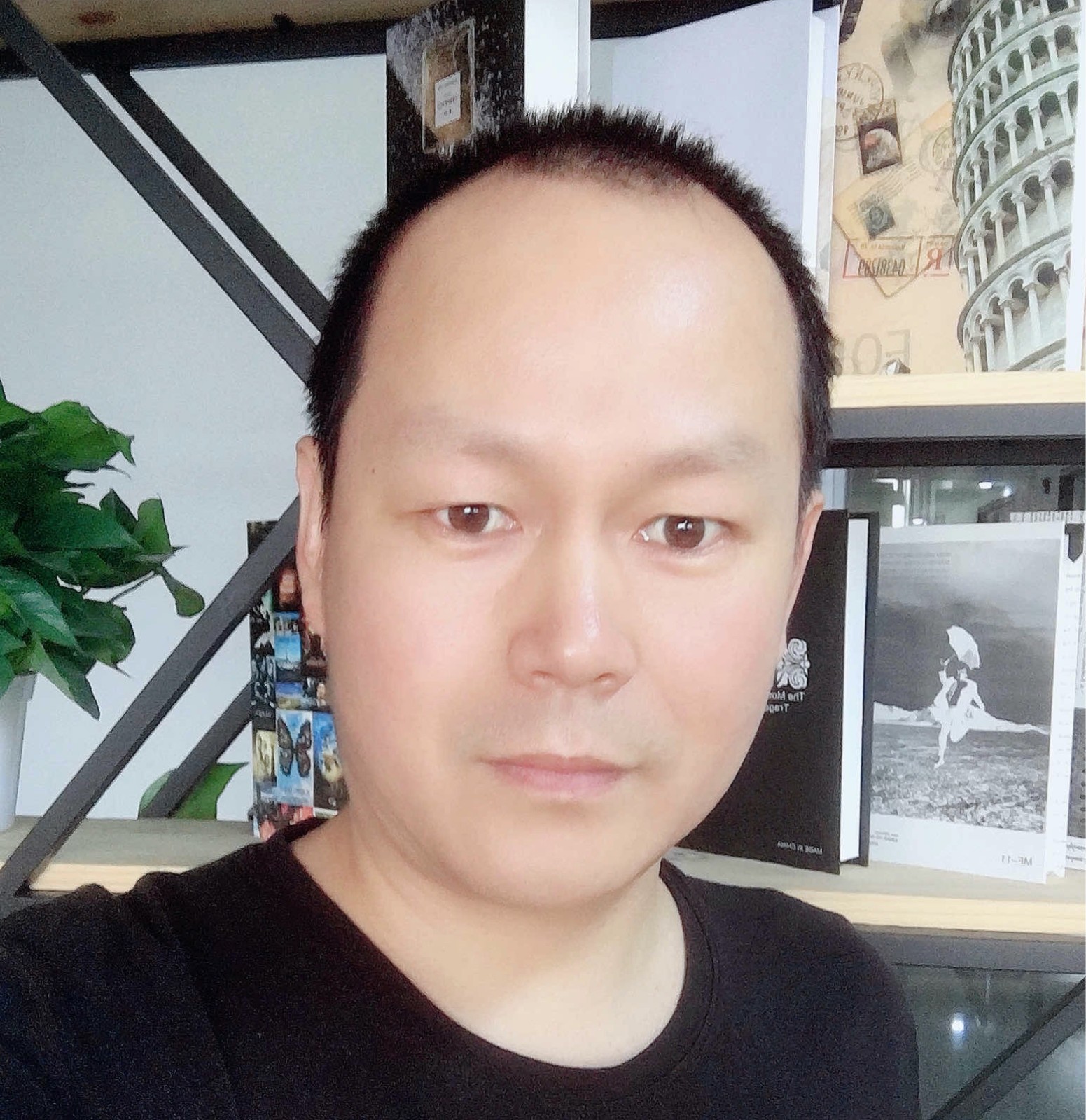
Correction status:qualified
Teacher's comments:代码整洁规范, 不错的, 少了一个总结
mysqli:只用于连接MySQL数据
mysqli对数据库的操作方法与PDO类似,可以通过query方法直接执行,也可以通过prepare()方法预处理
//配置数据库参数
$config = [
'host'=>'127.0.0.1',
'dbname'=>'www.merchant.office',
'username'=>'merchant',
'password'=>'merchant',
'charset'=>'utf8',
'port'=>'3306',
];
extract($config);//将关联数组扩展为变量,键为变量名,值为数组元素的值
$mysqli = new mysqli($host, $username, $password, $dbname);//连接数据库
if($mysqli->connect_errno)die('数据库连接失败:'.$mysqli->connect_errno);//判断是否连接失败,输出失败原因
$mysqli->set_charset($charset);
mysqli
中的query()
方法执行
$sql = "CREATE TABLE IF not EXISTS `goods`(
`id` int(10) NOT NULL auto_increment,
`name` VARCHAR(60) not null,
`model` VARCHAR(30) ,
`price` DECIMAL(8,2),
`number` VARCHAR(8),
`status` int(1) null DEFAULT 0 ,
PRIMARY KEY(`id`)
)ENGINE=INNODB DEFAULT CHARSET=utf8;";
var_dump($mysqli->query($sql));//执行并打印执行结果
foreach
循环把数组中的数据处理后insert
到数据表中
$goods=[['华为手机','P40','5999','300'],['小米手机','米10','2999','320'],['苹果手机','XR','8999','610'],
['华为手机','P30','3599','500'],['小米手机','米9','2099','300'],['苹果手机','8s','5999','110'],
];
$i=0;
$str = '';
//循环插入数据
foreach($goods as $value){
array_walk($value,function(&$item){
return $item = "'".$item."'";
});
$data = implode(",",$value);
$sql = "insert into `goods`(`name`,`model`,`price`,`number`) value({$data})";
//echo $sql,'<br>';
if($mysqli->query($sql)) echo '插入第:'.++$i.'条<br>';//打印插入的条数
}
//通过参数绑定方式新增数据
$sql = "insert into `goods`(`name`,`model`,`price`,`number`) value(?,?,?,?)";
$query = $mysqli->prepare($sql);
$name='oppo';$model='V8';$price='3599';$number='685';
$query->bind_param('ssss',$name,$model,$price,$number);
$query->execute();
echo $mysqli->insert_id;
mysqli
执行query()
方法后返回一个结果集对象,fetch_assoc()
、fetch_all()
、fetch_row()
等方法获取数据affected_rows
属性获取受影响的记录数
//查询数据
$sql = "select * from goods where id>2";
$res_fetch = $mysqli->query($sql);
var_dump($res);
print_r($res_fetch->fetch_assoc());//获取一条数据,返回关联数组
print_r($res_fetch->fetch_all(MYSQLI_ASSOC));//获取全部数据
print_r($res_fetch->fetch_row());//获取一条数据,返回索引数组
$res_fetch->fetch_array(MYSQLI_ASSOC);//每次获取一行,指针下移
//循环打印:
while($res = $res_fetch->fetch_array(MYSQLI_ASSOC)){
print_r($res);
}
//更新数据
$sql = "update `goods` set `status`=1 where id<4";
$mysqli->query($sql);
echo '受影响记录数:'.$mysqli->affected_rows;//受影响记录数:3
//删除数据
$sql = "delete from `goods` where id<5";
$mysqli->query($sql);
echo '受影响记录数:'.$mysqli->affected_rows;//受影响记录数:4
//关闭连接
mysqli_close($mysqli);