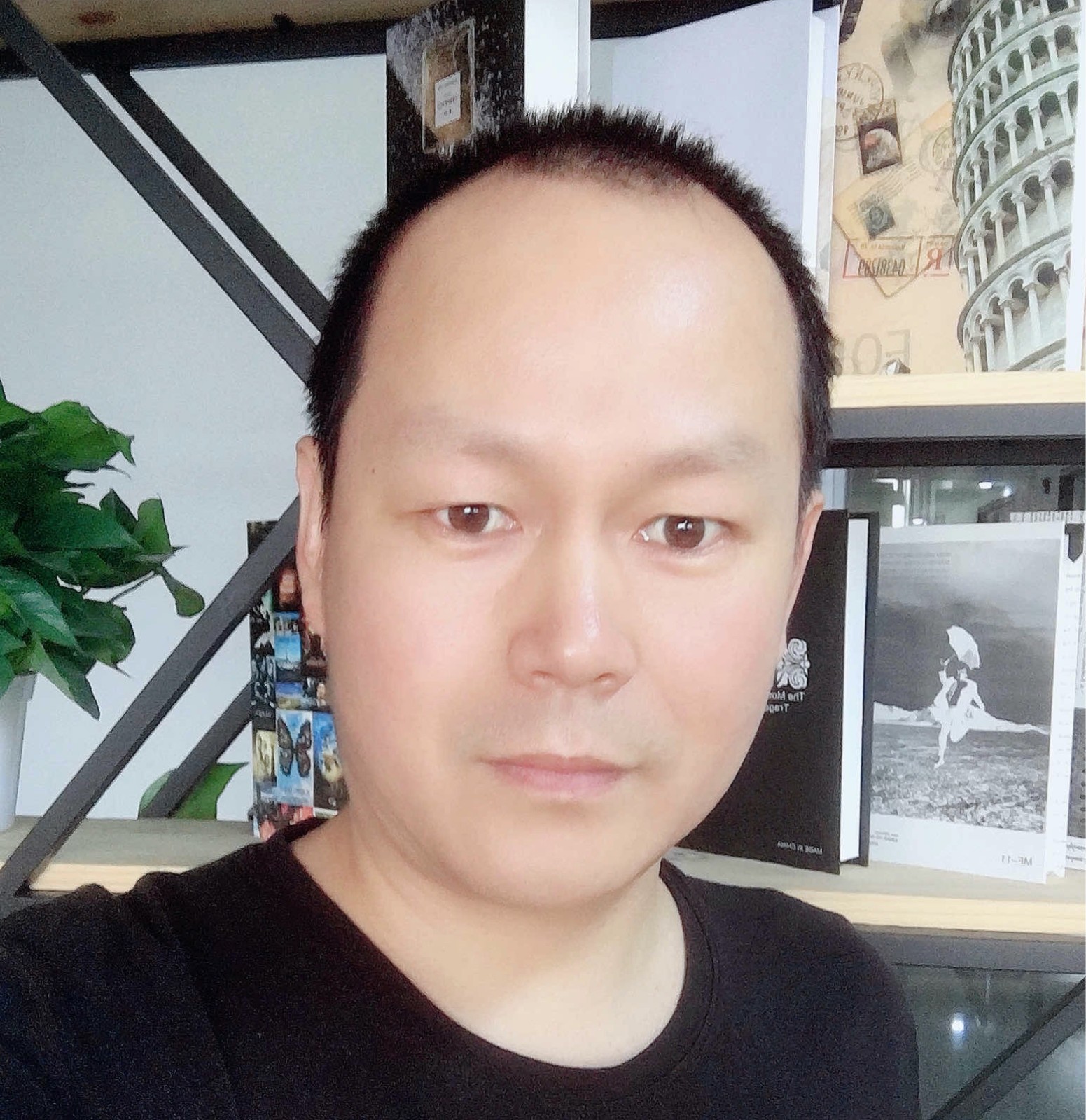
Correction status:qualified
Teacher's comments:写得不错, 忘了总结
<?php
namespace mvc_demo;
// 视图类
class View
{
public function fetch($data)
{
$table = '<table>';
$table .= '<caption>商品信息表</caption>';
$table .= '<tr><th>ID</th><th>名称</th><th>排序</th><th>父级商品</th></tr>';
// 将数据循环遍历出来
foreach ($data as $category) {
$table .= '<tr>';
$table .= '<td>' . $category['id'] . '</td>';
$table .= '<td>' . $category['name'] . '</td>';
$table .= '<td>' . ($category['sort'] ? '男' : '女') . '</td>';
$table .= '<td>' . $category['parentid'] . '</td>';
$table .= '</tr>';
}
$table .= '</table>';
return $table;
}
}
echo '<style>
table {border-collapse: collapse; border: 1px solid;text-align: center; width: 500px;height: 150px;width: 600px;}
caption {font-size: 1.2rem; margin-bottom: 10px;}
tr:first-of-type { background-color:wheat;}
td,th {border: 1px solid; padding:5px}
</style>';
// echo (new View)->fetch((new Model)->getData());
<?php
namespace mvc_demo;
use PDO;
use Exception;
//模型类,用于操作数据库
class Model
{
public function getData(){
$dsn= "mysql:host=127.0.0.1;dbname=phpedu";
try {
// 连接数据库
$pdo=new PDO($dsn,'root','root');
// var_dump($pdo);
} catch (Exception $e) {
die($e->getMessage());
}
return $pdo->query("select * from `category`")->fetchAll(PDO::FETCH_ASSOC);
}
}
<?php
namespace mvc_demo;
//1.加载模型类
require 'Model.php';
//2.加载视图类
require 'view.php';
//3.创建控制
class Controller2
{
public function index(Model $model, View $view)
{
$data = $model->getData();
return $view->fetch($data);
}
}
//客户端代码
//实例化控制器
$model = new Model;
$view = new View;
$controller = new Controller2;
echo $controller->index($model,$view);
<?php
namespace mvc_demo;
//1.加载模型类
require 'Model.php';
//2.加载视图类
require 'view.php';
//3.创建控制
class Controller3
{
private $model;
private $view;
public function __construct(Model $model,View $view)
{
$this->model = $model;
$this->view=$view;
}
public function index()
{
$data = $this->model->getData();
return $this->view->fetch($data);
}
}
//客户端代码
//实例化控制器
$model = new Model;
$view = new View;
$controller = new Controller3($model,$view);
echo $controller->index($model,$view);
<?php
namespace mvc_demo;
//1.加载模型类
require 'Model.php';
//2.加载视图类
require 'view.php';
//3.服务容器
class Container1
{
protected $instance = [];
//绑定:向对象容器中添加一个实例
public function bind($alias, \Closure $process)
{
$this->instance[$alias] = $process;
}
//取出:从容器中取出一个实例
public function make($alias, $param = [])
{
return call_user_func_array($this->instance[$alias], []);
}
public function destory($alias)
{
unset($this->instance[$alias]);
}
}
$container = new Container1;
$container->bind('model', function () {
return new Model;
});
$container->bind('view', function () {
return new View;
});
//3.创建控制
class Controller4
{
public function index(Container1 $container)
{
$data = $container->make('model')->getData();
return $container->make('view')->fetch($data);
}
}
//客户端代码
//实例化控制器
$model = new Model;
$view = new View;
$controller = new Controller4;
echo $controller->index($container);
<?php
namespace mvc_demo;
use mvc_demo\Model as M;
use mvc_demo\View as V;
//1.加载模型类
require 'Model.php';
//2.加载视图类
require 'view.php';
//3.服务容器
class Container2
{
protected $instance = [];
//绑定:向对象容器中添加一个实例
public function bind($alias, \Closure $process)
{
$this->instance[$alias] = $process;
}
//取出:从容器中取出一个实例
public function make($alias, $param = [])
{
return call_user_func_array($this->instance[$alias], []);
}
public function destory($alias)
{
unset($this->instance[$alias]);
}
}
$container = new Container2;
$container->bind('model', function () {
return new M;
});
$container->bind('view', function () {
return new V;
});
/////////////////////////////////
//4.Facade门面技术,可以接管对容器中的对象访问
Class Facade
{
protected static $container = null;
public static function initialize(Container2 $container){
static::$container = $container;
}
}
class Model extends Facade
{
protected static $data = [];
public static function getData(){
static::$data = static::$container->make('Model')->getData();
}
}
class View extends Facade
{
public static function fetch()
{
return static::$container->make('view')->fetch();
}
}
//////////////////////////////
//3.创建控制
class Controller5
{
public function __construct(Container2 $controller)
{
Facade::initialize($controller);
}
public function index()
{
$data = Model::getData();
return View::fetch($data);
}
}
//客户端代码
//实例化控制器
$container = new Container2;
$controller = new Controller5($container);
echo $controller->index();