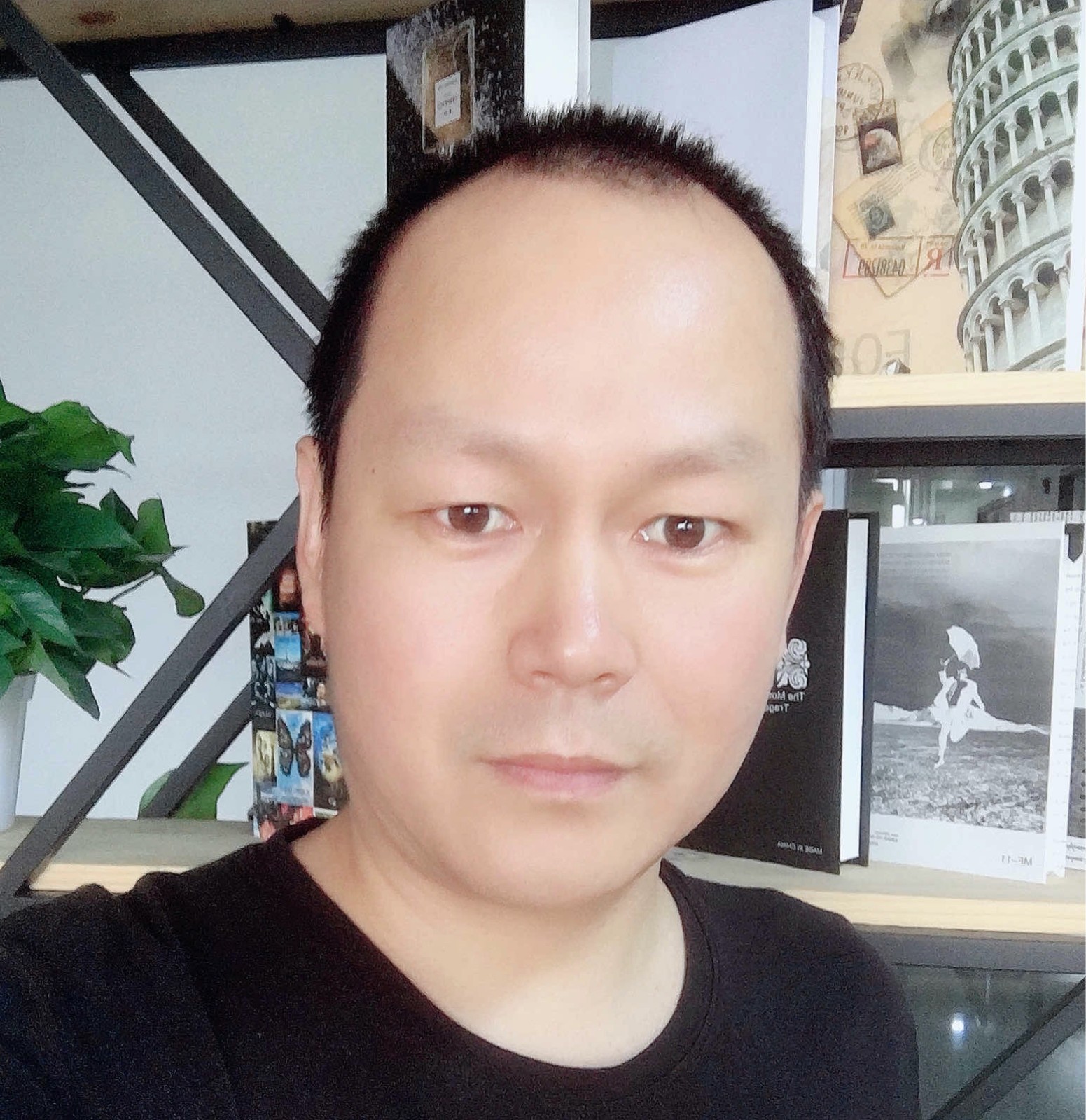
Correction status:qualified
Teacher's comments:sql语句绝对不止咱们课上讲得这些, 将全部语句讲一遍,即没必要也不现实, 所以, 课后还要多看看手册, 了解一些其它语句的功能
1.1连接参数
namespace pdo_edu;
return [
//1.数据库类型
'type'=>$type ?? 'mysql',
//2.数据库默认主机
'host'=>$host ?? 'localhost',
//3.默认数据库
'dbname'=>$dbname ?? 'phpedu' ,
//4.默认编码集
'charset'=>$charset ?? 'utf8',
'port'=>$port ?? '3306',
//5.默认用户名
'username'=>$username ?? 'root',
//6.默认密码
'password'=>$password ?? 'root',
];
1.2用pdo连接数据库
//设置命名空间
namespace pdo_edu;
use Exception;
use PDO;
//PDO连接数据库 加载参数
$config = require 'config/database.php';
//PDO连接数据库的三大要素
//数据源:DSN:数据库类型:host=数据库主机地址;dbname=默认的数据库名称;chart=...;port=...
//用户名:username
//用户密码:password
//拿到数据库连接的内容
$type = $config['type'];
$host = $config['host'];
$dbname = $config['dbname'];
$username = $config['username'];
$password = $config['password'];
//组装dsn
$dsn = sprintf('%s:host=%s;dbname=%s',$type,$host,$dbname);
//连接通常放到try中
try
{ //连接数据库
$pdo = new PDO($dsn,$username,$password);
} catch(Exception $e)
{
die($e->getMessage());
}
namespace pdo_edu;
use PDO;
//1.连接数据库
require 'connect.php';
//2.操作数据表
// $sql = 'SELECT 字段列表 FROM 数据表名称 WHERE 查询条件'
$sql = 'SELECT * FROM `staffs` WHERE `sex`=1';
//预处理对象,防止sql的注入
$stmt = $pdo->prepare($sql);
//使用预处理对象调用execute()执行sql语句
$stmt->execute();
//使用debugDumpParams()调试/查看
// var_dump($stmt->debugDumpParams());
//获取表中的一条记录
// $staff = $stmt->fetch(PDO::FETCH_ASSOC);
//查询全部
while($staff = $stmt->fetch(PDO::FETCH_ASSOC))
{
printf('<pre>%s</pre>',print_r($staff,true));
}
//多条查询
$staffs = $stmt->fetchAll(PDO::FETCH_ASSOC);
//结果是一个二维数组用foreach遍历
//3.关闭连接
unset($pdo);
namespace pdo_edu;
use PDO;
require 'connect.php';
//新增
// $sql = 'INSERT 表名 SET 字段...';
$sql = 'INSERT `staffs` SET `name`=?,`sex`=?,`phone`=?';
$stmt = $pdo->prepare($sql);
$data = ['Tim',1,15656565656];
$stmt->execute($data);
//判断是否执行成功
//$stmt->rowCount():返回写操作产生的受影响的记录数量
if ($stmt->rowCount()===1){
echo '新增成功,新增的主键是'.$pdo->lastInsertId();
}
unset($pdo);
namespace pdo_edu;
use PDO;
require 'connect.php';
// $sql = 'UPDATE 表名 SET 字段=新值 WHERE 更新条件 '
$sql = "UPDATE `staffs` SET `name`=? WHERE `id`=?";
$stmt = $pdo->prepare($sql);
$stmt->execute(['王老师',4]);
if ($stmt->rowCount()===1){
echo '更新成功';
}else{
echo'无记录被修改哦';
print_r($stmt->errorInfo());
}
unset($pdo);
namespace pdo_edu;
use PDO;
require 'connect.php';
// $sql = 'DELETE FROM 表名 WHERE 删除条件'
$sql = 'DELETE FROM `staffs` WHERE `id`=:id';
$stmt = $pdo->prepare($sql);
$id = filter_input(INPUT_GET,'id',FILTER_VALIDATE_INT);
$stmt->execute(['id'=>$_GET['id']]);
if ($stmt->rowCount()===1){
echo '删除成功';
}else{
echo '删除失败';
}
unset($pdo);
1.pdo操作数据库的步骤:连接数据库,操作数据库,关闭连接
2.增删改查insert,delete,update,select
3.预处理对象,防止sql的注入 $stmt = $pdo->prepare($sql)
4.使用预处理对象调用execute()执行sql语句 $stmt->execute();