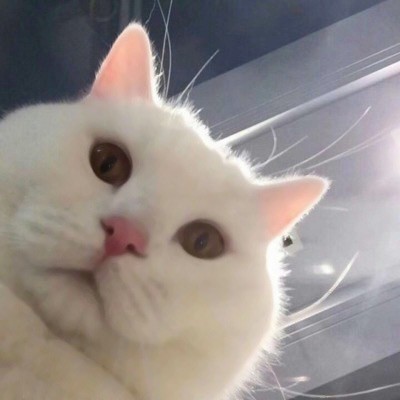
Correction status:qualified
Teacher's comments:总体写的还行,要注意作业的规范,尤其是代码部分要使用代码块!
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>选择器:简单选择器</title>
<style>
/ 使用九宫格演示:grid布局实现 /
.container {
width: 400px;
height: 400px;
display: grid;
grid-template-columns: repeat(3, 1fr);
gap: 5px;
}
.item {
font-size: 2rem;
background-color: rgb(161, 127, 255);
display: flex;
justify-content: center;
align-items: center;
}
/ 元素选择器,也就是标签选择器 /
body {
background-color: beige;
}
/ 类选择器:对应着html标签中的class属性 /
.item{
border: 1px solid black;}
/ 复合类样式,多个类复合应用 /
.item.center{
background-color: cadetblue;}
/ / id选择器 ,id,class可以添加到任何元素上,可以省略 /
#first{
background-color: crimson;
}
#first{
background-color: rgb(33, 151, 78);
}
.item#first{
background-color: rgb(71, 78, 74);
}
/ id,class可以添加到任何元素上,所以可以省略 /
/ 层叠样式表,相同元素后面的样式可以覆盖前面的样式,比如上面第2可以代替掉1 /
/ 第二个的属于元素级别,第三个属于id级别,所以优先级<class<id */
</style>
</head>
<body>
<div class="container">
<div class="item" id="first">1</div>
<div class="item">2</div>
<div class="item">3</div>
<div class="item">4</div>
<div class="item center">5</div>
<div class="item">6</div>
<div class="item">7</div>
<div class="item">8</div>
<div class="item">9</div>
</div>
</div>
</body>
</html>
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>上下文选择器</title>
<style>
.container {
width: 400px;
height: 400px;
display: grid;
grid-template-columns: repeat(3, 1fr);
gap: 5px;
}
.item {
font-size: 2rem;
background-color: aquamarine;
display: flex;
justify-content: center;
align-items: center;
}
/ 1.后代选择器: 空格 /
.container div {
border: 1px solid rgb(25, 26, 24);
}
/ 2.父子选择器:> /
body > div {
border: 5px solid red;
}
/ 3.同级相邻选择器 /
.item.center + .item {
background-color: green;
}
/ 4.同级所有选择器 /
.item.center ~ .item {
/ background-color: hotpink; /
}
</style>
</head>
<body>
<div class="container">
<div class="item" id="first">1</div>
<div class="item">2</div>
<div class="item">3</div>
<div class="item">4</div>
<div class="item center">5</div>
<div class="item">6</div>
<div class="item">7</div>
<div class="item">8</div>
<div class="item">9</div>
</div>
</body>
</html>
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>结构伪类选择器:不分组(不区分元素类型</title>
</head>
<style>
.container {
width: 400px;
height: 400px;
display: grid;
grid-template-columns: repeat(3, 1fr);
gap: 5px;
}
.item {
font-size: 2rem;
background-color: aquamarine;
display: flex;
justify-content: center;
align-items: center;
}
/ 匹配第一个子元素 /
.continer > :first-child {
background-color: lavender;
}
/ 最后一个子元素 /
.continer > :last-child {
background-color: lightcoral;
}
/ 选第三个子元素 /
.continer > :nth-child(3) {
background-color: mediumslateblue;
}
/ 只选择偶数单元格,可以用even代替2n /
.continer > :nth-child(2n) {
background-color: midnightblue;
}
/ 只选择奇数单元格 ,可以用odd代替2n-1/
.continer > :nth-child(2n-1) {
background-color: moccasin;
}
/ 从指定位置开始选择剩下所有元素 /
.continer > item:nth-child(n + 4) {
background-color: navy;
}
/ 只取前3个 /
.continer > item:nth-child(-n + 3) {
background-color: palevioletred;
}
/ 只取最后3个 /
.continer > item:nth-last-child(-n + 3) {
background-color: maroon;
}
/ 取第八个,用倒数的方式更快 /
.continer > item:nth-last-of-type(2) {
background-color: limegreen;
}
/ n出现在表达式中的时候从0开始计算,得到非法索引位置的时候停止 /
</style>
<body>
<div class="container">
<div class="item" id="first">1</div>
<div class="item">2</div>
<div class="item">3</div>
<div class="item">4</div>
<div class="item">5</div>
<div class="item">6</div>
<div class="item">7</div>
<div class="item">8</div>
<div class="item">9</div>
</div>
</body>
</html>
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>结构伪类选择器:分组(不区分元素类型)</title>
<style>
.container {
width: 400px;
height: 400px;
display: grid;
grid-template-columns: repeat(3, 1fr);
gap: 5px;
}
.item {
font-size: 2rem;
background-color: aquamarine;
display: flex;
justify-content: center;
align-items: center;
}
/ 分组结构伪类分两步: /
/ 1.元素按类型进行分组 /
/ 2.分组中跟据索引(位置)进行选择 /
.container span:last-of-type {
background-color: limegreen;
/ 显示结果是span里的最后一个,9 /
}
/ 在分组中匹配任何一个 /
.container span:nth-of-type(3) {
background-color: mediumslateblue;
/ 显示结果是span里的第三个,6 /
}
/ 最后3个 /
.container span:last-of-type(-n + 3) {
background-color: navajowhite;
}
/ 前3个 /
.container span:nth-of-type(3) {
background-color: lightslategray;
}
</style>
</head>
<body>
<div class="container">
<div class="item" id="first">1</div>
<div class="item">2</div>
<div class="item">3</div>
<span class="item">4</span>
<span class="item">5</span>
<span class="item">6</span>
<span class="item">7</span>
<span class="item">8</span>
<span class="item">9</span>
</div>
</body>
</html>
选择器 | 说明 |
---|---|
:target | 用于选取当前活动的目标元素。当 URL 末尾带有锚名称 #,就可以指向文档内某个具体的元素。这个被链接的元素就是目标元素(target element)。它需要一个 id 去匹配文档中的 target |
:not | 选择器匹配每个元素是不是指定的元素/选择器 |
:before | 选择器向选定的元素前插入内容。使用content 属性来指定要插入的内容。 |
:after | 选择器向选定的元素之后插入内容。 使用content 属性来指定要插入的内容。 |
:focus | 选择器用于选择具有焦点的元素。 |
<style>
#example:target {
background: lightpink;
}
</style>
<a href="#example">点击为div添加背景</a>
<div id="example">DIV</div>
<style>
/ 在h1前面插入文字 /
.example>h1::before {
content: “插入文字 “;
}
</style>
<div class="example">
<h1>aaaaaaaaaaa</h1>
<p> 11111111</p>
<p> 22222222</p>
<div>3333333333</div>
<a href=" " target="_blank">444444444444</a>
</div>
<style>
/ 在h1后面插入文字 /
.example>h1::after {
content: “插入文字 “;
}
</style>
<div class="example">
<h1>aaaaaaaaaaa</h1>
<p> 11111111</p>
<p> 22222222</p>
<div>3333333333</div>
<a href=" " target="_blank">444444444444</a>
</div>
<style>
/ 为文本框添加焦点样式 /
input:focus {
background: lightgreen;
}
</style>
<form>
name: <input type="text" name="name" /><br>
gender: <input type="text" name="gender" />
</form>