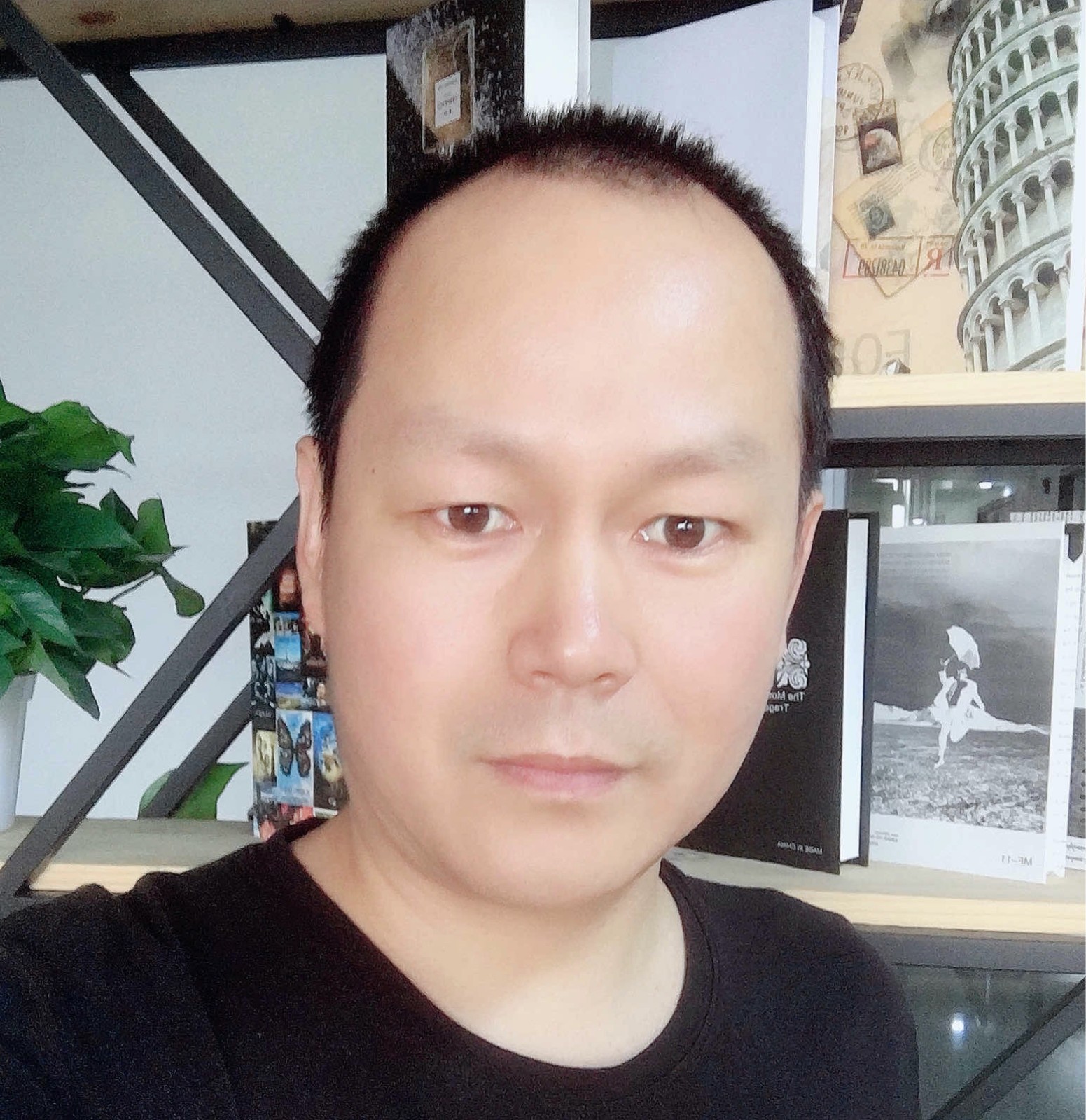
Correction status:qualified
Teacher's comments:作业名称后面的不要这么长的
//高级数据库链式调用查询方式
public function finds()
{
$res = DB::table('article')->where('id', 2)->first();
echo '<pre>';
print_r($res);
}
//多条查询
public function list()
{
// 返回的是框架自带的集合对象数据
// 返回全部数据
$res = DB::table('article')->get();
//返回指定字段内容
$res = DB::table('article')->select('cate_id', 'title')->get();
//给返回的字段名称起个别名
$res = DB::table('article')->select('cate_id as cid', 'title')->get();
//添加查询条件 栏目ID=5
$res = DB::table('article')->select('cate_id as cid', 'title')->where('cate_id', 5)->get();
//添加查询条件 栏目ID<5 带运算符的查询会影响MYSQL的速度,大数据并发时容易死机
$res = DB::table('article')->select('cate_id as cid', 'title')->where('cate_id', '<', 5)->get();
echo '<pre>';
// print_r($res);
// 转为数组
print_r($res->all());
}
//查询词查询
public function likes()
{
// 查询包含 风格 的信息 在查询词的前后加上% 但这种会影响MYSQL速度 只在查询词的后面加% 表示查询以查询词开头的信息
$res = DB::table('article')->where('title', 'like', '%风格%')->get()->all();
// select * from article where title like '%风格%' and cate_id=6
// 增加查询条件 栏目ID=6
$res = DB::table('article')->where('title', 'like', '%风格%')->where('cate_id', 6)->get()->all();
//如果需要查询 栏目ID=6 或 栏目ID=1 的信息
// select * from article where cate_id=6 or cate_id=1
// 查看生成的SQL语句 toSql()
// $res = DB::table('article')->where('cate_id',6)->orWhere('cate_id',1)->toSql();
$res = DB::table('article')->where('cate_id', 6)->orWhere('cate_id', 1)->get()->all();
echo '<pre>';
print_r($res);
}
//where in 查询 重点
public function wherein()
{
// select * from article where id in (1,2,5)
$res = DB::table('article')->whereIn('id', [1, 2, 5])->get()->all();
echo '<pre>';
print_r($res);
}
//连表查询
public function joins()
{
//查询文章表中的作者ID和用户表中的用户ID相同的
// $res = DB::table('article')->join('users', 'users.id', '=', 'article.uid')->get()->all();
// 上面的语句文章的ID会被覆盖 ,所以要给他添加条件
$res = DB::table('article')->join('users', 'users.id', '=', 'article.uid')->select('article.id', 'article.cate_id', 'article.title', 'users.username as nickname')->get()->all();
// echo '<pre>';
// print_r($res);
// 将数据在视图中渲染显示
$data['list'] = $res;
// echo '<pre>';
// print_r($data);
return view('mytest', $data);
}
//计算所有文章的平均浏览量
public function pvs()
{
// 1.取出所有文章数据
$res = DB::table('article')->get()->all();
// 2.计算平均值
$avg = 0;
// 3.计算总和
foreach ($res as $key => $value) {
$avg += $value->pv;
}
// 4.计算平均
$avg = $avg / count($res);
// 5.取整数
$avg = intval($avg);
echo '<pre>';
// print_r($avg);
// mysql 自带聚合函数查询平均浏览量
// select AVG(pv) from article
$res = DB::table('article')->avg('pv');
//强制转换为整数
$res = (int)$res;
// print_r($res);
// mysql 自带聚合函数查询总浏览量
$res = DB::table('article')->sum('pv');
//强制转换为整数
$res = (int)$res;
print_r($res);
echo '<hr>';
// mysql 自带聚合函数查询最小浏览量 最大用max 记录数count()不用参数
$res = DB::table('article')->min('pv');
//强制转换为整数
$res = (int)$res;
print_r($res);
}
// 增加数据
public function insert2()
{
//单条
// $res = DB::table('article')->insert(array('uid'=>2,'cate_id'=>5,'title'=>'insert测试','pv'=>0));
//一次增加多条
$item = array('uid' => 2, 'cate_id' => 5, 'title' => 'insert测试', 'pv' => 0);
$item2 = array('uid' => 3, 'cate_id' => 2, 'title' => '第二条insert测试', 'pv' => 10);
$data[] = $item;
$data[] = $item2;
$res = DB::table('article')->insert($data);
var_dump($res);
}
// 增加数据并返回主键ID
public function insert3()
{
//单条
$res = DB::table('article')->insertGetId(array('uid' => 2, 'cate_id' => 5, 'title' => 'insert测试', 'pv' => 0));
var_dump($res);
}
// 修改数据
public function update2()
{
// 修改单条
// $res = DB::table('article')->where('id',14)->update(array('title'=>'update测试'));
// 修改多条
$res = DB::table('article')->whereIn('id', [15, 16])->update(array('title' => 'update测试'));
var_dump($res);
}
// 删除数据
public function delete2()
{
// 删除一条
// $res = DB::table('article')->where('id',17)->delete();
// 删除多条
$res = DB::table('article')->whereIn('id', [15, 16])->delete();
var_dump($res);
}
// 模型 取出所有数据
//第一种方法:需要将数据表改成复数的,但可能这个表其他地方也在用
public function mymodels(Article $article){
$res = $article->get()->toArray();
echo '<pre>';
print_r($res);
}
//第二种方法:在模型类中指定查询的表名
}
namespace App;
use Illuminate\Database\Eloquent\Model;
class Article extends Model
{
//第二种方法:在模型类中指定查询的表名
//格式
protected $table = 'article';
}
数据库链式查询:
1.带运算符的查询会影响MYSQL的速度,大数据并发时容易死机
2.在查询词的前后加上% 但这种会影响MYSQL速度 只在查询词的后面加% 表示查询以查询词开头的信息
模型: 创建方法
1.XXXX 代表控制器名称,首字母要大写php artisan make:controller XXXX
2.XXXX 代表模型名称,首字母要大写php artisan make:model XXXX
模型: 取出所有数据
第一种方法:需要将数据表改成复数的,但可能这个表其他地方也在用
第二种方法:在模型类中指定查询的表名,格式: protected $table = 'article';