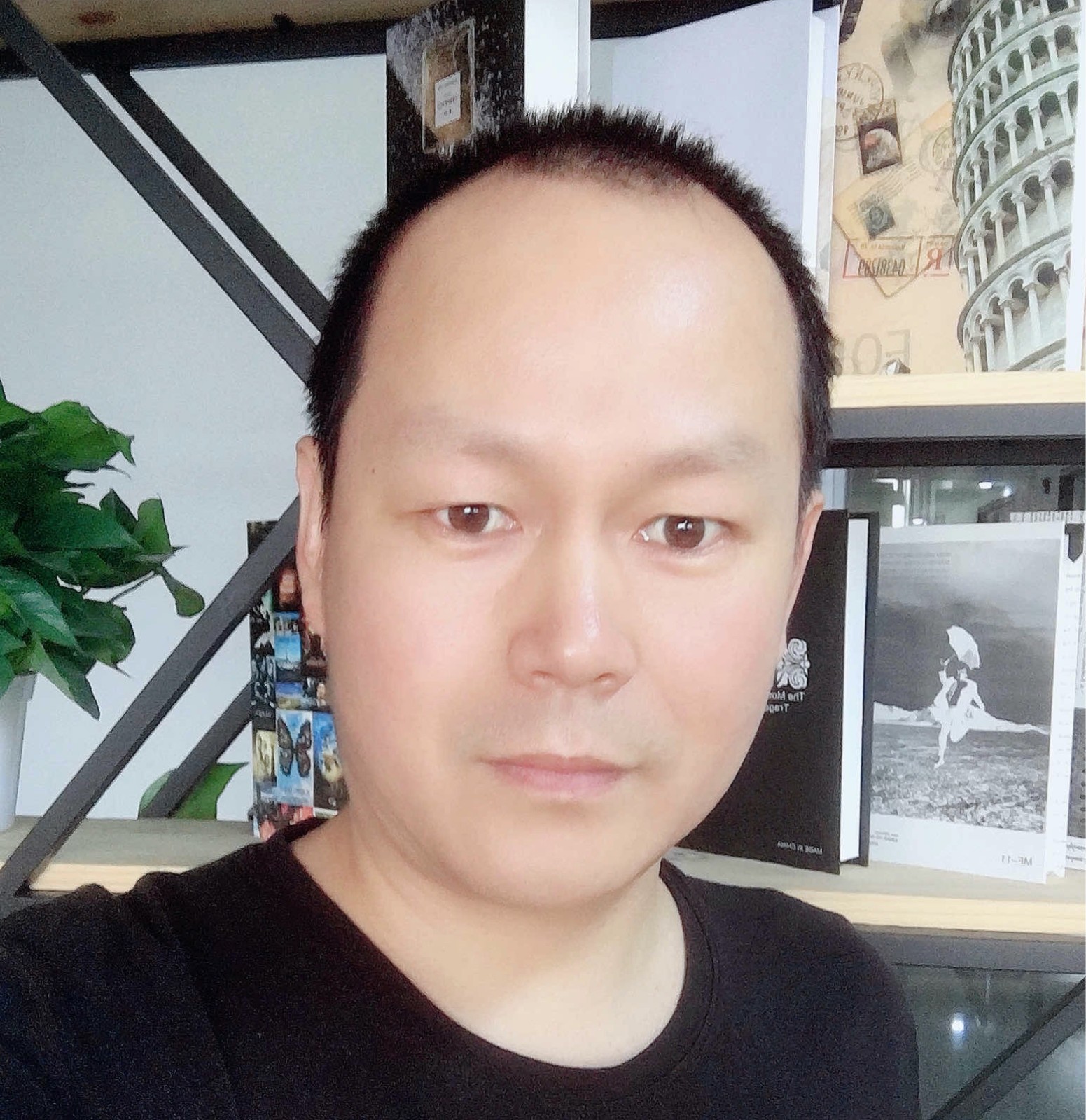
Correction status:qualified
Teacher's comments:这个案例写完, 是不是学到了不少新东西?
<?php
//检测是不是有 cookie 有的话就赋值给$user 没有就是空
isset($_COOKIE['user'])?$user=unserialize($_COOKIE['user']):null;
?>
<!doctype html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport"
content="width=device-width, user-scalable=no, initial-scale=1.0, maximum-scale=1.0, minimum-scale=1.0">
<meta http-equiv="X-UA-Compatible" content="ie=edge">
<title>爬宠乐园</title>
<style>
*{text-decoration: none;margin: 0;padding: 0;}
body{display: flex;flex-flow: column;font-weight: 800;}
header{ box-sizing: border-box;padding: 10px 30px;width: 100%;display: flex;flex-flow: row nowrap;justify-content: space-between; background: lightseagreen; }
/*.logo*/
header div:nth-of-type(2){color: #ffd012;text-align: center;line-height: 42px }
header div:nth-of-type(3){display: flex;justify-content: space-between;line-height: 42px }
header div:nth-of-type(3) a{color:#ffd012; }
</style>
</head>
<body>
<header>
<div class="logo"><h1>爬宠乐园</h1></div>
<div>一个程序员的爱好社区</div>
<div>
<?php if (isset($user)):?>
<a href=""><?php echo $user['name']?></a>
<a href="" id="logout">退出</a>
<?php else:?>
<a href="login.php">登陆</a>
<a href="register.php">注册</a>
<?php endif;?>
</div>
</header>
<script>
document.querySelector('#logout').addEventListener('click',function (event) {
if (confirm('确认退出吗?')) {
// 禁用默认行为, 其实就是禁用原<a>标签的点击跳转行为,使用事件中的自定义方法处理
event.preventDefault();
// 跳转到退出事件处理器
window.location.assign('handle.php?action=logout');
}
})
</script>
</body>
</html>
<?php
// 判断是否已登录
if (isset($_COOKIE['user']))
exit('<script>alert("请不要重复登录");location.href="index.php";</script>');
?>
?>
<!doctype html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport"
content="width=device-width, user-scalable=no, initial-scale=1.0, maximum-scale=1.0, minimum-scale=1.0">
<meta http-equiv="X-UA-Compatible" content="ie=edge">
<title>登录页面</title>
</head>
<body>
<div class="login">
<h3>提交</h3>
<form action="handle.php?action=login" method="post">
<div>
<label for="email"></label>
<input type="email" id="email" name="email" required >
</div>
<div>
<label for="password"></label>
<input type="password" id="password" name="password" required >
</div>
<div>
<button>提交</button>
</div>
</form>
</div>
</body>
</html>
<!doctype html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport"
content="width=device-width, user-scalable=no, initial-scale=1.0, maximum-scale=1.0, minimum-scale=1.0">
<meta http-equiv="X-UA-Compatible" content="ie=edge">
<title>登录页面</title>
</head>
<body>
<div class="login">
<h3>提交</h3>
<form action="handle.php?action=register" method="post" onsubmit="return compare()">
<div>
<label for="email"></label>
<input type="email" id="email" name="email" required >
</div>
<div>
<label for="password"></label>
<input type="password" id="password1" name="password1" required >
</div>
<div>
<label for="password"></label>
<input type="password" id="password2" name="password2" required >
</div>
<div>
<button>提交 </button><span id="tips"></span>
</div>
</form>
</div>
</body>
<script>
function compare(){
// var p1=document.getElementById('password1').value.trim();
// var p2=document.getElementById('password2').value.trim();
// // console.log(a);
if (document.forms[0].password1.value.trim() !== document.forms[0].password2.value.trim()) {
document.querySelector('#tips').innerText = '二次密码不相等';
return false;
}
}
</script>
</html>
<?php
$pdo=new PDO('mysql:host=localhost;dbname=phpedu','root','root');
$stmt=$pdo->prepare('SELECT * FROM `user`' );
$stmt->execute();
//fecthAll 是获取所有结果集PDO::FETCH_ASSOC是用关联的方式
$users=$stmt->fetchAll(PDO::FETCH_ASSOC);
//print_r($users);
$action=$_GET['action'];
//echo $_SERVER['REQUEST_METHOD'];
switch (strtolower($action)){
case 'login';
// $_SERVER['REQUEST_METHOD']判断是以什么方式提交上来的
if ($_SERVER['REQUEST_METHOD']==='POST'){
$name=$_POST['email'];
$password=$_POST['password'];
$results =array_filter($users ,function ($user ) use ( $name ,$password){
return $user['name']===$name&&$user['password']===$password;
});
if (count($results)===1){
setcookie('user',serialize(array_pop($results)));
exit('<script>alert("验证通过");location.href="index.php"</script>');
}else{
exit('<script>alert("请重新登陆");location.href="login.php"</script>');
}
}else{
die('请求类型错误');
}
break;
// 退出
case 'logout';
if (isset($_COOKIE['user'])){
setcookie("user", null, time() - 3600);
exit('<script>alert("已经退出");location.href="index.php"</script>');
}
break;
// 注册
case 'register';
$email=$_POST['email'];
$name=$_POST['name'];
$password=$_POST['password1'];
$register_time=time();
$sql="INSERT `user` SET `name`='{$name}',`email`='{$email}',`password`='{$password}',`register`='{$register_time}'";
$stmt=$pdo->prepare($sql);
if ($stmt->rowCount() === 1) exit('<script>alert("注册成功");location.assign("login.php")</script>');
else exit('<script>alert("注册失败");location.assign("login.php")</script>');
break;
default:
exit('未定义操作');
}
<?php
// 开启会话
session_start();
// 查询用户表中的数据
$pdo = new PDO('mysql:host=localhost;dbname=phpedu', 'root', 'root');
$stmt = $pdo->prepare('SELECT * FROM `users`');
$stmt->execute();
$users = $stmt->fetchAll(PDO::FETCH_ASSOC);
// 处理用户登录与注册
$action = $_GET['action'];
switch ( strtolower($action)) {
// 登录
case 'login':
// 判断请求是否合t法
if ($_SERVER['REQUEST_METHOD'] === 'POST') {
// 获取需要验证的数据
$email = $_POST['email'];
$password = sha1($_POST['password']);
$results = array_filter($users, function($user) use ($email, $password) {
return $user['email'] === $email && $user['password'] === $password;
});
if (count($results) === 1) {
$_SESSION['user'] = serialize(array_pop($results));
exit('<script>alert("验证通过");location.href="index.php"</script>');
} else {
exit('<script>alert("邮箱或密码错误,或者还没有帐号");location.href="login.php";</script>');
}
} else {
die('请求类型错误');
}
break;
// 退出
case 'logout':
if (isset($_SESSION['user'])) {
session_destroy();
exit('<script>alert("退出成功");location.assign("index.php")</script>');
}
break;
// 注册
case 'register':
// 1. 获取到所有新用户数据
$name = $_POST['name'];
$email = $_POST['email'];
$password = sha1($_POST['p1']);
$register_time = time();
// 2. 将新用户插入到表中
$sql = "INSERT `users` SET `name`='{$name}', `email`='{$email}', `password`='{$password}', `register_time`={$register_time}";
$stmt = $pdo->prepare($sql);
$stmt->execute();
if ($stmt->rowCount() === 1) exit('<script>alert("注册成功");location.assign("login.php")</script>');
else exit('<script>alert("注册失败");location.assign("login.php")</script>');
break;
// 未定义
default:
exit('未定义操作');
}
<?php
// 开启会话
session_start();
// 判断是否已经登录?
if (isset($_SESSION['user'])) $user = unserialize($_SESSION['user']);
?>
<!doctype html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport"
content="width=device-width, user-scalable=no, initial-scale=1.0, maximum-scale=1.0, minimum-scale=1.0">
<meta http-equiv="X-UA-Compatible" content="ie=edge">
<title>爬宠乐园</title>
<style>
*{text-decoration: none;margin: 0;padding: 0;}
body{display: flex;flex-flow: column;font-weight: 800;}
header{ box-sizing: border-box;padding: 10px 30px;width: 100%;display: flex;flex-flow: row nowrap;justify-content: space-between; background: lightseagreen; }
/*.logo*/
header div:nth-of-type(2){color: #ffd012;text-align: center;line-height: 42px }
header div:nth-of-type(3){display: flex;justify-content: space-between;line-height: 42px }
header div:nth-of-type(3) a{color:#ffd012; }
</style>
</head>
<body>
<header>
<div class="logo"><h1>爬宠乐园</h1></div>
<div>一个程序员的爱好社区</div>
<div>
<?php if (isset($user)):?>
<a href=""><?php echo $user['name']?></a>
<a href="" id="logout">退出</a>
<?php else:?>
<a href="login.php">登陆</a>
<a href="register.php">注册</a>
<?php endif;?>
</div>
</header>
<script>
document.querySelector('#logout').addEventListener('click',function (event) {
if (confirm('确认退出吗?')) {
// 禁用默认行为, 其实就是禁用原<a>标签的点击跳转行为,使用事件中的自定义方法处理
event.preventDefault();
// 跳转到退出事件处理器
window.location.assign('handle.php?action=logout');
}
})
</script>
</body>
</html>
<?php
// 开启会话
session_start();
// 判断是否已登录
if (isset($_SESSION['user']))
exit('<script>alert("请不要重复登录");location.href="index.php";</script>');
?>
<!doctype html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport"
content="width=device-width, user-scalable=no, initial-scale=1.0, maximum-scale=1.0, minimum-scale=1.0">
<meta http-equiv="X-UA-Compatible" content="ie=edge">
<title>登录页面</title>
</head>
<body>
<div class="login">
<h3>提交</h3>
<form action="handle.php?action=login" method="post">
<div>
<label for="email"></label>
<input type="email" id="email" name="email" required >
</div>
<div>
<label for="password"></label>
<input type="password" id="password" name="password" required >
</div>
<div>
<button>提交</button>
</div>
</form>
</div>
</body>
</html>