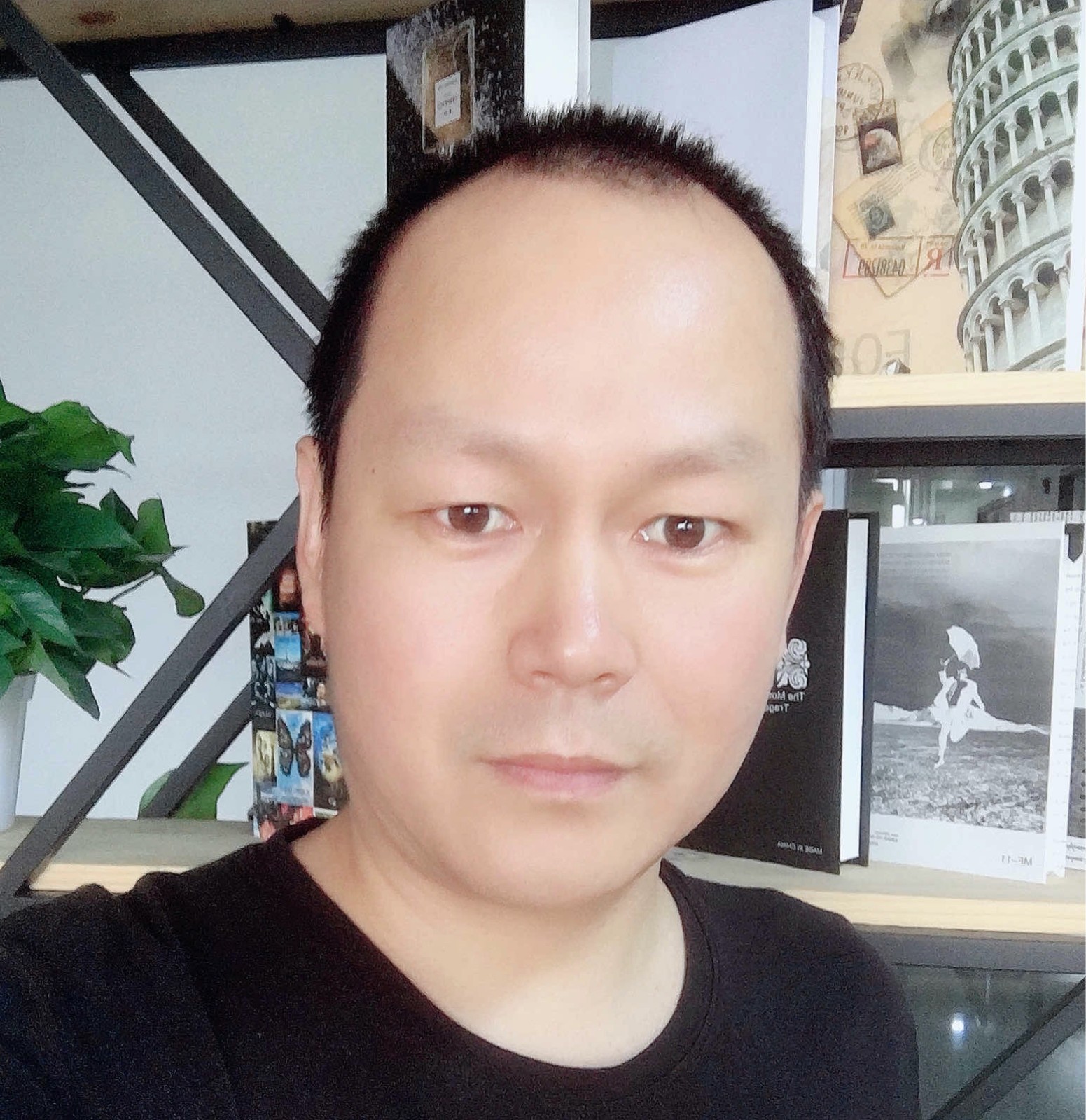
Correction status:qualified
Teacher's comments:轮播图点击后, 定时器顺序混乱了,检查一下为什么? 不过, 整体来说, 完成的相当棒, 其实理解了原理和流程, 实现起来并不难, 对不对?
一、JS实现选项卡
代码输出效果在线预览:
选项卡效果
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>选项卡</title>
<style>
* {
margin: 0;
padding: 0;
}
a {
text-decoration: none;
color: lightseagreen;
}
a:hover {
text-decoration: underline;
color: red;
}
li {
list-style: none;
}
li:hover {
cursor: default;
}
.tabs {
width: 300px;
height: 200px;
margin: 30px;
background-color: #efefef;
display: flex;
flex-direction: column;
}
.tab {
height: 36px;
display: flex;
}
.tab li {
flex: auto;
text-align: center;
line-height: 36px;
background-color: lightseagreen;
}
.tab li.active {
background-color: #ddd;
}
.item {
padding: 20px;
display: none;
}
.item.active {
display: block;
}
</style>
</head>
<body>
<div class="tabs">
<!-- 导航 -->
<ul class="tab">
<li class="active" data-index="1">声乐</li>
<li data-index="2">舞蹈</li>
<li data-index="3">器乐</li>
</ul>
<!-- 详情1 -->
<ul class="item active" data-index="1">
<li><a href="">美声唱法</a></li>
<li><a href="">通俗唱法</a></li>
<li><a href="">民族唱法</a></li>
</ul>
<!-- 详情2 -->
<ul class="item" data-index="2">
<li><a href="">古典舞</a></li>
<li><a href="">现代舞</a></li>
<li><a href="">民族舞</a></li>
</ul>
<!-- 详情2 -->
<ul class="item" data-index="3">
<li><a href="">古筝</a></li>
<li><a href="">琵琶</a></li>
<li><a href="">扬琴</a></li>
</ul>
</div>
<script>
// 导航:事件委托
var tab = document.querySelector(".tab");
// 获取列表
var items = document.querySelectorAll(".item");
// 给导航绑定点击事件
tab.addEventListener("click", show, false);
// 给导航绑定鼠标移动事件
tab.addEventListener("mouseover", show, false);
// 事件回调函数
function show(ev) {
console.log(ev.target);
// 1. 清空导航原有的激活状态
ev.target.parentNode.childNodes.forEach(function (item) {
if (item.nodeType === 1) item.classList.remove("active");
});
// 2. 将当前用户正在点击的导航设置为激活状态
ev.target.classList.toggle("active");
// 3. 清空列表原有的激活状态
items.forEach(function (item) {
item.classList.remove("active");
});
// 4. 在列表中查询data-index与导航的data-index相等的内容,将它设置为激活
items.forEach(function (item) {
if (item.dataset.index === ev.target.dataset.index)
item.classList.add("active");
});
}
</script>
</body>
</html>
输出效果:
二、JS实现轮播图
代码输出效果在线预览
轮播图效果
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<title>轮播图</title>
<style>
ul,
li {
margin: 0;
padding: 0;
list-style: none;
}
.box {
/*定位父级*/
position: relative;
width: 1000px;
height: 350px;
margin: 0 auto;
}
.box .slider {
width: 1000px;
height: 350px;
display: none;
}
.box .slider.active {
display: block;
}
.box .point-list {
position: absolute;
/*绝对定位的环境下的水平居中方式*/
left: 50%;
margin-left: -38px;
top: 310px;
}
.box .point-list .point {
display: inline-block;
width: 12px;
height: 12px;
margin: 0 5px;
background-color: white;
border-radius: 100%;
}
.box .point-list .point.active {
background-color: black;
}
.box .point-list .point:hover {
cursor: pointer;
}
.skip {
position: absolute;
top: 140px;
display: inline-block;
width: 40px;
height: 80px;
text-align: center;
line-height: 80px;
background-color: lightgray;
color: white;
opacity: 0.2;
font-size: 36px;
}
.box .prev {
left: 0;
}
.box .next {
right: 0;
}
.box .skip:hover {
cursor: pointer;
opacity: 0.5;
color: black;
}
</style>
</head>
<body>
<div class="box">
<img
src="banner/banner1.jpg"
alt=""
data-index="1"
class="slider active"
/>
<img src="banner/banner2.jpg" alt="" data-index="2" class="slider" />
<img src="banner/banner3.jpg" alt="" data-index="3" class="slider" />
<img src="banner/banner4.jpg" alt="" data-index="4" class="slider" />
<div class="point-list">
<!-- <span class="point active" data-index="1"></span>
<span class="point" data-index="2"></span>
<span class="point" data-index="3"></span> -->
</div>
<span class="skip prev"><</span>
<span class="skip next">></span>
</div>
<script>
// 获取轮播图片组
var imgs = document.querySelectorAll("img");
var pointList = document.querySelector(".point-list");
// 动态生成小圆点
imgs.forEach(function (img, index) {
var span = document.createElement("span");
if (index === 0) span.classList.add("point", "active");
span.classList.add("point");
// 将小圆点与当前显示的图片的索引进行关联
span.dataset.index = img.dataset.index;
pointList.appendChild(span);
});
// 为了正确的设置小圆点高亮显示, 这里需要获取到所有的小圆点
var points = document.querySelectorAll(".point");
// 事件代理/委托: 给小圆点添加点击事件
pointList.addEventListener(
"click",
function (ev) {
console.log(ev.target);
imgs.forEach(function (img) {
if (img.dataset.index === ev.target.dataset.index) {
imgs.forEach(function (img) {
img.classList.remove("active"); //移除原来图片上的激活样式
});
img.classList.add("active"); // 设置当前图片的激活样式
// 公共函数: 设置小圆点当前的高亮, 必须与图片一一对应, 或者同步
setPointActive(img.dataset.index);
}
});
},
false
);
// 公共函数:setPointActive
function setPointActive(imgIndex) {
// 清除原来的所有的小圆点上的高亮激活样式
points.forEach(function (point) {
point.classList.remove("active");
});
points.forEach(function (point) {
if (point.dataset.index === imgIndex) point.classList.add("active");
});
}
// 获取翻页按钮
var skip = document.querySelectorAll(".skip");
// 添加事件
skip.item(0).addEventListener("click", skipImg, false);
skip.item(1).addEventListener("click", skipImg, false);
// 翻页事件回调skipImg
function skipImg(ev) {
// 遍历并找到当前正在显示的图片
var currentImg = null;
imgs.forEach(function (img) {
if (img.classList.contains("active")) currentImg = img;
});
// 1. 判断是否是点击了显示前一张
if (ev.target.classList.contains("prev")) {
currentImg.classList.remove("active");
currentImg = currentImg.previousElementSibling;
console.log(currentImg);
// 如果存在前一张
if (currentImg !== null && currentImg.nodeName === "IMG")
// 将当前图片显示
currentImg.classList.add("active");
else {
//否则就显示最后一张,形成循环
currentImg = imgs[imgs.length - 1];
currentImg.classList.add("active");
}
}
// 2. 判断是否是点击了显示下一张
if (ev.target.classList.contains("next")) {
currentImg.classList.remove("active");
currentImg = currentImg.nextElementSibling;
console.log(currentImg);
// 如果存在前一张
if (currentImg !== null && currentImg.nodeName === "IMG")
// 将当前图片显示
currentImg.classList.add("active");
else {
//否则就显示最后一张,形成循环
currentImg = imgs[0];
currentImg.classList.add("active");
}
}
// 公共函数: 设置小圆点当前的高亮, 必须与图片一一对应, 同步
setPointActive(currentImg.dataset.index);
}
//获取整个轮播图
var box = document.querySelector(".box");
//创建定时器
var timer = null;
// 1. 当鼠标移出轮播图区域时, 启动定时器,每2秒自动切换图片
box.addEventListener("mouseout", startTimer, false);
// 2. 当鼠标移入轮播图区域时,清除定时器,由用户点击操作
box.addEventListener("mouseover", clearTimer, false);
// 启动定时器
function startTimer() {
var click = new Event("click");
//setInterval(): 间歇式执行
setInterval(function () {
skip.item(1).dispatchEvent(click);
}, 2000);
}
// 清除定时器
function clearTimer() {
clearInterval(timer);
}
</script>
</body>
</html>
输出效果:
三、总结
平时浏览网页常见的选项卡和轮播图效果,原来实现起来的步骤确实简单,但是切记每实现一个步骤的代码要及时打印检查,及时纠正,之前学习过的零散知识点,只是死记着,做案例时才明白原来是要用在哪里。真的是理论来源于实践,实践反过来又验证了理论。