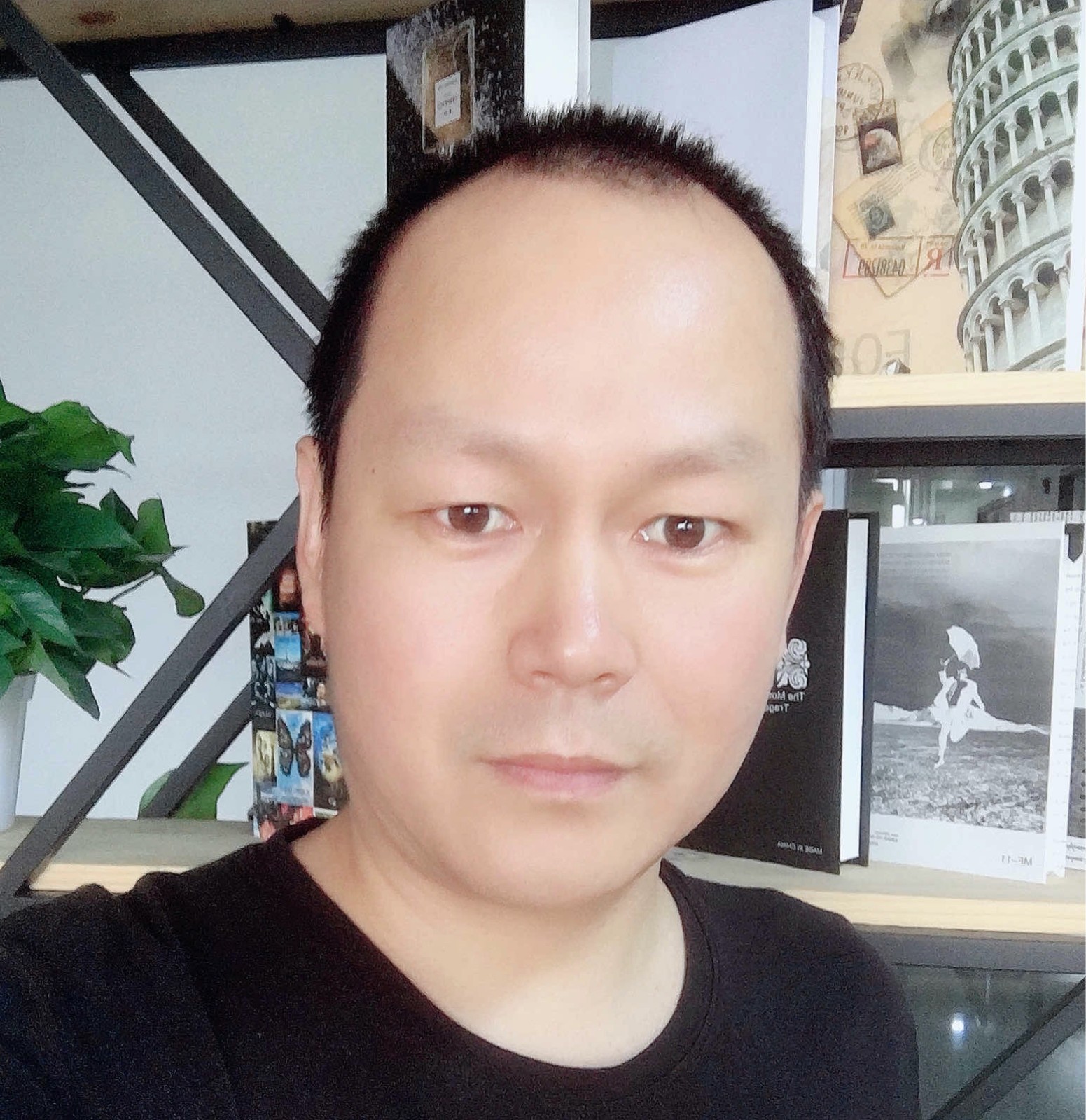
Correction status:qualified
Teacher's comments:规范的做法, 应该是将数据库连接参数通过构造方法传入, 试试看
一个简单的注册登录验证页面
用户名,邮箱,电话是不可重复注册的
db.php
<?php
/*
* 创建sql表
* CREATE TABLE `usertab` (
`id` int(3) NOT NULL AUTO_INCREMENT COMMENT '主键',
`name` varchar(30) CHARACTER SET utf8 NOT NULL COMMENT '用户名',
`password` varchar(40) CHARACTER SET utf8 NOT NULL COMMENT '密码',
`email` varchar(30) CHARACTER SET utf8 NOT NULL COMMENT '邮箱',
`tel` varchar(30) CHARACTER SET utf8 NOT NULL COMMENT '电话',
`time` int(10) unsigned NOT NULL COMMENT '注册时间',
UNIQUE KEY (`name`),
UNIQUE KEY (`email`),
UNIQUE KEY (`tel`),
PRIMARY KEY (`id`))
*/
/*
* @Author: 数据库类
* @Date: 2020-05-08 14:04:42
* @LastEditTime: 2020-05-09 18:04:54
* @LastEditors: Please set LastEditors
* @Description: In User Settings Edit
* @FilePath: \test\db.php
*/
class Darabase
{
private $config =
[
'host' => 'mysql:host=localhost;dbname=phpedu;',
'username' => 'root',
'password' => 'root'
];
private $pdo_obj;
public function __construct()
{
$this->pdo_obj = new PDO(
$this->config['host'],
$this->config['username'],
$this->config['password'],
);
}
/**
* 注册新用户,成功返回true,失败返回false
*
* @param [type] $name 用户名
* @param [type] $pass 密码
* @param [type] $email 邮箱
* @param [type] $tel 电话
* @param [type] $time 注册时间
* @return bool
*
*/
public function reguser($name, $pass, $email, $tel, $time)
{
$pass = sha1($pass);
$sql = "INSERT `usertab` SET
`name`='$name',
`password`='$pass',
email='$email',
tel='$tel',
`time`=$time";
$obj = $this->pdo_obj->prepare($sql);
$obj->execute();
if ($obj->rowCount() > 0) :
return true;
else :
return false;
endif;
}
/**
* 数据库查询,返回查询的结果或false
*
* @param [string] $result 要求返回的查询结果
* @param [string] $field 要查询的字段
* @param [string] $value 查询的字段的值
* @return mixed
*/
public function search($result, $field, $value)
{
$sql = "SELECT `$result` FROM `usertab` WHERE `$field`='$value'";
$obj = $this->pdo_obj->prepare($sql);
$obj->execute();
$result = $obj->fetch()[0];
return $result ? $result : false;
}
/**
* 查询用户是否存在,存在返回true,不存在返回false
*
* @param [string] $name 用户名
* @param [string] $pass 用于接收密码的变量
* @return bool
*/
public function searchName($name, &$pass = null)
{
$pass = $this->search('password', 'name', $name);
var_dump($pass);
return $pass ? true : false;
}
/**
* 登录验证,成功返回true,失败返回false
*
* @param [string] $name 用户名
* @param [string] $pass 密码
* @return bool
*/
public function login($name, $pass)
{
$pass = sha1($pass);
return $this->searchName($name, $rpass) ?
$pass === $rpass ? true : false
:
false;
}
}
handle.php
<?php
require('db.php');
$action = filter_input(INPUT_GET, 'action');
if ($action === null) die('参数非法');
//var_dump($_POST, $action);
switch ($action) {
case 'reg':
if (!$_POST) die('参数非法');
$data = new Darabase();
if ($data->search('name', 'name', $_POST['name'])) header('Location:reg.php?message=用户名已存在');
if ($data->search('email', 'email', $_POST['email'])) header('Location:reg.php?message=邮箱已注册');
if ($data->search('tel', 'tel', $_POST['tel'])) header('Location:reg.php?message=手机号已注册');
if ($data->reguser(
$_POST['name'],
$_POST['password'],
$_POST['email'],
$_POST['tel'],
(string) time()
)) :
echo '注册成功!<br><a href="login.php">>>>登录</a>';
else :
echo '注册失败';
endif;
break;
case 'login':
if (!$_POST) die('参数非法');
$data = new Darabase();
if ($data->login($_POST['name'], $_POST['password'])) :
session_start();
$pass = sha1($_POST['password']);
$_SESSION['name'] = $_POST['name'];
$_SESSION['password'] = $pass;
setcookie('name', $_POST['name'], (time() + 360 * 24));
setcookie('pass', $pass, (time() + 360 * 24));
header('location:index.php');
else :
header('Location:login.php?message=账号或密码错误');
endif;
break;
case 'out':
session_start();
session_destroy();
setcookie('name', null, 0);
header('location:index.php');
break;
default:
echo '参数非法';
break;
}
index.php
<?php
if ($_COOKIE['name'])
session_start();
?>
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<link rel="stylesheet" href="index.css">
<title>首页</title>
</head>
<body>
<section>
<ul class="nav black">
<li><a href="">主页</a></li>
<li><a href=""></a></li>
<li><a href=""></a></li>
<li><a href=""></a></li>
<li><a href=""></a></li>
<?php if (
$_COOKIE['name'] . $_COOKIE['pass'] === $_SESSION['name'] . $_SESSION['password']
&& $_COOKIE['name']
) : ?>
<li><a href="handle.php?action=out">退出</a></li>
<? else : ?>
<li><a href="login.php">登录</a></li>
<? endif; ?>
</ul>
</section>
</body>
</html>
login.php
<?php $mess = filter_input(INPUT_GET, 'message'); ?>
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<link rel="stylesheet" href="style.css">
<title>首页</title>
</head>
<body>
<div class='login'>
<h2 style='text-align: center;padding-top:20px'>用户登录</h3>
<form action="handle.php?action=login" method="post">
<div>
<label for="input_name" class=lab>用户名:</label>
<input type="text" name="name" id="input_name">
</div>
<div>
<label for="input_pass" class=lab>密码:</label>
<input type="password" name="password" id="input_pass">
</div>
<div>
<input type="submit" value="登录" id=sub>
</div>
<div>
<?php echo "<font style='color:#FF0000;'>" . $mess . '</font>'; ?> <a style='padding-left: 30%;' href="reg.php">注册>>></a>
</div>
</form>
</div>
</body>
</html>
reg.php
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<link rel="stylesheet" href="style.css">
<title>首页</title>
</head>
<body>
<?php $mess = filter_input(INPUT_GET, 'message'); ?>
<div class='login' style='height:460px;'>
<h2 style='text-align: center;padding-top:20px'>用户注册</h2>
<form action="handle.php?action=reg" method="post">
<div>
<label for="input_name" class=lab>用户名:</label>
<input type="text" name="name" id="input_name">
</div>
<div>
<label for="input_pass" class=lab>密码:</label>
<input type="password" name="password" id="input_pass">
</div>
<div>
<label for="input_pass1" class=lab>重复密码:</label>
<input type="password" name="" id="input_pass1">
</div>
<div>
<label for="input_email" class=lab>邮箱:</label>
<input type="email" name="email" id="input_email">
</div>
<div>
<label for="input_tel" class=lab>手机号:</label>
<input type="text" name="tel" id="input_tel">
</div>
<div>
<input type="submit" value="注册" id=sub>
</div>
</form>
<?php echo "<h4 style='text-align:center;color:#FF0000;'>" . $mess . '</h4>'; ?>
</div>
</body>
</html>
index.css
* {
margin : 0;
padding : 0;
list-style-type: none;
}
.nav a {
display : inline-block;
-webkit-transition: all 0.2s ease-in;
-moz-transition : all 0.2s ease-in;
-o-transition : all 0.2s ease-in;
-ms-transition : all 0.2s ease-in;
transition : all 0.2s ease-in;
}
.black {
background: #2c2c2c;
box-shadow: 0 7px 0 #0b0b0b;
}
.black li::before {
left : 0;
background: -moz-linear-gradient(top, #2c2c2c, #000 50%, #2c2c2c);
background: -webkit-linear-gradient(top, #2c2c2c, #000 50%, #2c2c2c);
background: -o-linear-gradient(top, #2c2c2c, #000 50%, #2c2c2c);
background: -ms-linear-gradient(top, #2c2c2c, #000 50%, #2c2c2c);
background: linear-gradient(top, #2c2c2c, #000 50%, #2c2c2c);
}
body {
background: #ebebeb;
}
.nav {
width : 100%;
height : 50px;
font : bold 0/50px Arial;
text-align : center;
margin : 40px auto 0;
border-radius: 8px;
}
.nav a:hover {
-webkit-transform: rotate(10deg);
-moz-transform : rotate(10deg);
-o-transform : rotate(10deg);
-ms-transform : rotate(10deg);
transform : rotate(10deg);
}
.nav li {
position : relative;
display : inline-block;
padding : 0 16px;
font-size : 13px;
text-shadow: 1px 2px 4px rgba(0, 0, 0, .5);
list-style : none outside none;
}
.nav a,
.nav a:hover {
color : #fff;
text-decoration: none;
}
style.css
div.login {
margin : auto;
background-color: cornflowerblue;
height : 310px;
width : 300px;
line-height : 50px;
position : relative;
}
label.lab {
padding-inline-start: 25px;
display : block;
width : 70px;
display : inline-block;
}
#sub {
background-color: honeydew;
height : 30px;
width : 250px;
margin : auto;
display : block;
margin-top : 30px;
}
https://www.90pan.com/b1872334