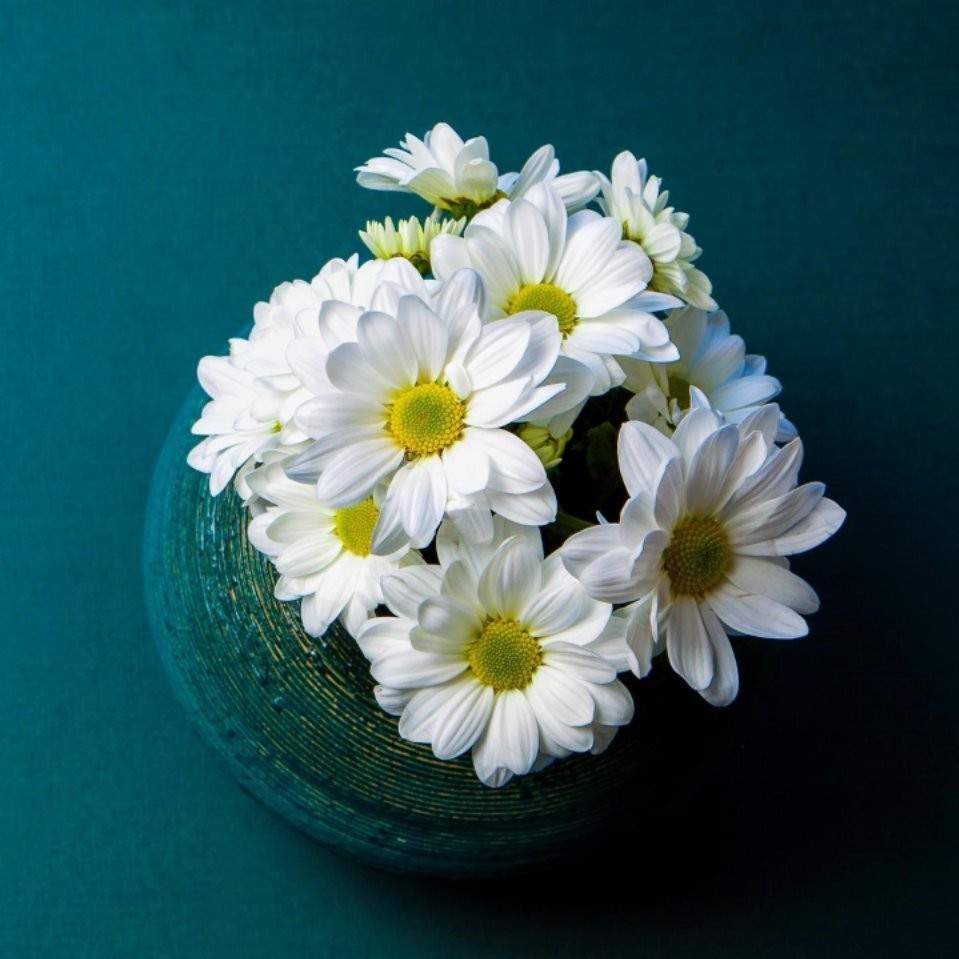
Correction status:qualified
Teacher's comments:写的没什么问题,一定要多练!
. 在单引号中,任何特殊字符都会按原样输出
<?php
$var = 'this is a string!';
echo 'evan $var php.cn';
<?php
$var = "this is a string!";
echo "$string var evan 888、\'、\";
. 以上两者主要区别:
1、 双引号会替换变量的值,而单引号会把它当做字符串输出。
2、对于转义的支持
3、由于双引号中的字符串需要检测是否含有$符号修饰的变量,因此从理论上讲,单引号是比较快的。
. 效果与使用双引号一致
<?php
$var = 'this is string';
echo <<<"HERE"
<h2>$var 888 evan</h2>
HERE
. 效果相当于使用单引号来定义的字符串
<?php
$var = 'this is string';
echo<<<'NOW'
<div id="now-id"class="now">$var</div>
NOW;
$str='php.cn';
$str1='欢迎来到';
$str2='学习';
printf($str1.$str .$str2);
// 输出“欢迎来到php.cn学习”
<?php
$table='users'; //表名
$limit=10; //返回条目
vprintf('SELECT * FROM `%s` LIMIT %d', [$table, $limit]);
//输出:SELECT * FROM `users` LIMIT 10
<?php
$table = 'users'; //表名
$limit = 10; //返回条目
$sql = sprintf('SELECT * FROM `%s` LIMIT %d', $table, $limit);
//输出:SELECT * FROM `users` LIMIT 10
<?php
$table='users'; //表名
$limit=10; //返回条目
$sql = sprintf('SELECT * FROM `%s` LIMIT %d', [$table, $limit]);
//输出:SELECT * FROM `users` LIMIT 10
<?php
echo file_put_contents("sites.txt","我"); //默认覆盖写入,返回字符长度
// 使用 FILE_APPEND 标记,可以在文件末尾追加内容
// LOCK_EX 标记可以防止多人同时写入
echo file_put_contents("sites.txt","要学习", FILE_APPEND | LOCK_EX);
<?php
$arr=['学生1','学生2','学生3','学生4','学生5','学生6'];
//将数组合为字符串,每个用“|”号分开
echo implode('|', $arr);
echo '
';
echo join('|', $arr);
// 输出:
//学生1|学生2|学生3|学生4|学生5|学生6
//学生1|学生2|学生3|学生4|学生5|学生6
//两个函数输出结果相同
<?php
print_r(explode('|','学生1|学生2|学生3|学生4|学生5|学生6'));
// 返回数组:Array ( [0] => 学生1 [1] => 学生2 [2] => 学生3 [3] => 学生4 [4] => 学生5 [5] => 学生6 )
<?php
echo substr('ABCDEFG', 0), '
'; //输出全部
echo substr('ABCDEFG', 1), '
'; //输出BCDEFG
echo substr('ABCDEFG', 3), '
'; //输出DEFG
echo substr('ABCDEFG', 3,2), '
'; //输出DE(第3位开始取两位)
//注意:UTF8 中文1个字为3个字节
<?php
print_r(str_split('ABCDEF'));
// 输出:Array ( [0] => A [1] => B [2] => C [3] => D [4] => E [5] => F )
print_r(str_split('ABCDEF', 2));
// 输出:Array ( [0] => AB [1] => CD [2] => EF )
<?php
echo file_get_contents("text.txt");
<?php
$str = "Hello World";
echo str_pad($str,20,".");
//填充字符串的右侧,到 20 个字符的新长度
<?php
//把字符串 "Hello world!" 中的字符 "world" 替换成 "Peter":
echo str_replace("world","Peter","Hello world!");
<?php
$str = "Hello World!";
echo $str . "<br>";
echo trim($str,"Hed!");
//移除字符串左侧的字符("Hello" 中的 "He"以及 "World" 中的 "d!")
<?php
echo strpos("I love php, I love php too!","php");
//查找 "php" 在字符串中第一次出现的位置,输出 7;
<?php
echo strstr("Hello world!","world"); // 输出 world!
//查找 "world" 在 "Hello world!" 中是否存在,如果是,返回该字符串及后面剩余部分:
//输出 world