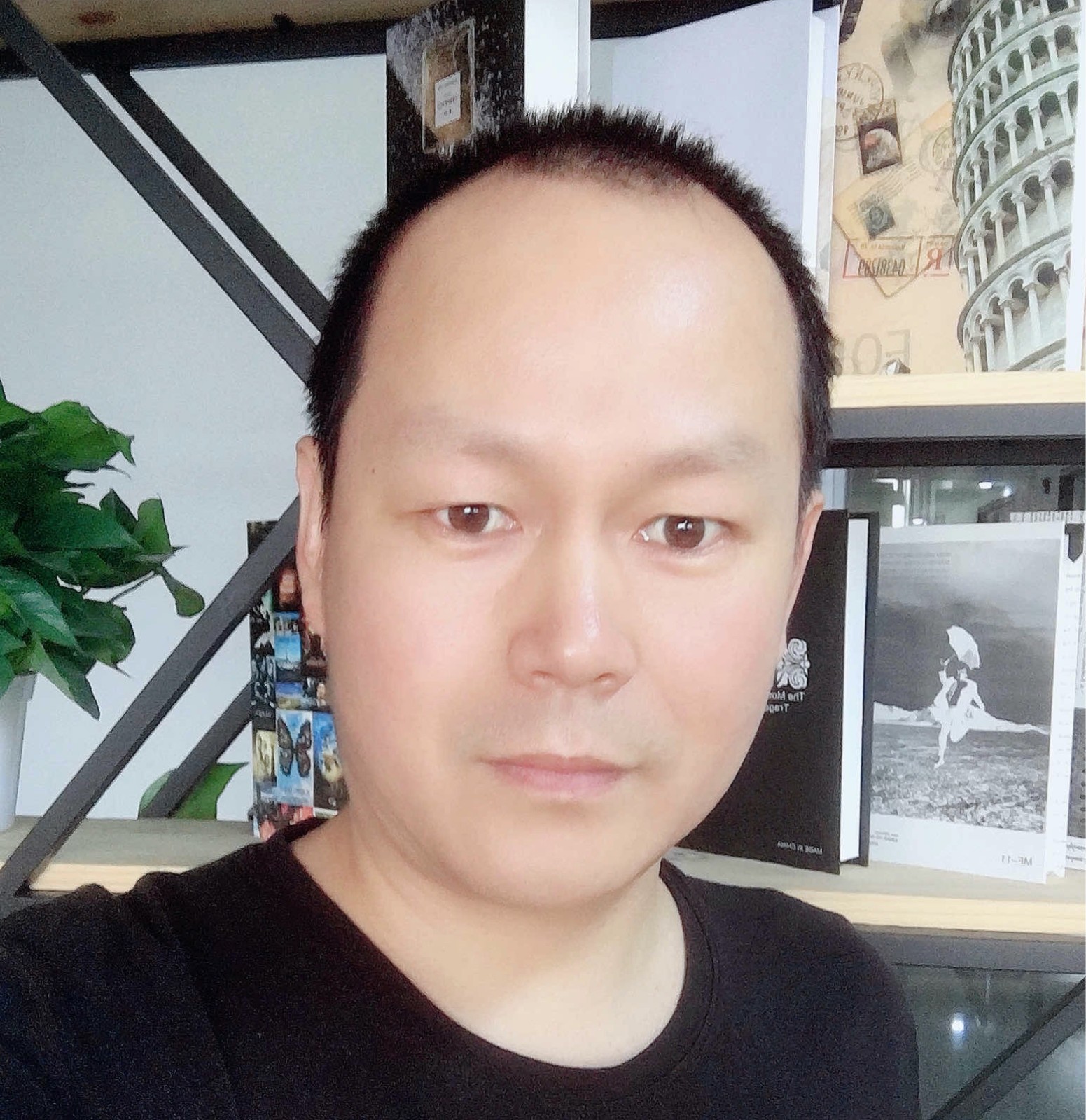
Correction status:qualified
Teacher's comments:很认真
一、JavaScript数据类型:
1- 原始类型:number(数值),string(字符串),boolean(布尔值)
var age = 18; var username = "admin"; var aaa = true; console.log(typeof age, typeof username, typeof aaa);
2-特殊类型:null(空), undefined(空/无)
var res; var res = undefined; console.log(role); var title = null; console.log(title); //null,undefine都有空/无的意义 console.log(null == undefined); //null 表示空对象 //undefined 专用于表示非对象类型变量的空/无 console.log(typeof null); if (!null) console.log(null + "是无"); if (!undefined) console.log(undefined + "也是无"); //null转为字符串是就是它本体null //null转为数值型为0 console.log(null + 100); console.log(0 + 100); //undefined---->NaN(not a Number) console.log(undefined + 100); //undefined弥补了null的不足
3- 对象类型:object(对象),array(数组),function(函数)
1)-对象(Object)
//js中的数组类似于php中的索引数组,js中的对象类似于php中的:关联数组 var people = { id: 1, name: "emy", age: "18", "jieguo": { chines: 90, computer: 98, }, }; console.log(people); console.table(people); //访问对象成员/属性,使用点操作符,php使用 -> console.log(people.age); console.log(people["jieguo"]); console.table(people["jieguo"]); console.table(people["jieguo"].js); // 遍历对象 for (key in people) // for (对象的键名 in 对象){ console.log(people[key]);// 对象[键名] } //借助数组的forEach()进行遍历 //第一步获取键名数组 var keys = Object.keys(people); console.log(keys); keys.forEach(function (item, index, arr) { console.log(this[item]); }, people);
2)-数组(Array)
var nations = ["china", "korea", "usa", "japan", "russian"]; //正确判断数组类型的方式 console.log(Array.isArray(nations)); //遍历一个数组 for (var i = 0; i < nations.length; i++) { console.log(nations[i]); } //php 中获取数组部分元素slice(),js也有 console.log(nations.slice(0, 3)); console.log(nations.slice(2, 3)); console.log(nations.slice(0)); console.log(nations); //php,splice(),可以实现数组的插入,替换,删除,js也有splice nations.splice(1, 0, "german", "friance"); //插入元素 console.log(nations); nations.splice(3, 2, "newland", "iriland"); //替换元素 console.log(nations); nations.splice(2, 2); console.log(nations); var res = nations.splice(2, 2); //删除后返回的值 console.log(res); console.log(nations);
3)-函数(function)
function c1(a, b) { console.log(a + "+" + b + "=", a + b); } c1(100, 10); //匿名函数,函数表达式 var c2 = function (a, b) { console.log(a + "+" + b + "=", a + b); }; c2(100, 60); // 立即调用函数 (function c1(a, b) { console.log(a + "+" + b + "=", a + b); })(1, 5);
二、JS对象
1-值以名称:值对的方式来书写(名称和值由冒号分隔)。
2-访问对象属性,访问对象成员使用 . 点操作符
方式一:objectName.propertyName
方式二:objectName["propertyName"]
3-如何遍历对象
方法一:JavaScript for...in 循环
语法:
for (对象的键名 in 对象) { 要执行的代码; }
方法二,把对象转为数组
1),获取键名数组
var keys = Object.keys(需要遍历的对象);
2)把新的键名组成的数组遍历出来
keys.forEach(function (value, index, arr) { console.log(this[value]); }, 需要遍历的对象);
三、JSON知识
1-JavaScript JSON:是存储和传输数据的格式,经常在数据从服务器发送到网页时使用。
2-JSON 语法:JSON 支持三种数据类型: 简单值, 对象, 数组。
3-JSON对象
1)没有变量声明: JSON中没有变量概念
2)没有分号: 因为JSON不是JS语句
3)属性名: 任何时候都必须添加双引号, 且必须是双引号
4)JSON属性值也支持复杂类型,如对象
4-JSON数组:JSON 数组采用了原生 JS 中的数组字面量语法
// 原生JS数组 var product = [101, "电脑", 9800]; // JSON表示: 无变量和分号 [101, "电脑", 9800][ // 最常见场景是将数组与对象组合表示更加复杂的数据类型 ({ id: 101, name: "电脑", price: 9800, }, { id: 102, name: "手机", price: 4500, }) ]; // 许多软件的配置文件都是采用这种数据格式,如VSCODE ```
5-JSON 解析与序列化:
1)ES5 提供了内置全局对象**JSON**,对 JSON 数据提供了全面支持.
JSON.stringify(): 将原生 JS 对象,序列化为 JSON 字符串,用于存储与传输.
var person = { name: "Peter Zhu", age: 29, isMarried: true, course: { name: "JavaScript", grade: 99, }, getName: function () { return this.name; }, hobby: undefined, toString: function () { return "继承属性"; }, }; var jsonStr = JSON.stringify(person); console.log(jsonStr);
序列化后的结果为:
{ "name": "Peter Zhu", "age": 29, "isMarried": true, "course": { "name": "JavaScript", "grade": 99 } }
2) JSON.parse(): 将 JSON 字符串,解析为原生 JS 对象.
var jsonStr = JSON.stringify(person, function (key, value) { switch (key) { case "age": return "lady's age is screts."; case "isMarried": return undefined; // 必须要有default, 才可以输出其它未被处理的属性 default: return value; } });
四、总结:以前对于JS效果只会是拿来主义多,这一节课终于系统地了解了JS数据类型,对象和数组的方法;JS流程控制;JSON.stringify()方法参数用法。