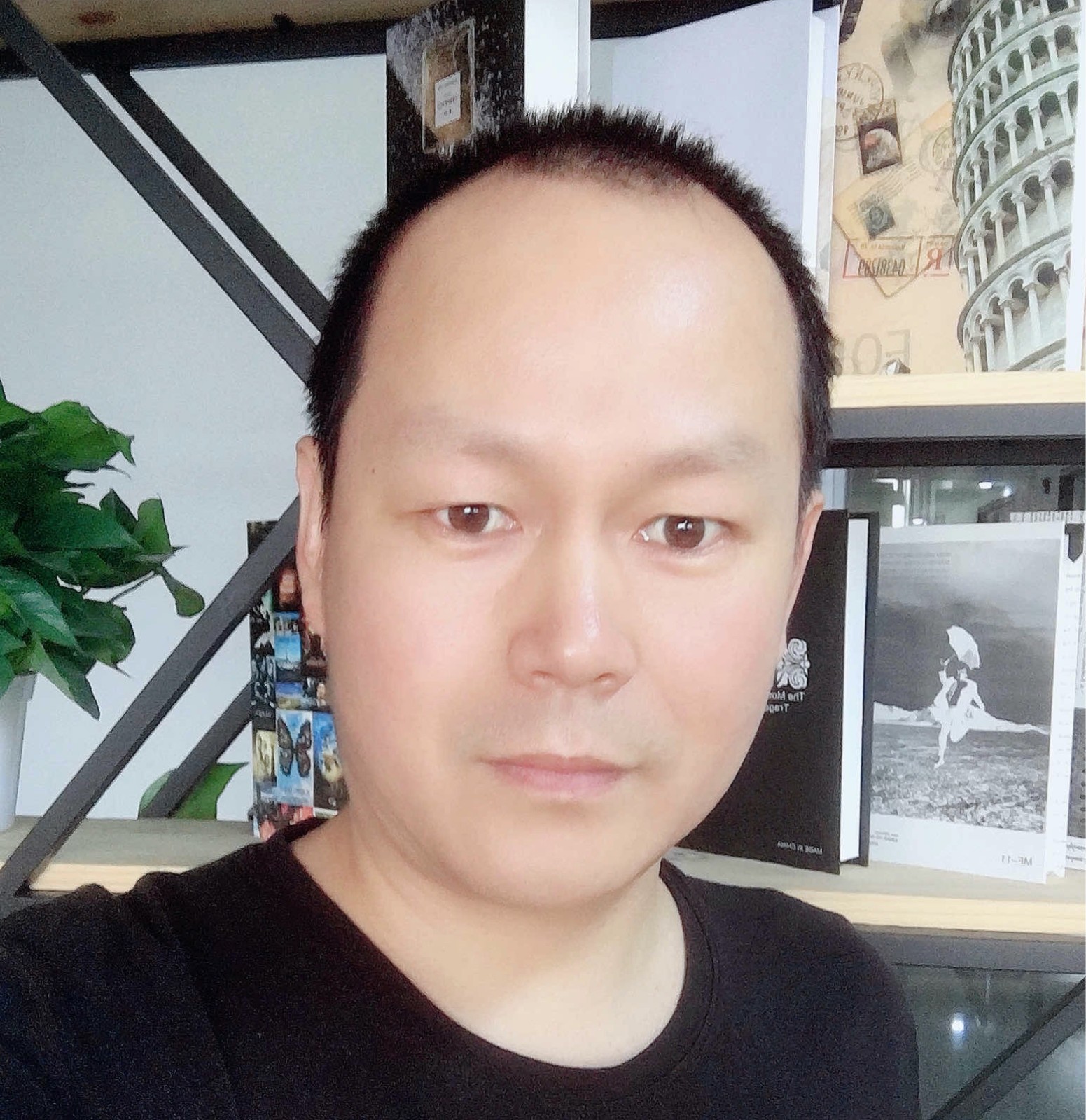
Correction status:qualified
Teacher's comments:不错, 分页效果棒
<?php
return [
'type' => $type ?? 'mysql',
'host' => $host ?? 'php.edu',
'dbname' => $dbname ?? 'first',
'charset' => $charset ?? 'utf8',
'port' => $port ?? '3306',
'username' => $username ?? 'root',
'password' => $password ?? 'root',
];
<?php
$config = require "config.php";
extract($config);
// echo $type,$host;
// $dsn = 'mysql:host=php.edu;dbname=first;charset=utf8;port=3306';
$dsn = sprintf('%s:host=%s;dbname=%s;charset=%s;port=%s',$type,$host,$dbname,$charset,$port);
try {
$pdo = new PDO($dsn,$username,$password);
// 设置结果集获取方式:关联数组
$pdo->setAttribute(PDO::ATTR_DEFAULT_FETCH_MODE,PDO::FETCH_ASSOC);
} catch (Throwable $e) {
exit($e->getMessage());
} catch (PDOException $e) {
exit($e->getMessage());
}
// var_dump($pdo);
<?php
// 1. 连接数据库
require "connect.php";
// 2. 获取分页的两个已知条件
// 2.1. 每页显示的记录数量
$num = 8;
// 2.2 获取当前页数(通过url传输 p ),默认为1,p=1
$page = $_GET['p'] ?? 1;
// 3. 计算偏移量
$offset = ($page - 1) * $num;
// 4. 获取分页数据
$sql = "SELECT * FROM `student` LIMIT {$num} OFFSET {$offset}";
$users = $pdo->query($sql)->fetchAll();
// print_r($result);
// 5. 计算总页数
$sql = "SELECT CEIL(COUNT(`id`)/{$num}) AS `total` FROM `student`";
$pages = $pdo->query($sql)->fetch()['total'];
// var_dump($pages);
// echo $pages;
<?php
// 引入分页数据
require "page-data.php";
?>
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>数据分页</title>
<link rel="stylesheet" href="style.css">
</head>
<body>
<!-- 显示数据表的信息 -->
<table>
<caption><h2>用户信息表<h2></caption>
<thead>
<tr>
<th>id</th>
<th>用户名</th>
<th>性别</th>
<th>年龄</th>
<th>联系电话</th>
<th>操作</th>
</tr>
</thead>
<tbody>
<!-- 使用模板语法循环每个用户信息 -->
<?php foreach ($users as $user): ?>
<tr>
<!-- 使用短标签简化变量的显示 -->
<td><?=$user['id']?></td>
<td><?=$user['username']?></td>
<td><?=$user['sex']?></td>
<td><?php echo $user['age']; ?></td>
<td><?php echo $user['tel']; ?></td>
<td>
<!-- 点击编辑时跳转到一个编辑页面,通过GET方式 -->
<button onclick="location.href='handle.php?action=edit&id=<?=$user['id']?>'">编辑</button>
<!-- 点击删除时,调用del()函数 -->
<button onclick="del(<?=$user['id']?>)">删除</button>
</td>
</tr>
<?php endforeach ?>
</tbody>
</table>
<!-- 分页条 -->
<div>
<!-- 2. 首页 -->
<a href="<?=$_SERVER['PHP_SELF'].'?p=1'?>">首页</a>
<!-- 3.上一页 -->
<?php
$prev = $page - 1;
// 当前页数为第一页时就不再前移
if ($page == 1) $prev = 1;
?>
<!-- 当前页为第一页时,不显示上一页 -->
<?php if ($page != 1) : ?>
<a href="<?=$_SERVER['PHP_SELF'].'?p='.$prev?>">上一页</a>
<?php endif ?>
<!-- 1. 分页条显示数量 -->
<!-- 1.1 小于10时 -->
<?php if ($page <= 10) : ?>
<?php for($i=1; $i<=10; $i++): ?>
<!-- 点击分页条时跳转 -->
<?php $jump = sprintf('%s?p=%s',$_SERVER['PHP_SELF'],$i); ?>
<!-- 当前页码高亮 -->
<?php
// 当前页数和url中的参数p 相等时高亮
$active = ($i == $page) ? 'active' : null;
?>
<a href="<?=$jump?>" class="<?=$active?>"><?=$i?></a>
<?php endfor ?>
<a>...</a>
<!-- 显示最后两页,这里不需要加class,因为后面还会有判断 -->
<a href="<?=sprintf('%s?p=%s',$_SERVER['PHP_SELF'],$pages-1)?>"><?=$pages-1?></a>
<a href="<?=sprintf('%s?p=%s',$_SERVER['PHP_SELF'],$pages)?>"><?=$pages?></a>
<?php endif ?>
<!-- 1.2 大于10且小于最后10页时 -->
<?php if ($page > 10 && $page<$pages-10) : ?>
<!-- 显示1、2 + 左3 + 当前 + 右3 + 最后两页 -->
<!-- 显示1、2 -->
<a href="<?=sprintf('%s?p=1',$_SERVER['PHP_SELF'])?>">1</a>
<a href="<?=sprintf('%s?p=2',$_SERVER['PHP_SELF'])?>">2</a>
<a>...</a>
<!-- 显示左3、当前、右3 -->
<?php for ($i=$page-3;$i<=$page+3;$i++) : ?>
<?php $jump = sprintf('%s?p=%s',$_SERVER['PHP_SELF'],$i); ?>
<?php $active = ($i == $page) ? 'active' : null; ?>
<a href="<?=$jump?>" class="<?=$active?>"><?=$i?></a>
<?php endfor ?>
<a>...</a>
<!-- 显示最后两页 -->
<a href="<?=sprintf('%s?p=%s',$_SERVER['PHP_SELF'],$pages-1)?>"><?=$pages-1?></a>
<a href="<?=sprintf('%s?p=%s',$_SERVER['PHP_SELF'],$pages)?>"><?=$pages?></a>
<?php endif ?>
<!-- 1.3 大于最后10页时 -->
<!-- 显示1、2、最后10页 -->
<?php if ($page>=$pages-10) : ?>
<!-- 显示1、2 -->
<a href="<?=sprintf('%s?p=1',$_SERVER['PHP_SELF'])?>">1</a>
<a href="<?=sprintf('%s?p=2',$_SERVER['PHP_SELF'])?>">2</a>
<a>...</a>
<!-- 显示最后10页 -->
<?php for ($i=$pages-10;$i<=$pages;$i++) :?>
<?php $jump = sprintf('%s?p=%s',$_SERVER['PHP_SELF'],$i); ?>
<?php $active = ($i == $page) ? 'active' : null; ?>
<a href="<?=$jump?>" class="<?=$active?>"><?=$i?></a>
<?php endfor ?>
<?php endif ?>
<!-- 4.尾页 -->
<a href="<?=$_SERVER['PHP_SELF'].'?p='.$pages?>">尾页</a>
<!-- 5.下一页 -->
<?php
$next = $page + 1;
// 下一页页数大于等于总页数时,不再后移
if ($next >= $pages) $next=$pages;
?>
<!-- 当前页是最后一页时,不显示下一页 -->
<?php if ($page != $pages) : ?>
<a href="<?=$_SERVER['PHP_SELF'].'?p='.$next?>">下一页</a>
<?php endif ?>
</div>
<script>
// del()函数:点击确定时跳转到handle.php进行数据删除操作
function del(id){
var isDelete = confirm('此操作不可恢复,确定删除吗?')
if (isDelete) {
location.href = 'handle.php?action=delete&id='+id;
}else{
return false;
}
}
</script>
</body>
</html>
<?php
// 连接数据库
require "connect.php";
$action = $_GET['action'];
$id = $_GET['id'];
switch ($action)
{
// 点击编辑按钮时
case 'edit' :
// 加载渲染表单
include "edit.php";
break;
// 在edit.php编辑表单中,提交新编辑的数据过来时,执行更新操作
case 'doedit' :
$sql = "UPDATE `student` SET `username`=?, `sex`=?, `age`=?, `tel`=? WHERE `id`=?";
$stmt = $pdo->prepare($sql);
// 新编辑的数据以POST的方式提交过来
if(!empty($_POST))
$stmt->execute([$_POST['username'],$_POST['sex'],$_POST['age'],$_POST['tel'],$id]);
if($stmt->rowCount()==1)
{
echo "
<script>
alert('更新信息成功');
location.href = 'page-list.php';
</script>
";
}
break;
// 点击删除按钮并确定时,执行删除操作
case 'delete' :
$sql = "DELETE FROM `student` WHERE `id`=?";
$stmt = $pdo->prepare($sql);
$stmt->execute([$id]);
if( $stmt->rowCount() == 1 )
{
echo "
<script>
alert('删除信息成功');
location.href = 'page-list.php';
</script>
";
}
break;
}
<?php
// 通过GET传过来的id,将用户信息取到
$sql = "SELECT * FROM `student` WHERE `id`={$id}";
$user = $pdo->query($sql)->fetch();
?>
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<link rel="stylesheet" href="edit.css">
<title>用户编辑</title>
</head>
<body>
<h2>用户信息编辑</h2>
<table>
<caption><h3>原始信息如下:<h3></caption>
<thead>
<tr>
<td>id</td>
<td>用户名</td>
<td>性别</td>
<td>年龄</td>
<td>联系电话</td>
</tr>
</thead>
<tbody>
<tr>
<td><?=$user['id']?></td>
<td><?=$user['username']?></td>
<td><?=$user['sex']?></td>
<td><?=$user['age']?></td>
<td><?=$user['tel']?></td>
</tr>
</tbody>
</table>
<hr>
<h3>请编辑您的要修改的信息:</h3>
<form action="<?=$_SERVER['PHP_SELF'].'?action=doedit&id='.$id?>" method="POST">
<div>
<label for="username">用户名:</label>
<input type="text" name="username" id="username">
</div>
<div>
<label for="male">性别:</label>
<div>
<input type="radio" name="sex" id="male" value="男">男
<input type="radio" name="sex" id="female" value="女">女
</div>
</div>
<div>
<label for="age">年龄:</label>
<input type="text" name="age" id="age">
</div>
<div>
<label for="tel">电话:</label>
<input type="tel" name="tel" id="tel">
</div>
<button>保存</button>
</form>
</body>
</html>
* {
margin: 0;
padding: 0;
box-sizing: border-box;
}
/* 表格样式 */
table {
border: 1px solid black;
border-collapse: collapse;
text-align: center;
width: 800px;
margin: auto;
}
th,
td {
border: 1px solid black;
line-height: 50px;
}
table h2 {
padding: 10px 0;
}
th {
background-color: lightcoral;
}
tr:hover {
background-color: lightcyan;
}
button {
padding: 5px 15px;
background-color: rgb(9, 9, 71);
color: white;
border-radius: 5px;
outline: none;
border: none;
}
button:hover {
background-color: rgb(77, 77, 163);
cursor: pointer;
}
/* 分页条样式 */
div {
text-align: center;
margin-top: 30px;
}
div > a {
text-decoration: none;
color: black;
padding: 5px 10px;
vertical-align: middle;
margin: 0 5px;
border-radius: 5px;
}
/* 当前页码高亮 */
.active {
background-color: red;
color: white;
}
* {
margin: 0;
padding: 0;
box-sizing: border-box;
}
body {
display: flex;
flex-flow: column nowrap;
align-items: center;
}
h2 {
padding: 20px 0;
border-bottom: 1px solid black;
}
table {
border: 1px solid black;
border-collapse: collapse;
width: 500px;
}
table td {
border: 1px solid black;
line-height: 50px;
text-align: center;
}
table > caption > h3 {
padding: 10px 0;
}
form > div {
display: grid;
grid-template-columns: 80px 300px;
grid-template-rows: 30px;
margin: 10px 0;
}
body > h3 {
padding-bottom: 10px;
margin-top: 50px;
}
form {
text-align: center;
}
form > div:nth-of-type(2) input {
margin: 0 20px;
}
button {
width: 100px;
padding: 5px 0;
outline: none;
}
button:hover {
cursor: pointer;
background-color: black;
color: white;
}