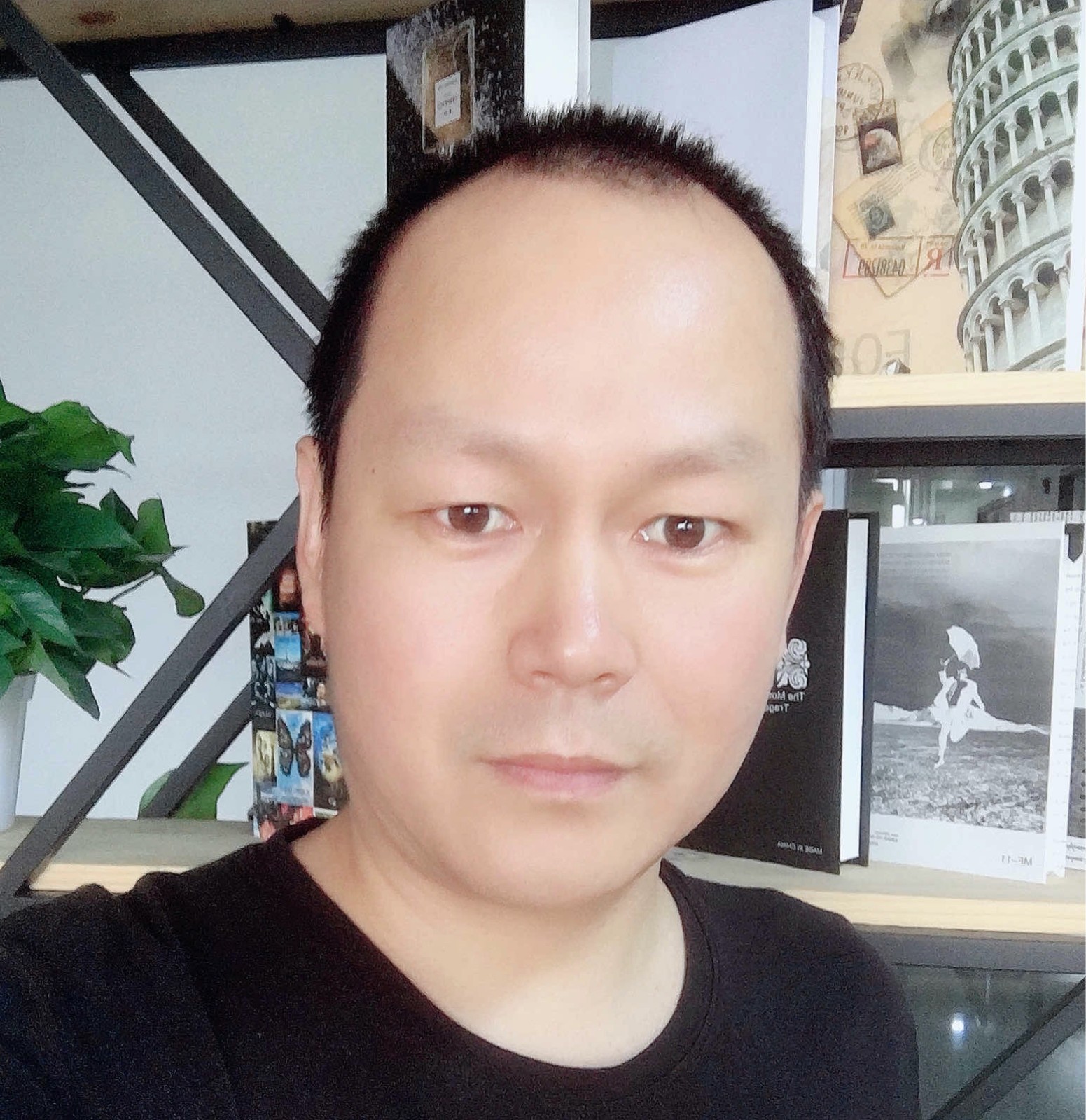
Correction status:qualified
Teacher's comments:pdo操作是目前 的主流
序号 | 步骤 | 描述 |
---|---|---|
1 | 连接数据库 | 创建$mysql或者$pdo对象 |
2 | 操作数据表 | 对数据库中数据增(INSERT)、删(DELETE)、查(SELECT)、改(UPDATE)操作 |
3 | 关闭数据库连接 | 也就是销毁数据库对象 |
操作数据表主要分为读和写
序号 | 参数 | 描述 |
---|---|---|
1 | type | 数据库类型 |
2 | host | 数据库主机名 |
3 | dbname | 数据库名 |
4 | username | 用户名 |
5 | password | 用户密码 |
6 | charset | 默认编码集 |
7 | port | 默认端口号 |
<?php
// 数据库类型
define('DB_TYPE', 'mysql');
// 数据库主机名
define('DB_HOST', 'localhost');
// 数据库名
define('DB_NAME', 'user');
// 数据库用户名
define('DB_USER', 'root');
// 数据库密码
define('DB_PSD', 'root');
// 数据库默认端口号
define('DB_PORT', 3306);
// 数据库编码集
define('DB_CHARSET', 'utf8');
// 定义pdo中dsn的主机名,数据库型号,数据库名和编码方式
define('DB_DSN', DB_TYPE . ':host=' . DB_HOST . ';dbname=' . DB_NAME . ';charset=' . DB_CHARSET);
<?php
// 引入配置文件
require __DIR__ . "/config.php";
// 1.连接数据库
$mysql = new mysqli(DB_HOST, DB_USER, DB_PSD, DB_NAME);
// 检测错误
if ($mysql->connect_errno) die('Connect_errno:' . $mysql->connect_error);
// 设置编码集
$mysql->set_charset(DB_CHARSET);
<?php
// 新增操作(插入)用预处理方式
$sql = 'INSERT `apple` SET `username`=? ,`password`=?, `sex`=?';
// 准备执行(转为statement对象)
$stmt = $mysql->prepare($sql);
// 绑定占位符
$stmt->bind_param('sss', $username, $password, $sex);
// 变量赋值
$username = '小孔';
$password = sha1(123);
$sex = '男';
// 执行
$stmt->execute();
// 返回受影响的行数
printf('新增了 %s 条记录,新增的id为 %d', $stmt->affected_rows, $stmt->insert_id);
// 也可以通过遍历一个二维数组实现一次性插入多条数据
$as = [
['username' => '小小', 'password' => sha1(123), 'sex' => '男'],
['username' => '小康', 'password' => sha1(222), 'sex' => '女'],
['username' => '小于', 'password' => sha1(333), 'sex' => '女'],
['username' => '小文', 'password' => sha1(444), 'sex' => '男'],
['username' => '小群', 'password' => sha1(555), 'sex' => '男'],
];
// 遍历数组
foreach ($as as $a) {
// 展开数组
extract($a);
// 判断是否执行成功
if ($stmt->execute()) {
printf('新增了 %s 条数据,新增的id为 %d<br>', $stmt->affected_rows, $stmt->insert_id);
} else {
exit(sprintf('新增失败,%d:%s', $stmt->connect_errno, $stmt->connect_error));
}
}
<?php
// 更新操作
$sql = "UPDATE `apple` SET `username`=?, `password`=?, `sex`=? WHERE `id`=?";
// 准备执行(转为statement对象)
$stmt = $mysql->prepare($sql);
// 绑定占位符
$stmt->bind_param('sssi', $username, $password, $sex, $id);
$a = ['username' => '小卡', 'password' => sha1(111), 'sex' => '女', 'id' => 25];
// 展开数组
extract($a);
if ($stmt->execute()) {
printf('更新成功,更新了 %s 条数据', $stmt->affected_rows);
} else {
exit(sprintf('更新失败,%d:%s', $stmt->connect_errno, $stmt->connect_error));
}
<?php
// 删除操作
$sql = "DELETE FROM `apple` WHERE `id`=?";
// 准备执行(转为statement对象)
$stmt = $mysql->prepare($sql);
// 绑定占位符
$stmt->bind_param('i', $id);
$id = 5;
// 执行
if ($stmt->execute()) {
printf('删除成功,删除了 %s 条数据', $stmt->affected_rows);
} else {
exit(sprintf('删除失败,%d:%s', $stmt->connect_errno, $stmt->connect_error));
}
<?php
$sql = "SELECT * FROM `apple` WHERE `id`>?";
// 准备执行(转为statement对象)
$stmt = $mysql->prepare($sql);
// 绑定占位符
$stmt->bind_param('i', $id);
$id = 20;
// 执行
$stmt->execute();
if ($stmt->affected_rows === 0) exit('查询失败');
// 获取结果集
$res = $stmt->get_result();
打印结果集
print_r($res->fetch_assoc());
print_r($res->fetch_assoc());
// 遍历结果
// 1.fetch_assoc()+while()获取结果集
while ($a = $res->fetch_assoc()) {
printf('id= %d 姓名= %s 性别= %s <br>', $a['id'], $a['username'], $a['sex']);
}
// 2.fetch_all()+foreach()获取结果集
foreach ($res->fetch_all(MYSQLI_ASSOC) as $a) {
printf('序号= %d:姓名= %s 性别= %s<br>', $a['id'], $a['username'], $a['sex']);
}
// 3.bind_result()+fetch()+while()将结果集的字段与一个变量绑定
$sql = "SELECT `id` , `username` , `sex` FROM `apple` WHERE `id`>? ";
// 准备执行(转为statement对象)
$stmt = $mysql->prepare($sql);
$stmt->bind_param('i', $id);
$id = 23;
$stmt->execute() or die($stmt->error);
// 将结果字段绑定到变量中(跳过了结果集)
$stmt->bind_result($id, $name, $sex);
// 用while()遍历
while ($stmt->fetch()) {
printf('序号= %d :姓名= %s 性别= %s <br>', $id, $name, $sex);
}
fetch_assoc()+while()获取结果集
2.fetch_all()+foreach()获取结果集
3.bind_result()+fetch()+while()将结果集的字段与一个变量绑定
<?php
$mysql->close();
<?php
// 引入配置文件
require __DIR__ . '/config.php';
// 连接数据库
try {
$pdo = new PDO(DB_DSN, DB_USER, DB_PSD);
} catch (PDOException $e) {
echo $e->getMessage();
}
// 设置结果集默认默认获取模式
$pdo->setAttribute(PDO::ATTR_DEFAULT_FETCH_MODE, PDO::FETCH_ASSOC);
<?php
// 新增(插入)操作
$sql = "INSERT `apple` SET `username`=?, `password`=?, `sex`=?";
// 准备执行
$stmt = $pdo->prepare($sql);
// 绑定占位符
// PDO::PARAM_STR, 30 确认数据类型和字符位数
$stmt->bindParam(1, $name, PDO::PARAM_STR, 30);
$stmt->bindParam(2, $password, PDO::PARAM_STR, 40);
$stmt->bindParam(3, $sex, PDO::PARAM_STR, 10);
// 变量赋值
$name = '小环';
$password = sha1(222);
$sex = '女';
// bindValue()值绑定,变量的变化实时映射到占位符上,因此每一次都必须重新赋值
// 值绑定可通过execute()实现
if ($stmt->execute(['小话', sha1(333), '男'])) {
// rowCount()获取受影响的行数
// lastInsertId()获取主键id
printf('新增成功 %s 条数据,新增的主键id= %d', $stmt->rowCount(), $pdo->lastInsertId());
}
值绑定
<?php
// 更新操作
$sql = "UPDATE `apple` SET `username`=?, `password`=?, `sex`=? WHERE `id`=?";
// 准备执行
$stmt = $pdo->prepare($sql);
// 值绑定占位符
if ($stmt->execute(['小星星', sha1(333), '男', 28])) {
printf('更新成功 %s 条数据', $stmt->rowCount());
}
<?php
// 删除操作
$sql = "DELETE FROM `apple` WHERE `id`=?";
// 准备执行
$stmt = $pdo->prepare($sql);
// 值绑定占位符
if ($stmt->execute([17])) {
printf('删除成功 %s 条数据', $stmt->rowCount());
}
<?php
$sql = "SELECT `id`, `username`, `sex` FROM `apple` WHERE `id`>?";
// 准备执行
$stmt = $pdo->prepare($sql);
// 值绑定占位符
$stmt->execute([20]);
// 1.通过fetch()+while()获取结果
while ($a = $stmt->fetch()) {
vprintf('<li>序号:%d,姓名= %s 性别= %s</li><br>', $a);
}
// 2.fetchAll()+foreach()获取结果
foreach ($stmt->fetchAll() as $a) {
vprintf('<li>序号:%d,姓名= %s 性别= %s</li><hr>', $a);
}
// 3.bindColumn()+fetch()+while()获取结果
// 将获取结果的字段和变量绑定
$stmt->bindColumn('id', $id);
$stmt->bindColumn('username', $name);
$stmt->bindColumn('sex', $sex);
while ($stmt->fetch()) {
printf('<li>序号:%d,姓名= %s 性别= %s</li><br>', $id, $name, $sex);
}
// 获取记录数量不要用rowCount()获取 用sql语句获取
$sql = "SELECT COUNT(`id`) AS `num` FROM `apple` WHERE `id`>?";
$stmt = $pdo->prepare($sql);
$stmt->execute([20]);
$stmt->bindColumn('num', $num);
$stmt->fetch(PDO::FETCH_BOUND);
printf('满足条件的记录数量:%d', $num);
1.通过fetch()+while()获取结果
2.fetchAll()+foreach()获取结果
3.bindColumn()+fetch()+while()获取结果
4.获取记录数量
1.对于mysqli对象和pdo的方法使用更清楚了
2.对于获取的结果集的多种方法有了更深的理解