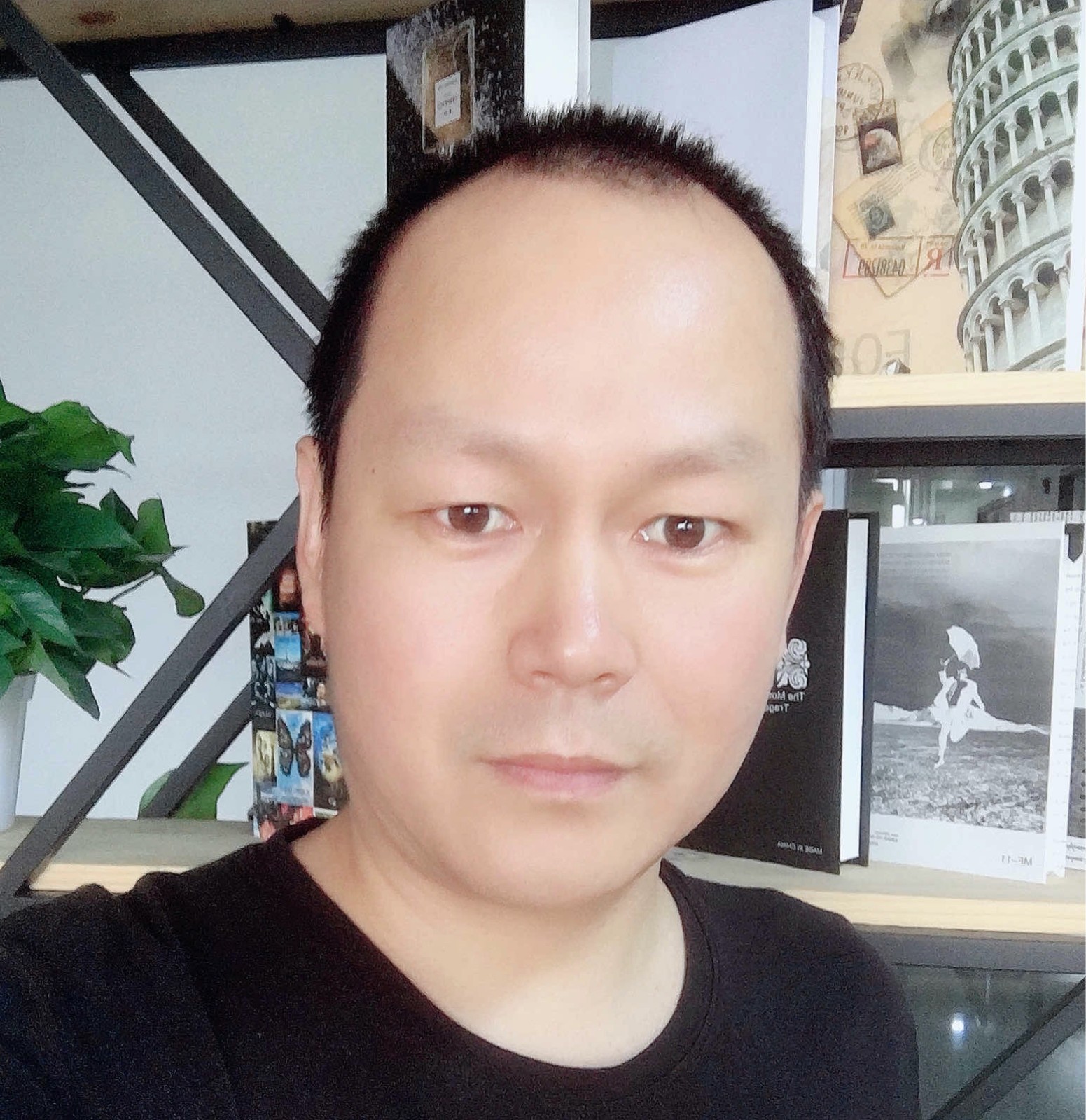
Correction status:qualified
Teacher's comments:mvc, 是不是没有想像中的难?
缩写 | 全拼 | 描述 | 备注 |
---|---|---|---|
M | Model | 模型 | 核心数据层,程序需要操作的数据放这里 |
V | View | 视图 | 提供给用户的操作页面 |
C | Controller | 控制器 | 根据用户从视图层的指令访问模型层,产生最终结果 |
namespace mvc_demo;
//模型类 :链接数据库
class Model
{
public function getData()
{
// 返回数据库的数据
return (new \PDO(‘mysql:host=localhost;dbname=php11.edu’,’php11.edu’,’php11.edu’))
->query(‘SELECT * FROM staffs
LIMIT 15’)
->fetchAll(\PDO::FETCH_ASSOC);
}
}
- 创建是视图类 View.php
```php
<?php
namespace mvc_demo;
//视图类
class View{
public function fetch($data){
$table = '<table>';
$table .= '<caption>员工信息表</caption>';
$table .= '<tr><th>ID</th><th>姓名</th><th>性别</th><th>职务</th><th>手机号</th><th>入职时间</th></tr>';
// 将数据循环遍历出来
foreach ($data as $staff) {
$table .= '<tr>';
$table .= '<td>' . $staff['id'] . '</td>';
$table .= '<td>' . $staff['name'] . '</td>';
$table .= '<td>' . ($staff['sex'] ? '男' : '女') . '</td>';
$table .= '<td>' . $staff['position'] . '</td>';
$table .= '<td>' . $staff['mobile'] . '</td>';
$table .= '<td>' . date('Y年m月d日', $staff['hiredate']) . '</td>';
$table .= '</tr>';
}
$table .= '</table>';
return $table;
}
}
echo '<style>
table {border-collapse: collapse; border: 1px solid;text-align: center; width: 500px;height: 150px;width: 600px;}
caption {font-size: 1.2rem; margin-bottom: 10px;}
tr:first-of-type { background-color:wheat;}
td,th {border: 1px solid; padding:5px}
</style>';
方法1
<?php
namespace mvc_demo;
//控制器1
// 加载模型类,视图类
require 'Model.php';
require 'View.php';
//创建控制
class Controller
{
public function index(){
// 获取数据
//实例化模型跟视图类
$model = new Model();
$data = $model->getData();
$view = new View();
return $view->fetch($data);
}
}
//实例化输出
echo (new Controller())->index();
//输出成功
方法2
<?php
namespace mvc_demo;
//控制器1
// 加载模型类,视图类
require 'Model.php';
require 'View.php';
//创建控制
class Controller
{
private $model;
private $view;
// 依赖注入点使用构造方法 这样可以实现代码的共用 比如类中有多个方法
public function __construct(Model $model, View $view)
{
$this->model = $model;
$this->view = $view;
}
//方法1
public function index(){
// 获取数据
$data = $this->model->getData();
return $this->view->fetch($data);
}
// 方法2
public function index1(){
// 获取数据
$data = $this->model->getData();
return $this->view->fetch($data);
}
}
//实例化输出
$model = new Model();
$view = new View();
echo (new Controller($model,$view))->index();
//输出成功
方法三
<?php
namespace mvc_demo;
//控制器1
// 加载模型类,视图类
require 'Model.php';
require 'View.php';
//服务容器
class Container
{
// 创建对象容器
protected $instances = [];
//绑定 向容器中添加一个类实例
public function bind($alias, \Closure $process)
{
$this->instances[$alias] = $process;
}
// 取出 从容器中取出一个类实例
public function make($alias, $params = [])
{
return call_user_func_array($this->instances[$alias],[]);
}
}
//创建类实例
$container = new Container();
//绑定
$container->bind('model',function (){return new Model();});
$container->bind('view',function (){return new View();});
//创建控制
class Controller
{
public function index(Container $container)
{
// 获取数据
$data = $container->make('model')->getData();
return $container->make('view')->fetch($data);
}
}
//实例化控制器类
$controller = new Controller();
echo $controller->index($container);
//输出成功
mvc 实现将逻辑,数据,界面分离 使同一个程序有不同的表现形式,易于程序维护和修改